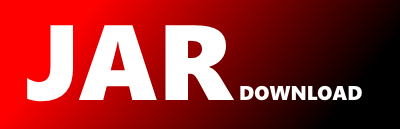
commonMain.com.paoapps.blockedcache.BlockedCollectionCache.kt Maven / Gradle / Ivy
package com.paoapps.blockedcache
import kotlinx.coroutines.flow.MutableStateFlow
import kotlinx.coroutines.sync.Mutex
/**
* Manages a collection of [BlockedCache] instances, each associated with a unique key.
* This class provides a way to dynamically create and retrieve [BlockedCache] instances for different keys
* using a factory function. It ensures that only one cache instance is created per key.
*
* @param K The type of the key used to identify each cache.
* @param T The type of data stored in each cache.
* @property cacheFactory A factory function to create a new [BlockedCache] instance for a given key.
*/
class BlockedCollectionCache(private val cacheFactory: (K) -> BlockedCache) {
private val caches = MutableStateFlow
© 2015 - 2025 Weber Informatics LLC | Privacy Policy