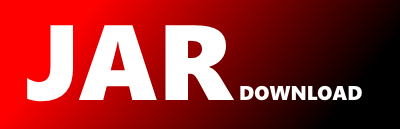
com.parzivail.pswg.features.blasters.BlasterUtil Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pswg Show documentation
Show all versions of pswg Show documentation
Explore the galaxy with Galaxies: Parzi's Star Wars Mod!
package com.parzivail.pswg.features.blasters;
import D;
import I;
import com.parzivail.pswg.container.SwgEntities;
import com.parzivail.pswg.container.SwgParticles;
import com.parzivail.pswg.entity.BlasterBoltEntity;
import com.parzivail.pswg.entity.BlasterIonBoltEntity;
import com.parzivail.pswg.entity.BlasterStunBoltEntity;
import com.parzivail.util.data.PacketByteBufHelper;
import com.parzivail.util.entity.EntityUtil;
import com.parzivail.util.math.MathUtil;
import net.fabricmc.fabric.api.networking.v1.PacketSender;
import net.minecraft.class_1293;
import net.minecraft.class_1294;
import net.minecraft.class_1297;
import net.minecraft.class_1309;
import net.minecraft.class_1657;
import net.minecraft.class_1937;
import net.minecraft.class_2338;
import net.minecraft.class_2388;
import net.minecraft.class_2398;
import net.minecraft.class_243;
import net.minecraft.class_2540;
import net.minecraft.class_310;
import net.minecraft.class_634;
import java.util.ArrayList;
import java.util.function.Consumer;
import java.util.function.Function;
public class BlasterUtil
{
public static void fireBolt(class_1937 world, class_1657 player, class_243 fromDir, float range, Function damage, boolean ignoreWater, Consumer entityInitializer)
{
final var bolt = new BlasterBoltEntity(SwgEntities.Misc.BlasterBolt, player, world, ignoreWater);
entityInitializer.accept(bolt);
bolt.setRange(range);
bolt.setDamageFunction(damage);
world.method_8649(bolt);
}
public static void fireIon(class_1937 world, class_1657 player, float range, boolean ignoreWater, Consumer entityInitializer)
{
final var bolt = new BlasterIonBoltEntity(SwgEntities.Misc.BlasterIonBolt, player, world, ignoreWater);
entityInitializer.accept(bolt);
bolt.setRange(range);
// TODO: ion effects
world.method_8649(bolt);
}
public static void fireStun(class_1937 world, class_1657 player, class_243 fromDir, float range, boolean ignoreWater, Consumer entityInitializer)
{
final var bolt = new BlasterStunBoltEntity(SwgEntities.Misc.BlasterStunBolt, player, world, ignoreWater);
entityInitializer.accept(bolt);
bolt.setRange(range);
world.method_8649(bolt);
var start = new class_243(bolt.method_23317(), bolt.method_23318() + bolt.method_17682() / 2f, bolt.method_23321());
var hit = EntityUtil.raycastEntitiesCone(start, fromDir, 10 / 180f * Math.PI, range, player, new class_1297[] { player });
// TODO: prevent stunning through walls
// TODO: ignore water
for (var e : hit)
{
if (e instanceof class_1309 le)
{
le.method_37222(new class_1293(class_1294.field_5909, 5 * 20, 3, true, false), player);
le.method_37222(new class_1293(class_1294.field_5916, 7 * 20, 0, true, false), player);
le.method_37222(new class_1293(class_1294.field_5919, 4 * 20, 0, true, false), player);
le.method_37222(new class_1293(class_1294.field_5911, 6 * 20, 1, true, false), player);
}
}
}
public static void handleBoltHit(class_310 client, class_634 handler, class_2540 buf, PacketSender responseSender)
{
var pos = PacketByteBufHelper.readVec3d(buf);
var incident = PacketByteBufHelper.readVec3d(buf);
var normal = PacketByteBufHelper.readVec3d(buf);
client.execute(() -> createScorchParticles(client, pos, incident, normal, true));
}
private static void createScorchParticles(class_310 client, class_243 pos, class_243 incident, class_243 normal, boolean energyScorch)
{
var blockPos = new class_2338(MathUtil.floorInt(pos.method_1020(normal.method_1021(0.1f))));
assert client.field_1687 != null;
var offset = 0.005 + 0.0005 * client.field_1687.field_9229.method_43058();
var heatEncodedNormal = normal.method_1021(energyScorch ? 1 : 0.3f);
client.field_1687.method_8406(SwgParticles.SCORCH, pos.field_1352 + normal.field_1352 * offset, pos.field_1351 + normal.field_1351 * offset, pos.field_1350 + normal.field_1350 * offset, heatEncodedNormal.field_1352, heatEncodedNormal.field_1351, heatEncodedNormal.field_1350);
var reflection = MathUtil.reflect(incident, normal);
for (var i = 0; i < 16; i++)
{
var vx = client.field_1687.field_9229.method_43059() * 0.03;
var vy = client.field_1687.field_9229.method_43059() * 0.03;
var vz = client.field_1687.field_9229.method_43059() * 0.03;
var sparkVelocity = reflection.method_1021(0.3f * (client.field_1687.field_9229.method_43058() * 0.5 + 0.5));
client.field_1687.method_8406(SwgParticles.SPARK, pos.field_1352, pos.field_1351, pos.field_1350, sparkVelocity.field_1352 + vx, sparkVelocity.field_1351 + vy, sparkVelocity.field_1350 + vz);
if (i % 3 == 0)
{
var debrisVelocity = reflection.method_1021(0.15f * (client.field_1687.field_9229.method_43058() * 0.5 + 0.5));
client.field_1687.method_8406(new class_2388(class_2398.field_11217, client.field_1687.method_8320(blockPos)), pos.field_1352, pos.field_1351, pos.field_1350, debrisVelocity.field_1352 + vx, debrisVelocity.field_1351 + vy, debrisVelocity.field_1350 + vz);
}
if (i % 2 == 0)
{
var smokeVelocity = reflection.method_1021(0.08f * (client.field_1687.field_9229.method_43058() * 0.5 + 0.5));
client.field_1687.method_8406(class_2398.field_11251, pos.field_1352, pos.field_1351, pos.field_1350, smokeVelocity.field_1352 + vx, smokeVelocity.field_1351 + vy, smokeVelocity.field_1350 + vz);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy