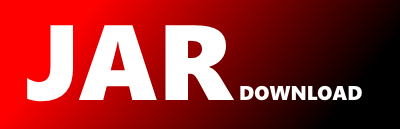
com.parzivail.util.block.AccumulatingBlock Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pswg Show documentation
Show all versions of pswg Show documentation
Explore the galaxy with Galaxies: Parzi's Star Wars Mod!
package com.parzivail.util.block;
import net.minecraft.block.*;
import net.minecraft.class_10;
import net.minecraft.class_1750;
import net.minecraft.class_1922;
import net.minecraft.class_1936;
import net.minecraft.class_2246;
import net.minecraft.class_2248;
import net.minecraft.class_2338;
import net.minecraft.class_2350;
import net.minecraft.class_259;
import net.minecraft.class_265;
import net.minecraft.class_2680;
import net.minecraft.class_2689;
import net.minecraft.class_2741;
import net.minecraft.class_2758;
import net.minecraft.class_3726;
import net.minecraft.class_4538;
import net.minecraft.class_4970;
import org.jetbrains.annotations.Nullable;
import java.util.function.Function;
public class AccumulatingBlock extends class_2248
{
public static final int maxTotalLayers = 8;
public static final int maxPathfindingLayers = 5;
public static final class_2758 LAYERS = class_2741.field_12536;
protected static final class_265[] LAYERS_TO_SHAPE = new class_265[] {
class_259.method_1073(),
class_2248.method_9541(0.0, 0.0, 0.0, 16.0, 2.0, 16.0),
class_2248.method_9541(0.0, 0.0, 0.0, 16.0, 4.0, 16.0),
class_2248.method_9541(0.0, 0.0, 0.0, 16.0, 6.0, 16.0),
class_2248.method_9541(0.0, 0.0, 0.0, 16.0, 8.0, 16.0),
class_2248.method_9541(0.0, 0.0, 0.0, 16.0, 10.0, 16.0),
class_2248.method_9541(0.0, 0.0, 0.0, 16.0, 12.0, 16.0),
class_2248.method_9541(0.0, 0.0, 0.0, 16.0, 14.0, 16.0),
class_2248.method_9541(0.0, 0.0, 0.0, 16.0, 16.0, 16.0)
};
private final Function fullBlockState;
public AccumulatingBlock(class_4970.class_2251 settings)
{
this(settings, null);
this.method_9590(this.field_10647.method_11664().method_11657(LAYERS, 1));
}
public AccumulatingBlock(class_4970.class_2251 settings, Function fullBlockState)
{
super(settings);
this.fullBlockState = fullBlockState;
}
@Override
public boolean method_9516(class_2680 state, class_1922 world, class_2338 pos, class_10 type)
{
if (type == class_10.field_50)
return state.method_11654(LAYERS) < maxPathfindingLayers;
return false;
}
@Override
public class_265 method_9530(class_2680 state, class_1922 world, class_2338 pos, class_3726 context)
{
return LAYERS_TO_SHAPE[state.method_11654(LAYERS)];
}
@Override
public class_265 method_9549(class_2680 state, class_1922 world, class_2338 pos, class_3726 context)
{
return LAYERS_TO_SHAPE[state.method_11654(LAYERS) - 1];
}
@Override
public class_265 method_25959(class_2680 state, class_1922 world, class_2338 pos)
{
return LAYERS_TO_SHAPE[state.method_11654(LAYERS)];
}
@Override
public class_265 method_26159(class_2680 state, class_1922 world, class_2338 pos, class_3726 context)
{
return LAYERS_TO_SHAPE[state.method_11654(LAYERS)];
}
@Override
public boolean method_9526(class_2680 state)
{
return true;
}
@Override
public boolean method_9558(class_2680 state, class_4538 world, class_2338 pos)
{
var blockState = world.method_8320(pos.method_10074());
return class_2248.method_9501(blockState.method_26220(world, pos.method_10074()), class_2350.field_11036) || blockState.method_27852(this) && blockState.method_11654(LAYERS) == maxTotalLayers;
}
@Override
public class_2680 method_9559(class_2680 state, class_2350 direction, class_2680 neighborState, class_1936 world, class_2338 pos, class_2338 neighborPos)
{
return !state.method_26184(world, pos)
? class_2246.field_10124.method_9564()
: super.method_9559(state, direction, neighborState, world, pos, neighborPos);
}
@Override
public boolean method_9616(class_2680 state, class_1750 context)
{
int i = state.method_11654(LAYERS);
if (context.method_8041().method_31574(this.method_8389()) && i < maxTotalLayers)
{
if (context.method_7717())
return context.method_8038() == class_2350.field_11036;
return true;
}
return i == 1;
}
@Nullable
@Override
public class_2680 method_9605(class_1750 ctx)
{
var blockState = ctx.method_8045().method_8320(ctx.method_8037());
if (blockState.method_27852(this))
{
int i = blockState.method_11654(LAYERS);
if (i + 1 >= maxTotalLayers && fullBlockState != null)
return fullBlockState.apply(ctx);
return blockState.method_11657(LAYERS, Math.min(maxTotalLayers, i + 1));
}
return super.method_9605(ctx);
}
@Override
protected void method_9515(class_2689.class_2690 builder)
{
super.method_9515(builder);
builder.method_11667(LAYERS);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy