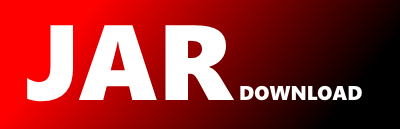
com.parzivail.util.client.render.StatelessWaterRenderer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pswg Show documentation
Show all versions of pswg Show documentation
Explore the galaxy with Galaxies: Parzi's Star Wars Mod!
package com.parzivail.util.client.render;
import ;
import F;
import Z;
import com.parzivail.util.ParziUtil;
import net.fabricmc.api.EnvType;
import net.fabricmc.api.Environment;
import net.fabricmc.fabric.api.resource.SimpleResourceReloadListener;
import net.minecraft.class_1058;
import net.minecraft.class_1088;
import net.minecraft.class_1920;
import net.minecraft.class_2246;
import net.minecraft.class_2350;
import net.minecraft.class_243;
import net.minecraft.class_2960;
import net.minecraft.class_310;
import net.minecraft.class_3300;
import net.minecraft.class_3302;
import net.minecraft.class_3532;
import net.minecraft.class_3695;
import net.minecraft.class_4588;
import org.joml.Matrix4f;
import java.util.concurrent.CompletableFuture;
import java.util.concurrent.Executor;
@Environment(EnvType.CLIENT)
public class StatelessWaterRenderer implements SimpleResourceReloadListener
{
public static final class_2960 ID = ParziUtil.id("stateless_water_renderer");
public static final StatelessWaterRenderer INSTANCE = new StatelessWaterRenderer();
private final class_1058[] waterSprites = new class_1058[2];
private class_1058 waterOverlaySprite;
private StatelessWaterRenderer()
{
}
@Override
public class_2960 getFabricId()
{
return ID;
}
@Override
public CompletableFuture method_25931(class_3302.class_4045 helper, class_3300 manager, class_3695 loadProfiler, class_3695 applyProfiler, Executor loadExecutor, Executor applyExecutor)
{
return load(manager, loadProfiler, loadExecutor).thenCompose(helper::method_18352).thenCompose(
(o) -> apply(o, manager, applyProfiler, applyExecutor)
);
}
@Override
public CompletableFuture load(class_3300 manager, class_3695 profiler, Executor executor)
{
return CompletableFuture.completedFuture(null);
}
@Override
public CompletableFuture apply(Void data, class_3300 manager, class_3695 profiler, Executor executor)
{
this.waterSprites[0] = class_310.method_1551().method_1554().method_4743().method_3335(class_2246.field_10382.method_9564()).method_4711();
this.waterSprites[1] = class_1088.field_5391.method_24148();
this.waterOverlaySprite = class_1088.field_5388.method_24148();
return CompletableFuture.completedFuture(null);
}
public boolean render(class_1920 world, int waterColor, class_4588 vertexConsumer, Matrix4f mat, boolean useOverlaySprite,
boolean renderUp, boolean renderUpInner, boolean renderDown, boolean renderN, boolean renderS, boolean renderE, boolean renderW,
float levelNE, float levelNW, float levelSE, float levelSW,
class_243 fluidVelocity, int lightmap, int lightmapDown)
{
if (!renderUp && !renderDown && !renderE && !renderW && !renderN && !renderS)
return false;
else
{
var sprites = this.waterSprites;
var colorR = (float)(waterColor >> 16 & 0xFF) / 255.0F;
var colorG = (float)(waterColor >> 8 & 0xFF) / 255.0F;
var colorB = (float)(waterColor & 0xFF) / 255.0F;
var renderedAnything = false;
var lightDown = world.method_24852(class_2350.field_11033, true);
var lightUp = world.method_24852(class_2350.field_11036, true);
var lightNorth = world.method_24852(class_2350.field_11043, true);
var lightWest = world.method_24852(class_2350.field_11039, true);
var bottomY = renderDown ? 0.001F : 0.0F;
if (renderUp)
{
renderedAnything = true;
levelNW -= 0.001F;
levelSW -= 0.001F;
levelSE -= 0.001F;
levelNE -= 0.001F;
float u0;
float u1;
float u2;
float u3;
float v0;
float v1;
float v2;
float v3;
if (fluidVelocity.field_1352 == 0.0 && fluidVelocity.field_1350 == 0.0)
{
var sprite = sprites[0];
u0 = sprite.method_4580(0.0F);
v0 = sprite.method_4570(0.0F);
u1 = u0;
v1 = sprite.method_4570(16.0F);
u2 = sprite.method_4580(16.0F);
v2 = v1;
u3 = u2;
v3 = v0;
}
else
{
var sprite = sprites[1];
var fluidAngle = (float)class_3532.method_15349(fluidVelocity.field_1350, fluidVelocity.field_1352) - (float)(Math.PI / 2);
var fluidDy = class_3532.method_15374(fluidAngle) * 0.25F;
var fluidDx = class_3532.method_15362(fluidAngle) * 0.25F;
var offset = 8.0F;
u0 = sprite.method_4580(offset + (-fluidDx - fluidDy) * 16.0F);
v0 = sprite.method_4570(offset + (-fluidDx + fluidDy) * 16.0F);
u1 = sprite.method_4580(offset + (-fluidDx + fluidDy) * 16.0F);
v1 = sprite.method_4570(offset + (fluidDx + fluidDy) * 16.0F);
u2 = sprite.method_4580(offset + (fluidDx + fluidDy) * 16.0F);
v2 = sprite.method_4570(offset + (fluidDx - fluidDy) * 16.0F);
u3 = sprite.method_4580(offset + (fluidDx - fluidDy) * 16.0F);
v3 = sprite.method_4570(offset + (-fluidDx - fluidDy) * 16.0F);
}
var avgU = (u0 + u1 + u2 + u3) / 4.0F;
var avgV = (v0 + v1 + v2 + v3) / 4.0F;
float coverageProportion = sprites[0].method_23842();
u0 = class_3532.method_16439(coverageProportion, u0, avgU);
u1 = class_3532.method_16439(coverageProportion, u1, avgU);
u2 = class_3532.method_16439(coverageProportion, u2, avgU);
u3 = class_3532.method_16439(coverageProportion, u3, avgU);
v0 = class_3532.method_16439(coverageProportion, v0, avgV);
v1 = class_3532.method_16439(coverageProportion, v1, avgV);
v2 = class_3532.method_16439(coverageProportion, v2, avgV);
v3 = class_3532.method_16439(coverageProportion, v3, avgV);
var r = lightUp * colorR;
var g = lightUp * colorG;
var b = lightUp * colorB;
this.vertex(mat, vertexConsumer, 0.0, levelNW, 0.0, r, g, b, u0, v0, lightmap);
this.vertex(mat, vertexConsumer, 0.0, levelSW, 1.0, r, g, b, u1, v1, lightmap);
this.vertex(mat, vertexConsumer, 1.0, levelSE, 1.0, r, g, b, u2, v2, lightmap);
this.vertex(mat, vertexConsumer, 1.0, levelNE, 0.0, r, g, b, u3, v3, lightmap);
if (renderUpInner)
{
this.vertex(mat, vertexConsumer, 0.0, levelNW, 0.0, r, g, b, u0, v0, lightmap);
this.vertex(mat, vertexConsumer, 1.0, levelNE, 0.0, r, g, b, u3, v3, lightmap);
this.vertex(mat, vertexConsumer, 1.0, levelSE, 1.0, r, g, b, u2, v2, lightmap);
this.vertex(mat, vertexConsumer, 0.0, levelSW, 1.0, r, g, b, u1, v1, lightmap);
}
}
if (renderDown)
{
var minU = sprites[0].method_4594();
var maxU = sprites[0].method_4577();
var minV = sprites[0].method_4593();
var maxV = sprites[0].method_4575();
var r = lightDown * colorR;
var g = lightDown * colorG;
var b = lightDown * colorB;
this.vertex(mat, vertexConsumer, 0, bottomY, 1.0, r, g, b, minU, maxV, lightmapDown);
this.vertex(mat, vertexConsumer, 0, bottomY, 0, r, g, b, minU, minV, lightmapDown);
this.vertex(mat, vertexConsumer, 1.0, bottomY, 0, r, g, b, maxU, minV, lightmapDown);
this.vertex(mat, vertexConsumer, 1.0, bottomY, 1.0, r, g, b, maxU, maxV, lightmapDown);
renderedAnything = true;
}
for (var direction : class_2350.class_2353.field_11062)
{
float y1;
float y2;
double x1;
double z1;
double x2;
double z2;
boolean renderThisSide;
switch (direction)
{
case field_11043 ->
{
y1 = levelNW;
y2 = levelNE;
x1 = 0;
x2 = 1.0;
z1 = 0.001f;
z2 = 0.001f;
renderThisSide = renderN;
}
case field_11035 ->
{
y1 = levelSE;
y2 = levelSW;
x1 = 1.0;
x2 = 0;
z1 = 1.0 - 0.001F;
z2 = 1.0 - 0.001F;
renderThisSide = renderS;
}
case field_11039 ->
{
y1 = levelSW;
y2 = levelNW;
x1 = 0.001F;
x2 = 0.001F;
z1 = 1.0;
z2 = 0;
renderThisSide = renderW;
}
default ->
{
y1 = levelNE;
y2 = levelSE;
x1 = 1.0 - 0.001F;
x2 = 1.0 - 0.001F;
z1 = 0;
z2 = 1.0;
renderThisSide = renderE;
}
}
if (renderThisSide)
{
renderedAnything = true;
var sprite2 = useOverlaySprite ? this.waterOverlaySprite : sprites[1];
var u1 = sprite2.method_4580(0.0F);
var u2 = sprite2.method_4580(8.0F);
var v1 = sprite2.method_4570((1.0F - y1) * 16.0F * 0.5F);
var v2 = sprite2.method_4570((1.0F - y2) * 16.0F * 0.5F);
var v3 = sprite2.method_4570(8.0F);
var dirLighting = direction.method_10166() == class_2350.class_2351.field_11051 ? lightNorth : lightWest;
var r = lightUp * dirLighting * colorR;
var g = lightUp * dirLighting * colorG;
var b = lightUp * dirLighting * colorB;
this.vertex(mat, vertexConsumer, x1, y1, z1, r, g, b, u1, v1, lightmap);
this.vertex(mat, vertexConsumer, x2, y2, z2, r, g, b, u2, v2, lightmap);
this.vertex(mat, vertexConsumer, x2, bottomY, z2, r, g, b, u2, v3, lightmap);
this.vertex(mat, vertexConsumer, x1, bottomY, z1, r, g, b, u1, v3, lightmap);
if (sprite2 != this.waterOverlaySprite)
{
this.vertex(mat, vertexConsumer, x1, bottomY, z1, r, g, b, u1, v3, lightmap);
this.vertex(mat, vertexConsumer, x2, bottomY, z2, r, g, b, u2, v3, lightmap);
this.vertex(mat, vertexConsumer, x2, y2, z2, r, g, b, u2, v2, lightmap);
this.vertex(mat, vertexConsumer, x1, y1, z1, r, g, b, u1, v1, lightmap);
}
}
}
return renderedAnything;
}
}
private void vertex(Matrix4f mat, class_4588 vertexConsumer, double x, double y, double z, float red, float green, float blue, float u, float v, int light)
{
vertexConsumer.method_22918(mat, (float)x, (float)y, (float)z).method_22915(red, green, blue, 1.0F).method_22913(u, v).method_22916(light).method_22914(0.0F, 1.0F, 0.0F).method_1344();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy