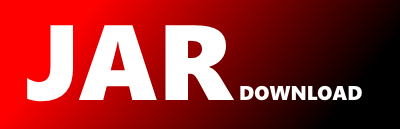
com.passkit.grpc.MessageOuterClass Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sdk Show documentation
Show all versions of sdk Show documentation
SDK for the PassKit gRPC API that can be used to create, configure and manage Membership, Loyalty, Event Ticket, Coupon, Transit and Boarding Pass content for mobile wallet applications, including Apple Pay and Google Pay.
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: io/common/message.proto
package com.passkit.grpc;
public final class MessageOuterClass {
private MessageOuterClass() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistryLite registry) {
}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
registerAllExtensions(
(com.google.protobuf.ExtensionRegistryLite) registry);
}
/**
* Protobuf enum {@code io.MessageStatus}
*/
public enum MessageStatus
implements com.google.protobuf.ProtocolMessageEnum {
/**
* MESSAGE_STATUS_DO_NOT_USE = 0;
*/
MESSAGE_STATUS_DO_NOT_USE(0),
/**
* SCHEDULED = 1;
*/
SCHEDULED(1),
/**
* ACTIVE = 2;
*/
ACTIVE(2),
/**
* EXPIRED = 3;
*/
EXPIRED(3),
/**
* CANCELLED = 4;
*/
CANCELLED(4),
UNRECOGNIZED(-1),
;
/**
* MESSAGE_STATUS_DO_NOT_USE = 0;
*/
public static final int MESSAGE_STATUS_DO_NOT_USE_VALUE = 0;
/**
* SCHEDULED = 1;
*/
public static final int SCHEDULED_VALUE = 1;
/**
* ACTIVE = 2;
*/
public static final int ACTIVE_VALUE = 2;
/**
* EXPIRED = 3;
*/
public static final int EXPIRED_VALUE = 3;
/**
* CANCELLED = 4;
*/
public static final int CANCELLED_VALUE = 4;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static MessageStatus valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static MessageStatus forNumber(int value) {
switch (value) {
case 0: return MESSAGE_STATUS_DO_NOT_USE;
case 1: return SCHEDULED;
case 2: return ACTIVE;
case 3: return EXPIRED;
case 4: return CANCELLED;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
MessageStatus> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public MessageStatus findValueByNumber(int number) {
return MessageStatus.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return com.passkit.grpc.MessageOuterClass.getDescriptor().getEnumTypes().get(0);
}
private static final MessageStatus[] VALUES = values();
public static MessageStatus valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private MessageStatus(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:io.MessageStatus)
}
public interface MessageOrBuilder extends
// @@protoc_insertion_point(interface_extends:io.Message)
com.google.protobuf.MessageOrBuilder {
/**
* string id = 1;
* @return The id.
*/
java.lang.String getId();
/**
* string id = 1;
* @return The bytes for id.
*/
com.google.protobuf.ByteString
getIdBytes();
/**
*
* The message title. This is used as a label in iOS and as the title in Google Pay.
*
*
* string title = 2;
* @return The title.
*/
java.lang.String getTitle();
/**
*
* The message title. This is used as a label in iOS and as the title in Google Pay.
*
*
* string title = 2;
* @return The bytes for title.
*/
com.google.protobuf.ByteString
getTitleBytes();
/**
*
* Localized message title.
*
*
* .io.LocalizedString localizedTitle = 3;
* @return Whether the localizedTitle field is set.
*/
boolean hasLocalizedTitle();
/**
*
* Localized message title.
*
*
* .io.LocalizedString localizedTitle = 3;
* @return The localizedTitle.
*/
com.passkit.grpc.Localization.LocalizedString getLocalizedTitle();
/**
*
* Localized message title.
*
*
* .io.LocalizedString localizedTitle = 3;
*/
com.passkit.grpc.Localization.LocalizedStringOrBuilder getLocalizedTitleOrBuilder();
/**
*
* Plain text content cannot contain hyperlinks. Google Pay messages cannot have links, use URLs instead.
*
*
* string plainTextContent = 4;
* @return The plainTextContent.
*/
java.lang.String getPlainTextContent();
/**
*
* Plain text content cannot contain hyperlinks. Google Pay messages cannot have links, use URLs instead.
*
*
* string plainTextContent = 4;
* @return The bytes for plainTextContent.
*/
com.google.protobuf.ByteString
getPlainTextContentBytes();
/**
*
* Localized plain text content.
*
*
* .io.LocalizedString localizedPlainTextContent = 5;
* @return Whether the localizedPlainTextContent field is set.
*/
boolean hasLocalizedPlainTextContent();
/**
*
* Localized plain text content.
*
*
* .io.LocalizedString localizedPlainTextContent = 5;
* @return The localizedPlainTextContent.
*/
com.passkit.grpc.Localization.LocalizedString getLocalizedPlainTextContent();
/**
*
* Localized plain text content.
*
*
* .io.LocalizedString localizedPlainTextContent = 5;
*/
com.passkit.grpc.Localization.LocalizedStringOrBuilder getLocalizedPlainTextContentOrBuilder();
/**
*
* Rich text content that can contain hyperlinks. No other markup is accepted. Links will only function on iOS devices. Ignored for Google Pay.
*
*
* string richTextContent = 6;
* @return The richTextContent.
*/
java.lang.String getRichTextContent();
/**
*
* Rich text content that can contain hyperlinks. No other markup is accepted. Links will only function on iOS devices. Ignored for Google Pay.
*
*
* string richTextContent = 6;
* @return The bytes for richTextContent.
*/
com.google.protobuf.ByteString
getRichTextContentBytes();
/**
*
* Localized rich text content.
*
*
* .io.LocalizedString localizedRichTextContent = 7;
* @return Whether the localizedRichTextContent field is set.
*/
boolean hasLocalizedRichTextContent();
/**
*
* Localized rich text content.
*
*
* .io.LocalizedString localizedRichTextContent = 7;
* @return The localizedRichTextContent.
*/
com.passkit.grpc.Localization.LocalizedString getLocalizedRichTextContent();
/**
*
* Localized rich text content.
*
*
* .io.LocalizedString localizedRichTextContent = 7;
*/
com.passkit.grpc.Localization.LocalizedStringOrBuilder getLocalizedRichTextContentOrBuilder();
/**
*
* Urls will be prioritized over existing urls on the Google Pay Pass. They are ignored for Apple Wallet.
*
*
* repeated .io.Url urls = 8;
*/
java.util.List
getUrlsList();
/**
*
* Urls will be prioritized over existing urls on the Google Pay Pass. They are ignored for Apple Wallet.
*
*
* repeated .io.Url urls = 8;
*/
com.passkit.grpc.CommonObjects.Url getUrls(int index);
/**
*
* Urls will be prioritized over existing urls on the Google Pay Pass. They are ignored for Apple Wallet.
*
*
* repeated .io.Url urls = 8;
*/
int getUrlsCount();
/**
*
* Urls will be prioritized over existing urls on the Google Pay Pass. They are ignored for Apple Wallet.
*
*
* repeated .io.Url urls = 8;
*/
java.util.List extends com.passkit.grpc.CommonObjects.UrlOrBuilder>
getUrlsOrBuilderList();
/**
*
* Urls will be prioritized over existing urls on the Google Pay Pass. They are ignored for Apple Wallet.
*
*
* repeated .io.Url urls = 8;
*/
com.passkit.grpc.CommonObjects.UrlOrBuilder getUrlsOrBuilder(
int index);
/**
*
* Images to display for the duration of the message. For Google Pay, only a 'message' type image will be used. For Apple Wallet, any of strip, eventStrip, background, logo, thumbnail and footer can be used, depending on the underlying pass type.
*
*
* .io.ImageIds images = 9;
* @return Whether the images field is set.
*/
boolean hasImages();
/**
*
* Images to display for the duration of the message. For Google Pay, only a 'message' type image will be used. For Apple Wallet, any of strip, eventStrip, background, logo, thumbnail and footer can be used, depending on the underlying pass type.
*
*
* .io.ImageIds images = 9;
* @return The images.
*/
com.passkit.grpc.Image.ImageIds getImages();
/**
*
* Images to display for the duration of the message. For Google Pay, only a 'message' type image will be used. For Apple Wallet, any of strip, eventStrip, background, logo, thumbnail and footer can be used, depending on the underlying pass type.
*
*
* .io.ImageIds images = 9;
*/
com.passkit.grpc.Image.ImageIdsOrBuilder getImagesOrBuilder();
/**
*
* Priority the lower the priority, the lower down the message will be rendered when there are multiple messages. Defaults to a value of 10.
*
*
* uint32 priority = 10;
* @return The priority.
*/
int getPriority();
/**
*
* The date and time to display the message. The value must be in RFC3339 format. If omitted, the current timestamp will be used.
*
*
* string displayFrom = 11;
* @return The displayFrom.
*/
java.lang.String getDisplayFrom();
/**
*
* The date and time to display the message. The value must be in RFC3339 format. If omitted, the current timestamp will be used.
*
*
* string displayFrom = 11;
* @return The bytes for displayFrom.
*/
com.google.protobuf.ByteString
getDisplayFromBytes();
/**
*
* The date and time to remove the message. The value must be in RFC3339 format. If omitted, the message will be displayed indefinitely until it is cancelled.
*
*
* string displayUntil = 12;
* @return The displayUntil.
*/
java.lang.String getDisplayUntil();
/**
*
* The date and time to remove the message. The value must be in RFC3339 format. If omitted, the message will be displayed indefinitely until it is cancelled.
*
*
* string displayUntil = 12;
* @return The bytes for displayUntil.
*/
com.google.protobuf.ByteString
getDisplayUntilBytes();
}
/**
* Protobuf type {@code io.Message}
*/
public static final class Message extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:io.Message)
MessageOrBuilder {
private static final long serialVersionUID = 0L;
// Use Message.newBuilder() to construct.
private Message(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Message() {
id_ = "";
title_ = "";
plainTextContent_ = "";
richTextContent_ = "";
urls_ = java.util.Collections.emptyList();
displayFrom_ = "";
displayUntil_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new Message();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Message(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
id_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
title_ = s;
break;
}
case 26: {
com.passkit.grpc.Localization.LocalizedString.Builder subBuilder = null;
if (localizedTitle_ != null) {
subBuilder = localizedTitle_.toBuilder();
}
localizedTitle_ = input.readMessage(com.passkit.grpc.Localization.LocalizedString.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(localizedTitle_);
localizedTitle_ = subBuilder.buildPartial();
}
break;
}
case 34: {
java.lang.String s = input.readStringRequireUtf8();
plainTextContent_ = s;
break;
}
case 42: {
com.passkit.grpc.Localization.LocalizedString.Builder subBuilder = null;
if (localizedPlainTextContent_ != null) {
subBuilder = localizedPlainTextContent_.toBuilder();
}
localizedPlainTextContent_ = input.readMessage(com.passkit.grpc.Localization.LocalizedString.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(localizedPlainTextContent_);
localizedPlainTextContent_ = subBuilder.buildPartial();
}
break;
}
case 50: {
java.lang.String s = input.readStringRequireUtf8();
richTextContent_ = s;
break;
}
case 58: {
com.passkit.grpc.Localization.LocalizedString.Builder subBuilder = null;
if (localizedRichTextContent_ != null) {
subBuilder = localizedRichTextContent_.toBuilder();
}
localizedRichTextContent_ = input.readMessage(com.passkit.grpc.Localization.LocalizedString.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(localizedRichTextContent_);
localizedRichTextContent_ = subBuilder.buildPartial();
}
break;
}
case 66: {
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
urls_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
urls_.add(
input.readMessage(com.passkit.grpc.CommonObjects.Url.parser(), extensionRegistry));
break;
}
case 74: {
com.passkit.grpc.Image.ImageIds.Builder subBuilder = null;
if (images_ != null) {
subBuilder = images_.toBuilder();
}
images_ = input.readMessage(com.passkit.grpc.Image.ImageIds.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(images_);
images_ = subBuilder.buildPartial();
}
break;
}
case 80: {
priority_ = input.readUInt32();
break;
}
case 90: {
java.lang.String s = input.readStringRequireUtf8();
displayFrom_ = s;
break;
}
case 98: {
java.lang.String s = input.readStringRequireUtf8();
displayUntil_ = s;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
urls_ = java.util.Collections.unmodifiableList(urls_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.passkit.grpc.MessageOuterClass.internal_static_io_Message_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.passkit.grpc.MessageOuterClass.internal_static_io_Message_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.passkit.grpc.MessageOuterClass.Message.class, com.passkit.grpc.MessageOuterClass.Message.Builder.class);
}
public static final int ID_FIELD_NUMBER = 1;
private volatile java.lang.Object id_;
/**
* string id = 1;
* @return The id.
*/
@java.lang.Override
public java.lang.String getId() {
java.lang.Object ref = id_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
id_ = s;
return s;
}
}
/**
* string id = 1;
* @return The bytes for id.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getIdBytes() {
java.lang.Object ref = id_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
id_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int TITLE_FIELD_NUMBER = 2;
private volatile java.lang.Object title_;
/**
*
* The message title. This is used as a label in iOS and as the title in Google Pay.
*
*
* string title = 2;
* @return The title.
*/
@java.lang.Override
public java.lang.String getTitle() {
java.lang.Object ref = title_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
title_ = s;
return s;
}
}
/**
*
* The message title. This is used as a label in iOS and as the title in Google Pay.
*
*
* string title = 2;
* @return The bytes for title.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getTitleBytes() {
java.lang.Object ref = title_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
title_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int LOCALIZEDTITLE_FIELD_NUMBER = 3;
private com.passkit.grpc.Localization.LocalizedString localizedTitle_;
/**
*
* Localized message title.
*
*
* .io.LocalizedString localizedTitle = 3;
* @return Whether the localizedTitle field is set.
*/
@java.lang.Override
public boolean hasLocalizedTitle() {
return localizedTitle_ != null;
}
/**
*
* Localized message title.
*
*
* .io.LocalizedString localizedTitle = 3;
* @return The localizedTitle.
*/
@java.lang.Override
public com.passkit.grpc.Localization.LocalizedString getLocalizedTitle() {
return localizedTitle_ == null ? com.passkit.grpc.Localization.LocalizedString.getDefaultInstance() : localizedTitle_;
}
/**
*
* Localized message title.
*
*
* .io.LocalizedString localizedTitle = 3;
*/
@java.lang.Override
public com.passkit.grpc.Localization.LocalizedStringOrBuilder getLocalizedTitleOrBuilder() {
return getLocalizedTitle();
}
public static final int PLAINTEXTCONTENT_FIELD_NUMBER = 4;
private volatile java.lang.Object plainTextContent_;
/**
*
* Plain text content cannot contain hyperlinks. Google Pay messages cannot have links, use URLs instead.
*
*
* string plainTextContent = 4;
* @return The plainTextContent.
*/
@java.lang.Override
public java.lang.String getPlainTextContent() {
java.lang.Object ref = plainTextContent_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
plainTextContent_ = s;
return s;
}
}
/**
*
* Plain text content cannot contain hyperlinks. Google Pay messages cannot have links, use URLs instead.
*
*
* string plainTextContent = 4;
* @return The bytes for plainTextContent.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getPlainTextContentBytes() {
java.lang.Object ref = plainTextContent_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
plainTextContent_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int LOCALIZEDPLAINTEXTCONTENT_FIELD_NUMBER = 5;
private com.passkit.grpc.Localization.LocalizedString localizedPlainTextContent_;
/**
*
* Localized plain text content.
*
*
* .io.LocalizedString localizedPlainTextContent = 5;
* @return Whether the localizedPlainTextContent field is set.
*/
@java.lang.Override
public boolean hasLocalizedPlainTextContent() {
return localizedPlainTextContent_ != null;
}
/**
*
* Localized plain text content.
*
*
* .io.LocalizedString localizedPlainTextContent = 5;
* @return The localizedPlainTextContent.
*/
@java.lang.Override
public com.passkit.grpc.Localization.LocalizedString getLocalizedPlainTextContent() {
return localizedPlainTextContent_ == null ? com.passkit.grpc.Localization.LocalizedString.getDefaultInstance() : localizedPlainTextContent_;
}
/**
*
* Localized plain text content.
*
*
* .io.LocalizedString localizedPlainTextContent = 5;
*/
@java.lang.Override
public com.passkit.grpc.Localization.LocalizedStringOrBuilder getLocalizedPlainTextContentOrBuilder() {
return getLocalizedPlainTextContent();
}
public static final int RICHTEXTCONTENT_FIELD_NUMBER = 6;
private volatile java.lang.Object richTextContent_;
/**
*
* Rich text content that can contain hyperlinks. No other markup is accepted. Links will only function on iOS devices. Ignored for Google Pay.
*
*
* string richTextContent = 6;
* @return The richTextContent.
*/
@java.lang.Override
public java.lang.String getRichTextContent() {
java.lang.Object ref = richTextContent_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
richTextContent_ = s;
return s;
}
}
/**
*
* Rich text content that can contain hyperlinks. No other markup is accepted. Links will only function on iOS devices. Ignored for Google Pay.
*
*
* string richTextContent = 6;
* @return The bytes for richTextContent.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getRichTextContentBytes() {
java.lang.Object ref = richTextContent_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
richTextContent_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int LOCALIZEDRICHTEXTCONTENT_FIELD_NUMBER = 7;
private com.passkit.grpc.Localization.LocalizedString localizedRichTextContent_;
/**
*
* Localized rich text content.
*
*
* .io.LocalizedString localizedRichTextContent = 7;
* @return Whether the localizedRichTextContent field is set.
*/
@java.lang.Override
public boolean hasLocalizedRichTextContent() {
return localizedRichTextContent_ != null;
}
/**
*
* Localized rich text content.
*
*
* .io.LocalizedString localizedRichTextContent = 7;
* @return The localizedRichTextContent.
*/
@java.lang.Override
public com.passkit.grpc.Localization.LocalizedString getLocalizedRichTextContent() {
return localizedRichTextContent_ == null ? com.passkit.grpc.Localization.LocalizedString.getDefaultInstance() : localizedRichTextContent_;
}
/**
*
* Localized rich text content.
*
*
* .io.LocalizedString localizedRichTextContent = 7;
*/
@java.lang.Override
public com.passkit.grpc.Localization.LocalizedStringOrBuilder getLocalizedRichTextContentOrBuilder() {
return getLocalizedRichTextContent();
}
public static final int URLS_FIELD_NUMBER = 8;
private java.util.List urls_;
/**
*
* Urls will be prioritized over existing urls on the Google Pay Pass. They are ignored for Apple Wallet.
*
*
* repeated .io.Url urls = 8;
*/
@java.lang.Override
public java.util.List getUrlsList() {
return urls_;
}
/**
*
* Urls will be prioritized over existing urls on the Google Pay Pass. They are ignored for Apple Wallet.
*
*
* repeated .io.Url urls = 8;
*/
@java.lang.Override
public java.util.List extends com.passkit.grpc.CommonObjects.UrlOrBuilder>
getUrlsOrBuilderList() {
return urls_;
}
/**
*
* Urls will be prioritized over existing urls on the Google Pay Pass. They are ignored for Apple Wallet.
*
*
* repeated .io.Url urls = 8;
*/
@java.lang.Override
public int getUrlsCount() {
return urls_.size();
}
/**
*
* Urls will be prioritized over existing urls on the Google Pay Pass. They are ignored for Apple Wallet.
*
*
* repeated .io.Url urls = 8;
*/
@java.lang.Override
public com.passkit.grpc.CommonObjects.Url getUrls(int index) {
return urls_.get(index);
}
/**
*
* Urls will be prioritized over existing urls on the Google Pay Pass. They are ignored for Apple Wallet.
*
*
* repeated .io.Url urls = 8;
*/
@java.lang.Override
public com.passkit.grpc.CommonObjects.UrlOrBuilder getUrlsOrBuilder(
int index) {
return urls_.get(index);
}
public static final int IMAGES_FIELD_NUMBER = 9;
private com.passkit.grpc.Image.ImageIds images_;
/**
*
* Images to display for the duration of the message. For Google Pay, only a 'message' type image will be used. For Apple Wallet, any of strip, eventStrip, background, logo, thumbnail and footer can be used, depending on the underlying pass type.
*
*
* .io.ImageIds images = 9;
* @return Whether the images field is set.
*/
@java.lang.Override
public boolean hasImages() {
return images_ != null;
}
/**
*
* Images to display for the duration of the message. For Google Pay, only a 'message' type image will be used. For Apple Wallet, any of strip, eventStrip, background, logo, thumbnail and footer can be used, depending on the underlying pass type.
*
*
* .io.ImageIds images = 9;
* @return The images.
*/
@java.lang.Override
public com.passkit.grpc.Image.ImageIds getImages() {
return images_ == null ? com.passkit.grpc.Image.ImageIds.getDefaultInstance() : images_;
}
/**
*
* Images to display for the duration of the message. For Google Pay, only a 'message' type image will be used. For Apple Wallet, any of strip, eventStrip, background, logo, thumbnail and footer can be used, depending on the underlying pass type.
*
*
* .io.ImageIds images = 9;
*/
@java.lang.Override
public com.passkit.grpc.Image.ImageIdsOrBuilder getImagesOrBuilder() {
return getImages();
}
public static final int PRIORITY_FIELD_NUMBER = 10;
private int priority_;
/**
*
* Priority the lower the priority, the lower down the message will be rendered when there are multiple messages. Defaults to a value of 10.
*
*
* uint32 priority = 10;
* @return The priority.
*/
@java.lang.Override
public int getPriority() {
return priority_;
}
public static final int DISPLAYFROM_FIELD_NUMBER = 11;
private volatile java.lang.Object displayFrom_;
/**
*
* The date and time to display the message. The value must be in RFC3339 format. If omitted, the current timestamp will be used.
*
*
* string displayFrom = 11;
* @return The displayFrom.
*/
@java.lang.Override
public java.lang.String getDisplayFrom() {
java.lang.Object ref = displayFrom_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
displayFrom_ = s;
return s;
}
}
/**
*
* The date and time to display the message. The value must be in RFC3339 format. If omitted, the current timestamp will be used.
*
*
* string displayFrom = 11;
* @return The bytes for displayFrom.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getDisplayFromBytes() {
java.lang.Object ref = displayFrom_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
displayFrom_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int DISPLAYUNTIL_FIELD_NUMBER = 12;
private volatile java.lang.Object displayUntil_;
/**
*
* The date and time to remove the message. The value must be in RFC3339 format. If omitted, the message will be displayed indefinitely until it is cancelled.
*
*
* string displayUntil = 12;
* @return The displayUntil.
*/
@java.lang.Override
public java.lang.String getDisplayUntil() {
java.lang.Object ref = displayUntil_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
displayUntil_ = s;
return s;
}
}
/**
*
* The date and time to remove the message. The value must be in RFC3339 format. If omitted, the message will be displayed indefinitely until it is cancelled.
*
*
* string displayUntil = 12;
* @return The bytes for displayUntil.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getDisplayUntilBytes() {
java.lang.Object ref = displayUntil_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
displayUntil_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(id_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, id_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(title_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, title_);
}
if (localizedTitle_ != null) {
output.writeMessage(3, getLocalizedTitle());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(plainTextContent_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, plainTextContent_);
}
if (localizedPlainTextContent_ != null) {
output.writeMessage(5, getLocalizedPlainTextContent());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(richTextContent_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 6, richTextContent_);
}
if (localizedRichTextContent_ != null) {
output.writeMessage(7, getLocalizedRichTextContent());
}
for (int i = 0; i < urls_.size(); i++) {
output.writeMessage(8, urls_.get(i));
}
if (images_ != null) {
output.writeMessage(9, getImages());
}
if (priority_ != 0) {
output.writeUInt32(10, priority_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(displayFrom_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 11, displayFrom_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(displayUntil_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 12, displayUntil_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(id_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, id_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(title_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, title_);
}
if (localizedTitle_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getLocalizedTitle());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(plainTextContent_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(4, plainTextContent_);
}
if (localizedPlainTextContent_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, getLocalizedPlainTextContent());
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(richTextContent_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(6, richTextContent_);
}
if (localizedRichTextContent_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(7, getLocalizedRichTextContent());
}
for (int i = 0; i < urls_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(8, urls_.get(i));
}
if (images_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(9, getImages());
}
if (priority_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(10, priority_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(displayFrom_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(11, displayFrom_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(displayUntil_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(12, displayUntil_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.passkit.grpc.MessageOuterClass.Message)) {
return super.equals(obj);
}
com.passkit.grpc.MessageOuterClass.Message other = (com.passkit.grpc.MessageOuterClass.Message) obj;
if (!getId()
.equals(other.getId())) return false;
if (!getTitle()
.equals(other.getTitle())) return false;
if (hasLocalizedTitle() != other.hasLocalizedTitle()) return false;
if (hasLocalizedTitle()) {
if (!getLocalizedTitle()
.equals(other.getLocalizedTitle())) return false;
}
if (!getPlainTextContent()
.equals(other.getPlainTextContent())) return false;
if (hasLocalizedPlainTextContent() != other.hasLocalizedPlainTextContent()) return false;
if (hasLocalizedPlainTextContent()) {
if (!getLocalizedPlainTextContent()
.equals(other.getLocalizedPlainTextContent())) return false;
}
if (!getRichTextContent()
.equals(other.getRichTextContent())) return false;
if (hasLocalizedRichTextContent() != other.hasLocalizedRichTextContent()) return false;
if (hasLocalizedRichTextContent()) {
if (!getLocalizedRichTextContent()
.equals(other.getLocalizedRichTextContent())) return false;
}
if (!getUrlsList()
.equals(other.getUrlsList())) return false;
if (hasImages() != other.hasImages()) return false;
if (hasImages()) {
if (!getImages()
.equals(other.getImages())) return false;
}
if (getPriority()
!= other.getPriority()) return false;
if (!getDisplayFrom()
.equals(other.getDisplayFrom())) return false;
if (!getDisplayUntil()
.equals(other.getDisplayUntil())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + ID_FIELD_NUMBER;
hash = (53 * hash) + getId().hashCode();
hash = (37 * hash) + TITLE_FIELD_NUMBER;
hash = (53 * hash) + getTitle().hashCode();
if (hasLocalizedTitle()) {
hash = (37 * hash) + LOCALIZEDTITLE_FIELD_NUMBER;
hash = (53 * hash) + getLocalizedTitle().hashCode();
}
hash = (37 * hash) + PLAINTEXTCONTENT_FIELD_NUMBER;
hash = (53 * hash) + getPlainTextContent().hashCode();
if (hasLocalizedPlainTextContent()) {
hash = (37 * hash) + LOCALIZEDPLAINTEXTCONTENT_FIELD_NUMBER;
hash = (53 * hash) + getLocalizedPlainTextContent().hashCode();
}
hash = (37 * hash) + RICHTEXTCONTENT_FIELD_NUMBER;
hash = (53 * hash) + getRichTextContent().hashCode();
if (hasLocalizedRichTextContent()) {
hash = (37 * hash) + LOCALIZEDRICHTEXTCONTENT_FIELD_NUMBER;
hash = (53 * hash) + getLocalizedRichTextContent().hashCode();
}
if (getUrlsCount() > 0) {
hash = (37 * hash) + URLS_FIELD_NUMBER;
hash = (53 * hash) + getUrlsList().hashCode();
}
if (hasImages()) {
hash = (37 * hash) + IMAGES_FIELD_NUMBER;
hash = (53 * hash) + getImages().hashCode();
}
hash = (37 * hash) + PRIORITY_FIELD_NUMBER;
hash = (53 * hash) + getPriority();
hash = (37 * hash) + DISPLAYFROM_FIELD_NUMBER;
hash = (53 * hash) + getDisplayFrom().hashCode();
hash = (37 * hash) + DISPLAYUNTIL_FIELD_NUMBER;
hash = (53 * hash) + getDisplayUntil().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.passkit.grpc.MessageOuterClass.Message parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.passkit.grpc.MessageOuterClass.Message parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.passkit.grpc.MessageOuterClass.Message parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.passkit.grpc.MessageOuterClass.Message parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.passkit.grpc.MessageOuterClass.Message parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.passkit.grpc.MessageOuterClass.Message parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.passkit.grpc.MessageOuterClass.Message parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.passkit.grpc.MessageOuterClass.Message parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.passkit.grpc.MessageOuterClass.Message parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.passkit.grpc.MessageOuterClass.Message parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.passkit.grpc.MessageOuterClass.Message parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.passkit.grpc.MessageOuterClass.Message parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.passkit.grpc.MessageOuterClass.Message prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code io.Message}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:io.Message)
com.passkit.grpc.MessageOuterClass.MessageOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.passkit.grpc.MessageOuterClass.internal_static_io_Message_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.passkit.grpc.MessageOuterClass.internal_static_io_Message_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.passkit.grpc.MessageOuterClass.Message.class, com.passkit.grpc.MessageOuterClass.Message.Builder.class);
}
// Construct using com.passkit.grpc.MessageOuterClass.Message.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getUrlsFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
id_ = "";
title_ = "";
if (localizedTitleBuilder_ == null) {
localizedTitle_ = null;
} else {
localizedTitle_ = null;
localizedTitleBuilder_ = null;
}
plainTextContent_ = "";
if (localizedPlainTextContentBuilder_ == null) {
localizedPlainTextContent_ = null;
} else {
localizedPlainTextContent_ = null;
localizedPlainTextContentBuilder_ = null;
}
richTextContent_ = "";
if (localizedRichTextContentBuilder_ == null) {
localizedRichTextContent_ = null;
} else {
localizedRichTextContent_ = null;
localizedRichTextContentBuilder_ = null;
}
if (urlsBuilder_ == null) {
urls_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
urlsBuilder_.clear();
}
if (imagesBuilder_ == null) {
images_ = null;
} else {
images_ = null;
imagesBuilder_ = null;
}
priority_ = 0;
displayFrom_ = "";
displayUntil_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.passkit.grpc.MessageOuterClass.internal_static_io_Message_descriptor;
}
@java.lang.Override
public com.passkit.grpc.MessageOuterClass.Message getDefaultInstanceForType() {
return com.passkit.grpc.MessageOuterClass.Message.getDefaultInstance();
}
@java.lang.Override
public com.passkit.grpc.MessageOuterClass.Message build() {
com.passkit.grpc.MessageOuterClass.Message result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.passkit.grpc.MessageOuterClass.Message buildPartial() {
com.passkit.grpc.MessageOuterClass.Message result = new com.passkit.grpc.MessageOuterClass.Message(this);
int from_bitField0_ = bitField0_;
result.id_ = id_;
result.title_ = title_;
if (localizedTitleBuilder_ == null) {
result.localizedTitle_ = localizedTitle_;
} else {
result.localizedTitle_ = localizedTitleBuilder_.build();
}
result.plainTextContent_ = plainTextContent_;
if (localizedPlainTextContentBuilder_ == null) {
result.localizedPlainTextContent_ = localizedPlainTextContent_;
} else {
result.localizedPlainTextContent_ = localizedPlainTextContentBuilder_.build();
}
result.richTextContent_ = richTextContent_;
if (localizedRichTextContentBuilder_ == null) {
result.localizedRichTextContent_ = localizedRichTextContent_;
} else {
result.localizedRichTextContent_ = localizedRichTextContentBuilder_.build();
}
if (urlsBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0)) {
urls_ = java.util.Collections.unmodifiableList(urls_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.urls_ = urls_;
} else {
result.urls_ = urlsBuilder_.build();
}
if (imagesBuilder_ == null) {
result.images_ = images_;
} else {
result.images_ = imagesBuilder_.build();
}
result.priority_ = priority_;
result.displayFrom_ = displayFrom_;
result.displayUntil_ = displayUntil_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.passkit.grpc.MessageOuterClass.Message) {
return mergeFrom((com.passkit.grpc.MessageOuterClass.Message)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.passkit.grpc.MessageOuterClass.Message other) {
if (other == com.passkit.grpc.MessageOuterClass.Message.getDefaultInstance()) return this;
if (!other.getId().isEmpty()) {
id_ = other.id_;
onChanged();
}
if (!other.getTitle().isEmpty()) {
title_ = other.title_;
onChanged();
}
if (other.hasLocalizedTitle()) {
mergeLocalizedTitle(other.getLocalizedTitle());
}
if (!other.getPlainTextContent().isEmpty()) {
plainTextContent_ = other.plainTextContent_;
onChanged();
}
if (other.hasLocalizedPlainTextContent()) {
mergeLocalizedPlainTextContent(other.getLocalizedPlainTextContent());
}
if (!other.getRichTextContent().isEmpty()) {
richTextContent_ = other.richTextContent_;
onChanged();
}
if (other.hasLocalizedRichTextContent()) {
mergeLocalizedRichTextContent(other.getLocalizedRichTextContent());
}
if (urlsBuilder_ == null) {
if (!other.urls_.isEmpty()) {
if (urls_.isEmpty()) {
urls_ = other.urls_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureUrlsIsMutable();
urls_.addAll(other.urls_);
}
onChanged();
}
} else {
if (!other.urls_.isEmpty()) {
if (urlsBuilder_.isEmpty()) {
urlsBuilder_.dispose();
urlsBuilder_ = null;
urls_ = other.urls_;
bitField0_ = (bitField0_ & ~0x00000001);
urlsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getUrlsFieldBuilder() : null;
} else {
urlsBuilder_.addAllMessages(other.urls_);
}
}
}
if (other.hasImages()) {
mergeImages(other.getImages());
}
if (other.getPriority() != 0) {
setPriority(other.getPriority());
}
if (!other.getDisplayFrom().isEmpty()) {
displayFrom_ = other.displayFrom_;
onChanged();
}
if (!other.getDisplayUntil().isEmpty()) {
displayUntil_ = other.displayUntil_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.passkit.grpc.MessageOuterClass.Message parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.passkit.grpc.MessageOuterClass.Message) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object id_ = "";
/**
* string id = 1;
* @return The id.
*/
public java.lang.String getId() {
java.lang.Object ref = id_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
id_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string id = 1;
* @return The bytes for id.
*/
public com.google.protobuf.ByteString
getIdBytes() {
java.lang.Object ref = id_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
id_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string id = 1;
* @param value The id to set.
* @return This builder for chaining.
*/
public Builder setId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
id_ = value;
onChanged();
return this;
}
/**
* string id = 1;
* @return This builder for chaining.
*/
public Builder clearId() {
id_ = getDefaultInstance().getId();
onChanged();
return this;
}
/**
* string id = 1;
* @param value The bytes for id to set.
* @return This builder for chaining.
*/
public Builder setIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
id_ = value;
onChanged();
return this;
}
private java.lang.Object title_ = "";
/**
*
* The message title. This is used as a label in iOS and as the title in Google Pay.
*
*
* string title = 2;
* @return The title.
*/
public java.lang.String getTitle() {
java.lang.Object ref = title_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
title_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* The message title. This is used as a label in iOS and as the title in Google Pay.
*
*
* string title = 2;
* @return The bytes for title.
*/
public com.google.protobuf.ByteString
getTitleBytes() {
java.lang.Object ref = title_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
title_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* The message title. This is used as a label in iOS and as the title in Google Pay.
*
*
* string title = 2;
* @param value The title to set.
* @return This builder for chaining.
*/
public Builder setTitle(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
title_ = value;
onChanged();
return this;
}
/**
*
* The message title. This is used as a label in iOS and as the title in Google Pay.
*
*
* string title = 2;
* @return This builder for chaining.
*/
public Builder clearTitle() {
title_ = getDefaultInstance().getTitle();
onChanged();
return this;
}
/**
*
* The message title. This is used as a label in iOS and as the title in Google Pay.
*
*
* string title = 2;
* @param value The bytes for title to set.
* @return This builder for chaining.
*/
public Builder setTitleBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
title_ = value;
onChanged();
return this;
}
private com.passkit.grpc.Localization.LocalizedString localizedTitle_;
private com.google.protobuf.SingleFieldBuilderV3<
com.passkit.grpc.Localization.LocalizedString, com.passkit.grpc.Localization.LocalizedString.Builder, com.passkit.grpc.Localization.LocalizedStringOrBuilder> localizedTitleBuilder_;
/**
*
* Localized message title.
*
*
* .io.LocalizedString localizedTitle = 3;
* @return Whether the localizedTitle field is set.
*/
public boolean hasLocalizedTitle() {
return localizedTitleBuilder_ != null || localizedTitle_ != null;
}
/**
*
* Localized message title.
*
*
* .io.LocalizedString localizedTitle = 3;
* @return The localizedTitle.
*/
public com.passkit.grpc.Localization.LocalizedString getLocalizedTitle() {
if (localizedTitleBuilder_ == null) {
return localizedTitle_ == null ? com.passkit.grpc.Localization.LocalizedString.getDefaultInstance() : localizedTitle_;
} else {
return localizedTitleBuilder_.getMessage();
}
}
/**
*
* Localized message title.
*
*
* .io.LocalizedString localizedTitle = 3;
*/
public Builder setLocalizedTitle(com.passkit.grpc.Localization.LocalizedString value) {
if (localizedTitleBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
localizedTitle_ = value;
onChanged();
} else {
localizedTitleBuilder_.setMessage(value);
}
return this;
}
/**
*
* Localized message title.
*
*
* .io.LocalizedString localizedTitle = 3;
*/
public Builder setLocalizedTitle(
com.passkit.grpc.Localization.LocalizedString.Builder builderForValue) {
if (localizedTitleBuilder_ == null) {
localizedTitle_ = builderForValue.build();
onChanged();
} else {
localizedTitleBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Localized message title.
*
*
* .io.LocalizedString localizedTitle = 3;
*/
public Builder mergeLocalizedTitle(com.passkit.grpc.Localization.LocalizedString value) {
if (localizedTitleBuilder_ == null) {
if (localizedTitle_ != null) {
localizedTitle_ =
com.passkit.grpc.Localization.LocalizedString.newBuilder(localizedTitle_).mergeFrom(value).buildPartial();
} else {
localizedTitle_ = value;
}
onChanged();
} else {
localizedTitleBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Localized message title.
*
*
* .io.LocalizedString localizedTitle = 3;
*/
public Builder clearLocalizedTitle() {
if (localizedTitleBuilder_ == null) {
localizedTitle_ = null;
onChanged();
} else {
localizedTitle_ = null;
localizedTitleBuilder_ = null;
}
return this;
}
/**
*
* Localized message title.
*
*
* .io.LocalizedString localizedTitle = 3;
*/
public com.passkit.grpc.Localization.LocalizedString.Builder getLocalizedTitleBuilder() {
onChanged();
return getLocalizedTitleFieldBuilder().getBuilder();
}
/**
*
* Localized message title.
*
*
* .io.LocalizedString localizedTitle = 3;
*/
public com.passkit.grpc.Localization.LocalizedStringOrBuilder getLocalizedTitleOrBuilder() {
if (localizedTitleBuilder_ != null) {
return localizedTitleBuilder_.getMessageOrBuilder();
} else {
return localizedTitle_ == null ?
com.passkit.grpc.Localization.LocalizedString.getDefaultInstance() : localizedTitle_;
}
}
/**
*
* Localized message title.
*
*
* .io.LocalizedString localizedTitle = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.passkit.grpc.Localization.LocalizedString, com.passkit.grpc.Localization.LocalizedString.Builder, com.passkit.grpc.Localization.LocalizedStringOrBuilder>
getLocalizedTitleFieldBuilder() {
if (localizedTitleBuilder_ == null) {
localizedTitleBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.passkit.grpc.Localization.LocalizedString, com.passkit.grpc.Localization.LocalizedString.Builder, com.passkit.grpc.Localization.LocalizedStringOrBuilder>(
getLocalizedTitle(),
getParentForChildren(),
isClean());
localizedTitle_ = null;
}
return localizedTitleBuilder_;
}
private java.lang.Object plainTextContent_ = "";
/**
*
* Plain text content cannot contain hyperlinks. Google Pay messages cannot have links, use URLs instead.
*
*
* string plainTextContent = 4;
* @return The plainTextContent.
*/
public java.lang.String getPlainTextContent() {
java.lang.Object ref = plainTextContent_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
plainTextContent_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Plain text content cannot contain hyperlinks. Google Pay messages cannot have links, use URLs instead.
*
*
* string plainTextContent = 4;
* @return The bytes for plainTextContent.
*/
public com.google.protobuf.ByteString
getPlainTextContentBytes() {
java.lang.Object ref = plainTextContent_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
plainTextContent_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Plain text content cannot contain hyperlinks. Google Pay messages cannot have links, use URLs instead.
*
*
* string plainTextContent = 4;
* @param value The plainTextContent to set.
* @return This builder for chaining.
*/
public Builder setPlainTextContent(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
plainTextContent_ = value;
onChanged();
return this;
}
/**
*
* Plain text content cannot contain hyperlinks. Google Pay messages cannot have links, use URLs instead.
*
*
* string plainTextContent = 4;
* @return This builder for chaining.
*/
public Builder clearPlainTextContent() {
plainTextContent_ = getDefaultInstance().getPlainTextContent();
onChanged();
return this;
}
/**
*
* Plain text content cannot contain hyperlinks. Google Pay messages cannot have links, use URLs instead.
*
*
* string plainTextContent = 4;
* @param value The bytes for plainTextContent to set.
* @return This builder for chaining.
*/
public Builder setPlainTextContentBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
plainTextContent_ = value;
onChanged();
return this;
}
private com.passkit.grpc.Localization.LocalizedString localizedPlainTextContent_;
private com.google.protobuf.SingleFieldBuilderV3<
com.passkit.grpc.Localization.LocalizedString, com.passkit.grpc.Localization.LocalizedString.Builder, com.passkit.grpc.Localization.LocalizedStringOrBuilder> localizedPlainTextContentBuilder_;
/**
*
* Localized plain text content.
*
*
* .io.LocalizedString localizedPlainTextContent = 5;
* @return Whether the localizedPlainTextContent field is set.
*/
public boolean hasLocalizedPlainTextContent() {
return localizedPlainTextContentBuilder_ != null || localizedPlainTextContent_ != null;
}
/**
*
* Localized plain text content.
*
*
* .io.LocalizedString localizedPlainTextContent = 5;
* @return The localizedPlainTextContent.
*/
public com.passkit.grpc.Localization.LocalizedString getLocalizedPlainTextContent() {
if (localizedPlainTextContentBuilder_ == null) {
return localizedPlainTextContent_ == null ? com.passkit.grpc.Localization.LocalizedString.getDefaultInstance() : localizedPlainTextContent_;
} else {
return localizedPlainTextContentBuilder_.getMessage();
}
}
/**
*
* Localized plain text content.
*
*
* .io.LocalizedString localizedPlainTextContent = 5;
*/
public Builder setLocalizedPlainTextContent(com.passkit.grpc.Localization.LocalizedString value) {
if (localizedPlainTextContentBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
localizedPlainTextContent_ = value;
onChanged();
} else {
localizedPlainTextContentBuilder_.setMessage(value);
}
return this;
}
/**
*
* Localized plain text content.
*
*
* .io.LocalizedString localizedPlainTextContent = 5;
*/
public Builder setLocalizedPlainTextContent(
com.passkit.grpc.Localization.LocalizedString.Builder builderForValue) {
if (localizedPlainTextContentBuilder_ == null) {
localizedPlainTextContent_ = builderForValue.build();
onChanged();
} else {
localizedPlainTextContentBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Localized plain text content.
*
*
* .io.LocalizedString localizedPlainTextContent = 5;
*/
public Builder mergeLocalizedPlainTextContent(com.passkit.grpc.Localization.LocalizedString value) {
if (localizedPlainTextContentBuilder_ == null) {
if (localizedPlainTextContent_ != null) {
localizedPlainTextContent_ =
com.passkit.grpc.Localization.LocalizedString.newBuilder(localizedPlainTextContent_).mergeFrom(value).buildPartial();
} else {
localizedPlainTextContent_ = value;
}
onChanged();
} else {
localizedPlainTextContentBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Localized plain text content.
*
*
* .io.LocalizedString localizedPlainTextContent = 5;
*/
public Builder clearLocalizedPlainTextContent() {
if (localizedPlainTextContentBuilder_ == null) {
localizedPlainTextContent_ = null;
onChanged();
} else {
localizedPlainTextContent_ = null;
localizedPlainTextContentBuilder_ = null;
}
return this;
}
/**
*
* Localized plain text content.
*
*
* .io.LocalizedString localizedPlainTextContent = 5;
*/
public com.passkit.grpc.Localization.LocalizedString.Builder getLocalizedPlainTextContentBuilder() {
onChanged();
return getLocalizedPlainTextContentFieldBuilder().getBuilder();
}
/**
*
* Localized plain text content.
*
*
* .io.LocalizedString localizedPlainTextContent = 5;
*/
public com.passkit.grpc.Localization.LocalizedStringOrBuilder getLocalizedPlainTextContentOrBuilder() {
if (localizedPlainTextContentBuilder_ != null) {
return localizedPlainTextContentBuilder_.getMessageOrBuilder();
} else {
return localizedPlainTextContent_ == null ?
com.passkit.grpc.Localization.LocalizedString.getDefaultInstance() : localizedPlainTextContent_;
}
}
/**
*
* Localized plain text content.
*
*
* .io.LocalizedString localizedPlainTextContent = 5;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.passkit.grpc.Localization.LocalizedString, com.passkit.grpc.Localization.LocalizedString.Builder, com.passkit.grpc.Localization.LocalizedStringOrBuilder>
getLocalizedPlainTextContentFieldBuilder() {
if (localizedPlainTextContentBuilder_ == null) {
localizedPlainTextContentBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.passkit.grpc.Localization.LocalizedString, com.passkit.grpc.Localization.LocalizedString.Builder, com.passkit.grpc.Localization.LocalizedStringOrBuilder>(
getLocalizedPlainTextContent(),
getParentForChildren(),
isClean());
localizedPlainTextContent_ = null;
}
return localizedPlainTextContentBuilder_;
}
private java.lang.Object richTextContent_ = "";
/**
*
* Rich text content that can contain hyperlinks. No other markup is accepted. Links will only function on iOS devices. Ignored for Google Pay.
*
*
* string richTextContent = 6;
* @return The richTextContent.
*/
public java.lang.String getRichTextContent() {
java.lang.Object ref = richTextContent_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
richTextContent_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Rich text content that can contain hyperlinks. No other markup is accepted. Links will only function on iOS devices. Ignored for Google Pay.
*
*
* string richTextContent = 6;
* @return The bytes for richTextContent.
*/
public com.google.protobuf.ByteString
getRichTextContentBytes() {
java.lang.Object ref = richTextContent_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
richTextContent_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Rich text content that can contain hyperlinks. No other markup is accepted. Links will only function on iOS devices. Ignored for Google Pay.
*
*
* string richTextContent = 6;
* @param value The richTextContent to set.
* @return This builder for chaining.
*/
public Builder setRichTextContent(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
richTextContent_ = value;
onChanged();
return this;
}
/**
*
* Rich text content that can contain hyperlinks. No other markup is accepted. Links will only function on iOS devices. Ignored for Google Pay.
*
*
* string richTextContent = 6;
* @return This builder for chaining.
*/
public Builder clearRichTextContent() {
richTextContent_ = getDefaultInstance().getRichTextContent();
onChanged();
return this;
}
/**
*
* Rich text content that can contain hyperlinks. No other markup is accepted. Links will only function on iOS devices. Ignored for Google Pay.
*
*
* string richTextContent = 6;
* @param value The bytes for richTextContent to set.
* @return This builder for chaining.
*/
public Builder setRichTextContentBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
richTextContent_ = value;
onChanged();
return this;
}
private com.passkit.grpc.Localization.LocalizedString localizedRichTextContent_;
private com.google.protobuf.SingleFieldBuilderV3<
com.passkit.grpc.Localization.LocalizedString, com.passkit.grpc.Localization.LocalizedString.Builder, com.passkit.grpc.Localization.LocalizedStringOrBuilder> localizedRichTextContentBuilder_;
/**
*
* Localized rich text content.
*
*
* .io.LocalizedString localizedRichTextContent = 7;
* @return Whether the localizedRichTextContent field is set.
*/
public boolean hasLocalizedRichTextContent() {
return localizedRichTextContentBuilder_ != null || localizedRichTextContent_ != null;
}
/**
*
* Localized rich text content.
*
*
* .io.LocalizedString localizedRichTextContent = 7;
* @return The localizedRichTextContent.
*/
public com.passkit.grpc.Localization.LocalizedString getLocalizedRichTextContent() {
if (localizedRichTextContentBuilder_ == null) {
return localizedRichTextContent_ == null ? com.passkit.grpc.Localization.LocalizedString.getDefaultInstance() : localizedRichTextContent_;
} else {
return localizedRichTextContentBuilder_.getMessage();
}
}
/**
*
* Localized rich text content.
*
*
* .io.LocalizedString localizedRichTextContent = 7;
*/
public Builder setLocalizedRichTextContent(com.passkit.grpc.Localization.LocalizedString value) {
if (localizedRichTextContentBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
localizedRichTextContent_ = value;
onChanged();
} else {
localizedRichTextContentBuilder_.setMessage(value);
}
return this;
}
/**
*
* Localized rich text content.
*
*
* .io.LocalizedString localizedRichTextContent = 7;
*/
public Builder setLocalizedRichTextContent(
com.passkit.grpc.Localization.LocalizedString.Builder builderForValue) {
if (localizedRichTextContentBuilder_ == null) {
localizedRichTextContent_ = builderForValue.build();
onChanged();
} else {
localizedRichTextContentBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Localized rich text content.
*
*
* .io.LocalizedString localizedRichTextContent = 7;
*/
public Builder mergeLocalizedRichTextContent(com.passkit.grpc.Localization.LocalizedString value) {
if (localizedRichTextContentBuilder_ == null) {
if (localizedRichTextContent_ != null) {
localizedRichTextContent_ =
com.passkit.grpc.Localization.LocalizedString.newBuilder(localizedRichTextContent_).mergeFrom(value).buildPartial();
} else {
localizedRichTextContent_ = value;
}
onChanged();
} else {
localizedRichTextContentBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Localized rich text content.
*
*
* .io.LocalizedString localizedRichTextContent = 7;
*/
public Builder clearLocalizedRichTextContent() {
if (localizedRichTextContentBuilder_ == null) {
localizedRichTextContent_ = null;
onChanged();
} else {
localizedRichTextContent_ = null;
localizedRichTextContentBuilder_ = null;
}
return this;
}
/**
*
* Localized rich text content.
*
*
* .io.LocalizedString localizedRichTextContent = 7;
*/
public com.passkit.grpc.Localization.LocalizedString.Builder getLocalizedRichTextContentBuilder() {
onChanged();
return getLocalizedRichTextContentFieldBuilder().getBuilder();
}
/**
*
* Localized rich text content.
*
*
* .io.LocalizedString localizedRichTextContent = 7;
*/
public com.passkit.grpc.Localization.LocalizedStringOrBuilder getLocalizedRichTextContentOrBuilder() {
if (localizedRichTextContentBuilder_ != null) {
return localizedRichTextContentBuilder_.getMessageOrBuilder();
} else {
return localizedRichTextContent_ == null ?
com.passkit.grpc.Localization.LocalizedString.getDefaultInstance() : localizedRichTextContent_;
}
}
/**
*
* Localized rich text content.
*
*
* .io.LocalizedString localizedRichTextContent = 7;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.passkit.grpc.Localization.LocalizedString, com.passkit.grpc.Localization.LocalizedString.Builder, com.passkit.grpc.Localization.LocalizedStringOrBuilder>
getLocalizedRichTextContentFieldBuilder() {
if (localizedRichTextContentBuilder_ == null) {
localizedRichTextContentBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.passkit.grpc.Localization.LocalizedString, com.passkit.grpc.Localization.LocalizedString.Builder, com.passkit.grpc.Localization.LocalizedStringOrBuilder>(
getLocalizedRichTextContent(),
getParentForChildren(),
isClean());
localizedRichTextContent_ = null;
}
return localizedRichTextContentBuilder_;
}
private java.util.List urls_ =
java.util.Collections.emptyList();
private void ensureUrlsIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
urls_ = new java.util.ArrayList(urls_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.passkit.grpc.CommonObjects.Url, com.passkit.grpc.CommonObjects.Url.Builder, com.passkit.grpc.CommonObjects.UrlOrBuilder> urlsBuilder_;
/**
*
* Urls will be prioritized over existing urls on the Google Pay Pass. They are ignored for Apple Wallet.
*
*
* repeated .io.Url urls = 8;
*/
public java.util.List getUrlsList() {
if (urlsBuilder_ == null) {
return java.util.Collections.unmodifiableList(urls_);
} else {
return urlsBuilder_.getMessageList();
}
}
/**
*
* Urls will be prioritized over existing urls on the Google Pay Pass. They are ignored for Apple Wallet.
*
*
* repeated .io.Url urls = 8;
*/
public int getUrlsCount() {
if (urlsBuilder_ == null) {
return urls_.size();
} else {
return urlsBuilder_.getCount();
}
}
/**
*
* Urls will be prioritized over existing urls on the Google Pay Pass. They are ignored for Apple Wallet.
*
*
* repeated .io.Url urls = 8;
*/
public com.passkit.grpc.CommonObjects.Url getUrls(int index) {
if (urlsBuilder_ == null) {
return urls_.get(index);
} else {
return urlsBuilder_.getMessage(index);
}
}
/**
*
* Urls will be prioritized over existing urls on the Google Pay Pass. They are ignored for Apple Wallet.
*
*
* repeated .io.Url urls = 8;
*/
public Builder setUrls(
int index, com.passkit.grpc.CommonObjects.Url value) {
if (urlsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureUrlsIsMutable();
urls_.set(index, value);
onChanged();
} else {
urlsBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* Urls will be prioritized over existing urls on the Google Pay Pass. They are ignored for Apple Wallet.
*
*
* repeated .io.Url urls = 8;
*/
public Builder setUrls(
int index, com.passkit.grpc.CommonObjects.Url.Builder builderForValue) {
if (urlsBuilder_ == null) {
ensureUrlsIsMutable();
urls_.set(index, builderForValue.build());
onChanged();
} else {
urlsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Urls will be prioritized over existing urls on the Google Pay Pass. They are ignored for Apple Wallet.
*
*
* repeated .io.Url urls = 8;
*/
public Builder addUrls(com.passkit.grpc.CommonObjects.Url value) {
if (urlsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureUrlsIsMutable();
urls_.add(value);
onChanged();
} else {
urlsBuilder_.addMessage(value);
}
return this;
}
/**
*
* Urls will be prioritized over existing urls on the Google Pay Pass. They are ignored for Apple Wallet.
*
*
* repeated .io.Url urls = 8;
*/
public Builder addUrls(
int index, com.passkit.grpc.CommonObjects.Url value) {
if (urlsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureUrlsIsMutable();
urls_.add(index, value);
onChanged();
} else {
urlsBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* Urls will be prioritized over existing urls on the Google Pay Pass. They are ignored for Apple Wallet.
*
*
* repeated .io.Url urls = 8;
*/
public Builder addUrls(
com.passkit.grpc.CommonObjects.Url.Builder builderForValue) {
if (urlsBuilder_ == null) {
ensureUrlsIsMutable();
urls_.add(builderForValue.build());
onChanged();
} else {
urlsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* Urls will be prioritized over existing urls on the Google Pay Pass. They are ignored for Apple Wallet.
*
*
* repeated .io.Url urls = 8;
*/
public Builder addUrls(
int index, com.passkit.grpc.CommonObjects.Url.Builder builderForValue) {
if (urlsBuilder_ == null) {
ensureUrlsIsMutable();
urls_.add(index, builderForValue.build());
onChanged();
} else {
urlsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Urls will be prioritized over existing urls on the Google Pay Pass. They are ignored for Apple Wallet.
*
*
* repeated .io.Url urls = 8;
*/
public Builder addAllUrls(
java.lang.Iterable extends com.passkit.grpc.CommonObjects.Url> values) {
if (urlsBuilder_ == null) {
ensureUrlsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, urls_);
onChanged();
} else {
urlsBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* Urls will be prioritized over existing urls on the Google Pay Pass. They are ignored for Apple Wallet.
*
*
* repeated .io.Url urls = 8;
*/
public Builder clearUrls() {
if (urlsBuilder_ == null) {
urls_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
urlsBuilder_.clear();
}
return this;
}
/**
*
* Urls will be prioritized over existing urls on the Google Pay Pass. They are ignored for Apple Wallet.
*
*
* repeated .io.Url urls = 8;
*/
public Builder removeUrls(int index) {
if (urlsBuilder_ == null) {
ensureUrlsIsMutable();
urls_.remove(index);
onChanged();
} else {
urlsBuilder_.remove(index);
}
return this;
}
/**
*
* Urls will be prioritized over existing urls on the Google Pay Pass. They are ignored for Apple Wallet.
*
*
* repeated .io.Url urls = 8;
*/
public com.passkit.grpc.CommonObjects.Url.Builder getUrlsBuilder(
int index) {
return getUrlsFieldBuilder().getBuilder(index);
}
/**
*
* Urls will be prioritized over existing urls on the Google Pay Pass. They are ignored for Apple Wallet.
*
*
* repeated .io.Url urls = 8;
*/
public com.passkit.grpc.CommonObjects.UrlOrBuilder getUrlsOrBuilder(
int index) {
if (urlsBuilder_ == null) {
return urls_.get(index); } else {
return urlsBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* Urls will be prioritized over existing urls on the Google Pay Pass. They are ignored for Apple Wallet.
*
*
* repeated .io.Url urls = 8;
*/
public java.util.List extends com.passkit.grpc.CommonObjects.UrlOrBuilder>
getUrlsOrBuilderList() {
if (urlsBuilder_ != null) {
return urlsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(urls_);
}
}
/**
*
* Urls will be prioritized over existing urls on the Google Pay Pass. They are ignored for Apple Wallet.
*
*
* repeated .io.Url urls = 8;
*/
public com.passkit.grpc.CommonObjects.Url.Builder addUrlsBuilder() {
return getUrlsFieldBuilder().addBuilder(
com.passkit.grpc.CommonObjects.Url.getDefaultInstance());
}
/**
*
* Urls will be prioritized over existing urls on the Google Pay Pass. They are ignored for Apple Wallet.
*
*
* repeated .io.Url urls = 8;
*/
public com.passkit.grpc.CommonObjects.Url.Builder addUrlsBuilder(
int index) {
return getUrlsFieldBuilder().addBuilder(
index, com.passkit.grpc.CommonObjects.Url.getDefaultInstance());
}
/**
*
* Urls will be prioritized over existing urls on the Google Pay Pass. They are ignored for Apple Wallet.
*
*
* repeated .io.Url urls = 8;
*/
public java.util.List
getUrlsBuilderList() {
return getUrlsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.passkit.grpc.CommonObjects.Url, com.passkit.grpc.CommonObjects.Url.Builder, com.passkit.grpc.CommonObjects.UrlOrBuilder>
getUrlsFieldBuilder() {
if (urlsBuilder_ == null) {
urlsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.passkit.grpc.CommonObjects.Url, com.passkit.grpc.CommonObjects.Url.Builder, com.passkit.grpc.CommonObjects.UrlOrBuilder>(
urls_,
((bitField0_ & 0x00000001) != 0),
getParentForChildren(),
isClean());
urls_ = null;
}
return urlsBuilder_;
}
private com.passkit.grpc.Image.ImageIds images_;
private com.google.protobuf.SingleFieldBuilderV3<
com.passkit.grpc.Image.ImageIds, com.passkit.grpc.Image.ImageIds.Builder, com.passkit.grpc.Image.ImageIdsOrBuilder> imagesBuilder_;
/**
*
* Images to display for the duration of the message. For Google Pay, only a 'message' type image will be used. For Apple Wallet, any of strip, eventStrip, background, logo, thumbnail and footer can be used, depending on the underlying pass type.
*
*
* .io.ImageIds images = 9;
* @return Whether the images field is set.
*/
public boolean hasImages() {
return imagesBuilder_ != null || images_ != null;
}
/**
*
* Images to display for the duration of the message. For Google Pay, only a 'message' type image will be used. For Apple Wallet, any of strip, eventStrip, background, logo, thumbnail and footer can be used, depending on the underlying pass type.
*
*
* .io.ImageIds images = 9;
* @return The images.
*/
public com.passkit.grpc.Image.ImageIds getImages() {
if (imagesBuilder_ == null) {
return images_ == null ? com.passkit.grpc.Image.ImageIds.getDefaultInstance() : images_;
} else {
return imagesBuilder_.getMessage();
}
}
/**
*
* Images to display for the duration of the message. For Google Pay, only a 'message' type image will be used. For Apple Wallet, any of strip, eventStrip, background, logo, thumbnail and footer can be used, depending on the underlying pass type.
*
*
* .io.ImageIds images = 9;
*/
public Builder setImages(com.passkit.grpc.Image.ImageIds value) {
if (imagesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
images_ = value;
onChanged();
} else {
imagesBuilder_.setMessage(value);
}
return this;
}
/**
*
* Images to display for the duration of the message. For Google Pay, only a 'message' type image will be used. For Apple Wallet, any of strip, eventStrip, background, logo, thumbnail and footer can be used, depending on the underlying pass type.
*
*
* .io.ImageIds images = 9;
*/
public Builder setImages(
com.passkit.grpc.Image.ImageIds.Builder builderForValue) {
if (imagesBuilder_ == null) {
images_ = builderForValue.build();
onChanged();
} else {
imagesBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Images to display for the duration of the message. For Google Pay, only a 'message' type image will be used. For Apple Wallet, any of strip, eventStrip, background, logo, thumbnail and footer can be used, depending on the underlying pass type.
*
*
* .io.ImageIds images = 9;
*/
public Builder mergeImages(com.passkit.grpc.Image.ImageIds value) {
if (imagesBuilder_ == null) {
if (images_ != null) {
images_ =
com.passkit.grpc.Image.ImageIds.newBuilder(images_).mergeFrom(value).buildPartial();
} else {
images_ = value;
}
onChanged();
} else {
imagesBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Images to display for the duration of the message. For Google Pay, only a 'message' type image will be used. For Apple Wallet, any of strip, eventStrip, background, logo, thumbnail and footer can be used, depending on the underlying pass type.
*
*
* .io.ImageIds images = 9;
*/
public Builder clearImages() {
if (imagesBuilder_ == null) {
images_ = null;
onChanged();
} else {
images_ = null;
imagesBuilder_ = null;
}
return this;
}
/**
*
* Images to display for the duration of the message. For Google Pay, only a 'message' type image will be used. For Apple Wallet, any of strip, eventStrip, background, logo, thumbnail and footer can be used, depending on the underlying pass type.
*
*
* .io.ImageIds images = 9;
*/
public com.passkit.grpc.Image.ImageIds.Builder getImagesBuilder() {
onChanged();
return getImagesFieldBuilder().getBuilder();
}
/**
*
* Images to display for the duration of the message. For Google Pay, only a 'message' type image will be used. For Apple Wallet, any of strip, eventStrip, background, logo, thumbnail and footer can be used, depending on the underlying pass type.
*
*
* .io.ImageIds images = 9;
*/
public com.passkit.grpc.Image.ImageIdsOrBuilder getImagesOrBuilder() {
if (imagesBuilder_ != null) {
return imagesBuilder_.getMessageOrBuilder();
} else {
return images_ == null ?
com.passkit.grpc.Image.ImageIds.getDefaultInstance() : images_;
}
}
/**
*
* Images to display for the duration of the message. For Google Pay, only a 'message' type image will be used. For Apple Wallet, any of strip, eventStrip, background, logo, thumbnail and footer can be used, depending on the underlying pass type.
*
*
* .io.ImageIds images = 9;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.passkit.grpc.Image.ImageIds, com.passkit.grpc.Image.ImageIds.Builder, com.passkit.grpc.Image.ImageIdsOrBuilder>
getImagesFieldBuilder() {
if (imagesBuilder_ == null) {
imagesBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.passkit.grpc.Image.ImageIds, com.passkit.grpc.Image.ImageIds.Builder, com.passkit.grpc.Image.ImageIdsOrBuilder>(
getImages(),
getParentForChildren(),
isClean());
images_ = null;
}
return imagesBuilder_;
}
private int priority_ ;
/**
*
* Priority the lower the priority, the lower down the message will be rendered when there are multiple messages. Defaults to a value of 10.
*
*
* uint32 priority = 10;
* @return The priority.
*/
@java.lang.Override
public int getPriority() {
return priority_;
}
/**
*
* Priority the lower the priority, the lower down the message will be rendered when there are multiple messages. Defaults to a value of 10.
*
*
* uint32 priority = 10;
* @param value The priority to set.
* @return This builder for chaining.
*/
public Builder setPriority(int value) {
priority_ = value;
onChanged();
return this;
}
/**
*
* Priority the lower the priority, the lower down the message will be rendered when there are multiple messages. Defaults to a value of 10.
*
*
* uint32 priority = 10;
* @return This builder for chaining.
*/
public Builder clearPriority() {
priority_ = 0;
onChanged();
return this;
}
private java.lang.Object displayFrom_ = "";
/**
*
* The date and time to display the message. The value must be in RFC3339 format. If omitted, the current timestamp will be used.
*
*
* string displayFrom = 11;
* @return The displayFrom.
*/
public java.lang.String getDisplayFrom() {
java.lang.Object ref = displayFrom_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
displayFrom_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* The date and time to display the message. The value must be in RFC3339 format. If omitted, the current timestamp will be used.
*
*
* string displayFrom = 11;
* @return The bytes for displayFrom.
*/
public com.google.protobuf.ByteString
getDisplayFromBytes() {
java.lang.Object ref = displayFrom_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
displayFrom_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* The date and time to display the message. The value must be in RFC3339 format. If omitted, the current timestamp will be used.
*
*
* string displayFrom = 11;
* @param value The displayFrom to set.
* @return This builder for chaining.
*/
public Builder setDisplayFrom(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
displayFrom_ = value;
onChanged();
return this;
}
/**
*
* The date and time to display the message. The value must be in RFC3339 format. If omitted, the current timestamp will be used.
*
*
* string displayFrom = 11;
* @return This builder for chaining.
*/
public Builder clearDisplayFrom() {
displayFrom_ = getDefaultInstance().getDisplayFrom();
onChanged();
return this;
}
/**
*
* The date and time to display the message. The value must be in RFC3339 format. If omitted, the current timestamp will be used.
*
*
* string displayFrom = 11;
* @param value The bytes for displayFrom to set.
* @return This builder for chaining.
*/
public Builder setDisplayFromBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
displayFrom_ = value;
onChanged();
return this;
}
private java.lang.Object displayUntil_ = "";
/**
*
* The date and time to remove the message. The value must be in RFC3339 format. If omitted, the message will be displayed indefinitely until it is cancelled.
*
*
* string displayUntil = 12;
* @return The displayUntil.
*/
public java.lang.String getDisplayUntil() {
java.lang.Object ref = displayUntil_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
displayUntil_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* The date and time to remove the message. The value must be in RFC3339 format. If omitted, the message will be displayed indefinitely until it is cancelled.
*
*
* string displayUntil = 12;
* @return The bytes for displayUntil.
*/
public com.google.protobuf.ByteString
getDisplayUntilBytes() {
java.lang.Object ref = displayUntil_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
displayUntil_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* The date and time to remove the message. The value must be in RFC3339 format. If omitted, the message will be displayed indefinitely until it is cancelled.
*
*
* string displayUntil = 12;
* @param value The displayUntil to set.
* @return This builder for chaining.
*/
public Builder setDisplayUntil(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
displayUntil_ = value;
onChanged();
return this;
}
/**
*
* The date and time to remove the message. The value must be in RFC3339 format. If omitted, the message will be displayed indefinitely until it is cancelled.
*
*
* string displayUntil = 12;
* @return This builder for chaining.
*/
public Builder clearDisplayUntil() {
displayUntil_ = getDefaultInstance().getDisplayUntil();
onChanged();
return this;
}
/**
*
* The date and time to remove the message. The value must be in RFC3339 format. If omitted, the message will be displayed indefinitely until it is cancelled.
*
*
* string displayUntil = 12;
* @param value The bytes for displayUntil to set.
* @return This builder for chaining.
*/
public Builder setDisplayUntilBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
displayUntil_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:io.Message)
}
// @@protoc_insertion_point(class_scope:io.Message)
private static final com.passkit.grpc.MessageOuterClass.Message DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.passkit.grpc.MessageOuterClass.Message();
}
public static com.passkit.grpc.MessageOuterClass.Message getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Message parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Message(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.passkit.grpc.MessageOuterClass.Message getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface GetMessagesForProtocolRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:io.GetMessagesForProtocolRequest)
com.google.protobuf.MessageOrBuilder {
/**
*
* Optional protocol. If no protocol is provided, all messages for the company that meet the pagination criteria will be returned.
* @tag "validateGeneric:required_with:ClassId"
*
*
* string protocol = 1;
* @return The protocol.
*/
java.lang.String getProtocol();
/**
*
* Optional protocol. If no protocol is provided, all messages for the company that meet the pagination criteria will be returned.
* @tag "validateGeneric:required_with:ClassId"
*
*
* string protocol = 1;
* @return The bytes for protocol.
*/
com.google.protobuf.ByteString
getProtocolBytes();
/**
*
* Optional class id must be provided if protocol is not empty.
* @tag "validateGeneric:required_with:Protocol"
*
*
* string classId = 2;
* @return The classId.
*/
java.lang.String getClassId();
/**
*
* Optional class id must be provided if protocol is not empty.
* @tag "validateGeneric:required_with:Protocol"
*
*
* string classId = 2;
* @return The bytes for classId.
*/
com.google.protobuf.ByteString
getClassIdBytes();
/**
*
* Optional pagination criteria. If not provided, then all messages for the class will be returned. Ignored if protocol not provided.
*
*
* .io.Pagination pagination = 3;
* @return Whether the pagination field is set.
*/
boolean hasPagination();
/**
*
* Optional pagination criteria. If not provided, then all messages for the class will be returned. Ignored if protocol not provided.
*
*
* .io.Pagination pagination = 3;
* @return The pagination.
*/
com.passkit.grpc.PaginationOuterClass.Pagination getPagination();
/**
*
* Optional pagination criteria. If not provided, then all messages for the class will be returned. Ignored if protocol not provided.
*
*
* .io.Pagination pagination = 3;
*/
com.passkit.grpc.PaginationOuterClass.PaginationOrBuilder getPaginationOrBuilder();
}
/**
*
* If no parameters are provided, all messages will be returned
*
*
* Protobuf type {@code io.GetMessagesForProtocolRequest}
*/
public static final class GetMessagesForProtocolRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:io.GetMessagesForProtocolRequest)
GetMessagesForProtocolRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use GetMessagesForProtocolRequest.newBuilder() to construct.
private GetMessagesForProtocolRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private GetMessagesForProtocolRequest() {
protocol_ = "";
classId_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new GetMessagesForProtocolRequest();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private GetMessagesForProtocolRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
protocol_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
classId_ = s;
break;
}
case 26: {
com.passkit.grpc.PaginationOuterClass.Pagination.Builder subBuilder = null;
if (pagination_ != null) {
subBuilder = pagination_.toBuilder();
}
pagination_ = input.readMessage(com.passkit.grpc.PaginationOuterClass.Pagination.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(pagination_);
pagination_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.passkit.grpc.MessageOuterClass.internal_static_io_GetMessagesForProtocolRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.passkit.grpc.MessageOuterClass.internal_static_io_GetMessagesForProtocolRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.passkit.grpc.MessageOuterClass.GetMessagesForProtocolRequest.class, com.passkit.grpc.MessageOuterClass.GetMessagesForProtocolRequest.Builder.class);
}
public static final int PROTOCOL_FIELD_NUMBER = 1;
private volatile java.lang.Object protocol_;
/**
*
* Optional protocol. If no protocol is provided, all messages for the company that meet the pagination criteria will be returned.
* @tag "validateGeneric:required_with:ClassId"
*
*
* string protocol = 1;
* @return The protocol.
*/
@java.lang.Override
public java.lang.String getProtocol() {
java.lang.Object ref = protocol_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
protocol_ = s;
return s;
}
}
/**
*
* Optional protocol. If no protocol is provided, all messages for the company that meet the pagination criteria will be returned.
* @tag "validateGeneric:required_with:ClassId"
*
*
* string protocol = 1;
* @return The bytes for protocol.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getProtocolBytes() {
java.lang.Object ref = protocol_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
protocol_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CLASSID_FIELD_NUMBER = 2;
private volatile java.lang.Object classId_;
/**
*
* Optional class id must be provided if protocol is not empty.
* @tag "validateGeneric:required_with:Protocol"
*
*
* string classId = 2;
* @return The classId.
*/
@java.lang.Override
public java.lang.String getClassId() {
java.lang.Object ref = classId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
classId_ = s;
return s;
}
}
/**
*
* Optional class id must be provided if protocol is not empty.
* @tag "validateGeneric:required_with:Protocol"
*
*
* string classId = 2;
* @return The bytes for classId.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getClassIdBytes() {
java.lang.Object ref = classId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
classId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int PAGINATION_FIELD_NUMBER = 3;
private com.passkit.grpc.PaginationOuterClass.Pagination pagination_;
/**
*
* Optional pagination criteria. If not provided, then all messages for the class will be returned. Ignored if protocol not provided.
*
*
* .io.Pagination pagination = 3;
* @return Whether the pagination field is set.
*/
@java.lang.Override
public boolean hasPagination() {
return pagination_ != null;
}
/**
*
* Optional pagination criteria. If not provided, then all messages for the class will be returned. Ignored if protocol not provided.
*
*
* .io.Pagination pagination = 3;
* @return The pagination.
*/
@java.lang.Override
public com.passkit.grpc.PaginationOuterClass.Pagination getPagination() {
return pagination_ == null ? com.passkit.grpc.PaginationOuterClass.Pagination.getDefaultInstance() : pagination_;
}
/**
*
* Optional pagination criteria. If not provided, then all messages for the class will be returned. Ignored if protocol not provided.
*
*
* .io.Pagination pagination = 3;
*/
@java.lang.Override
public com.passkit.grpc.PaginationOuterClass.PaginationOrBuilder getPaginationOrBuilder() {
return getPagination();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(protocol_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, protocol_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(classId_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, classId_);
}
if (pagination_ != null) {
output.writeMessage(3, getPagination());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(protocol_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, protocol_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(classId_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, classId_);
}
if (pagination_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getPagination());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.passkit.grpc.MessageOuterClass.GetMessagesForProtocolRequest)) {
return super.equals(obj);
}
com.passkit.grpc.MessageOuterClass.GetMessagesForProtocolRequest other = (com.passkit.grpc.MessageOuterClass.GetMessagesForProtocolRequest) obj;
if (!getProtocol()
.equals(other.getProtocol())) return false;
if (!getClassId()
.equals(other.getClassId())) return false;
if (hasPagination() != other.hasPagination()) return false;
if (hasPagination()) {
if (!getPagination()
.equals(other.getPagination())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + PROTOCOL_FIELD_NUMBER;
hash = (53 * hash) + getProtocol().hashCode();
hash = (37 * hash) + CLASSID_FIELD_NUMBER;
hash = (53 * hash) + getClassId().hashCode();
if (hasPagination()) {
hash = (37 * hash) + PAGINATION_FIELD_NUMBER;
hash = (53 * hash) + getPagination().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.passkit.grpc.MessageOuterClass.GetMessagesForProtocolRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.passkit.grpc.MessageOuterClass.GetMessagesForProtocolRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.passkit.grpc.MessageOuterClass.GetMessagesForProtocolRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.passkit.grpc.MessageOuterClass.GetMessagesForProtocolRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.passkit.grpc.MessageOuterClass.GetMessagesForProtocolRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.passkit.grpc.MessageOuterClass.GetMessagesForProtocolRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.passkit.grpc.MessageOuterClass.GetMessagesForProtocolRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.passkit.grpc.MessageOuterClass.GetMessagesForProtocolRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.passkit.grpc.MessageOuterClass.GetMessagesForProtocolRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.passkit.grpc.MessageOuterClass.GetMessagesForProtocolRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.passkit.grpc.MessageOuterClass.GetMessagesForProtocolRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.passkit.grpc.MessageOuterClass.GetMessagesForProtocolRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.passkit.grpc.MessageOuterClass.GetMessagesForProtocolRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* If no parameters are provided, all messages will be returned
*
*
* Protobuf type {@code io.GetMessagesForProtocolRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:io.GetMessagesForProtocolRequest)
com.passkit.grpc.MessageOuterClass.GetMessagesForProtocolRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.passkit.grpc.MessageOuterClass.internal_static_io_GetMessagesForProtocolRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.passkit.grpc.MessageOuterClass.internal_static_io_GetMessagesForProtocolRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.passkit.grpc.MessageOuterClass.GetMessagesForProtocolRequest.class, com.passkit.grpc.MessageOuterClass.GetMessagesForProtocolRequest.Builder.class);
}
// Construct using com.passkit.grpc.MessageOuterClass.GetMessagesForProtocolRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
protocol_ = "";
classId_ = "";
if (paginationBuilder_ == null) {
pagination_ = null;
} else {
pagination_ = null;
paginationBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.passkit.grpc.MessageOuterClass.internal_static_io_GetMessagesForProtocolRequest_descriptor;
}
@java.lang.Override
public com.passkit.grpc.MessageOuterClass.GetMessagesForProtocolRequest getDefaultInstanceForType() {
return com.passkit.grpc.MessageOuterClass.GetMessagesForProtocolRequest.getDefaultInstance();
}
@java.lang.Override
public com.passkit.grpc.MessageOuterClass.GetMessagesForProtocolRequest build() {
com.passkit.grpc.MessageOuterClass.GetMessagesForProtocolRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.passkit.grpc.MessageOuterClass.GetMessagesForProtocolRequest buildPartial() {
com.passkit.grpc.MessageOuterClass.GetMessagesForProtocolRequest result = new com.passkit.grpc.MessageOuterClass.GetMessagesForProtocolRequest(this);
result.protocol_ = protocol_;
result.classId_ = classId_;
if (paginationBuilder_ == null) {
result.pagination_ = pagination_;
} else {
result.pagination_ = paginationBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.passkit.grpc.MessageOuterClass.GetMessagesForProtocolRequest) {
return mergeFrom((com.passkit.grpc.MessageOuterClass.GetMessagesForProtocolRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.passkit.grpc.MessageOuterClass.GetMessagesForProtocolRequest other) {
if (other == com.passkit.grpc.MessageOuterClass.GetMessagesForProtocolRequest.getDefaultInstance()) return this;
if (!other.getProtocol().isEmpty()) {
protocol_ = other.protocol_;
onChanged();
}
if (!other.getClassId().isEmpty()) {
classId_ = other.classId_;
onChanged();
}
if (other.hasPagination()) {
mergePagination(other.getPagination());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.passkit.grpc.MessageOuterClass.GetMessagesForProtocolRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.passkit.grpc.MessageOuterClass.GetMessagesForProtocolRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object protocol_ = "";
/**
*
* Optional protocol. If no protocol is provided, all messages for the company that meet the pagination criteria will be returned.
* @tag "validateGeneric:required_with:ClassId"
*
*
* string protocol = 1;
* @return The protocol.
*/
public java.lang.String getProtocol() {
java.lang.Object ref = protocol_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
protocol_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Optional protocol. If no protocol is provided, all messages for the company that meet the pagination criteria will be returned.
* @tag "validateGeneric:required_with:ClassId"
*
*
* string protocol = 1;
* @return The bytes for protocol.
*/
public com.google.protobuf.ByteString
getProtocolBytes() {
java.lang.Object ref = protocol_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
protocol_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Optional protocol. If no protocol is provided, all messages for the company that meet the pagination criteria will be returned.
* @tag "validateGeneric:required_with:ClassId"
*
*
* string protocol = 1;
* @param value The protocol to set.
* @return This builder for chaining.
*/
public Builder setProtocol(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
protocol_ = value;
onChanged();
return this;
}
/**
*
* Optional protocol. If no protocol is provided, all messages for the company that meet the pagination criteria will be returned.
* @tag "validateGeneric:required_with:ClassId"
*
*
* string protocol = 1;
* @return This builder for chaining.
*/
public Builder clearProtocol() {
protocol_ = getDefaultInstance().getProtocol();
onChanged();
return this;
}
/**
*
* Optional protocol. If no protocol is provided, all messages for the company that meet the pagination criteria will be returned.
* @tag "validateGeneric:required_with:ClassId"
*
*
* string protocol = 1;
* @param value The bytes for protocol to set.
* @return This builder for chaining.
*/
public Builder setProtocolBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
protocol_ = value;
onChanged();
return this;
}
private java.lang.Object classId_ = "";
/**
*
* Optional class id must be provided if protocol is not empty.
* @tag "validateGeneric:required_with:Protocol"
*
*
* string classId = 2;
* @return The classId.
*/
public java.lang.String getClassId() {
java.lang.Object ref = classId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
classId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Optional class id must be provided if protocol is not empty.
* @tag "validateGeneric:required_with:Protocol"
*
*
* string classId = 2;
* @return The bytes for classId.
*/
public com.google.protobuf.ByteString
getClassIdBytes() {
java.lang.Object ref = classId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
classId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Optional class id must be provided if protocol is not empty.
* @tag "validateGeneric:required_with:Protocol"
*
*
* string classId = 2;
* @param value The classId to set.
* @return This builder for chaining.
*/
public Builder setClassId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
classId_ = value;
onChanged();
return this;
}
/**
*
* Optional class id must be provided if protocol is not empty.
* @tag "validateGeneric:required_with:Protocol"
*
*
* string classId = 2;
* @return This builder for chaining.
*/
public Builder clearClassId() {
classId_ = getDefaultInstance().getClassId();
onChanged();
return this;
}
/**
*
* Optional class id must be provided if protocol is not empty.
* @tag "validateGeneric:required_with:Protocol"
*
*
* string classId = 2;
* @param value The bytes for classId to set.
* @return This builder for chaining.
*/
public Builder setClassIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
classId_ = value;
onChanged();
return this;
}
private com.passkit.grpc.PaginationOuterClass.Pagination pagination_;
private com.google.protobuf.SingleFieldBuilderV3<
com.passkit.grpc.PaginationOuterClass.Pagination, com.passkit.grpc.PaginationOuterClass.Pagination.Builder, com.passkit.grpc.PaginationOuterClass.PaginationOrBuilder> paginationBuilder_;
/**
*
* Optional pagination criteria. If not provided, then all messages for the class will be returned. Ignored if protocol not provided.
*
*
* .io.Pagination pagination = 3;
* @return Whether the pagination field is set.
*/
public boolean hasPagination() {
return paginationBuilder_ != null || pagination_ != null;
}
/**
*
* Optional pagination criteria. If not provided, then all messages for the class will be returned. Ignored if protocol not provided.
*
*
* .io.Pagination pagination = 3;
* @return The pagination.
*/
public com.passkit.grpc.PaginationOuterClass.Pagination getPagination() {
if (paginationBuilder_ == null) {
return pagination_ == null ? com.passkit.grpc.PaginationOuterClass.Pagination.getDefaultInstance() : pagination_;
} else {
return paginationBuilder_.getMessage();
}
}
/**
*
* Optional pagination criteria. If not provided, then all messages for the class will be returned. Ignored if protocol not provided.
*
*
* .io.Pagination pagination = 3;
*/
public Builder setPagination(com.passkit.grpc.PaginationOuterClass.Pagination value) {
if (paginationBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
pagination_ = value;
onChanged();
} else {
paginationBuilder_.setMessage(value);
}
return this;
}
/**
*
* Optional pagination criteria. If not provided, then all messages for the class will be returned. Ignored if protocol not provided.
*
*
* .io.Pagination pagination = 3;
*/
public Builder setPagination(
com.passkit.grpc.PaginationOuterClass.Pagination.Builder builderForValue) {
if (paginationBuilder_ == null) {
pagination_ = builderForValue.build();
onChanged();
} else {
paginationBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Optional pagination criteria. If not provided, then all messages for the class will be returned. Ignored if protocol not provided.
*
*
* .io.Pagination pagination = 3;
*/
public Builder mergePagination(com.passkit.grpc.PaginationOuterClass.Pagination value) {
if (paginationBuilder_ == null) {
if (pagination_ != null) {
pagination_ =
com.passkit.grpc.PaginationOuterClass.Pagination.newBuilder(pagination_).mergeFrom(value).buildPartial();
} else {
pagination_ = value;
}
onChanged();
} else {
paginationBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Optional pagination criteria. If not provided, then all messages for the class will be returned. Ignored if protocol not provided.
*
*
* .io.Pagination pagination = 3;
*/
public Builder clearPagination() {
if (paginationBuilder_ == null) {
pagination_ = null;
onChanged();
} else {
pagination_ = null;
paginationBuilder_ = null;
}
return this;
}
/**
*
* Optional pagination criteria. If not provided, then all messages for the class will be returned. Ignored if protocol not provided.
*
*
* .io.Pagination pagination = 3;
*/
public com.passkit.grpc.PaginationOuterClass.Pagination.Builder getPaginationBuilder() {
onChanged();
return getPaginationFieldBuilder().getBuilder();
}
/**
*
* Optional pagination criteria. If not provided, then all messages for the class will be returned. Ignored if protocol not provided.
*
*
* .io.Pagination pagination = 3;
*/
public com.passkit.grpc.PaginationOuterClass.PaginationOrBuilder getPaginationOrBuilder() {
if (paginationBuilder_ != null) {
return paginationBuilder_.getMessageOrBuilder();
} else {
return pagination_ == null ?
com.passkit.grpc.PaginationOuterClass.Pagination.getDefaultInstance() : pagination_;
}
}
/**
*
* Optional pagination criteria. If not provided, then all messages for the class will be returned. Ignored if protocol not provided.
*
*
* .io.Pagination pagination = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.passkit.grpc.PaginationOuterClass.Pagination, com.passkit.grpc.PaginationOuterClass.Pagination.Builder, com.passkit.grpc.PaginationOuterClass.PaginationOrBuilder>
getPaginationFieldBuilder() {
if (paginationBuilder_ == null) {
paginationBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.passkit.grpc.PaginationOuterClass.Pagination, com.passkit.grpc.PaginationOuterClass.Pagination.Builder, com.passkit.grpc.PaginationOuterClass.PaginationOrBuilder>(
getPagination(),
getParentForChildren(),
isClean());
pagination_ = null;
}
return paginationBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:io.GetMessagesForProtocolRequest)
}
// @@protoc_insertion_point(class_scope:io.GetMessagesForProtocolRequest)
private static final com.passkit.grpc.MessageOuterClass.GetMessagesForProtocolRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.passkit.grpc.MessageOuterClass.GetMessagesForProtocolRequest();
}
public static com.passkit.grpc.MessageOuterClass.GetMessagesForProtocolRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public GetMessagesForProtocolRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new GetMessagesForProtocolRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.passkit.grpc.MessageOuterClass.GetMessagesForProtocolRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface GetMessageResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:io.GetMessageResponse)
com.google.protobuf.MessageOrBuilder {
/**
* .io.Message message = 1;
* @return Whether the message field is set.
*/
boolean hasMessage();
/**
* .io.Message message = 1;
* @return The message.
*/
com.passkit.grpc.MessageOuterClass.Message getMessage();
/**
* .io.Message message = 1;
*/
com.passkit.grpc.MessageOuterClass.MessageOrBuilder getMessageOrBuilder();
/**
* .io.MessageStatus status = 2;
* @return The enum numeric value on the wire for status.
*/
int getStatusValue();
/**
* .io.MessageStatus status = 2;
* @return The status.
*/
com.passkit.grpc.MessageOuterClass.MessageStatus getStatus();
/**
* uint32 recipients = 3;
* @return The recipients.
*/
int getRecipients();
/**
* .google.protobuf.Timestamp distributedAt = 4;
* @return Whether the distributedAt field is set.
*/
boolean hasDistributedAt();
/**
* .google.protobuf.Timestamp distributedAt = 4;
* @return The distributedAt.
*/
com.google.protobuf.Timestamp getDistributedAt();
/**
* .google.protobuf.Timestamp distributedAt = 4;
*/
com.google.protobuf.TimestampOrBuilder getDistributedAtOrBuilder();
/**
* .google.protobuf.Timestamp withdrawnAt = 5;
* @return Whether the withdrawnAt field is set.
*/
boolean hasWithdrawnAt();
/**
* .google.protobuf.Timestamp withdrawnAt = 5;
* @return The withdrawnAt.
*/
com.google.protobuf.Timestamp getWithdrawnAt();
/**
* .google.protobuf.Timestamp withdrawnAt = 5;
*/
com.google.protobuf.TimestampOrBuilder getWithdrawnAtOrBuilder();
/**
* .google.protobuf.Timestamp createdAt = 6;
* @return Whether the createdAt field is set.
*/
boolean hasCreatedAt();
/**
* .google.protobuf.Timestamp createdAt = 6;
* @return The createdAt.
*/
com.google.protobuf.Timestamp getCreatedAt();
/**
* .google.protobuf.Timestamp createdAt = 6;
*/
com.google.protobuf.TimestampOrBuilder getCreatedAtOrBuilder();
/**
* .google.protobuf.Timestamp updatedAt = 7;
* @return Whether the updatedAt field is set.
*/
boolean hasUpdatedAt();
/**
* .google.protobuf.Timestamp updatedAt = 7;
* @return The updatedAt.
*/
com.google.protobuf.Timestamp getUpdatedAt();
/**
* .google.protobuf.Timestamp updatedAt = 7;
*/
com.google.protobuf.TimestampOrBuilder getUpdatedAtOrBuilder();
}
/**
* Protobuf type {@code io.GetMessageResponse}
*/
public static final class GetMessageResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:io.GetMessageResponse)
GetMessageResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use GetMessageResponse.newBuilder() to construct.
private GetMessageResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private GetMessageResponse() {
status_ = 0;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new GetMessageResponse();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private GetMessageResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
com.passkit.grpc.MessageOuterClass.Message.Builder subBuilder = null;
if (message_ != null) {
subBuilder = message_.toBuilder();
}
message_ = input.readMessage(com.passkit.grpc.MessageOuterClass.Message.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(message_);
message_ = subBuilder.buildPartial();
}
break;
}
case 16: {
int rawValue = input.readEnum();
status_ = rawValue;
break;
}
case 24: {
recipients_ = input.readUInt32();
break;
}
case 34: {
com.google.protobuf.Timestamp.Builder subBuilder = null;
if (distributedAt_ != null) {
subBuilder = distributedAt_.toBuilder();
}
distributedAt_ = input.readMessage(com.google.protobuf.Timestamp.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(distributedAt_);
distributedAt_ = subBuilder.buildPartial();
}
break;
}
case 42: {
com.google.protobuf.Timestamp.Builder subBuilder = null;
if (withdrawnAt_ != null) {
subBuilder = withdrawnAt_.toBuilder();
}
withdrawnAt_ = input.readMessage(com.google.protobuf.Timestamp.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(withdrawnAt_);
withdrawnAt_ = subBuilder.buildPartial();
}
break;
}
case 50: {
com.google.protobuf.Timestamp.Builder subBuilder = null;
if (createdAt_ != null) {
subBuilder = createdAt_.toBuilder();
}
createdAt_ = input.readMessage(com.google.protobuf.Timestamp.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(createdAt_);
createdAt_ = subBuilder.buildPartial();
}
break;
}
case 58: {
com.google.protobuf.Timestamp.Builder subBuilder = null;
if (updatedAt_ != null) {
subBuilder = updatedAt_.toBuilder();
}
updatedAt_ = input.readMessage(com.google.protobuf.Timestamp.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(updatedAt_);
updatedAt_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.passkit.grpc.MessageOuterClass.internal_static_io_GetMessageResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.passkit.grpc.MessageOuterClass.internal_static_io_GetMessageResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.passkit.grpc.MessageOuterClass.GetMessageResponse.class, com.passkit.grpc.MessageOuterClass.GetMessageResponse.Builder.class);
}
public static final int MESSAGE_FIELD_NUMBER = 1;
private com.passkit.grpc.MessageOuterClass.Message message_;
/**
* .io.Message message = 1;
* @return Whether the message field is set.
*/
@java.lang.Override
public boolean hasMessage() {
return message_ != null;
}
/**
* .io.Message message = 1;
* @return The message.
*/
@java.lang.Override
public com.passkit.grpc.MessageOuterClass.Message getMessage() {
return message_ == null ? com.passkit.grpc.MessageOuterClass.Message.getDefaultInstance() : message_;
}
/**
* .io.Message message = 1;
*/
@java.lang.Override
public com.passkit.grpc.MessageOuterClass.MessageOrBuilder getMessageOrBuilder() {
return getMessage();
}
public static final int STATUS_FIELD_NUMBER = 2;
private int status_;
/**
* .io.MessageStatus status = 2;
* @return The enum numeric value on the wire for status.
*/
@java.lang.Override public int getStatusValue() {
return status_;
}
/**
* .io.MessageStatus status = 2;
* @return The status.
*/
@java.lang.Override public com.passkit.grpc.MessageOuterClass.MessageStatus getStatus() {
@SuppressWarnings("deprecation")
com.passkit.grpc.MessageOuterClass.MessageStatus result = com.passkit.grpc.MessageOuterClass.MessageStatus.valueOf(status_);
return result == null ? com.passkit.grpc.MessageOuterClass.MessageStatus.UNRECOGNIZED : result;
}
public static final int RECIPIENTS_FIELD_NUMBER = 3;
private int recipients_;
/**
* uint32 recipients = 3;
* @return The recipients.
*/
@java.lang.Override
public int getRecipients() {
return recipients_;
}
public static final int DISTRIBUTEDAT_FIELD_NUMBER = 4;
private com.google.protobuf.Timestamp distributedAt_;
/**
* .google.protobuf.Timestamp distributedAt = 4;
* @return Whether the distributedAt field is set.
*/
@java.lang.Override
public boolean hasDistributedAt() {
return distributedAt_ != null;
}
/**
* .google.protobuf.Timestamp distributedAt = 4;
* @return The distributedAt.
*/
@java.lang.Override
public com.google.protobuf.Timestamp getDistributedAt() {
return distributedAt_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : distributedAt_;
}
/**
* .google.protobuf.Timestamp distributedAt = 4;
*/
@java.lang.Override
public com.google.protobuf.TimestampOrBuilder getDistributedAtOrBuilder() {
return getDistributedAt();
}
public static final int WITHDRAWNAT_FIELD_NUMBER = 5;
private com.google.protobuf.Timestamp withdrawnAt_;
/**
* .google.protobuf.Timestamp withdrawnAt = 5;
* @return Whether the withdrawnAt field is set.
*/
@java.lang.Override
public boolean hasWithdrawnAt() {
return withdrawnAt_ != null;
}
/**
* .google.protobuf.Timestamp withdrawnAt = 5;
* @return The withdrawnAt.
*/
@java.lang.Override
public com.google.protobuf.Timestamp getWithdrawnAt() {
return withdrawnAt_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : withdrawnAt_;
}
/**
* .google.protobuf.Timestamp withdrawnAt = 5;
*/
@java.lang.Override
public com.google.protobuf.TimestampOrBuilder getWithdrawnAtOrBuilder() {
return getWithdrawnAt();
}
public static final int CREATEDAT_FIELD_NUMBER = 6;
private com.google.protobuf.Timestamp createdAt_;
/**
* .google.protobuf.Timestamp createdAt = 6;
* @return Whether the createdAt field is set.
*/
@java.lang.Override
public boolean hasCreatedAt() {
return createdAt_ != null;
}
/**
* .google.protobuf.Timestamp createdAt = 6;
* @return The createdAt.
*/
@java.lang.Override
public com.google.protobuf.Timestamp getCreatedAt() {
return createdAt_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : createdAt_;
}
/**
* .google.protobuf.Timestamp createdAt = 6;
*/
@java.lang.Override
public com.google.protobuf.TimestampOrBuilder getCreatedAtOrBuilder() {
return getCreatedAt();
}
public static final int UPDATEDAT_FIELD_NUMBER = 7;
private com.google.protobuf.Timestamp updatedAt_;
/**
* .google.protobuf.Timestamp updatedAt = 7;
* @return Whether the updatedAt field is set.
*/
@java.lang.Override
public boolean hasUpdatedAt() {
return updatedAt_ != null;
}
/**
* .google.protobuf.Timestamp updatedAt = 7;
* @return The updatedAt.
*/
@java.lang.Override
public com.google.protobuf.Timestamp getUpdatedAt() {
return updatedAt_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : updatedAt_;
}
/**
* .google.protobuf.Timestamp updatedAt = 7;
*/
@java.lang.Override
public com.google.protobuf.TimestampOrBuilder getUpdatedAtOrBuilder() {
return getUpdatedAt();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (message_ != null) {
output.writeMessage(1, getMessage());
}
if (status_ != com.passkit.grpc.MessageOuterClass.MessageStatus.MESSAGE_STATUS_DO_NOT_USE.getNumber()) {
output.writeEnum(2, status_);
}
if (recipients_ != 0) {
output.writeUInt32(3, recipients_);
}
if (distributedAt_ != null) {
output.writeMessage(4, getDistributedAt());
}
if (withdrawnAt_ != null) {
output.writeMessage(5, getWithdrawnAt());
}
if (createdAt_ != null) {
output.writeMessage(6, getCreatedAt());
}
if (updatedAt_ != null) {
output.writeMessage(7, getUpdatedAt());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (message_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getMessage());
}
if (status_ != com.passkit.grpc.MessageOuterClass.MessageStatus.MESSAGE_STATUS_DO_NOT_USE.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(2, status_);
}
if (recipients_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(3, recipients_);
}
if (distributedAt_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, getDistributedAt());
}
if (withdrawnAt_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, getWithdrawnAt());
}
if (createdAt_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(6, getCreatedAt());
}
if (updatedAt_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(7, getUpdatedAt());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.passkit.grpc.MessageOuterClass.GetMessageResponse)) {
return super.equals(obj);
}
com.passkit.grpc.MessageOuterClass.GetMessageResponse other = (com.passkit.grpc.MessageOuterClass.GetMessageResponse) obj;
if (hasMessage() != other.hasMessage()) return false;
if (hasMessage()) {
if (!getMessage()
.equals(other.getMessage())) return false;
}
if (status_ != other.status_) return false;
if (getRecipients()
!= other.getRecipients()) return false;
if (hasDistributedAt() != other.hasDistributedAt()) return false;
if (hasDistributedAt()) {
if (!getDistributedAt()
.equals(other.getDistributedAt())) return false;
}
if (hasWithdrawnAt() != other.hasWithdrawnAt()) return false;
if (hasWithdrawnAt()) {
if (!getWithdrawnAt()
.equals(other.getWithdrawnAt())) return false;
}
if (hasCreatedAt() != other.hasCreatedAt()) return false;
if (hasCreatedAt()) {
if (!getCreatedAt()
.equals(other.getCreatedAt())) return false;
}
if (hasUpdatedAt() != other.hasUpdatedAt()) return false;
if (hasUpdatedAt()) {
if (!getUpdatedAt()
.equals(other.getUpdatedAt())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasMessage()) {
hash = (37 * hash) + MESSAGE_FIELD_NUMBER;
hash = (53 * hash) + getMessage().hashCode();
}
hash = (37 * hash) + STATUS_FIELD_NUMBER;
hash = (53 * hash) + status_;
hash = (37 * hash) + RECIPIENTS_FIELD_NUMBER;
hash = (53 * hash) + getRecipients();
if (hasDistributedAt()) {
hash = (37 * hash) + DISTRIBUTEDAT_FIELD_NUMBER;
hash = (53 * hash) + getDistributedAt().hashCode();
}
if (hasWithdrawnAt()) {
hash = (37 * hash) + WITHDRAWNAT_FIELD_NUMBER;
hash = (53 * hash) + getWithdrawnAt().hashCode();
}
if (hasCreatedAt()) {
hash = (37 * hash) + CREATEDAT_FIELD_NUMBER;
hash = (53 * hash) + getCreatedAt().hashCode();
}
if (hasUpdatedAt()) {
hash = (37 * hash) + UPDATEDAT_FIELD_NUMBER;
hash = (53 * hash) + getUpdatedAt().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.passkit.grpc.MessageOuterClass.GetMessageResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.passkit.grpc.MessageOuterClass.GetMessageResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.passkit.grpc.MessageOuterClass.GetMessageResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.passkit.grpc.MessageOuterClass.GetMessageResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.passkit.grpc.MessageOuterClass.GetMessageResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.passkit.grpc.MessageOuterClass.GetMessageResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.passkit.grpc.MessageOuterClass.GetMessageResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.passkit.grpc.MessageOuterClass.GetMessageResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.passkit.grpc.MessageOuterClass.GetMessageResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.passkit.grpc.MessageOuterClass.GetMessageResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.passkit.grpc.MessageOuterClass.GetMessageResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.passkit.grpc.MessageOuterClass.GetMessageResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.passkit.grpc.MessageOuterClass.GetMessageResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code io.GetMessageResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:io.GetMessageResponse)
com.passkit.grpc.MessageOuterClass.GetMessageResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.passkit.grpc.MessageOuterClass.internal_static_io_GetMessageResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.passkit.grpc.MessageOuterClass.internal_static_io_GetMessageResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.passkit.grpc.MessageOuterClass.GetMessageResponse.class, com.passkit.grpc.MessageOuterClass.GetMessageResponse.Builder.class);
}
// Construct using com.passkit.grpc.MessageOuterClass.GetMessageResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (messageBuilder_ == null) {
message_ = null;
} else {
message_ = null;
messageBuilder_ = null;
}
status_ = 0;
recipients_ = 0;
if (distributedAtBuilder_ == null) {
distributedAt_ = null;
} else {
distributedAt_ = null;
distributedAtBuilder_ = null;
}
if (withdrawnAtBuilder_ == null) {
withdrawnAt_ = null;
} else {
withdrawnAt_ = null;
withdrawnAtBuilder_ = null;
}
if (createdAtBuilder_ == null) {
createdAt_ = null;
} else {
createdAt_ = null;
createdAtBuilder_ = null;
}
if (updatedAtBuilder_ == null) {
updatedAt_ = null;
} else {
updatedAt_ = null;
updatedAtBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.passkit.grpc.MessageOuterClass.internal_static_io_GetMessageResponse_descriptor;
}
@java.lang.Override
public com.passkit.grpc.MessageOuterClass.GetMessageResponse getDefaultInstanceForType() {
return com.passkit.grpc.MessageOuterClass.GetMessageResponse.getDefaultInstance();
}
@java.lang.Override
public com.passkit.grpc.MessageOuterClass.GetMessageResponse build() {
com.passkit.grpc.MessageOuterClass.GetMessageResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.passkit.grpc.MessageOuterClass.GetMessageResponse buildPartial() {
com.passkit.grpc.MessageOuterClass.GetMessageResponse result = new com.passkit.grpc.MessageOuterClass.GetMessageResponse(this);
if (messageBuilder_ == null) {
result.message_ = message_;
} else {
result.message_ = messageBuilder_.build();
}
result.status_ = status_;
result.recipients_ = recipients_;
if (distributedAtBuilder_ == null) {
result.distributedAt_ = distributedAt_;
} else {
result.distributedAt_ = distributedAtBuilder_.build();
}
if (withdrawnAtBuilder_ == null) {
result.withdrawnAt_ = withdrawnAt_;
} else {
result.withdrawnAt_ = withdrawnAtBuilder_.build();
}
if (createdAtBuilder_ == null) {
result.createdAt_ = createdAt_;
} else {
result.createdAt_ = createdAtBuilder_.build();
}
if (updatedAtBuilder_ == null) {
result.updatedAt_ = updatedAt_;
} else {
result.updatedAt_ = updatedAtBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.passkit.grpc.MessageOuterClass.GetMessageResponse) {
return mergeFrom((com.passkit.grpc.MessageOuterClass.GetMessageResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.passkit.grpc.MessageOuterClass.GetMessageResponse other) {
if (other == com.passkit.grpc.MessageOuterClass.GetMessageResponse.getDefaultInstance()) return this;
if (other.hasMessage()) {
mergeMessage(other.getMessage());
}
if (other.status_ != 0) {
setStatusValue(other.getStatusValue());
}
if (other.getRecipients() != 0) {
setRecipients(other.getRecipients());
}
if (other.hasDistributedAt()) {
mergeDistributedAt(other.getDistributedAt());
}
if (other.hasWithdrawnAt()) {
mergeWithdrawnAt(other.getWithdrawnAt());
}
if (other.hasCreatedAt()) {
mergeCreatedAt(other.getCreatedAt());
}
if (other.hasUpdatedAt()) {
mergeUpdatedAt(other.getUpdatedAt());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.passkit.grpc.MessageOuterClass.GetMessageResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.passkit.grpc.MessageOuterClass.GetMessageResponse) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private com.passkit.grpc.MessageOuterClass.Message message_;
private com.google.protobuf.SingleFieldBuilderV3<
com.passkit.grpc.MessageOuterClass.Message, com.passkit.grpc.MessageOuterClass.Message.Builder, com.passkit.grpc.MessageOuterClass.MessageOrBuilder> messageBuilder_;
/**
* .io.Message message = 1;
* @return Whether the message field is set.
*/
public boolean hasMessage() {
return messageBuilder_ != null || message_ != null;
}
/**
* .io.Message message = 1;
* @return The message.
*/
public com.passkit.grpc.MessageOuterClass.Message getMessage() {
if (messageBuilder_ == null) {
return message_ == null ? com.passkit.grpc.MessageOuterClass.Message.getDefaultInstance() : message_;
} else {
return messageBuilder_.getMessage();
}
}
/**
* .io.Message message = 1;
*/
public Builder setMessage(com.passkit.grpc.MessageOuterClass.Message value) {
if (messageBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
message_ = value;
onChanged();
} else {
messageBuilder_.setMessage(value);
}
return this;
}
/**
* .io.Message message = 1;
*/
public Builder setMessage(
com.passkit.grpc.MessageOuterClass.Message.Builder builderForValue) {
if (messageBuilder_ == null) {
message_ = builderForValue.build();
onChanged();
} else {
messageBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .io.Message message = 1;
*/
public Builder mergeMessage(com.passkit.grpc.MessageOuterClass.Message value) {
if (messageBuilder_ == null) {
if (message_ != null) {
message_ =
com.passkit.grpc.MessageOuterClass.Message.newBuilder(message_).mergeFrom(value).buildPartial();
} else {
message_ = value;
}
onChanged();
} else {
messageBuilder_.mergeFrom(value);
}
return this;
}
/**
* .io.Message message = 1;
*/
public Builder clearMessage() {
if (messageBuilder_ == null) {
message_ = null;
onChanged();
} else {
message_ = null;
messageBuilder_ = null;
}
return this;
}
/**
* .io.Message message = 1;
*/
public com.passkit.grpc.MessageOuterClass.Message.Builder getMessageBuilder() {
onChanged();
return getMessageFieldBuilder().getBuilder();
}
/**
* .io.Message message = 1;
*/
public com.passkit.grpc.MessageOuterClass.MessageOrBuilder getMessageOrBuilder() {
if (messageBuilder_ != null) {
return messageBuilder_.getMessageOrBuilder();
} else {
return message_ == null ?
com.passkit.grpc.MessageOuterClass.Message.getDefaultInstance() : message_;
}
}
/**
* .io.Message message = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.passkit.grpc.MessageOuterClass.Message, com.passkit.grpc.MessageOuterClass.Message.Builder, com.passkit.grpc.MessageOuterClass.MessageOrBuilder>
getMessageFieldBuilder() {
if (messageBuilder_ == null) {
messageBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.passkit.grpc.MessageOuterClass.Message, com.passkit.grpc.MessageOuterClass.Message.Builder, com.passkit.grpc.MessageOuterClass.MessageOrBuilder>(
getMessage(),
getParentForChildren(),
isClean());
message_ = null;
}
return messageBuilder_;
}
private int status_ = 0;
/**
* .io.MessageStatus status = 2;
* @return The enum numeric value on the wire for status.
*/
@java.lang.Override public int getStatusValue() {
return status_;
}
/**
* .io.MessageStatus status = 2;
* @param value The enum numeric value on the wire for status to set.
* @return This builder for chaining.
*/
public Builder setStatusValue(int value) {
status_ = value;
onChanged();
return this;
}
/**
* .io.MessageStatus status = 2;
* @return The status.
*/
@java.lang.Override
public com.passkit.grpc.MessageOuterClass.MessageStatus getStatus() {
@SuppressWarnings("deprecation")
com.passkit.grpc.MessageOuterClass.MessageStatus result = com.passkit.grpc.MessageOuterClass.MessageStatus.valueOf(status_);
return result == null ? com.passkit.grpc.MessageOuterClass.MessageStatus.UNRECOGNIZED : result;
}
/**
* .io.MessageStatus status = 2;
* @param value The status to set.
* @return This builder for chaining.
*/
public Builder setStatus(com.passkit.grpc.MessageOuterClass.MessageStatus value) {
if (value == null) {
throw new NullPointerException();
}
status_ = value.getNumber();
onChanged();
return this;
}
/**
* .io.MessageStatus status = 2;
* @return This builder for chaining.
*/
public Builder clearStatus() {
status_ = 0;
onChanged();
return this;
}
private int recipients_ ;
/**
* uint32 recipients = 3;
* @return The recipients.
*/
@java.lang.Override
public int getRecipients() {
return recipients_;
}
/**
* uint32 recipients = 3;
* @param value The recipients to set.
* @return This builder for chaining.
*/
public Builder setRecipients(int value) {
recipients_ = value;
onChanged();
return this;
}
/**
* uint32 recipients = 3;
* @return This builder for chaining.
*/
public Builder clearRecipients() {
recipients_ = 0;
onChanged();
return this;
}
private com.google.protobuf.Timestamp distributedAt_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder> distributedAtBuilder_;
/**
* .google.protobuf.Timestamp distributedAt = 4;
* @return Whether the distributedAt field is set.
*/
public boolean hasDistributedAt() {
return distributedAtBuilder_ != null || distributedAt_ != null;
}
/**
* .google.protobuf.Timestamp distributedAt = 4;
* @return The distributedAt.
*/
public com.google.protobuf.Timestamp getDistributedAt() {
if (distributedAtBuilder_ == null) {
return distributedAt_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : distributedAt_;
} else {
return distributedAtBuilder_.getMessage();
}
}
/**
* .google.protobuf.Timestamp distributedAt = 4;
*/
public Builder setDistributedAt(com.google.protobuf.Timestamp value) {
if (distributedAtBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
distributedAt_ = value;
onChanged();
} else {
distributedAtBuilder_.setMessage(value);
}
return this;
}
/**
* .google.protobuf.Timestamp distributedAt = 4;
*/
public Builder setDistributedAt(
com.google.protobuf.Timestamp.Builder builderForValue) {
if (distributedAtBuilder_ == null) {
distributedAt_ = builderForValue.build();
onChanged();
} else {
distributedAtBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .google.protobuf.Timestamp distributedAt = 4;
*/
public Builder mergeDistributedAt(com.google.protobuf.Timestamp value) {
if (distributedAtBuilder_ == null) {
if (distributedAt_ != null) {
distributedAt_ =
com.google.protobuf.Timestamp.newBuilder(distributedAt_).mergeFrom(value).buildPartial();
} else {
distributedAt_ = value;
}
onChanged();
} else {
distributedAtBuilder_.mergeFrom(value);
}
return this;
}
/**
* .google.protobuf.Timestamp distributedAt = 4;
*/
public Builder clearDistributedAt() {
if (distributedAtBuilder_ == null) {
distributedAt_ = null;
onChanged();
} else {
distributedAt_ = null;
distributedAtBuilder_ = null;
}
return this;
}
/**
* .google.protobuf.Timestamp distributedAt = 4;
*/
public com.google.protobuf.Timestamp.Builder getDistributedAtBuilder() {
onChanged();
return getDistributedAtFieldBuilder().getBuilder();
}
/**
* .google.protobuf.Timestamp distributedAt = 4;
*/
public com.google.protobuf.TimestampOrBuilder getDistributedAtOrBuilder() {
if (distributedAtBuilder_ != null) {
return distributedAtBuilder_.getMessageOrBuilder();
} else {
return distributedAt_ == null ?
com.google.protobuf.Timestamp.getDefaultInstance() : distributedAt_;
}
}
/**
* .google.protobuf.Timestamp distributedAt = 4;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder>
getDistributedAtFieldBuilder() {
if (distributedAtBuilder_ == null) {
distributedAtBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder>(
getDistributedAt(),
getParentForChildren(),
isClean());
distributedAt_ = null;
}
return distributedAtBuilder_;
}
private com.google.protobuf.Timestamp withdrawnAt_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder> withdrawnAtBuilder_;
/**
* .google.protobuf.Timestamp withdrawnAt = 5;
* @return Whether the withdrawnAt field is set.
*/
public boolean hasWithdrawnAt() {
return withdrawnAtBuilder_ != null || withdrawnAt_ != null;
}
/**
* .google.protobuf.Timestamp withdrawnAt = 5;
* @return The withdrawnAt.
*/
public com.google.protobuf.Timestamp getWithdrawnAt() {
if (withdrawnAtBuilder_ == null) {
return withdrawnAt_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : withdrawnAt_;
} else {
return withdrawnAtBuilder_.getMessage();
}
}
/**
* .google.protobuf.Timestamp withdrawnAt = 5;
*/
public Builder setWithdrawnAt(com.google.protobuf.Timestamp value) {
if (withdrawnAtBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
withdrawnAt_ = value;
onChanged();
} else {
withdrawnAtBuilder_.setMessage(value);
}
return this;
}
/**
* .google.protobuf.Timestamp withdrawnAt = 5;
*/
public Builder setWithdrawnAt(
com.google.protobuf.Timestamp.Builder builderForValue) {
if (withdrawnAtBuilder_ == null) {
withdrawnAt_ = builderForValue.build();
onChanged();
} else {
withdrawnAtBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .google.protobuf.Timestamp withdrawnAt = 5;
*/
public Builder mergeWithdrawnAt(com.google.protobuf.Timestamp value) {
if (withdrawnAtBuilder_ == null) {
if (withdrawnAt_ != null) {
withdrawnAt_ =
com.google.protobuf.Timestamp.newBuilder(withdrawnAt_).mergeFrom(value).buildPartial();
} else {
withdrawnAt_ = value;
}
onChanged();
} else {
withdrawnAtBuilder_.mergeFrom(value);
}
return this;
}
/**
* .google.protobuf.Timestamp withdrawnAt = 5;
*/
public Builder clearWithdrawnAt() {
if (withdrawnAtBuilder_ == null) {
withdrawnAt_ = null;
onChanged();
} else {
withdrawnAt_ = null;
withdrawnAtBuilder_ = null;
}
return this;
}
/**
* .google.protobuf.Timestamp withdrawnAt = 5;
*/
public com.google.protobuf.Timestamp.Builder getWithdrawnAtBuilder() {
onChanged();
return getWithdrawnAtFieldBuilder().getBuilder();
}
/**
* .google.protobuf.Timestamp withdrawnAt = 5;
*/
public com.google.protobuf.TimestampOrBuilder getWithdrawnAtOrBuilder() {
if (withdrawnAtBuilder_ != null) {
return withdrawnAtBuilder_.getMessageOrBuilder();
} else {
return withdrawnAt_ == null ?
com.google.protobuf.Timestamp.getDefaultInstance() : withdrawnAt_;
}
}
/**
* .google.protobuf.Timestamp withdrawnAt = 5;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder>
getWithdrawnAtFieldBuilder() {
if (withdrawnAtBuilder_ == null) {
withdrawnAtBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder>(
getWithdrawnAt(),
getParentForChildren(),
isClean());
withdrawnAt_ = null;
}
return withdrawnAtBuilder_;
}
private com.google.protobuf.Timestamp createdAt_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder> createdAtBuilder_;
/**
* .google.protobuf.Timestamp createdAt = 6;
* @return Whether the createdAt field is set.
*/
public boolean hasCreatedAt() {
return createdAtBuilder_ != null || createdAt_ != null;
}
/**
* .google.protobuf.Timestamp createdAt = 6;
* @return The createdAt.
*/
public com.google.protobuf.Timestamp getCreatedAt() {
if (createdAtBuilder_ == null) {
return createdAt_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : createdAt_;
} else {
return createdAtBuilder_.getMessage();
}
}
/**
* .google.protobuf.Timestamp createdAt = 6;
*/
public Builder setCreatedAt(com.google.protobuf.Timestamp value) {
if (createdAtBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
createdAt_ = value;
onChanged();
} else {
createdAtBuilder_.setMessage(value);
}
return this;
}
/**
* .google.protobuf.Timestamp createdAt = 6;
*/
public Builder setCreatedAt(
com.google.protobuf.Timestamp.Builder builderForValue) {
if (createdAtBuilder_ == null) {
createdAt_ = builderForValue.build();
onChanged();
} else {
createdAtBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .google.protobuf.Timestamp createdAt = 6;
*/
public Builder mergeCreatedAt(com.google.protobuf.Timestamp value) {
if (createdAtBuilder_ == null) {
if (createdAt_ != null) {
createdAt_ =
com.google.protobuf.Timestamp.newBuilder(createdAt_).mergeFrom(value).buildPartial();
} else {
createdAt_ = value;
}
onChanged();
} else {
createdAtBuilder_.mergeFrom(value);
}
return this;
}
/**
* .google.protobuf.Timestamp createdAt = 6;
*/
public Builder clearCreatedAt() {
if (createdAtBuilder_ == null) {
createdAt_ = null;
onChanged();
} else {
createdAt_ = null;
createdAtBuilder_ = null;
}
return this;
}
/**
* .google.protobuf.Timestamp createdAt = 6;
*/
public com.google.protobuf.Timestamp.Builder getCreatedAtBuilder() {
onChanged();
return getCreatedAtFieldBuilder().getBuilder();
}
/**
* .google.protobuf.Timestamp createdAt = 6;
*/
public com.google.protobuf.TimestampOrBuilder getCreatedAtOrBuilder() {
if (createdAtBuilder_ != null) {
return createdAtBuilder_.getMessageOrBuilder();
} else {
return createdAt_ == null ?
com.google.protobuf.Timestamp.getDefaultInstance() : createdAt_;
}
}
/**
* .google.protobuf.Timestamp createdAt = 6;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder>
getCreatedAtFieldBuilder() {
if (createdAtBuilder_ == null) {
createdAtBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder>(
getCreatedAt(),
getParentForChildren(),
isClean());
createdAt_ = null;
}
return createdAtBuilder_;
}
private com.google.protobuf.Timestamp updatedAt_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder> updatedAtBuilder_;
/**
* .google.protobuf.Timestamp updatedAt = 7;
* @return Whether the updatedAt field is set.
*/
public boolean hasUpdatedAt() {
return updatedAtBuilder_ != null || updatedAt_ != null;
}
/**
* .google.protobuf.Timestamp updatedAt = 7;
* @return The updatedAt.
*/
public com.google.protobuf.Timestamp getUpdatedAt() {
if (updatedAtBuilder_ == null) {
return updatedAt_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : updatedAt_;
} else {
return updatedAtBuilder_.getMessage();
}
}
/**
* .google.protobuf.Timestamp updatedAt = 7;
*/
public Builder setUpdatedAt(com.google.protobuf.Timestamp value) {
if (updatedAtBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
updatedAt_ = value;
onChanged();
} else {
updatedAtBuilder_.setMessage(value);
}
return this;
}
/**
* .google.protobuf.Timestamp updatedAt = 7;
*/
public Builder setUpdatedAt(
com.google.protobuf.Timestamp.Builder builderForValue) {
if (updatedAtBuilder_ == null) {
updatedAt_ = builderForValue.build();
onChanged();
} else {
updatedAtBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .google.protobuf.Timestamp updatedAt = 7;
*/
public Builder mergeUpdatedAt(com.google.protobuf.Timestamp value) {
if (updatedAtBuilder_ == null) {
if (updatedAt_ != null) {
updatedAt_ =
com.google.protobuf.Timestamp.newBuilder(updatedAt_).mergeFrom(value).buildPartial();
} else {
updatedAt_ = value;
}
onChanged();
} else {
updatedAtBuilder_.mergeFrom(value);
}
return this;
}
/**
* .google.protobuf.Timestamp updatedAt = 7;
*/
public Builder clearUpdatedAt() {
if (updatedAtBuilder_ == null) {
updatedAt_ = null;
onChanged();
} else {
updatedAt_ = null;
updatedAtBuilder_ = null;
}
return this;
}
/**
* .google.protobuf.Timestamp updatedAt = 7;
*/
public com.google.protobuf.Timestamp.Builder getUpdatedAtBuilder() {
onChanged();
return getUpdatedAtFieldBuilder().getBuilder();
}
/**
* .google.protobuf.Timestamp updatedAt = 7;
*/
public com.google.protobuf.TimestampOrBuilder getUpdatedAtOrBuilder() {
if (updatedAtBuilder_ != null) {
return updatedAtBuilder_.getMessageOrBuilder();
} else {
return updatedAt_ == null ?
com.google.protobuf.Timestamp.getDefaultInstance() : updatedAt_;
}
}
/**
* .google.protobuf.Timestamp updatedAt = 7;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder>
getUpdatedAtFieldBuilder() {
if (updatedAtBuilder_ == null) {
updatedAtBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder>(
getUpdatedAt(),
getParentForChildren(),
isClean());
updatedAt_ = null;
}
return updatedAtBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:io.GetMessageResponse)
}
// @@protoc_insertion_point(class_scope:io.GetMessageResponse)
private static final com.passkit.grpc.MessageOuterClass.GetMessageResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.passkit.grpc.MessageOuterClass.GetMessageResponse();
}
public static com.passkit.grpc.MessageOuterClass.GetMessageResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public GetMessageResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new GetMessageResponse(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.passkit.grpc.MessageOuterClass.GetMessageResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface GetMessageHistoryResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:io.GetMessageHistoryResponse)
com.google.protobuf.MessageOrBuilder {
/**
* repeated .io.GetMessageResponse messages = 1;
*/
java.util.List
getMessagesList();
/**
* repeated .io.GetMessageResponse messages = 1;
*/
com.passkit.grpc.MessageOuterClass.GetMessageResponse getMessages(int index);
/**
* repeated .io.GetMessageResponse messages = 1;
*/
int getMessagesCount();
/**
* repeated .io.GetMessageResponse messages = 1;
*/
java.util.List extends com.passkit.grpc.MessageOuterClass.GetMessageResponseOrBuilder>
getMessagesOrBuilderList();
/**
* repeated .io.GetMessageResponse messages = 1;
*/
com.passkit.grpc.MessageOuterClass.GetMessageResponseOrBuilder getMessagesOrBuilder(
int index);
}
/**
* Protobuf type {@code io.GetMessageHistoryResponse}
*/
public static final class GetMessageHistoryResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:io.GetMessageHistoryResponse)
GetMessageHistoryResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use GetMessageHistoryResponse.newBuilder() to construct.
private GetMessageHistoryResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private GetMessageHistoryResponse() {
messages_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new GetMessageHistoryResponse();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private GetMessageHistoryResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
messages_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
messages_.add(
input.readMessage(com.passkit.grpc.MessageOuterClass.GetMessageResponse.parser(), extensionRegistry));
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
messages_ = java.util.Collections.unmodifiableList(messages_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.passkit.grpc.MessageOuterClass.internal_static_io_GetMessageHistoryResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.passkit.grpc.MessageOuterClass.internal_static_io_GetMessageHistoryResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.passkit.grpc.MessageOuterClass.GetMessageHistoryResponse.class, com.passkit.grpc.MessageOuterClass.GetMessageHistoryResponse.Builder.class);
}
public static final int MESSAGES_FIELD_NUMBER = 1;
private java.util.List messages_;
/**
* repeated .io.GetMessageResponse messages = 1;
*/
@java.lang.Override
public java.util.List getMessagesList() {
return messages_;
}
/**
* repeated .io.GetMessageResponse messages = 1;
*/
@java.lang.Override
public java.util.List extends com.passkit.grpc.MessageOuterClass.GetMessageResponseOrBuilder>
getMessagesOrBuilderList() {
return messages_;
}
/**
* repeated .io.GetMessageResponse messages = 1;
*/
@java.lang.Override
public int getMessagesCount() {
return messages_.size();
}
/**
* repeated .io.GetMessageResponse messages = 1;
*/
@java.lang.Override
public com.passkit.grpc.MessageOuterClass.GetMessageResponse getMessages(int index) {
return messages_.get(index);
}
/**
* repeated .io.GetMessageResponse messages = 1;
*/
@java.lang.Override
public com.passkit.grpc.MessageOuterClass.GetMessageResponseOrBuilder getMessagesOrBuilder(
int index) {
return messages_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < messages_.size(); i++) {
output.writeMessage(1, messages_.get(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < messages_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, messages_.get(i));
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.passkit.grpc.MessageOuterClass.GetMessageHistoryResponse)) {
return super.equals(obj);
}
com.passkit.grpc.MessageOuterClass.GetMessageHistoryResponse other = (com.passkit.grpc.MessageOuterClass.GetMessageHistoryResponse) obj;
if (!getMessagesList()
.equals(other.getMessagesList())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getMessagesCount() > 0) {
hash = (37 * hash) + MESSAGES_FIELD_NUMBER;
hash = (53 * hash) + getMessagesList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.passkit.grpc.MessageOuterClass.GetMessageHistoryResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.passkit.grpc.MessageOuterClass.GetMessageHistoryResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.passkit.grpc.MessageOuterClass.GetMessageHistoryResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.passkit.grpc.MessageOuterClass.GetMessageHistoryResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.passkit.grpc.MessageOuterClass.GetMessageHistoryResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.passkit.grpc.MessageOuterClass.GetMessageHistoryResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.passkit.grpc.MessageOuterClass.GetMessageHistoryResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.passkit.grpc.MessageOuterClass.GetMessageHistoryResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.passkit.grpc.MessageOuterClass.GetMessageHistoryResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.passkit.grpc.MessageOuterClass.GetMessageHistoryResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.passkit.grpc.MessageOuterClass.GetMessageHistoryResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.passkit.grpc.MessageOuterClass.GetMessageHistoryResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.passkit.grpc.MessageOuterClass.GetMessageHistoryResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code io.GetMessageHistoryResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:io.GetMessageHistoryResponse)
com.passkit.grpc.MessageOuterClass.GetMessageHistoryResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.passkit.grpc.MessageOuterClass.internal_static_io_GetMessageHistoryResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.passkit.grpc.MessageOuterClass.internal_static_io_GetMessageHistoryResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.passkit.grpc.MessageOuterClass.GetMessageHistoryResponse.class, com.passkit.grpc.MessageOuterClass.GetMessageHistoryResponse.Builder.class);
}
// Construct using com.passkit.grpc.MessageOuterClass.GetMessageHistoryResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getMessagesFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (messagesBuilder_ == null) {
messages_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
messagesBuilder_.clear();
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.passkit.grpc.MessageOuterClass.internal_static_io_GetMessageHistoryResponse_descriptor;
}
@java.lang.Override
public com.passkit.grpc.MessageOuterClass.GetMessageHistoryResponse getDefaultInstanceForType() {
return com.passkit.grpc.MessageOuterClass.GetMessageHistoryResponse.getDefaultInstance();
}
@java.lang.Override
public com.passkit.grpc.MessageOuterClass.GetMessageHistoryResponse build() {
com.passkit.grpc.MessageOuterClass.GetMessageHistoryResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.passkit.grpc.MessageOuterClass.GetMessageHistoryResponse buildPartial() {
com.passkit.grpc.MessageOuterClass.GetMessageHistoryResponse result = new com.passkit.grpc.MessageOuterClass.GetMessageHistoryResponse(this);
int from_bitField0_ = bitField0_;
if (messagesBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0)) {
messages_ = java.util.Collections.unmodifiableList(messages_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.messages_ = messages_;
} else {
result.messages_ = messagesBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.passkit.grpc.MessageOuterClass.GetMessageHistoryResponse) {
return mergeFrom((com.passkit.grpc.MessageOuterClass.GetMessageHistoryResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.passkit.grpc.MessageOuterClass.GetMessageHistoryResponse other) {
if (other == com.passkit.grpc.MessageOuterClass.GetMessageHistoryResponse.getDefaultInstance()) return this;
if (messagesBuilder_ == null) {
if (!other.messages_.isEmpty()) {
if (messages_.isEmpty()) {
messages_ = other.messages_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureMessagesIsMutable();
messages_.addAll(other.messages_);
}
onChanged();
}
} else {
if (!other.messages_.isEmpty()) {
if (messagesBuilder_.isEmpty()) {
messagesBuilder_.dispose();
messagesBuilder_ = null;
messages_ = other.messages_;
bitField0_ = (bitField0_ & ~0x00000001);
messagesBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getMessagesFieldBuilder() : null;
} else {
messagesBuilder_.addAllMessages(other.messages_);
}
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.passkit.grpc.MessageOuterClass.GetMessageHistoryResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.passkit.grpc.MessageOuterClass.GetMessageHistoryResponse) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List messages_ =
java.util.Collections.emptyList();
private void ensureMessagesIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
messages_ = new java.util.ArrayList(messages_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.passkit.grpc.MessageOuterClass.GetMessageResponse, com.passkit.grpc.MessageOuterClass.GetMessageResponse.Builder, com.passkit.grpc.MessageOuterClass.GetMessageResponseOrBuilder> messagesBuilder_;
/**
* repeated .io.GetMessageResponse messages = 1;
*/
public java.util.List getMessagesList() {
if (messagesBuilder_ == null) {
return java.util.Collections.unmodifiableList(messages_);
} else {
return messagesBuilder_.getMessageList();
}
}
/**
* repeated .io.GetMessageResponse messages = 1;
*/
public int getMessagesCount() {
if (messagesBuilder_ == null) {
return messages_.size();
} else {
return messagesBuilder_.getCount();
}
}
/**
* repeated .io.GetMessageResponse messages = 1;
*/
public com.passkit.grpc.MessageOuterClass.GetMessageResponse getMessages(int index) {
if (messagesBuilder_ == null) {
return messages_.get(index);
} else {
return messagesBuilder_.getMessage(index);
}
}
/**
* repeated .io.GetMessageResponse messages = 1;
*/
public Builder setMessages(
int index, com.passkit.grpc.MessageOuterClass.GetMessageResponse value) {
if (messagesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureMessagesIsMutable();
messages_.set(index, value);
onChanged();
} else {
messagesBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .io.GetMessageResponse messages = 1;
*/
public Builder setMessages(
int index, com.passkit.grpc.MessageOuterClass.GetMessageResponse.Builder builderForValue) {
if (messagesBuilder_ == null) {
ensureMessagesIsMutable();
messages_.set(index, builderForValue.build());
onChanged();
} else {
messagesBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .io.GetMessageResponse messages = 1;
*/
public Builder addMessages(com.passkit.grpc.MessageOuterClass.GetMessageResponse value) {
if (messagesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureMessagesIsMutable();
messages_.add(value);
onChanged();
} else {
messagesBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .io.GetMessageResponse messages = 1;
*/
public Builder addMessages(
int index, com.passkit.grpc.MessageOuterClass.GetMessageResponse value) {
if (messagesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureMessagesIsMutable();
messages_.add(index, value);
onChanged();
} else {
messagesBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .io.GetMessageResponse messages = 1;
*/
public Builder addMessages(
com.passkit.grpc.MessageOuterClass.GetMessageResponse.Builder builderForValue) {
if (messagesBuilder_ == null) {
ensureMessagesIsMutable();
messages_.add(builderForValue.build());
onChanged();
} else {
messagesBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .io.GetMessageResponse messages = 1;
*/
public Builder addMessages(
int index, com.passkit.grpc.MessageOuterClass.GetMessageResponse.Builder builderForValue) {
if (messagesBuilder_ == null) {
ensureMessagesIsMutable();
messages_.add(index, builderForValue.build());
onChanged();
} else {
messagesBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .io.GetMessageResponse messages = 1;
*/
public Builder addAllMessages(
java.lang.Iterable extends com.passkit.grpc.MessageOuterClass.GetMessageResponse> values) {
if (messagesBuilder_ == null) {
ensureMessagesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, messages_);
onChanged();
} else {
messagesBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .io.GetMessageResponse messages = 1;
*/
public Builder clearMessages() {
if (messagesBuilder_ == null) {
messages_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
messagesBuilder_.clear();
}
return this;
}
/**
* repeated .io.GetMessageResponse messages = 1;
*/
public Builder removeMessages(int index) {
if (messagesBuilder_ == null) {
ensureMessagesIsMutable();
messages_.remove(index);
onChanged();
} else {
messagesBuilder_.remove(index);
}
return this;
}
/**
* repeated .io.GetMessageResponse messages = 1;
*/
public com.passkit.grpc.MessageOuterClass.GetMessageResponse.Builder getMessagesBuilder(
int index) {
return getMessagesFieldBuilder().getBuilder(index);
}
/**
* repeated .io.GetMessageResponse messages = 1;
*/
public com.passkit.grpc.MessageOuterClass.GetMessageResponseOrBuilder getMessagesOrBuilder(
int index) {
if (messagesBuilder_ == null) {
return messages_.get(index); } else {
return messagesBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .io.GetMessageResponse messages = 1;
*/
public java.util.List extends com.passkit.grpc.MessageOuterClass.GetMessageResponseOrBuilder>
getMessagesOrBuilderList() {
if (messagesBuilder_ != null) {
return messagesBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(messages_);
}
}
/**
* repeated .io.GetMessageResponse messages = 1;
*/
public com.passkit.grpc.MessageOuterClass.GetMessageResponse.Builder addMessagesBuilder() {
return getMessagesFieldBuilder().addBuilder(
com.passkit.grpc.MessageOuterClass.GetMessageResponse.getDefaultInstance());
}
/**
* repeated .io.GetMessageResponse messages = 1;
*/
public com.passkit.grpc.MessageOuterClass.GetMessageResponse.Builder addMessagesBuilder(
int index) {
return getMessagesFieldBuilder().addBuilder(
index, com.passkit.grpc.MessageOuterClass.GetMessageResponse.getDefaultInstance());
}
/**
* repeated .io.GetMessageResponse messages = 1;
*/
public java.util.List
getMessagesBuilderList() {
return getMessagesFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.passkit.grpc.MessageOuterClass.GetMessageResponse, com.passkit.grpc.MessageOuterClass.GetMessageResponse.Builder, com.passkit.grpc.MessageOuterClass.GetMessageResponseOrBuilder>
getMessagesFieldBuilder() {
if (messagesBuilder_ == null) {
messagesBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.passkit.grpc.MessageOuterClass.GetMessageResponse, com.passkit.grpc.MessageOuterClass.GetMessageResponse.Builder, com.passkit.grpc.MessageOuterClass.GetMessageResponseOrBuilder>(
messages_,
((bitField0_ & 0x00000001) != 0),
getParentForChildren(),
isClean());
messages_ = null;
}
return messagesBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:io.GetMessageHistoryResponse)
}
// @@protoc_insertion_point(class_scope:io.GetMessageHistoryResponse)
private static final com.passkit.grpc.MessageOuterClass.GetMessageHistoryResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.passkit.grpc.MessageOuterClass.GetMessageHistoryResponse();
}
public static com.passkit.grpc.MessageOuterClass.GetMessageHistoryResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public GetMessageHistoryResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new GetMessageHistoryResponse(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.passkit.grpc.MessageOuterClass.GetMessageHistoryResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface SendMessageRequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:io.SendMessageRequest)
com.google.protobuf.MessageOrBuilder {
/**
* string messageId = 1;
* @return The messageId.
*/
java.lang.String getMessageId();
/**
* string messageId = 1;
* @return The bytes for messageId.
*/
com.google.protobuf.ByteString
getMessageIdBytes();
/**
* .io.PassIds passIds = 2;
* @return Whether the passIds field is set.
*/
boolean hasPassIds();
/**
* .io.PassIds passIds = 2;
* @return The passIds.
*/
com.passkit.grpc.CommonObjects.PassIds getPassIds();
/**
* .io.PassIds passIds = 2;
*/
com.passkit.grpc.CommonObjects.PassIdsOrBuilder getPassIdsOrBuilder();
/**
* .io.Protocol protocol = 3;
* @return Whether the protocol field is set.
*/
boolean hasProtocol();
/**
* .io.Protocol protocol = 3;
* @return The protocol.
*/
com.passkit.grpc.CommonObjects.Protocol getProtocol();
/**
* .io.Protocol protocol = 3;
*/
com.passkit.grpc.CommonObjects.ProtocolOrBuilder getProtocolOrBuilder();
public com.passkit.grpc.MessageOuterClass.SendMessageRequest.TargetCase getTargetCase();
}
/**
* Protobuf type {@code io.SendMessageRequest}
*/
public static final class SendMessageRequest extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:io.SendMessageRequest)
SendMessageRequestOrBuilder {
private static final long serialVersionUID = 0L;
// Use SendMessageRequest.newBuilder() to construct.
private SendMessageRequest(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private SendMessageRequest() {
messageId_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new SendMessageRequest();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private SendMessageRequest(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
messageId_ = s;
break;
}
case 18: {
com.passkit.grpc.CommonObjects.PassIds.Builder subBuilder = null;
if (targetCase_ == 2) {
subBuilder = ((com.passkit.grpc.CommonObjects.PassIds) target_).toBuilder();
}
target_ =
input.readMessage(com.passkit.grpc.CommonObjects.PassIds.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((com.passkit.grpc.CommonObjects.PassIds) target_);
target_ = subBuilder.buildPartial();
}
targetCase_ = 2;
break;
}
case 26: {
com.passkit.grpc.CommonObjects.Protocol.Builder subBuilder = null;
if (targetCase_ == 3) {
subBuilder = ((com.passkit.grpc.CommonObjects.Protocol) target_).toBuilder();
}
target_ =
input.readMessage(com.passkit.grpc.CommonObjects.Protocol.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((com.passkit.grpc.CommonObjects.Protocol) target_);
target_ = subBuilder.buildPartial();
}
targetCase_ = 3;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.passkit.grpc.MessageOuterClass.internal_static_io_SendMessageRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.passkit.grpc.MessageOuterClass.internal_static_io_SendMessageRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.passkit.grpc.MessageOuterClass.SendMessageRequest.class, com.passkit.grpc.MessageOuterClass.SendMessageRequest.Builder.class);
}
private int targetCase_ = 0;
private java.lang.Object target_;
public enum TargetCase
implements com.google.protobuf.Internal.EnumLite,
com.google.protobuf.AbstractMessage.InternalOneOfEnum {
PASSIDS(2),
PROTOCOL(3),
TARGET_NOT_SET(0);
private final int value;
private TargetCase(int value) {
this.value = value;
}
/**
* @param value The number of the enum to look for.
* @return The enum associated with the given number.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static TargetCase valueOf(int value) {
return forNumber(value);
}
public static TargetCase forNumber(int value) {
switch (value) {
case 2: return PASSIDS;
case 3: return PROTOCOL;
case 0: return TARGET_NOT_SET;
default: return null;
}
}
public int getNumber() {
return this.value;
}
};
public TargetCase
getTargetCase() {
return TargetCase.forNumber(
targetCase_);
}
public static final int MESSAGEID_FIELD_NUMBER = 1;
private volatile java.lang.Object messageId_;
/**
* string messageId = 1;
* @return The messageId.
*/
@java.lang.Override
public java.lang.String getMessageId() {
java.lang.Object ref = messageId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
messageId_ = s;
return s;
}
}
/**
* string messageId = 1;
* @return The bytes for messageId.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getMessageIdBytes() {
java.lang.Object ref = messageId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
messageId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int PASSIDS_FIELD_NUMBER = 2;
/**
* .io.PassIds passIds = 2;
* @return Whether the passIds field is set.
*/
@java.lang.Override
public boolean hasPassIds() {
return targetCase_ == 2;
}
/**
* .io.PassIds passIds = 2;
* @return The passIds.
*/
@java.lang.Override
public com.passkit.grpc.CommonObjects.PassIds getPassIds() {
if (targetCase_ == 2) {
return (com.passkit.grpc.CommonObjects.PassIds) target_;
}
return com.passkit.grpc.CommonObjects.PassIds.getDefaultInstance();
}
/**
* .io.PassIds passIds = 2;
*/
@java.lang.Override
public com.passkit.grpc.CommonObjects.PassIdsOrBuilder getPassIdsOrBuilder() {
if (targetCase_ == 2) {
return (com.passkit.grpc.CommonObjects.PassIds) target_;
}
return com.passkit.grpc.CommonObjects.PassIds.getDefaultInstance();
}
public static final int PROTOCOL_FIELD_NUMBER = 3;
/**
* .io.Protocol protocol = 3;
* @return Whether the protocol field is set.
*/
@java.lang.Override
public boolean hasProtocol() {
return targetCase_ == 3;
}
/**
* .io.Protocol protocol = 3;
* @return The protocol.
*/
@java.lang.Override
public com.passkit.grpc.CommonObjects.Protocol getProtocol() {
if (targetCase_ == 3) {
return (com.passkit.grpc.CommonObjects.Protocol) target_;
}
return com.passkit.grpc.CommonObjects.Protocol.getDefaultInstance();
}
/**
* .io.Protocol protocol = 3;
*/
@java.lang.Override
public com.passkit.grpc.CommonObjects.ProtocolOrBuilder getProtocolOrBuilder() {
if (targetCase_ == 3) {
return (com.passkit.grpc.CommonObjects.Protocol) target_;
}
return com.passkit.grpc.CommonObjects.Protocol.getDefaultInstance();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(messageId_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, messageId_);
}
if (targetCase_ == 2) {
output.writeMessage(2, (com.passkit.grpc.CommonObjects.PassIds) target_);
}
if (targetCase_ == 3) {
output.writeMessage(3, (com.passkit.grpc.CommonObjects.Protocol) target_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(messageId_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, messageId_);
}
if (targetCase_ == 2) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, (com.passkit.grpc.CommonObjects.PassIds) target_);
}
if (targetCase_ == 3) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, (com.passkit.grpc.CommonObjects.Protocol) target_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.passkit.grpc.MessageOuterClass.SendMessageRequest)) {
return super.equals(obj);
}
com.passkit.grpc.MessageOuterClass.SendMessageRequest other = (com.passkit.grpc.MessageOuterClass.SendMessageRequest) obj;
if (!getMessageId()
.equals(other.getMessageId())) return false;
if (!getTargetCase().equals(other.getTargetCase())) return false;
switch (targetCase_) {
case 2:
if (!getPassIds()
.equals(other.getPassIds())) return false;
break;
case 3:
if (!getProtocol()
.equals(other.getProtocol())) return false;
break;
case 0:
default:
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + MESSAGEID_FIELD_NUMBER;
hash = (53 * hash) + getMessageId().hashCode();
switch (targetCase_) {
case 2:
hash = (37 * hash) + PASSIDS_FIELD_NUMBER;
hash = (53 * hash) + getPassIds().hashCode();
break;
case 3:
hash = (37 * hash) + PROTOCOL_FIELD_NUMBER;
hash = (53 * hash) + getProtocol().hashCode();
break;
case 0:
default:
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.passkit.grpc.MessageOuterClass.SendMessageRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.passkit.grpc.MessageOuterClass.SendMessageRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.passkit.grpc.MessageOuterClass.SendMessageRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.passkit.grpc.MessageOuterClass.SendMessageRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.passkit.grpc.MessageOuterClass.SendMessageRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.passkit.grpc.MessageOuterClass.SendMessageRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.passkit.grpc.MessageOuterClass.SendMessageRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.passkit.grpc.MessageOuterClass.SendMessageRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.passkit.grpc.MessageOuterClass.SendMessageRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.passkit.grpc.MessageOuterClass.SendMessageRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.passkit.grpc.MessageOuterClass.SendMessageRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.passkit.grpc.MessageOuterClass.SendMessageRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.passkit.grpc.MessageOuterClass.SendMessageRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code io.SendMessageRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:io.SendMessageRequest)
com.passkit.grpc.MessageOuterClass.SendMessageRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.passkit.grpc.MessageOuterClass.internal_static_io_SendMessageRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.passkit.grpc.MessageOuterClass.internal_static_io_SendMessageRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.passkit.grpc.MessageOuterClass.SendMessageRequest.class, com.passkit.grpc.MessageOuterClass.SendMessageRequest.Builder.class);
}
// Construct using com.passkit.grpc.MessageOuterClass.SendMessageRequest.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
messageId_ = "";
targetCase_ = 0;
target_ = null;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.passkit.grpc.MessageOuterClass.internal_static_io_SendMessageRequest_descriptor;
}
@java.lang.Override
public com.passkit.grpc.MessageOuterClass.SendMessageRequest getDefaultInstanceForType() {
return com.passkit.grpc.MessageOuterClass.SendMessageRequest.getDefaultInstance();
}
@java.lang.Override
public com.passkit.grpc.MessageOuterClass.SendMessageRequest build() {
com.passkit.grpc.MessageOuterClass.SendMessageRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.passkit.grpc.MessageOuterClass.SendMessageRequest buildPartial() {
com.passkit.grpc.MessageOuterClass.SendMessageRequest result = new com.passkit.grpc.MessageOuterClass.SendMessageRequest(this);
result.messageId_ = messageId_;
if (targetCase_ == 2) {
if (passIdsBuilder_ == null) {
result.target_ = target_;
} else {
result.target_ = passIdsBuilder_.build();
}
}
if (targetCase_ == 3) {
if (protocolBuilder_ == null) {
result.target_ = target_;
} else {
result.target_ = protocolBuilder_.build();
}
}
result.targetCase_ = targetCase_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.passkit.grpc.MessageOuterClass.SendMessageRequest) {
return mergeFrom((com.passkit.grpc.MessageOuterClass.SendMessageRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.passkit.grpc.MessageOuterClass.SendMessageRequest other) {
if (other == com.passkit.grpc.MessageOuterClass.SendMessageRequest.getDefaultInstance()) return this;
if (!other.getMessageId().isEmpty()) {
messageId_ = other.messageId_;
onChanged();
}
switch (other.getTargetCase()) {
case PASSIDS: {
mergePassIds(other.getPassIds());
break;
}
case PROTOCOL: {
mergeProtocol(other.getProtocol());
break;
}
case TARGET_NOT_SET: {
break;
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.passkit.grpc.MessageOuterClass.SendMessageRequest parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.passkit.grpc.MessageOuterClass.SendMessageRequest) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int targetCase_ = 0;
private java.lang.Object target_;
public TargetCase
getTargetCase() {
return TargetCase.forNumber(
targetCase_);
}
public Builder clearTarget() {
targetCase_ = 0;
target_ = null;
onChanged();
return this;
}
private java.lang.Object messageId_ = "";
/**
* string messageId = 1;
* @return The messageId.
*/
public java.lang.String getMessageId() {
java.lang.Object ref = messageId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
messageId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string messageId = 1;
* @return The bytes for messageId.
*/
public com.google.protobuf.ByteString
getMessageIdBytes() {
java.lang.Object ref = messageId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
messageId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string messageId = 1;
* @param value The messageId to set.
* @return This builder for chaining.
*/
public Builder setMessageId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
messageId_ = value;
onChanged();
return this;
}
/**
* string messageId = 1;
* @return This builder for chaining.
*/
public Builder clearMessageId() {
messageId_ = getDefaultInstance().getMessageId();
onChanged();
return this;
}
/**
* string messageId = 1;
* @param value The bytes for messageId to set.
* @return This builder for chaining.
*/
public Builder setMessageIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
messageId_ = value;
onChanged();
return this;
}
private com.google.protobuf.SingleFieldBuilderV3<
com.passkit.grpc.CommonObjects.PassIds, com.passkit.grpc.CommonObjects.PassIds.Builder, com.passkit.grpc.CommonObjects.PassIdsOrBuilder> passIdsBuilder_;
/**
* .io.PassIds passIds = 2;
* @return Whether the passIds field is set.
*/
@java.lang.Override
public boolean hasPassIds() {
return targetCase_ == 2;
}
/**
* .io.PassIds passIds = 2;
* @return The passIds.
*/
@java.lang.Override
public com.passkit.grpc.CommonObjects.PassIds getPassIds() {
if (passIdsBuilder_ == null) {
if (targetCase_ == 2) {
return (com.passkit.grpc.CommonObjects.PassIds) target_;
}
return com.passkit.grpc.CommonObjects.PassIds.getDefaultInstance();
} else {
if (targetCase_ == 2) {
return passIdsBuilder_.getMessage();
}
return com.passkit.grpc.CommonObjects.PassIds.getDefaultInstance();
}
}
/**
* .io.PassIds passIds = 2;
*/
public Builder setPassIds(com.passkit.grpc.CommonObjects.PassIds value) {
if (passIdsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
target_ = value;
onChanged();
} else {
passIdsBuilder_.setMessage(value);
}
targetCase_ = 2;
return this;
}
/**
* .io.PassIds passIds = 2;
*/
public Builder setPassIds(
com.passkit.grpc.CommonObjects.PassIds.Builder builderForValue) {
if (passIdsBuilder_ == null) {
target_ = builderForValue.build();
onChanged();
} else {
passIdsBuilder_.setMessage(builderForValue.build());
}
targetCase_ = 2;
return this;
}
/**
* .io.PassIds passIds = 2;
*/
public Builder mergePassIds(com.passkit.grpc.CommonObjects.PassIds value) {
if (passIdsBuilder_ == null) {
if (targetCase_ == 2 &&
target_ != com.passkit.grpc.CommonObjects.PassIds.getDefaultInstance()) {
target_ = com.passkit.grpc.CommonObjects.PassIds.newBuilder((com.passkit.grpc.CommonObjects.PassIds) target_)
.mergeFrom(value).buildPartial();
} else {
target_ = value;
}
onChanged();
} else {
if (targetCase_ == 2) {
passIdsBuilder_.mergeFrom(value);
}
passIdsBuilder_.setMessage(value);
}
targetCase_ = 2;
return this;
}
/**
* .io.PassIds passIds = 2;
*/
public Builder clearPassIds() {
if (passIdsBuilder_ == null) {
if (targetCase_ == 2) {
targetCase_ = 0;
target_ = null;
onChanged();
}
} else {
if (targetCase_ == 2) {
targetCase_ = 0;
target_ = null;
}
passIdsBuilder_.clear();
}
return this;
}
/**
* .io.PassIds passIds = 2;
*/
public com.passkit.grpc.CommonObjects.PassIds.Builder getPassIdsBuilder() {
return getPassIdsFieldBuilder().getBuilder();
}
/**
* .io.PassIds passIds = 2;
*/
@java.lang.Override
public com.passkit.grpc.CommonObjects.PassIdsOrBuilder getPassIdsOrBuilder() {
if ((targetCase_ == 2) && (passIdsBuilder_ != null)) {
return passIdsBuilder_.getMessageOrBuilder();
} else {
if (targetCase_ == 2) {
return (com.passkit.grpc.CommonObjects.PassIds) target_;
}
return com.passkit.grpc.CommonObjects.PassIds.getDefaultInstance();
}
}
/**
* .io.PassIds passIds = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.passkit.grpc.CommonObjects.PassIds, com.passkit.grpc.CommonObjects.PassIds.Builder, com.passkit.grpc.CommonObjects.PassIdsOrBuilder>
getPassIdsFieldBuilder() {
if (passIdsBuilder_ == null) {
if (!(targetCase_ == 2)) {
target_ = com.passkit.grpc.CommonObjects.PassIds.getDefaultInstance();
}
passIdsBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.passkit.grpc.CommonObjects.PassIds, com.passkit.grpc.CommonObjects.PassIds.Builder, com.passkit.grpc.CommonObjects.PassIdsOrBuilder>(
(com.passkit.grpc.CommonObjects.PassIds) target_,
getParentForChildren(),
isClean());
target_ = null;
}
targetCase_ = 2;
onChanged();;
return passIdsBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
com.passkit.grpc.CommonObjects.Protocol, com.passkit.grpc.CommonObjects.Protocol.Builder, com.passkit.grpc.CommonObjects.ProtocolOrBuilder> protocolBuilder_;
/**
* .io.Protocol protocol = 3;
* @return Whether the protocol field is set.
*/
@java.lang.Override
public boolean hasProtocol() {
return targetCase_ == 3;
}
/**
* .io.Protocol protocol = 3;
* @return The protocol.
*/
@java.lang.Override
public com.passkit.grpc.CommonObjects.Protocol getProtocol() {
if (protocolBuilder_ == null) {
if (targetCase_ == 3) {
return (com.passkit.grpc.CommonObjects.Protocol) target_;
}
return com.passkit.grpc.CommonObjects.Protocol.getDefaultInstance();
} else {
if (targetCase_ == 3) {
return protocolBuilder_.getMessage();
}
return com.passkit.grpc.CommonObjects.Protocol.getDefaultInstance();
}
}
/**
* .io.Protocol protocol = 3;
*/
public Builder setProtocol(com.passkit.grpc.CommonObjects.Protocol value) {
if (protocolBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
target_ = value;
onChanged();
} else {
protocolBuilder_.setMessage(value);
}
targetCase_ = 3;
return this;
}
/**
* .io.Protocol protocol = 3;
*/
public Builder setProtocol(
com.passkit.grpc.CommonObjects.Protocol.Builder builderForValue) {
if (protocolBuilder_ == null) {
target_ = builderForValue.build();
onChanged();
} else {
protocolBuilder_.setMessage(builderForValue.build());
}
targetCase_ = 3;
return this;
}
/**
* .io.Protocol protocol = 3;
*/
public Builder mergeProtocol(com.passkit.grpc.CommonObjects.Protocol value) {
if (protocolBuilder_ == null) {
if (targetCase_ == 3 &&
target_ != com.passkit.grpc.CommonObjects.Protocol.getDefaultInstance()) {
target_ = com.passkit.grpc.CommonObjects.Protocol.newBuilder((com.passkit.grpc.CommonObjects.Protocol) target_)
.mergeFrom(value).buildPartial();
} else {
target_ = value;
}
onChanged();
} else {
if (targetCase_ == 3) {
protocolBuilder_.mergeFrom(value);
}
protocolBuilder_.setMessage(value);
}
targetCase_ = 3;
return this;
}
/**
* .io.Protocol protocol = 3;
*/
public Builder clearProtocol() {
if (protocolBuilder_ == null) {
if (targetCase_ == 3) {
targetCase_ = 0;
target_ = null;
onChanged();
}
} else {
if (targetCase_ == 3) {
targetCase_ = 0;
target_ = null;
}
protocolBuilder_.clear();
}
return this;
}
/**
* .io.Protocol protocol = 3;
*/
public com.passkit.grpc.CommonObjects.Protocol.Builder getProtocolBuilder() {
return getProtocolFieldBuilder().getBuilder();
}
/**
* .io.Protocol protocol = 3;
*/
@java.lang.Override
public com.passkit.grpc.CommonObjects.ProtocolOrBuilder getProtocolOrBuilder() {
if ((targetCase_ == 3) && (protocolBuilder_ != null)) {
return protocolBuilder_.getMessageOrBuilder();
} else {
if (targetCase_ == 3) {
return (com.passkit.grpc.CommonObjects.Protocol) target_;
}
return com.passkit.grpc.CommonObjects.Protocol.getDefaultInstance();
}
}
/**
* .io.Protocol protocol = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.passkit.grpc.CommonObjects.Protocol, com.passkit.grpc.CommonObjects.Protocol.Builder, com.passkit.grpc.CommonObjects.ProtocolOrBuilder>
getProtocolFieldBuilder() {
if (protocolBuilder_ == null) {
if (!(targetCase_ == 3)) {
target_ = com.passkit.grpc.CommonObjects.Protocol.getDefaultInstance();
}
protocolBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.passkit.grpc.CommonObjects.Protocol, com.passkit.grpc.CommonObjects.Protocol.Builder, com.passkit.grpc.CommonObjects.ProtocolOrBuilder>(
(com.passkit.grpc.CommonObjects.Protocol) target_,
getParentForChildren(),
isClean());
target_ = null;
}
targetCase_ = 3;
onChanged();;
return protocolBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:io.SendMessageRequest)
}
// @@protoc_insertion_point(class_scope:io.SendMessageRequest)
private static final com.passkit.grpc.MessageOuterClass.SendMessageRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.passkit.grpc.MessageOuterClass.SendMessageRequest();
}
public static com.passkit.grpc.MessageOuterClass.SendMessageRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public SendMessageRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new SendMessageRequest(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.passkit.grpc.MessageOuterClass.SendMessageRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface SendMessageResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:io.SendMessageResponse)
com.google.protobuf.MessageOrBuilder {
/**
* int32 count = 1;
* @return The count.
*/
int getCount();
}
/**
* Protobuf type {@code io.SendMessageResponse}
*/
public static final class SendMessageResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:io.SendMessageResponse)
SendMessageResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use SendMessageResponse.newBuilder() to construct.
private SendMessageResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private SendMessageResponse() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new SendMessageResponse();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private SendMessageResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
count_ = input.readInt32();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.passkit.grpc.MessageOuterClass.internal_static_io_SendMessageResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.passkit.grpc.MessageOuterClass.internal_static_io_SendMessageResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.passkit.grpc.MessageOuterClass.SendMessageResponse.class, com.passkit.grpc.MessageOuterClass.SendMessageResponse.Builder.class);
}
public static final int COUNT_FIELD_NUMBER = 1;
private int count_;
/**
* int32 count = 1;
* @return The count.
*/
@java.lang.Override
public int getCount() {
return count_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (count_ != 0) {
output.writeInt32(1, count_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (count_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1, count_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.passkit.grpc.MessageOuterClass.SendMessageResponse)) {
return super.equals(obj);
}
com.passkit.grpc.MessageOuterClass.SendMessageResponse other = (com.passkit.grpc.MessageOuterClass.SendMessageResponse) obj;
if (getCount()
!= other.getCount()) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + COUNT_FIELD_NUMBER;
hash = (53 * hash) + getCount();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.passkit.grpc.MessageOuterClass.SendMessageResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.passkit.grpc.MessageOuterClass.SendMessageResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.passkit.grpc.MessageOuterClass.SendMessageResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.passkit.grpc.MessageOuterClass.SendMessageResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.passkit.grpc.MessageOuterClass.SendMessageResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.passkit.grpc.MessageOuterClass.SendMessageResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.passkit.grpc.MessageOuterClass.SendMessageResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.passkit.grpc.MessageOuterClass.SendMessageResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.passkit.grpc.MessageOuterClass.SendMessageResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.passkit.grpc.MessageOuterClass.SendMessageResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.passkit.grpc.MessageOuterClass.SendMessageResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.passkit.grpc.MessageOuterClass.SendMessageResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.passkit.grpc.MessageOuterClass.SendMessageResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code io.SendMessageResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:io.SendMessageResponse)
com.passkit.grpc.MessageOuterClass.SendMessageResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.passkit.grpc.MessageOuterClass.internal_static_io_SendMessageResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.passkit.grpc.MessageOuterClass.internal_static_io_SendMessageResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.passkit.grpc.MessageOuterClass.SendMessageResponse.class, com.passkit.grpc.MessageOuterClass.SendMessageResponse.Builder.class);
}
// Construct using com.passkit.grpc.MessageOuterClass.SendMessageResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
count_ = 0;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.passkit.grpc.MessageOuterClass.internal_static_io_SendMessageResponse_descriptor;
}
@java.lang.Override
public com.passkit.grpc.MessageOuterClass.SendMessageResponse getDefaultInstanceForType() {
return com.passkit.grpc.MessageOuterClass.SendMessageResponse.getDefaultInstance();
}
@java.lang.Override
public com.passkit.grpc.MessageOuterClass.SendMessageResponse build() {
com.passkit.grpc.MessageOuterClass.SendMessageResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.passkit.grpc.MessageOuterClass.SendMessageResponse buildPartial() {
com.passkit.grpc.MessageOuterClass.SendMessageResponse result = new com.passkit.grpc.MessageOuterClass.SendMessageResponse(this);
result.count_ = count_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.passkit.grpc.MessageOuterClass.SendMessageResponse) {
return mergeFrom((com.passkit.grpc.MessageOuterClass.SendMessageResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.passkit.grpc.MessageOuterClass.SendMessageResponse other) {
if (other == com.passkit.grpc.MessageOuterClass.SendMessageResponse.getDefaultInstance()) return this;
if (other.getCount() != 0) {
setCount(other.getCount());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.passkit.grpc.MessageOuterClass.SendMessageResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.passkit.grpc.MessageOuterClass.SendMessageResponse) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int count_ ;
/**
* int32 count = 1;
* @return The count.
*/
@java.lang.Override
public int getCount() {
return count_;
}
/**
* int32 count = 1;
* @param value The count to set.
* @return This builder for chaining.
*/
public Builder setCount(int value) {
count_ = value;
onChanged();
return this;
}
/**
* int32 count = 1;
* @return This builder for chaining.
*/
public Builder clearCount() {
count_ = 0;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:io.SendMessageResponse)
}
// @@protoc_insertion_point(class_scope:io.SendMessageResponse)
private static final com.passkit.grpc.MessageOuterClass.SendMessageResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.passkit.grpc.MessageOuterClass.SendMessageResponse();
}
public static com.passkit.grpc.MessageOuterClass.SendMessageResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public SendMessageResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new SendMessageResponse(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.passkit.grpc.MessageOuterClass.SendMessageResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_io_Message_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_io_Message_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_io_GetMessagesForProtocolRequest_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_io_GetMessagesForProtocolRequest_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_io_GetMessageResponse_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_io_GetMessageResponse_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_io_GetMessageHistoryResponse_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_io_GetMessageHistoryResponse_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_io_SendMessageRequest_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_io_SendMessageRequest_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_io_SendMessageResponse_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_io_SendMessageResponse_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n\027io/common/message.proto\022\002io\032\034io/common" +
"/localization.proto\032\032io/common/paginatio" +
"n.proto\032\036io/common/common_objects.proto\032" +
"\024io/image/image.proto\032\037google/protobuf/t" +
"imestamp.proto\"\345\002\n\007Message\022\n\n\002id\030\001 \001(\t\022\r" +
"\n\005title\030\002 \001(\t\022+\n\016localizedTitle\030\003 \001(\0132\023." +
"io.LocalizedString\022\030\n\020plainTextContent\030\004" +
" \001(\t\0226\n\031localizedPlainTextContent\030\005 \001(\0132" +
"\023.io.LocalizedString\022\027\n\017richTextContent\030" +
"\006 \001(\t\0225\n\030localizedRichTextContent\030\007 \001(\0132" +
"\023.io.LocalizedString\022\025\n\004urls\030\010 \003(\0132\007.io." +
"Url\022\034\n\006images\030\t \001(\0132\014.io.ImageIds\022\020\n\010pri" +
"ority\030\n \001(\r\022\023\n\013displayFrom\030\013 \001(\t\022\024\n\014disp" +
"layUntil\030\014 \001(\t\"f\n\035GetMessagesForProtocol" +
"Request\022\020\n\010protocol\030\001 \001(\t\022\017\n\007classId\030\002 \001" +
"(\t\022\"\n\npagination\030\003 \001(\0132\016.io.Pagination\"\253" +
"\002\n\022GetMessageResponse\022\034\n\007message\030\001 \001(\0132\013" +
".io.Message\022!\n\006status\030\002 \001(\0162\021.io.Message" +
"Status\022\022\n\nrecipients\030\003 \001(\r\0221\n\rdistribute" +
"dAt\030\004 \001(\0132\032.google.protobuf.Timestamp\022/\n" +
"\013withdrawnAt\030\005 \001(\0132\032.google.protobuf.Tim" +
"estamp\022-\n\tcreatedAt\030\006 \001(\0132\032.google.proto" +
"buf.Timestamp\022-\n\tupdatedAt\030\007 \001(\0132\032.googl" +
"e.protobuf.Timestamp\"E\n\031GetMessageHistor" +
"yResponse\022(\n\010messages\030\001 \003(\0132\026.io.GetMess" +
"ageResponse\"s\n\022SendMessageRequest\022\021\n\tmes" +
"sageId\030\001 \001(\t\022\036\n\007passIds\030\002 \001(\0132\013.io.PassI" +
"dsH\000\022 \n\010protocol\030\003 \001(\0132\014.io.ProtocolH\000B\010" +
"\n\006target\"$\n\023SendMessageResponse\022\r\n\005count" +
"\030\001 \001(\005*e\n\rMessageStatus\022\035\n\031MESSAGE_STATU" +
"S_DO_NOT_USE\020\000\022\r\n\tSCHEDULED\020\001\022\n\n\006ACTIVE\020" +
"\002\022\013\n\007EXPIRED\020\003\022\r\n\tCANCELLED\020\004BG\n\020com.pas" +
"skit.grpcZ$stash.passkit.com/io/model/sd" +
"k/go/io\252\002\014PassKit.Grpcb\006proto3"
};
descriptor = com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
com.passkit.grpc.Localization.getDescriptor(),
com.passkit.grpc.PaginationOuterClass.getDescriptor(),
com.passkit.grpc.CommonObjects.getDescriptor(),
com.passkit.grpc.Image.getDescriptor(),
com.google.protobuf.TimestampProto.getDescriptor(),
});
internal_static_io_Message_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_io_Message_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_io_Message_descriptor,
new java.lang.String[] { "Id", "Title", "LocalizedTitle", "PlainTextContent", "LocalizedPlainTextContent", "RichTextContent", "LocalizedRichTextContent", "Urls", "Images", "Priority", "DisplayFrom", "DisplayUntil", });
internal_static_io_GetMessagesForProtocolRequest_descriptor =
getDescriptor().getMessageTypes().get(1);
internal_static_io_GetMessagesForProtocolRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_io_GetMessagesForProtocolRequest_descriptor,
new java.lang.String[] { "Protocol", "ClassId", "Pagination", });
internal_static_io_GetMessageResponse_descriptor =
getDescriptor().getMessageTypes().get(2);
internal_static_io_GetMessageResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_io_GetMessageResponse_descriptor,
new java.lang.String[] { "Message", "Status", "Recipients", "DistributedAt", "WithdrawnAt", "CreatedAt", "UpdatedAt", });
internal_static_io_GetMessageHistoryResponse_descriptor =
getDescriptor().getMessageTypes().get(3);
internal_static_io_GetMessageHistoryResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_io_GetMessageHistoryResponse_descriptor,
new java.lang.String[] { "Messages", });
internal_static_io_SendMessageRequest_descriptor =
getDescriptor().getMessageTypes().get(4);
internal_static_io_SendMessageRequest_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_io_SendMessageRequest_descriptor,
new java.lang.String[] { "MessageId", "PassIds", "Protocol", "Target", });
internal_static_io_SendMessageResponse_descriptor =
getDescriptor().getMessageTypes().get(5);
internal_static_io_SendMessageResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_io_SendMessageResponse_descriptor,
new java.lang.String[] { "Count", });
com.passkit.grpc.Localization.getDescriptor();
com.passkit.grpc.PaginationOuterClass.getDescriptor();
com.passkit.grpc.CommonObjects.getDescriptor();
com.passkit.grpc.Image.getDescriptor();
com.google.protobuf.TimestampProto.getDescriptor();
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy