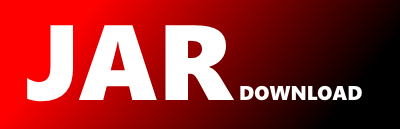
com.passkit.grpc.Scheduler.SchedulerGrpc Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sdk Show documentation
Show all versions of sdk Show documentation
SDK for the PassKit gRPC API that can be used to create, configure and manage Membership, Loyalty, Event Ticket, Coupon, Transit and Boarding Pass content for mobile wallet applications, including Apple Pay and Google Pay.
package com.passkit.grpc.Scheduler;
import static io.grpc.MethodDescriptor.generateFullMethodName;
/**
*/
@javax.annotation.Generated(
value = "by gRPC proto compiler (version 1.44.1)",
comments = "Source: io/scheduler/a_rpc.proto")
@io.grpc.stub.annotations.GrpcGenerated
public final class SchedulerGrpc {
private SchedulerGrpc() {}
public static final String SERVICE_NAME = "scheduler.Scheduler";
// Static method descriptors that strictly reflect the proto.
private static volatile io.grpc.MethodDescriptor getCreateSchedulingJobMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "createSchedulingJob",
requestType = com.passkit.grpc.ct.Scheduler.SchedulingJob.class,
responseType = com.passkit.grpc.ct.Scheduler.SchedulingJobResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getCreateSchedulingJobMethod() {
io.grpc.MethodDescriptor getCreateSchedulingJobMethod;
if ((getCreateSchedulingJobMethod = SchedulerGrpc.getCreateSchedulingJobMethod) == null) {
synchronized (SchedulerGrpc.class) {
if ((getCreateSchedulingJobMethod = SchedulerGrpc.getCreateSchedulingJobMethod) == null) {
SchedulerGrpc.getCreateSchedulingJobMethod = getCreateSchedulingJobMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "createSchedulingJob"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.passkit.grpc.ct.Scheduler.SchedulingJob.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.passkit.grpc.ct.Scheduler.SchedulingJobResponse.getDefaultInstance()))
.setSchemaDescriptor(new SchedulerMethodDescriptorSupplier("createSchedulingJob"))
.build();
}
}
}
return getCreateSchedulingJobMethod;
}
private static volatile io.grpc.MethodDescriptor getGetSchedulingJobMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "getSchedulingJob",
requestType = com.passkit.grpc.CommonObjects.Id.class,
responseType = com.passkit.grpc.ct.Scheduler.SchedulingJob.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getGetSchedulingJobMethod() {
io.grpc.MethodDescriptor getGetSchedulingJobMethod;
if ((getGetSchedulingJobMethod = SchedulerGrpc.getGetSchedulingJobMethod) == null) {
synchronized (SchedulerGrpc.class) {
if ((getGetSchedulingJobMethod = SchedulerGrpc.getGetSchedulingJobMethod) == null) {
SchedulerGrpc.getGetSchedulingJobMethod = getGetSchedulingJobMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "getSchedulingJob"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.passkit.grpc.CommonObjects.Id.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.passkit.grpc.ct.Scheduler.SchedulingJob.getDefaultInstance()))
.setSchemaDescriptor(new SchedulerMethodDescriptorSupplier("getSchedulingJob"))
.build();
}
}
}
return getGetSchedulingJobMethod;
}
private static volatile io.grpc.MethodDescriptor getUpdateSchedulingJobMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "updateSchedulingJob",
requestType = com.passkit.grpc.ct.Scheduler.SchedulingJob.class,
responseType = com.passkit.grpc.ct.Scheduler.SchedulingJobResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getUpdateSchedulingJobMethod() {
io.grpc.MethodDescriptor getUpdateSchedulingJobMethod;
if ((getUpdateSchedulingJobMethod = SchedulerGrpc.getUpdateSchedulingJobMethod) == null) {
synchronized (SchedulerGrpc.class) {
if ((getUpdateSchedulingJobMethod = SchedulerGrpc.getUpdateSchedulingJobMethod) == null) {
SchedulerGrpc.getUpdateSchedulingJobMethod = getUpdateSchedulingJobMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "updateSchedulingJob"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.passkit.grpc.ct.Scheduler.SchedulingJob.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.passkit.grpc.ct.Scheduler.SchedulingJobResponse.getDefaultInstance()))
.setSchemaDescriptor(new SchedulerMethodDescriptorSupplier("updateSchedulingJob"))
.build();
}
}
}
return getUpdateSchedulingJobMethod;
}
private static volatile io.grpc.MethodDescriptor getPatchSchedulingJobMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "patchSchedulingJob",
requestType = com.passkit.grpc.ct.Scheduler.SchedulingJob.class,
responseType = com.passkit.grpc.ct.Scheduler.SchedulingJobResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getPatchSchedulingJobMethod() {
io.grpc.MethodDescriptor getPatchSchedulingJobMethod;
if ((getPatchSchedulingJobMethod = SchedulerGrpc.getPatchSchedulingJobMethod) == null) {
synchronized (SchedulerGrpc.class) {
if ((getPatchSchedulingJobMethod = SchedulerGrpc.getPatchSchedulingJobMethod) == null) {
SchedulerGrpc.getPatchSchedulingJobMethod = getPatchSchedulingJobMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "patchSchedulingJob"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.passkit.grpc.ct.Scheduler.SchedulingJob.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.passkit.grpc.ct.Scheduler.SchedulingJobResponse.getDefaultInstance()))
.setSchemaDescriptor(new SchedulerMethodDescriptorSupplier("patchSchedulingJob"))
.build();
}
}
}
return getPatchSchedulingJobMethod;
}
private static volatile io.grpc.MethodDescriptor getDeleteSchedulingJobMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "deleteSchedulingJob",
requestType = com.passkit.grpc.CommonObjects.Id.class,
responseType = com.google.protobuf.Empty.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getDeleteSchedulingJobMethod() {
io.grpc.MethodDescriptor getDeleteSchedulingJobMethod;
if ((getDeleteSchedulingJobMethod = SchedulerGrpc.getDeleteSchedulingJobMethod) == null) {
synchronized (SchedulerGrpc.class) {
if ((getDeleteSchedulingJobMethod = SchedulerGrpc.getDeleteSchedulingJobMethod) == null) {
SchedulerGrpc.getDeleteSchedulingJobMethod = getDeleteSchedulingJobMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "deleteSchedulingJob"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.passkit.grpc.CommonObjects.Id.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.google.protobuf.Empty.getDefaultInstance()))
.setSchemaDescriptor(new SchedulerMethodDescriptorSupplier("deleteSchedulingJob"))
.build();
}
}
}
return getDeleteSchedulingJobMethod;
}
private static volatile io.grpc.MethodDescriptor getGetSchedulingJobHistoryMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "getSchedulingJobHistory",
requestType = com.passkit.grpc.CommonObjects.Id.class,
responseType = com.passkit.grpc.ct.Scheduler.JobHistory.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getGetSchedulingJobHistoryMethod() {
io.grpc.MethodDescriptor getGetSchedulingJobHistoryMethod;
if ((getGetSchedulingJobHistoryMethod = SchedulerGrpc.getGetSchedulingJobHistoryMethod) == null) {
synchronized (SchedulerGrpc.class) {
if ((getGetSchedulingJobHistoryMethod = SchedulerGrpc.getGetSchedulingJobHistoryMethod) == null) {
SchedulerGrpc.getGetSchedulingJobHistoryMethod = getGetSchedulingJobHistoryMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "getSchedulingJobHistory"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.passkit.grpc.CommonObjects.Id.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.passkit.grpc.ct.Scheduler.JobHistory.getDefaultInstance()))
.setSchemaDescriptor(new SchedulerMethodDescriptorSupplier("getSchedulingJobHistory"))
.build();
}
}
}
return getGetSchedulingJobHistoryMethod;
}
private static volatile io.grpc.MethodDescriptor getListSchedulingJobHistoriesMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "listSchedulingJobHistories",
requestType = com.passkit.grpc.Scheduler.Scheduler.ListRequest.class,
responseType = com.passkit.grpc.ct.Scheduler.JobHistory.class,
methodType = io.grpc.MethodDescriptor.MethodType.SERVER_STREAMING)
public static io.grpc.MethodDescriptor getListSchedulingJobHistoriesMethod() {
io.grpc.MethodDescriptor getListSchedulingJobHistoriesMethod;
if ((getListSchedulingJobHistoriesMethod = SchedulerGrpc.getListSchedulingJobHistoriesMethod) == null) {
synchronized (SchedulerGrpc.class) {
if ((getListSchedulingJobHistoriesMethod = SchedulerGrpc.getListSchedulingJobHistoriesMethod) == null) {
SchedulerGrpc.getListSchedulingJobHistoriesMethod = getListSchedulingJobHistoriesMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.SERVER_STREAMING)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "listSchedulingJobHistories"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.passkit.grpc.Scheduler.Scheduler.ListRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.passkit.grpc.ct.Scheduler.JobHistory.getDefaultInstance()))
.setSchemaDescriptor(new SchedulerMethodDescriptorSupplier("listSchedulingJobHistories"))
.build();
}
}
}
return getListSchedulingJobHistoriesMethod;
}
/**
* Creates a new async stub that supports all call types for the service
*/
public static SchedulerStub newStub(io.grpc.Channel channel) {
io.grpc.stub.AbstractStub.StubFactory factory =
new io.grpc.stub.AbstractStub.StubFactory() {
@java.lang.Override
public SchedulerStub newStub(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new SchedulerStub(channel, callOptions);
}
};
return SchedulerStub.newStub(factory, channel);
}
/**
* Creates a new blocking-style stub that supports unary and streaming output calls on the service
*/
public static SchedulerBlockingStub newBlockingStub(
io.grpc.Channel channel) {
io.grpc.stub.AbstractStub.StubFactory factory =
new io.grpc.stub.AbstractStub.StubFactory() {
@java.lang.Override
public SchedulerBlockingStub newStub(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new SchedulerBlockingStub(channel, callOptions);
}
};
return SchedulerBlockingStub.newStub(factory, channel);
}
/**
* Creates a new ListenableFuture-style stub that supports unary calls on the service
*/
public static SchedulerFutureStub newFutureStub(
io.grpc.Channel channel) {
io.grpc.stub.AbstractStub.StubFactory factory =
new io.grpc.stub.AbstractStub.StubFactory() {
@java.lang.Override
public SchedulerFutureStub newStub(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new SchedulerFutureStub(channel, callOptions);
}
};
return SchedulerFutureStub.newStub(factory, channel);
}
/**
*/
public static abstract class SchedulerImplBase implements io.grpc.BindableService {
/**
*/
public void createSchedulingJob(com.passkit.grpc.ct.Scheduler.SchedulingJob request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getCreateSchedulingJobMethod(), responseObserver);
}
/**
*/
public void getSchedulingJob(com.passkit.grpc.CommonObjects.Id request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getGetSchedulingJobMethod(), responseObserver);
}
/**
*/
public void updateSchedulingJob(com.passkit.grpc.ct.Scheduler.SchedulingJob request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getUpdateSchedulingJobMethod(), responseObserver);
}
/**
*/
public void patchSchedulingJob(com.passkit.grpc.ct.Scheduler.SchedulingJob request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getPatchSchedulingJobMethod(), responseObserver);
}
/**
*/
public void deleteSchedulingJob(com.passkit.grpc.CommonObjects.Id request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getDeleteSchedulingJobMethod(), responseObserver);
}
/**
*/
public void getSchedulingJobHistory(com.passkit.grpc.CommonObjects.Id request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getGetSchedulingJobHistoryMethod(), responseObserver);
}
/**
*/
public void listSchedulingJobHistories(com.passkit.grpc.Scheduler.Scheduler.ListRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getListSchedulingJobHistoriesMethod(), responseObserver);
}
@java.lang.Override public final io.grpc.ServerServiceDefinition bindService() {
return io.grpc.ServerServiceDefinition.builder(getServiceDescriptor())
.addMethod(
getCreateSchedulingJobMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.passkit.grpc.ct.Scheduler.SchedulingJob,
com.passkit.grpc.ct.Scheduler.SchedulingJobResponse>(
this, METHODID_CREATE_SCHEDULING_JOB)))
.addMethod(
getGetSchedulingJobMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.passkit.grpc.CommonObjects.Id,
com.passkit.grpc.ct.Scheduler.SchedulingJob>(
this, METHODID_GET_SCHEDULING_JOB)))
.addMethod(
getUpdateSchedulingJobMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.passkit.grpc.ct.Scheduler.SchedulingJob,
com.passkit.grpc.ct.Scheduler.SchedulingJobResponse>(
this, METHODID_UPDATE_SCHEDULING_JOB)))
.addMethod(
getPatchSchedulingJobMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.passkit.grpc.ct.Scheduler.SchedulingJob,
com.passkit.grpc.ct.Scheduler.SchedulingJobResponse>(
this, METHODID_PATCH_SCHEDULING_JOB)))
.addMethod(
getDeleteSchedulingJobMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.passkit.grpc.CommonObjects.Id,
com.google.protobuf.Empty>(
this, METHODID_DELETE_SCHEDULING_JOB)))
.addMethod(
getGetSchedulingJobHistoryMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.passkit.grpc.CommonObjects.Id,
com.passkit.grpc.ct.Scheduler.JobHistory>(
this, METHODID_GET_SCHEDULING_JOB_HISTORY)))
.addMethod(
getListSchedulingJobHistoriesMethod(),
io.grpc.stub.ServerCalls.asyncServerStreamingCall(
new MethodHandlers<
com.passkit.grpc.Scheduler.Scheduler.ListRequest,
com.passkit.grpc.ct.Scheduler.JobHistory>(
this, METHODID_LIST_SCHEDULING_JOB_HISTORIES)))
.build();
}
}
/**
*/
public static final class SchedulerStub extends io.grpc.stub.AbstractAsyncStub {
private SchedulerStub(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected SchedulerStub build(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new SchedulerStub(channel, callOptions);
}
/**
*/
public void createSchedulingJob(com.passkit.grpc.ct.Scheduler.SchedulingJob request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getCreateSchedulingJobMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void getSchedulingJob(com.passkit.grpc.CommonObjects.Id request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getGetSchedulingJobMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void updateSchedulingJob(com.passkit.grpc.ct.Scheduler.SchedulingJob request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getUpdateSchedulingJobMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void patchSchedulingJob(com.passkit.grpc.ct.Scheduler.SchedulingJob request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getPatchSchedulingJobMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void deleteSchedulingJob(com.passkit.grpc.CommonObjects.Id request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getDeleteSchedulingJobMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void getSchedulingJobHistory(com.passkit.grpc.CommonObjects.Id request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getGetSchedulingJobHistoryMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void listSchedulingJobHistories(com.passkit.grpc.Scheduler.Scheduler.ListRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncServerStreamingCall(
getChannel().newCall(getListSchedulingJobHistoriesMethod(), getCallOptions()), request, responseObserver);
}
}
/**
*/
public static final class SchedulerBlockingStub extends io.grpc.stub.AbstractBlockingStub {
private SchedulerBlockingStub(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected SchedulerBlockingStub build(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new SchedulerBlockingStub(channel, callOptions);
}
/**
*/
public com.passkit.grpc.ct.Scheduler.SchedulingJobResponse createSchedulingJob(com.passkit.grpc.ct.Scheduler.SchedulingJob request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getCreateSchedulingJobMethod(), getCallOptions(), request);
}
/**
*/
public com.passkit.grpc.ct.Scheduler.SchedulingJob getSchedulingJob(com.passkit.grpc.CommonObjects.Id request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getGetSchedulingJobMethod(), getCallOptions(), request);
}
/**
*/
public com.passkit.grpc.ct.Scheduler.SchedulingJobResponse updateSchedulingJob(com.passkit.grpc.ct.Scheduler.SchedulingJob request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getUpdateSchedulingJobMethod(), getCallOptions(), request);
}
/**
*/
public com.passkit.grpc.ct.Scheduler.SchedulingJobResponse patchSchedulingJob(com.passkit.grpc.ct.Scheduler.SchedulingJob request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getPatchSchedulingJobMethod(), getCallOptions(), request);
}
/**
*/
public com.google.protobuf.Empty deleteSchedulingJob(com.passkit.grpc.CommonObjects.Id request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getDeleteSchedulingJobMethod(), getCallOptions(), request);
}
/**
*/
public com.passkit.grpc.ct.Scheduler.JobHistory getSchedulingJobHistory(com.passkit.grpc.CommonObjects.Id request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getGetSchedulingJobHistoryMethod(), getCallOptions(), request);
}
/**
*/
public java.util.Iterator listSchedulingJobHistories(
com.passkit.grpc.Scheduler.Scheduler.ListRequest request) {
return io.grpc.stub.ClientCalls.blockingServerStreamingCall(
getChannel(), getListSchedulingJobHistoriesMethod(), getCallOptions(), request);
}
}
/**
*/
public static final class SchedulerFutureStub extends io.grpc.stub.AbstractFutureStub {
private SchedulerFutureStub(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected SchedulerFutureStub build(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new SchedulerFutureStub(channel, callOptions);
}
/**
*/
public com.google.common.util.concurrent.ListenableFuture createSchedulingJob(
com.passkit.grpc.ct.Scheduler.SchedulingJob request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getCreateSchedulingJobMethod(), getCallOptions()), request);
}
/**
*/
public com.google.common.util.concurrent.ListenableFuture getSchedulingJob(
com.passkit.grpc.CommonObjects.Id request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getGetSchedulingJobMethod(), getCallOptions()), request);
}
/**
*/
public com.google.common.util.concurrent.ListenableFuture updateSchedulingJob(
com.passkit.grpc.ct.Scheduler.SchedulingJob request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getUpdateSchedulingJobMethod(), getCallOptions()), request);
}
/**
*/
public com.google.common.util.concurrent.ListenableFuture patchSchedulingJob(
com.passkit.grpc.ct.Scheduler.SchedulingJob request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getPatchSchedulingJobMethod(), getCallOptions()), request);
}
/**
*/
public com.google.common.util.concurrent.ListenableFuture deleteSchedulingJob(
com.passkit.grpc.CommonObjects.Id request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getDeleteSchedulingJobMethod(), getCallOptions()), request);
}
/**
*/
public com.google.common.util.concurrent.ListenableFuture getSchedulingJobHistory(
com.passkit.grpc.CommonObjects.Id request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getGetSchedulingJobHistoryMethod(), getCallOptions()), request);
}
}
private static final int METHODID_CREATE_SCHEDULING_JOB = 0;
private static final int METHODID_GET_SCHEDULING_JOB = 1;
private static final int METHODID_UPDATE_SCHEDULING_JOB = 2;
private static final int METHODID_PATCH_SCHEDULING_JOB = 3;
private static final int METHODID_DELETE_SCHEDULING_JOB = 4;
private static final int METHODID_GET_SCHEDULING_JOB_HISTORY = 5;
private static final int METHODID_LIST_SCHEDULING_JOB_HISTORIES = 6;
private static final class MethodHandlers implements
io.grpc.stub.ServerCalls.UnaryMethod,
io.grpc.stub.ServerCalls.ServerStreamingMethod,
io.grpc.stub.ServerCalls.ClientStreamingMethod,
io.grpc.stub.ServerCalls.BidiStreamingMethod {
private final SchedulerImplBase serviceImpl;
private final int methodId;
MethodHandlers(SchedulerImplBase serviceImpl, int methodId) {
this.serviceImpl = serviceImpl;
this.methodId = methodId;
}
@java.lang.Override
@java.lang.SuppressWarnings("unchecked")
public void invoke(Req request, io.grpc.stub.StreamObserver responseObserver) {
switch (methodId) {
case METHODID_CREATE_SCHEDULING_JOB:
serviceImpl.createSchedulingJob((com.passkit.grpc.ct.Scheduler.SchedulingJob) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_GET_SCHEDULING_JOB:
serviceImpl.getSchedulingJob((com.passkit.grpc.CommonObjects.Id) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_UPDATE_SCHEDULING_JOB:
serviceImpl.updateSchedulingJob((com.passkit.grpc.ct.Scheduler.SchedulingJob) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_PATCH_SCHEDULING_JOB:
serviceImpl.patchSchedulingJob((com.passkit.grpc.ct.Scheduler.SchedulingJob) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_DELETE_SCHEDULING_JOB:
serviceImpl.deleteSchedulingJob((com.passkit.grpc.CommonObjects.Id) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_GET_SCHEDULING_JOB_HISTORY:
serviceImpl.getSchedulingJobHistory((com.passkit.grpc.CommonObjects.Id) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_LIST_SCHEDULING_JOB_HISTORIES:
serviceImpl.listSchedulingJobHistories((com.passkit.grpc.Scheduler.Scheduler.ListRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
default:
throw new AssertionError();
}
}
@java.lang.Override
@java.lang.SuppressWarnings("unchecked")
public io.grpc.stub.StreamObserver invoke(
io.grpc.stub.StreamObserver responseObserver) {
switch (methodId) {
default:
throw new AssertionError();
}
}
}
private static abstract class SchedulerBaseDescriptorSupplier
implements io.grpc.protobuf.ProtoFileDescriptorSupplier, io.grpc.protobuf.ProtoServiceDescriptorSupplier {
SchedulerBaseDescriptorSupplier() {}
@java.lang.Override
public com.google.protobuf.Descriptors.FileDescriptor getFileDescriptor() {
return com.passkit.grpc.Scheduler.ARpc.getDescriptor();
}
@java.lang.Override
public com.google.protobuf.Descriptors.ServiceDescriptor getServiceDescriptor() {
return getFileDescriptor().findServiceByName("Scheduler");
}
}
private static final class SchedulerFileDescriptorSupplier
extends SchedulerBaseDescriptorSupplier {
SchedulerFileDescriptorSupplier() {}
}
private static final class SchedulerMethodDescriptorSupplier
extends SchedulerBaseDescriptorSupplier
implements io.grpc.protobuf.ProtoMethodDescriptorSupplier {
private final String methodName;
SchedulerMethodDescriptorSupplier(String methodName) {
this.methodName = methodName;
}
@java.lang.Override
public com.google.protobuf.Descriptors.MethodDescriptor getMethodDescriptor() {
return getServiceDescriptor().findMethodByName(methodName);
}
}
private static volatile io.grpc.ServiceDescriptor serviceDescriptor;
public static io.grpc.ServiceDescriptor getServiceDescriptor() {
io.grpc.ServiceDescriptor result = serviceDescriptor;
if (result == null) {
synchronized (SchedulerGrpc.class) {
result = serviceDescriptor;
if (result == null) {
serviceDescriptor = result = io.grpc.ServiceDescriptor.newBuilder(SERVICE_NAME)
.setSchemaDescriptor(new SchedulerFileDescriptorSupplier())
.addMethod(getCreateSchedulingJobMethod())
.addMethod(getGetSchedulingJobMethod())
.addMethod(getUpdateSchedulingJobMethod())
.addMethod(getPatchSchedulingJobMethod())
.addMethod(getDeleteSchedulingJobMethod())
.addMethod(getGetSchedulingJobHistoryMethod())
.addMethod(getListSchedulingJobHistoriesMethod())
.build();
}
}
}
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy