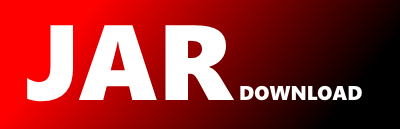
commonMain.com.paoapps.blockedcache.FetchRefreshTrigger.kt Maven / Gradle / Ivy
package com.paoapps.blockedcache
import kotlinx.coroutines.flow.Flow
import kotlinx.coroutines.flow.MutableStateFlow
import kotlinx.coroutines.flow.distinctUntilChanged
import kotlinx.coroutines.flow.flatMapLatest
import kotlin.random.Random
sealed class Fetch {
/**
* Force fetch from network.
* minimumDelay: Minimum delay between fetches in milliseconds. Default is 5 seconds.
*/
data class Force(val minimumDelay: Long = 5000L) : Fetch()
/**
* Fetch from cache if available, otherwise fetch from network.
*/
object Cache : Fetch()
/**
* Fetch from cache if available, otherwise do not fetch.
*/
object NoFetch : Fetch()
}
sealed class FetchRefreshTrigger {
data class FromCache(private val id: String = Random.nextInt().toString()) : FetchRefreshTrigger()
/**
* Every instance has a unique id so the refresh StateFlow sees it as a new value.
* StateFlow does not emit the same value twice if the current value is the same as the new value.
*/
data class Refresh(private val id: String = Random.nextInt().toString()) : FetchRefreshTrigger()
companion object {
fun refreshTriggerFlow(): MutableStateFlow = MutableStateFlow(
FromCache()
)
}
}
fun Flow.createRefreshableFlow(data: suspend (refresh: Boolean) -> Flow): Flow {
return flatMapLatest { trigger ->
val refresh = trigger is FetchRefreshTrigger.Refresh
data(refresh)
}.distinctUntilChanged()
}
fun Flow.createRefreshableFetchFlow(data: (refresh: Fetch) -> Flow) = createRefreshableFetchFlow(default = Fetch.Cache, data = data)
fun Flow.createRefreshableFetchFlow(default: Fetch, data: (refresh: Fetch) -> Flow): Flow =
createRefreshableFlow { data(if (it) Fetch.Force() else default) }
fun MutableStateFlow.refresh() {
value = FetchRefreshTrigger.Refresh()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy