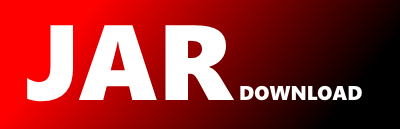
commonMain.com.paoapps.fifi.viewmodel.AbstractViewModel.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of fifi-framework Show documentation
Show all versions of fifi-framework Show documentation
Kotlin Multiplatform Mobile framework for optimal code sharing between iOS and Android.
package com.paoapps.fifi.viewmodel
import com.paoapps.blockedcache.Fetch
import com.paoapps.fifi.log.warn
import com.paoapps.fifi.utils.ActionHandler
import com.paoapps.fifi.utils.flow.FlowAdapter
import com.paoapps.fifi.utils.flow.FlowRefreshTrigger
import com.paoapps.fifi.utils.flow.wrap
import kotlinx.coroutines.flow.Flow
import kotlinx.coroutines.flow.MutableSharedFlow
import kotlinx.coroutines.flow.MutableStateFlow
import kotlinx.coroutines.flow.SharingStarted
import kotlinx.coroutines.flow.StateFlow
import kotlinx.coroutines.flow.asSharedFlow
import kotlinx.coroutines.flow.combine
import kotlinx.coroutines.flow.distinctUntilChanged
import kotlinx.coroutines.flow.flatMapLatest
import kotlinx.coroutines.flow.merge
import kotlinx.coroutines.flow.shareIn
import kotlinx.coroutines.launch
import kotlinx.coroutines.yield
import org.koin.core.component.KoinComponent
abstract class AbstractEvent(val animate: Boolean = false)
object VoidEvent : AbstractEvent()
abstract class AbstractViewModel
© 2015 - 2025 Weber Informatics LLC | Privacy Policy