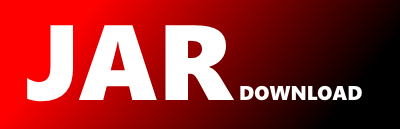
com.passkit.grpc.ct.Scheduler Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sdk Show documentation
Show all versions of sdk Show documentation
SDK for the PassKit gRPC API that can be used to create, configure and manage Membership, Loyalty, Event Ticket, Coupon, Transit and Boarding Pass content for mobile wallet applications, including Apple Pay and Google Pay.
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: ct/scheduler/scheduler.proto
package com.passkit.grpc.ct;
public final class Scheduler {
private Scheduler() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistryLite registry) {
}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
registerAllExtensions(
(com.google.protobuf.ExtensionRegistryLite) registry);
}
/**
* Protobuf enum {@code ct.JobStatus}
*/
public enum JobStatus
implements com.google.protobuf.ProtocolMessageEnum {
/**
* JOB_STATUS_NONE = 0;
*/
JOB_STATUS_NONE(0),
/**
*
* A job has been successfully created.
*
*
* JOB_STATUS_CREATED = 1;
*/
JOB_STATUS_CREATED(1),
/**
*
* A job has a next run datetime and in standby mode.
*
*
* JOB_STATUS_READY = 2;
*/
JOB_STATUS_READY(2),
/**
*
* When a job is flagged with inflight, the job has been dispatched and in process.
*
*
* JOB_STATUS_INFLIGHT = 3;
*/
JOB_STATUS_INFLIGHT(3),
/**
*
* A job has been completed and waiting to be assigned the next run datetime.
*
*
* JOB_STATUS_SUCCEEDED = 4;
*/
JOB_STATUS_SUCCEEDED(4),
/**
*
* A job has been failed. The history logging is available for the details.
*
*
* JOB_STATUS_FAILED = 5;
*/
JOB_STATUS_FAILED(5),
/**
*
* A job has been paused until resumed datetime or when resumed.
*
*
* JOB_STATUS_PAUSED = 6;
*/
JOB_STATUS_PAUSED(6),
/**
*
* A job has been expired and ready to be deleted. Expired jobs will be deleted.
*
*
* JOB_STATUS_EXPIRED = 7;
*/
JOB_STATUS_EXPIRED(7),
/**
*
* A job has been deleted and the SchedulingJob record does not exist.
*
*
* JOB_STATUS_DELETED = 8;
*/
JOB_STATUS_DELETED(8),
UNRECOGNIZED(-1),
;
/**
* JOB_STATUS_NONE = 0;
*/
public static final int JOB_STATUS_NONE_VALUE = 0;
/**
*
* A job has been successfully created.
*
*
* JOB_STATUS_CREATED = 1;
*/
public static final int JOB_STATUS_CREATED_VALUE = 1;
/**
*
* A job has a next run datetime and in standby mode.
*
*
* JOB_STATUS_READY = 2;
*/
public static final int JOB_STATUS_READY_VALUE = 2;
/**
*
* When a job is flagged with inflight, the job has been dispatched and in process.
*
*
* JOB_STATUS_INFLIGHT = 3;
*/
public static final int JOB_STATUS_INFLIGHT_VALUE = 3;
/**
*
* A job has been completed and waiting to be assigned the next run datetime.
*
*
* JOB_STATUS_SUCCEEDED = 4;
*/
public static final int JOB_STATUS_SUCCEEDED_VALUE = 4;
/**
*
* A job has been failed. The history logging is available for the details.
*
*
* JOB_STATUS_FAILED = 5;
*/
public static final int JOB_STATUS_FAILED_VALUE = 5;
/**
*
* A job has been paused until resumed datetime or when resumed.
*
*
* JOB_STATUS_PAUSED = 6;
*/
public static final int JOB_STATUS_PAUSED_VALUE = 6;
/**
*
* A job has been expired and ready to be deleted. Expired jobs will be deleted.
*
*
* JOB_STATUS_EXPIRED = 7;
*/
public static final int JOB_STATUS_EXPIRED_VALUE = 7;
/**
*
* A job has been deleted and the SchedulingJob record does not exist.
*
*
* JOB_STATUS_DELETED = 8;
*/
public static final int JOB_STATUS_DELETED_VALUE = 8;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static JobStatus valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static JobStatus forNumber(int value) {
switch (value) {
case 0: return JOB_STATUS_NONE;
case 1: return JOB_STATUS_CREATED;
case 2: return JOB_STATUS_READY;
case 3: return JOB_STATUS_INFLIGHT;
case 4: return JOB_STATUS_SUCCEEDED;
case 5: return JOB_STATUS_FAILED;
case 6: return JOB_STATUS_PAUSED;
case 7: return JOB_STATUS_EXPIRED;
case 8: return JOB_STATUS_DELETED;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
JobStatus> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public JobStatus findValueByNumber(int number) {
return JobStatus.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return com.passkit.grpc.ct.Scheduler.getDescriptor().getEnumTypes().get(0);
}
private static final JobStatus[] VALUES = values();
public static JobStatus valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private JobStatus(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:ct.JobStatus)
}
public interface SchedulingJobOrBuilder extends
// @@protoc_insertion_point(interface_extends:ct.SchedulingJob)
com.google.protobuf.MessageOrBuilder {
/**
*
* Auto generated 22 char identifier. Read only.
*
*
* string id = 1;
* @return The id.
*/
java.lang.String getId();
/**
*
* Auto generated 22 char identifier. Read only.
*
*
* string id = 1;
* @return The bytes for id.
*/
com.google.protobuf.ByteString
getIdBytes();
/**
*
* HttpMethod and Endpoint with comma separation.
* Eg. POST,https://api.pub1.passkit.io/members/count/05QFES9HNRMZb1gwcsepww
*
*
* string jobFunction = 2;
* @return The jobFunction.
*/
java.lang.String getJobFunction();
/**
*
* HttpMethod and Endpoint with comma separation.
* Eg. POST,https://api.pub1.passkit.io/members/count/05QFES9HNRMZb1gwcsepww
*
*
* string jobFunction = 2;
* @return The bytes for jobFunction.
*/
com.google.protobuf.ByteString
getJobFunctionBytes();
/**
*
* Json string of the job function payload.
*
*
* string jobPayload = 3;
* @return The jobPayload.
*/
java.lang.String getJobPayload();
/**
*
* Json string of the job function payload.
*
*
* string jobPayload = 3;
* @return The bytes for jobPayload.
*/
com.google.protobuf.ByteString
getJobPayloadBytes();
/**
*
* Job name.
*
*
* string name = 4;
* @return The name.
*/
java.lang.String getName();
/**
*
* Job name.
*
*
* string name = 4;
* @return The bytes for name.
*/
com.google.protobuf.ByteString
getNameBytes();
/**
*
* Job description.
*
*
* string description = 5;
* @return The description.
*/
java.lang.String getDescription();
/**
*
* Job description.
*
*
* string description = 5;
* @return The bytes for description.
*/
com.google.protobuf.ByteString
getDescriptionBytes();
/**
*
* Job schedule. You can set recursive or one-off job.
*
*
* .ct.Schedule schedule = 6;
* @return Whether the schedule field is set.
*/
boolean hasSchedule();
/**
*
* Job schedule. You can set recursive or one-off job.
*
*
* .ct.Schedule schedule = 6;
* @return The schedule.
*/
com.passkit.grpc.ct.Scheduler.Schedule getSchedule();
/**
*
* Job schedule. You can set recursive or one-off job.
*
*
* .ct.Schedule schedule = 6;
*/
com.passkit.grpc.ct.Scheduler.ScheduleOrBuilder getScheduleOrBuilder();
/**
*
* Job status. Writable values are JOB_STATUS_READY and JOB_STATUS_PAUSED only. Default is JOB_STATUS_READY.
* Setting status=JOB_STATUS_PAUSED sets nextRunDatetime=null only when Schedule.StartsAt is not set.
* Status will be always JOB_STATUS_READY, if you set Schedule.StartsAt.
*
*
* .ct.JobStatus status = 7;
* @return The enum numeric value on the wire for status.
*/
int getStatusValue();
/**
*
* Job status. Writable values are JOB_STATUS_READY and JOB_STATUS_PAUSED only. Default is JOB_STATUS_READY.
* Setting status=JOB_STATUS_PAUSED sets nextRunDatetime=null only when Schedule.StartsAt is not set.
* Status will be always JOB_STATUS_READY, if you set Schedule.StartsAt.
*
*
* .ct.JobStatus status = 7;
* @return The status.
*/
com.passkit.grpc.ct.Scheduler.JobStatus getStatus();
/**
*
* Next scheduled run datetime. Read only.
*
*
* .google.protobuf.Timestamp nextRunDatetime = 8;
* @return Whether the nextRunDatetime field is set.
*/
boolean hasNextRunDatetime();
/**
*
* Next scheduled run datetime. Read only.
*
*
* .google.protobuf.Timestamp nextRunDatetime = 8;
* @return The nextRunDatetime.
*/
com.google.protobuf.Timestamp getNextRunDatetime();
/**
*
* Next scheduled run datetime. Read only.
*
*
* .google.protobuf.Timestamp nextRunDatetime = 8;
*/
com.google.protobuf.TimestampOrBuilder getNextRunDatetimeOrBuilder();
/**
*
* The datetime when the job was executed last time. Read only.
*
*
* .google.protobuf.Timestamp lastRunDatetime = 9;
* @return Whether the lastRunDatetime field is set.
*/
boolean hasLastRunDatetime();
/**
*
* The datetime when the job was executed last time. Read only.
*
*
* .google.protobuf.Timestamp lastRunDatetime = 9;
* @return The lastRunDatetime.
*/
com.google.protobuf.Timestamp getLastRunDatetime();
/**
*
* The datetime when the job was executed last time. Read only.
*
*
* .google.protobuf.Timestamp lastRunDatetime = 9;
*/
com.google.protobuf.TimestampOrBuilder getLastRunDatetimeOrBuilder();
/**
*
* 5 latest logs of executed jobs. Read only.
*
*
* repeated .ct.JobHistory logs = 10;
*/
java.util.List
getLogsList();
/**
*
* 5 latest logs of executed jobs. Read only.
*
*
* repeated .ct.JobHistory logs = 10;
*/
com.passkit.grpc.ct.Scheduler.JobHistory getLogs(int index);
/**
*
* 5 latest logs of executed jobs. Read only.
*
*
* repeated .ct.JobHistory logs = 10;
*/
int getLogsCount();
/**
*
* 5 latest logs of executed jobs. Read only.
*
*
* repeated .ct.JobHistory logs = 10;
*/
java.util.List extends com.passkit.grpc.ct.Scheduler.JobHistoryOrBuilder>
getLogsOrBuilderList();
/**
*
* 5 latest logs of executed jobs. Read only.
*
*
* repeated .ct.JobHistory logs = 10;
*/
com.passkit.grpc.ct.Scheduler.JobHistoryOrBuilder getLogsOrBuilder(
int index);
/**
*
* Datetime the job was created. Read only.
*
*
* .google.protobuf.Timestamp created = 11;
* @return Whether the created field is set.
*/
boolean hasCreated();
/**
*
* Datetime the job was created. Read only.
*
*
* .google.protobuf.Timestamp created = 11;
* @return The created.
*/
com.google.protobuf.Timestamp getCreated();
/**
*
* Datetime the job was created. Read only.
*
*
* .google.protobuf.Timestamp created = 11;
*/
com.google.protobuf.TimestampOrBuilder getCreatedOrBuilder();
/**
*
* Datetime the job was last updated. Read only.
*
*
* .google.protobuf.Timestamp updated = 12;
* @return Whether the updated field is set.
*/
boolean hasUpdated();
/**
*
* Datetime the job was last updated. Read only.
*
*
* .google.protobuf.Timestamp updated = 12;
* @return The updated.
*/
com.google.protobuf.Timestamp getUpdated();
/**
*
* Datetime the job was last updated. Read only.
*
*
* .google.protobuf.Timestamp updated = 12;
*/
com.google.protobuf.TimestampOrBuilder getUpdatedOrBuilder();
/**
*
* Datetime when the job will for the last time and expires. Read only. Expiry setting is available in the Schedule field.
*
*
* .google.protobuf.Timestamp expiry = 13;
* @return Whether the expiry field is set.
*/
boolean hasExpiry();
/**
*
* Datetime when the job will for the last time and expires. Read only. Expiry setting is available in the Schedule field.
*
*
* .google.protobuf.Timestamp expiry = 13;
* @return The expiry.
*/
com.google.protobuf.Timestamp getExpiry();
/**
*
* Datetime when the job will for the last time and expires. Read only. Expiry setting is available in the Schedule field.
*
*
* .google.protobuf.Timestamp expiry = 13;
*/
com.google.protobuf.TimestampOrBuilder getExpiryOrBuilder();
}
/**
* Protobuf type {@code ct.SchedulingJob}
*/
public static final class SchedulingJob extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:ct.SchedulingJob)
SchedulingJobOrBuilder {
private static final long serialVersionUID = 0L;
// Use SchedulingJob.newBuilder() to construct.
private SchedulingJob(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private SchedulingJob() {
id_ = "";
jobFunction_ = "";
jobPayload_ = "";
name_ = "";
description_ = "";
status_ = 0;
logs_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new SchedulingJob();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private SchedulingJob(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
id_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
jobFunction_ = s;
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
jobPayload_ = s;
break;
}
case 34: {
java.lang.String s = input.readStringRequireUtf8();
name_ = s;
break;
}
case 42: {
java.lang.String s = input.readStringRequireUtf8();
description_ = s;
break;
}
case 50: {
com.passkit.grpc.ct.Scheduler.Schedule.Builder subBuilder = null;
if (schedule_ != null) {
subBuilder = schedule_.toBuilder();
}
schedule_ = input.readMessage(com.passkit.grpc.ct.Scheduler.Schedule.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(schedule_);
schedule_ = subBuilder.buildPartial();
}
break;
}
case 56: {
int rawValue = input.readEnum();
status_ = rawValue;
break;
}
case 66: {
com.google.protobuf.Timestamp.Builder subBuilder = null;
if (nextRunDatetime_ != null) {
subBuilder = nextRunDatetime_.toBuilder();
}
nextRunDatetime_ = input.readMessage(com.google.protobuf.Timestamp.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(nextRunDatetime_);
nextRunDatetime_ = subBuilder.buildPartial();
}
break;
}
case 74: {
com.google.protobuf.Timestamp.Builder subBuilder = null;
if (lastRunDatetime_ != null) {
subBuilder = lastRunDatetime_.toBuilder();
}
lastRunDatetime_ = input.readMessage(com.google.protobuf.Timestamp.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(lastRunDatetime_);
lastRunDatetime_ = subBuilder.buildPartial();
}
break;
}
case 82: {
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
logs_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
logs_.add(
input.readMessage(com.passkit.grpc.ct.Scheduler.JobHistory.parser(), extensionRegistry));
break;
}
case 90: {
com.google.protobuf.Timestamp.Builder subBuilder = null;
if (created_ != null) {
subBuilder = created_.toBuilder();
}
created_ = input.readMessage(com.google.protobuf.Timestamp.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(created_);
created_ = subBuilder.buildPartial();
}
break;
}
case 98: {
com.google.protobuf.Timestamp.Builder subBuilder = null;
if (updated_ != null) {
subBuilder = updated_.toBuilder();
}
updated_ = input.readMessage(com.google.protobuf.Timestamp.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(updated_);
updated_ = subBuilder.buildPartial();
}
break;
}
case 106: {
com.google.protobuf.Timestamp.Builder subBuilder = null;
if (expiry_ != null) {
subBuilder = expiry_.toBuilder();
}
expiry_ = input.readMessage(com.google.protobuf.Timestamp.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(expiry_);
expiry_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
logs_ = java.util.Collections.unmodifiableList(logs_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.passkit.grpc.ct.Scheduler.internal_static_ct_SchedulingJob_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.passkit.grpc.ct.Scheduler.internal_static_ct_SchedulingJob_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.passkit.grpc.ct.Scheduler.SchedulingJob.class, com.passkit.grpc.ct.Scheduler.SchedulingJob.Builder.class);
}
public static final int ID_FIELD_NUMBER = 1;
private volatile java.lang.Object id_;
/**
*
* Auto generated 22 char identifier. Read only.
*
*
* string id = 1;
* @return The id.
*/
@java.lang.Override
public java.lang.String getId() {
java.lang.Object ref = id_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
id_ = s;
return s;
}
}
/**
*
* Auto generated 22 char identifier. Read only.
*
*
* string id = 1;
* @return The bytes for id.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getIdBytes() {
java.lang.Object ref = id_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
id_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int JOBFUNCTION_FIELD_NUMBER = 2;
private volatile java.lang.Object jobFunction_;
/**
*
* HttpMethod and Endpoint with comma separation.
* Eg. POST,https://api.pub1.passkit.io/members/count/05QFES9HNRMZb1gwcsepww
*
*
* string jobFunction = 2;
* @return The jobFunction.
*/
@java.lang.Override
public java.lang.String getJobFunction() {
java.lang.Object ref = jobFunction_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
jobFunction_ = s;
return s;
}
}
/**
*
* HttpMethod and Endpoint with comma separation.
* Eg. POST,https://api.pub1.passkit.io/members/count/05QFES9HNRMZb1gwcsepww
*
*
* string jobFunction = 2;
* @return The bytes for jobFunction.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getJobFunctionBytes() {
java.lang.Object ref = jobFunction_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
jobFunction_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int JOBPAYLOAD_FIELD_NUMBER = 3;
private volatile java.lang.Object jobPayload_;
/**
*
* Json string of the job function payload.
*
*
* string jobPayload = 3;
* @return The jobPayload.
*/
@java.lang.Override
public java.lang.String getJobPayload() {
java.lang.Object ref = jobPayload_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
jobPayload_ = s;
return s;
}
}
/**
*
* Json string of the job function payload.
*
*
* string jobPayload = 3;
* @return The bytes for jobPayload.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getJobPayloadBytes() {
java.lang.Object ref = jobPayload_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
jobPayload_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int NAME_FIELD_NUMBER = 4;
private volatile java.lang.Object name_;
/**
*
* Job name.
*
*
* string name = 4;
* @return The name.
*/
@java.lang.Override
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
}
}
/**
*
* Job name.
*
*
* string name = 4;
* @return The bytes for name.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int DESCRIPTION_FIELD_NUMBER = 5;
private volatile java.lang.Object description_;
/**
*
* Job description.
*
*
* string description = 5;
* @return The description.
*/
@java.lang.Override
public java.lang.String getDescription() {
java.lang.Object ref = description_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
description_ = s;
return s;
}
}
/**
*
* Job description.
*
*
* string description = 5;
* @return The bytes for description.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getDescriptionBytes() {
java.lang.Object ref = description_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
description_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SCHEDULE_FIELD_NUMBER = 6;
private com.passkit.grpc.ct.Scheduler.Schedule schedule_;
/**
*
* Job schedule. You can set recursive or one-off job.
*
*
* .ct.Schedule schedule = 6;
* @return Whether the schedule field is set.
*/
@java.lang.Override
public boolean hasSchedule() {
return schedule_ != null;
}
/**
*
* Job schedule. You can set recursive or one-off job.
*
*
* .ct.Schedule schedule = 6;
* @return The schedule.
*/
@java.lang.Override
public com.passkit.grpc.ct.Scheduler.Schedule getSchedule() {
return schedule_ == null ? com.passkit.grpc.ct.Scheduler.Schedule.getDefaultInstance() : schedule_;
}
/**
*
* Job schedule. You can set recursive or one-off job.
*
*
* .ct.Schedule schedule = 6;
*/
@java.lang.Override
public com.passkit.grpc.ct.Scheduler.ScheduleOrBuilder getScheduleOrBuilder() {
return getSchedule();
}
public static final int STATUS_FIELD_NUMBER = 7;
private int status_;
/**
*
* Job status. Writable values are JOB_STATUS_READY and JOB_STATUS_PAUSED only. Default is JOB_STATUS_READY.
* Setting status=JOB_STATUS_PAUSED sets nextRunDatetime=null only when Schedule.StartsAt is not set.
* Status will be always JOB_STATUS_READY, if you set Schedule.StartsAt.
*
*
* .ct.JobStatus status = 7;
* @return The enum numeric value on the wire for status.
*/
@java.lang.Override public int getStatusValue() {
return status_;
}
/**
*
* Job status. Writable values are JOB_STATUS_READY and JOB_STATUS_PAUSED only. Default is JOB_STATUS_READY.
* Setting status=JOB_STATUS_PAUSED sets nextRunDatetime=null only when Schedule.StartsAt is not set.
* Status will be always JOB_STATUS_READY, if you set Schedule.StartsAt.
*
*
* .ct.JobStatus status = 7;
* @return The status.
*/
@java.lang.Override public com.passkit.grpc.ct.Scheduler.JobStatus getStatus() {
@SuppressWarnings("deprecation")
com.passkit.grpc.ct.Scheduler.JobStatus result = com.passkit.grpc.ct.Scheduler.JobStatus.valueOf(status_);
return result == null ? com.passkit.grpc.ct.Scheduler.JobStatus.UNRECOGNIZED : result;
}
public static final int NEXTRUNDATETIME_FIELD_NUMBER = 8;
private com.google.protobuf.Timestamp nextRunDatetime_;
/**
*
* Next scheduled run datetime. Read only.
*
*
* .google.protobuf.Timestamp nextRunDatetime = 8;
* @return Whether the nextRunDatetime field is set.
*/
@java.lang.Override
public boolean hasNextRunDatetime() {
return nextRunDatetime_ != null;
}
/**
*
* Next scheduled run datetime. Read only.
*
*
* .google.protobuf.Timestamp nextRunDatetime = 8;
* @return The nextRunDatetime.
*/
@java.lang.Override
public com.google.protobuf.Timestamp getNextRunDatetime() {
return nextRunDatetime_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : nextRunDatetime_;
}
/**
*
* Next scheduled run datetime. Read only.
*
*
* .google.protobuf.Timestamp nextRunDatetime = 8;
*/
@java.lang.Override
public com.google.protobuf.TimestampOrBuilder getNextRunDatetimeOrBuilder() {
return getNextRunDatetime();
}
public static final int LASTRUNDATETIME_FIELD_NUMBER = 9;
private com.google.protobuf.Timestamp lastRunDatetime_;
/**
*
* The datetime when the job was executed last time. Read only.
*
*
* .google.protobuf.Timestamp lastRunDatetime = 9;
* @return Whether the lastRunDatetime field is set.
*/
@java.lang.Override
public boolean hasLastRunDatetime() {
return lastRunDatetime_ != null;
}
/**
*
* The datetime when the job was executed last time. Read only.
*
*
* .google.protobuf.Timestamp lastRunDatetime = 9;
* @return The lastRunDatetime.
*/
@java.lang.Override
public com.google.protobuf.Timestamp getLastRunDatetime() {
return lastRunDatetime_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : lastRunDatetime_;
}
/**
*
* The datetime when the job was executed last time. Read only.
*
*
* .google.protobuf.Timestamp lastRunDatetime = 9;
*/
@java.lang.Override
public com.google.protobuf.TimestampOrBuilder getLastRunDatetimeOrBuilder() {
return getLastRunDatetime();
}
public static final int LOGS_FIELD_NUMBER = 10;
private java.util.List logs_;
/**
*
* 5 latest logs of executed jobs. Read only.
*
*
* repeated .ct.JobHistory logs = 10;
*/
@java.lang.Override
public java.util.List getLogsList() {
return logs_;
}
/**
*
* 5 latest logs of executed jobs. Read only.
*
*
* repeated .ct.JobHistory logs = 10;
*/
@java.lang.Override
public java.util.List extends com.passkit.grpc.ct.Scheduler.JobHistoryOrBuilder>
getLogsOrBuilderList() {
return logs_;
}
/**
*
* 5 latest logs of executed jobs. Read only.
*
*
* repeated .ct.JobHistory logs = 10;
*/
@java.lang.Override
public int getLogsCount() {
return logs_.size();
}
/**
*
* 5 latest logs of executed jobs. Read only.
*
*
* repeated .ct.JobHistory logs = 10;
*/
@java.lang.Override
public com.passkit.grpc.ct.Scheduler.JobHistory getLogs(int index) {
return logs_.get(index);
}
/**
*
* 5 latest logs of executed jobs. Read only.
*
*
* repeated .ct.JobHistory logs = 10;
*/
@java.lang.Override
public com.passkit.grpc.ct.Scheduler.JobHistoryOrBuilder getLogsOrBuilder(
int index) {
return logs_.get(index);
}
public static final int CREATED_FIELD_NUMBER = 11;
private com.google.protobuf.Timestamp created_;
/**
*
* Datetime the job was created. Read only.
*
*
* .google.protobuf.Timestamp created = 11;
* @return Whether the created field is set.
*/
@java.lang.Override
public boolean hasCreated() {
return created_ != null;
}
/**
*
* Datetime the job was created. Read only.
*
*
* .google.protobuf.Timestamp created = 11;
* @return The created.
*/
@java.lang.Override
public com.google.protobuf.Timestamp getCreated() {
return created_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : created_;
}
/**
*
* Datetime the job was created. Read only.
*
*
* .google.protobuf.Timestamp created = 11;
*/
@java.lang.Override
public com.google.protobuf.TimestampOrBuilder getCreatedOrBuilder() {
return getCreated();
}
public static final int UPDATED_FIELD_NUMBER = 12;
private com.google.protobuf.Timestamp updated_;
/**
*
* Datetime the job was last updated. Read only.
*
*
* .google.protobuf.Timestamp updated = 12;
* @return Whether the updated field is set.
*/
@java.lang.Override
public boolean hasUpdated() {
return updated_ != null;
}
/**
*
* Datetime the job was last updated. Read only.
*
*
* .google.protobuf.Timestamp updated = 12;
* @return The updated.
*/
@java.lang.Override
public com.google.protobuf.Timestamp getUpdated() {
return updated_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : updated_;
}
/**
*
* Datetime the job was last updated. Read only.
*
*
* .google.protobuf.Timestamp updated = 12;
*/
@java.lang.Override
public com.google.protobuf.TimestampOrBuilder getUpdatedOrBuilder() {
return getUpdated();
}
public static final int EXPIRY_FIELD_NUMBER = 13;
private com.google.protobuf.Timestamp expiry_;
/**
*
* Datetime when the job will for the last time and expires. Read only. Expiry setting is available in the Schedule field.
*
*
* .google.protobuf.Timestamp expiry = 13;
* @return Whether the expiry field is set.
*/
@java.lang.Override
public boolean hasExpiry() {
return expiry_ != null;
}
/**
*
* Datetime when the job will for the last time and expires. Read only. Expiry setting is available in the Schedule field.
*
*
* .google.protobuf.Timestamp expiry = 13;
* @return The expiry.
*/
@java.lang.Override
public com.google.protobuf.Timestamp getExpiry() {
return expiry_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : expiry_;
}
/**
*
* Datetime when the job will for the last time and expires. Read only. Expiry setting is available in the Schedule field.
*
*
* .google.protobuf.Timestamp expiry = 13;
*/
@java.lang.Override
public com.google.protobuf.TimestampOrBuilder getExpiryOrBuilder() {
return getExpiry();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(id_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, id_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(jobFunction_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, jobFunction_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(jobPayload_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, jobPayload_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(name_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, name_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(description_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 5, description_);
}
if (schedule_ != null) {
output.writeMessage(6, getSchedule());
}
if (status_ != com.passkit.grpc.ct.Scheduler.JobStatus.JOB_STATUS_NONE.getNumber()) {
output.writeEnum(7, status_);
}
if (nextRunDatetime_ != null) {
output.writeMessage(8, getNextRunDatetime());
}
if (lastRunDatetime_ != null) {
output.writeMessage(9, getLastRunDatetime());
}
for (int i = 0; i < logs_.size(); i++) {
output.writeMessage(10, logs_.get(i));
}
if (created_ != null) {
output.writeMessage(11, getCreated());
}
if (updated_ != null) {
output.writeMessage(12, getUpdated());
}
if (expiry_ != null) {
output.writeMessage(13, getExpiry());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(id_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, id_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(jobFunction_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, jobFunction_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(jobPayload_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, jobPayload_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(name_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(4, name_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(description_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(5, description_);
}
if (schedule_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(6, getSchedule());
}
if (status_ != com.passkit.grpc.ct.Scheduler.JobStatus.JOB_STATUS_NONE.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(7, status_);
}
if (nextRunDatetime_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(8, getNextRunDatetime());
}
if (lastRunDatetime_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(9, getLastRunDatetime());
}
for (int i = 0; i < logs_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(10, logs_.get(i));
}
if (created_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(11, getCreated());
}
if (updated_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(12, getUpdated());
}
if (expiry_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(13, getExpiry());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.passkit.grpc.ct.Scheduler.SchedulingJob)) {
return super.equals(obj);
}
com.passkit.grpc.ct.Scheduler.SchedulingJob other = (com.passkit.grpc.ct.Scheduler.SchedulingJob) obj;
if (!getId()
.equals(other.getId())) return false;
if (!getJobFunction()
.equals(other.getJobFunction())) return false;
if (!getJobPayload()
.equals(other.getJobPayload())) return false;
if (!getName()
.equals(other.getName())) return false;
if (!getDescription()
.equals(other.getDescription())) return false;
if (hasSchedule() != other.hasSchedule()) return false;
if (hasSchedule()) {
if (!getSchedule()
.equals(other.getSchedule())) return false;
}
if (status_ != other.status_) return false;
if (hasNextRunDatetime() != other.hasNextRunDatetime()) return false;
if (hasNextRunDatetime()) {
if (!getNextRunDatetime()
.equals(other.getNextRunDatetime())) return false;
}
if (hasLastRunDatetime() != other.hasLastRunDatetime()) return false;
if (hasLastRunDatetime()) {
if (!getLastRunDatetime()
.equals(other.getLastRunDatetime())) return false;
}
if (!getLogsList()
.equals(other.getLogsList())) return false;
if (hasCreated() != other.hasCreated()) return false;
if (hasCreated()) {
if (!getCreated()
.equals(other.getCreated())) return false;
}
if (hasUpdated() != other.hasUpdated()) return false;
if (hasUpdated()) {
if (!getUpdated()
.equals(other.getUpdated())) return false;
}
if (hasExpiry() != other.hasExpiry()) return false;
if (hasExpiry()) {
if (!getExpiry()
.equals(other.getExpiry())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + ID_FIELD_NUMBER;
hash = (53 * hash) + getId().hashCode();
hash = (37 * hash) + JOBFUNCTION_FIELD_NUMBER;
hash = (53 * hash) + getJobFunction().hashCode();
hash = (37 * hash) + JOBPAYLOAD_FIELD_NUMBER;
hash = (53 * hash) + getJobPayload().hashCode();
hash = (37 * hash) + NAME_FIELD_NUMBER;
hash = (53 * hash) + getName().hashCode();
hash = (37 * hash) + DESCRIPTION_FIELD_NUMBER;
hash = (53 * hash) + getDescription().hashCode();
if (hasSchedule()) {
hash = (37 * hash) + SCHEDULE_FIELD_NUMBER;
hash = (53 * hash) + getSchedule().hashCode();
}
hash = (37 * hash) + STATUS_FIELD_NUMBER;
hash = (53 * hash) + status_;
if (hasNextRunDatetime()) {
hash = (37 * hash) + NEXTRUNDATETIME_FIELD_NUMBER;
hash = (53 * hash) + getNextRunDatetime().hashCode();
}
if (hasLastRunDatetime()) {
hash = (37 * hash) + LASTRUNDATETIME_FIELD_NUMBER;
hash = (53 * hash) + getLastRunDatetime().hashCode();
}
if (getLogsCount() > 0) {
hash = (37 * hash) + LOGS_FIELD_NUMBER;
hash = (53 * hash) + getLogsList().hashCode();
}
if (hasCreated()) {
hash = (37 * hash) + CREATED_FIELD_NUMBER;
hash = (53 * hash) + getCreated().hashCode();
}
if (hasUpdated()) {
hash = (37 * hash) + UPDATED_FIELD_NUMBER;
hash = (53 * hash) + getUpdated().hashCode();
}
if (hasExpiry()) {
hash = (37 * hash) + EXPIRY_FIELD_NUMBER;
hash = (53 * hash) + getExpiry().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.passkit.grpc.ct.Scheduler.SchedulingJob parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.passkit.grpc.ct.Scheduler.SchedulingJob parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.passkit.grpc.ct.Scheduler.SchedulingJob parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.passkit.grpc.ct.Scheduler.SchedulingJob parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.passkit.grpc.ct.Scheduler.SchedulingJob parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.passkit.grpc.ct.Scheduler.SchedulingJob parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.passkit.grpc.ct.Scheduler.SchedulingJob parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.passkit.grpc.ct.Scheduler.SchedulingJob parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.passkit.grpc.ct.Scheduler.SchedulingJob parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.passkit.grpc.ct.Scheduler.SchedulingJob parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.passkit.grpc.ct.Scheduler.SchedulingJob parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.passkit.grpc.ct.Scheduler.SchedulingJob parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.passkit.grpc.ct.Scheduler.SchedulingJob prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code ct.SchedulingJob}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:ct.SchedulingJob)
com.passkit.grpc.ct.Scheduler.SchedulingJobOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.passkit.grpc.ct.Scheduler.internal_static_ct_SchedulingJob_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.passkit.grpc.ct.Scheduler.internal_static_ct_SchedulingJob_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.passkit.grpc.ct.Scheduler.SchedulingJob.class, com.passkit.grpc.ct.Scheduler.SchedulingJob.Builder.class);
}
// Construct using com.passkit.grpc.ct.Scheduler.SchedulingJob.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getLogsFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
id_ = "";
jobFunction_ = "";
jobPayload_ = "";
name_ = "";
description_ = "";
if (scheduleBuilder_ == null) {
schedule_ = null;
} else {
schedule_ = null;
scheduleBuilder_ = null;
}
status_ = 0;
if (nextRunDatetimeBuilder_ == null) {
nextRunDatetime_ = null;
} else {
nextRunDatetime_ = null;
nextRunDatetimeBuilder_ = null;
}
if (lastRunDatetimeBuilder_ == null) {
lastRunDatetime_ = null;
} else {
lastRunDatetime_ = null;
lastRunDatetimeBuilder_ = null;
}
if (logsBuilder_ == null) {
logs_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
logsBuilder_.clear();
}
if (createdBuilder_ == null) {
created_ = null;
} else {
created_ = null;
createdBuilder_ = null;
}
if (updatedBuilder_ == null) {
updated_ = null;
} else {
updated_ = null;
updatedBuilder_ = null;
}
if (expiryBuilder_ == null) {
expiry_ = null;
} else {
expiry_ = null;
expiryBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.passkit.grpc.ct.Scheduler.internal_static_ct_SchedulingJob_descriptor;
}
@java.lang.Override
public com.passkit.grpc.ct.Scheduler.SchedulingJob getDefaultInstanceForType() {
return com.passkit.grpc.ct.Scheduler.SchedulingJob.getDefaultInstance();
}
@java.lang.Override
public com.passkit.grpc.ct.Scheduler.SchedulingJob build() {
com.passkit.grpc.ct.Scheduler.SchedulingJob result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.passkit.grpc.ct.Scheduler.SchedulingJob buildPartial() {
com.passkit.grpc.ct.Scheduler.SchedulingJob result = new com.passkit.grpc.ct.Scheduler.SchedulingJob(this);
int from_bitField0_ = bitField0_;
result.id_ = id_;
result.jobFunction_ = jobFunction_;
result.jobPayload_ = jobPayload_;
result.name_ = name_;
result.description_ = description_;
if (scheduleBuilder_ == null) {
result.schedule_ = schedule_;
} else {
result.schedule_ = scheduleBuilder_.build();
}
result.status_ = status_;
if (nextRunDatetimeBuilder_ == null) {
result.nextRunDatetime_ = nextRunDatetime_;
} else {
result.nextRunDatetime_ = nextRunDatetimeBuilder_.build();
}
if (lastRunDatetimeBuilder_ == null) {
result.lastRunDatetime_ = lastRunDatetime_;
} else {
result.lastRunDatetime_ = lastRunDatetimeBuilder_.build();
}
if (logsBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0)) {
logs_ = java.util.Collections.unmodifiableList(logs_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.logs_ = logs_;
} else {
result.logs_ = logsBuilder_.build();
}
if (createdBuilder_ == null) {
result.created_ = created_;
} else {
result.created_ = createdBuilder_.build();
}
if (updatedBuilder_ == null) {
result.updated_ = updated_;
} else {
result.updated_ = updatedBuilder_.build();
}
if (expiryBuilder_ == null) {
result.expiry_ = expiry_;
} else {
result.expiry_ = expiryBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.passkit.grpc.ct.Scheduler.SchedulingJob) {
return mergeFrom((com.passkit.grpc.ct.Scheduler.SchedulingJob)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.passkit.grpc.ct.Scheduler.SchedulingJob other) {
if (other == com.passkit.grpc.ct.Scheduler.SchedulingJob.getDefaultInstance()) return this;
if (!other.getId().isEmpty()) {
id_ = other.id_;
onChanged();
}
if (!other.getJobFunction().isEmpty()) {
jobFunction_ = other.jobFunction_;
onChanged();
}
if (!other.getJobPayload().isEmpty()) {
jobPayload_ = other.jobPayload_;
onChanged();
}
if (!other.getName().isEmpty()) {
name_ = other.name_;
onChanged();
}
if (!other.getDescription().isEmpty()) {
description_ = other.description_;
onChanged();
}
if (other.hasSchedule()) {
mergeSchedule(other.getSchedule());
}
if (other.status_ != 0) {
setStatusValue(other.getStatusValue());
}
if (other.hasNextRunDatetime()) {
mergeNextRunDatetime(other.getNextRunDatetime());
}
if (other.hasLastRunDatetime()) {
mergeLastRunDatetime(other.getLastRunDatetime());
}
if (logsBuilder_ == null) {
if (!other.logs_.isEmpty()) {
if (logs_.isEmpty()) {
logs_ = other.logs_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureLogsIsMutable();
logs_.addAll(other.logs_);
}
onChanged();
}
} else {
if (!other.logs_.isEmpty()) {
if (logsBuilder_.isEmpty()) {
logsBuilder_.dispose();
logsBuilder_ = null;
logs_ = other.logs_;
bitField0_ = (bitField0_ & ~0x00000001);
logsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getLogsFieldBuilder() : null;
} else {
logsBuilder_.addAllMessages(other.logs_);
}
}
}
if (other.hasCreated()) {
mergeCreated(other.getCreated());
}
if (other.hasUpdated()) {
mergeUpdated(other.getUpdated());
}
if (other.hasExpiry()) {
mergeExpiry(other.getExpiry());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.passkit.grpc.ct.Scheduler.SchedulingJob parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.passkit.grpc.ct.Scheduler.SchedulingJob) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object id_ = "";
/**
*
* Auto generated 22 char identifier. Read only.
*
*
* string id = 1;
* @return The id.
*/
public java.lang.String getId() {
java.lang.Object ref = id_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
id_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Auto generated 22 char identifier. Read only.
*
*
* string id = 1;
* @return The bytes for id.
*/
public com.google.protobuf.ByteString
getIdBytes() {
java.lang.Object ref = id_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
id_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Auto generated 22 char identifier. Read only.
*
*
* string id = 1;
* @param value The id to set.
* @return This builder for chaining.
*/
public Builder setId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
id_ = value;
onChanged();
return this;
}
/**
*
* Auto generated 22 char identifier. Read only.
*
*
* string id = 1;
* @return This builder for chaining.
*/
public Builder clearId() {
id_ = getDefaultInstance().getId();
onChanged();
return this;
}
/**
*
* Auto generated 22 char identifier. Read only.
*
*
* string id = 1;
* @param value The bytes for id to set.
* @return This builder for chaining.
*/
public Builder setIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
id_ = value;
onChanged();
return this;
}
private java.lang.Object jobFunction_ = "";
/**
*
* HttpMethod and Endpoint with comma separation.
* Eg. POST,https://api.pub1.passkit.io/members/count/05QFES9HNRMZb1gwcsepww
*
*
* string jobFunction = 2;
* @return The jobFunction.
*/
public java.lang.String getJobFunction() {
java.lang.Object ref = jobFunction_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
jobFunction_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* HttpMethod and Endpoint with comma separation.
* Eg. POST,https://api.pub1.passkit.io/members/count/05QFES9HNRMZb1gwcsepww
*
*
* string jobFunction = 2;
* @return The bytes for jobFunction.
*/
public com.google.protobuf.ByteString
getJobFunctionBytes() {
java.lang.Object ref = jobFunction_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
jobFunction_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* HttpMethod and Endpoint with comma separation.
* Eg. POST,https://api.pub1.passkit.io/members/count/05QFES9HNRMZb1gwcsepww
*
*
* string jobFunction = 2;
* @param value The jobFunction to set.
* @return This builder for chaining.
*/
public Builder setJobFunction(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
jobFunction_ = value;
onChanged();
return this;
}
/**
*
* HttpMethod and Endpoint with comma separation.
* Eg. POST,https://api.pub1.passkit.io/members/count/05QFES9HNRMZb1gwcsepww
*
*
* string jobFunction = 2;
* @return This builder for chaining.
*/
public Builder clearJobFunction() {
jobFunction_ = getDefaultInstance().getJobFunction();
onChanged();
return this;
}
/**
*
* HttpMethod and Endpoint with comma separation.
* Eg. POST,https://api.pub1.passkit.io/members/count/05QFES9HNRMZb1gwcsepww
*
*
* string jobFunction = 2;
* @param value The bytes for jobFunction to set.
* @return This builder for chaining.
*/
public Builder setJobFunctionBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
jobFunction_ = value;
onChanged();
return this;
}
private java.lang.Object jobPayload_ = "";
/**
*
* Json string of the job function payload.
*
*
* string jobPayload = 3;
* @return The jobPayload.
*/
public java.lang.String getJobPayload() {
java.lang.Object ref = jobPayload_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
jobPayload_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Json string of the job function payload.
*
*
* string jobPayload = 3;
* @return The bytes for jobPayload.
*/
public com.google.protobuf.ByteString
getJobPayloadBytes() {
java.lang.Object ref = jobPayload_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
jobPayload_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Json string of the job function payload.
*
*
* string jobPayload = 3;
* @param value The jobPayload to set.
* @return This builder for chaining.
*/
public Builder setJobPayload(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
jobPayload_ = value;
onChanged();
return this;
}
/**
*
* Json string of the job function payload.
*
*
* string jobPayload = 3;
* @return This builder for chaining.
*/
public Builder clearJobPayload() {
jobPayload_ = getDefaultInstance().getJobPayload();
onChanged();
return this;
}
/**
*
* Json string of the job function payload.
*
*
* string jobPayload = 3;
* @param value The bytes for jobPayload to set.
* @return This builder for chaining.
*/
public Builder setJobPayloadBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
jobPayload_ = value;
onChanged();
return this;
}
private java.lang.Object name_ = "";
/**
*
* Job name.
*
*
* string name = 4;
* @return The name.
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Job name.
*
*
* string name = 4;
* @return The bytes for name.
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Job name.
*
*
* string name = 4;
* @param value The name to set.
* @return This builder for chaining.
*/
public Builder setName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
name_ = value;
onChanged();
return this;
}
/**
*
* Job name.
*
*
* string name = 4;
* @return This builder for chaining.
*/
public Builder clearName() {
name_ = getDefaultInstance().getName();
onChanged();
return this;
}
/**
*
* Job name.
*
*
* string name = 4;
* @param value The bytes for name to set.
* @return This builder for chaining.
*/
public Builder setNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
name_ = value;
onChanged();
return this;
}
private java.lang.Object description_ = "";
/**
*
* Job description.
*
*
* string description = 5;
* @return The description.
*/
public java.lang.String getDescription() {
java.lang.Object ref = description_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
description_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Job description.
*
*
* string description = 5;
* @return The bytes for description.
*/
public com.google.protobuf.ByteString
getDescriptionBytes() {
java.lang.Object ref = description_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
description_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Job description.
*
*
* string description = 5;
* @param value The description to set.
* @return This builder for chaining.
*/
public Builder setDescription(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
description_ = value;
onChanged();
return this;
}
/**
*
* Job description.
*
*
* string description = 5;
* @return This builder for chaining.
*/
public Builder clearDescription() {
description_ = getDefaultInstance().getDescription();
onChanged();
return this;
}
/**
*
* Job description.
*
*
* string description = 5;
* @param value The bytes for description to set.
* @return This builder for chaining.
*/
public Builder setDescriptionBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
description_ = value;
onChanged();
return this;
}
private com.passkit.grpc.ct.Scheduler.Schedule schedule_;
private com.google.protobuf.SingleFieldBuilderV3<
com.passkit.grpc.ct.Scheduler.Schedule, com.passkit.grpc.ct.Scheduler.Schedule.Builder, com.passkit.grpc.ct.Scheduler.ScheduleOrBuilder> scheduleBuilder_;
/**
*
* Job schedule. You can set recursive or one-off job.
*
*
* .ct.Schedule schedule = 6;
* @return Whether the schedule field is set.
*/
public boolean hasSchedule() {
return scheduleBuilder_ != null || schedule_ != null;
}
/**
*
* Job schedule. You can set recursive or one-off job.
*
*
* .ct.Schedule schedule = 6;
* @return The schedule.
*/
public com.passkit.grpc.ct.Scheduler.Schedule getSchedule() {
if (scheduleBuilder_ == null) {
return schedule_ == null ? com.passkit.grpc.ct.Scheduler.Schedule.getDefaultInstance() : schedule_;
} else {
return scheduleBuilder_.getMessage();
}
}
/**
*
* Job schedule. You can set recursive or one-off job.
*
*
* .ct.Schedule schedule = 6;
*/
public Builder setSchedule(com.passkit.grpc.ct.Scheduler.Schedule value) {
if (scheduleBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
schedule_ = value;
onChanged();
} else {
scheduleBuilder_.setMessage(value);
}
return this;
}
/**
*
* Job schedule. You can set recursive or one-off job.
*
*
* .ct.Schedule schedule = 6;
*/
public Builder setSchedule(
com.passkit.grpc.ct.Scheduler.Schedule.Builder builderForValue) {
if (scheduleBuilder_ == null) {
schedule_ = builderForValue.build();
onChanged();
} else {
scheduleBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Job schedule. You can set recursive or one-off job.
*
*
* .ct.Schedule schedule = 6;
*/
public Builder mergeSchedule(com.passkit.grpc.ct.Scheduler.Schedule value) {
if (scheduleBuilder_ == null) {
if (schedule_ != null) {
schedule_ =
com.passkit.grpc.ct.Scheduler.Schedule.newBuilder(schedule_).mergeFrom(value).buildPartial();
} else {
schedule_ = value;
}
onChanged();
} else {
scheduleBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Job schedule. You can set recursive or one-off job.
*
*
* .ct.Schedule schedule = 6;
*/
public Builder clearSchedule() {
if (scheduleBuilder_ == null) {
schedule_ = null;
onChanged();
} else {
schedule_ = null;
scheduleBuilder_ = null;
}
return this;
}
/**
*
* Job schedule. You can set recursive or one-off job.
*
*
* .ct.Schedule schedule = 6;
*/
public com.passkit.grpc.ct.Scheduler.Schedule.Builder getScheduleBuilder() {
onChanged();
return getScheduleFieldBuilder().getBuilder();
}
/**
*
* Job schedule. You can set recursive or one-off job.
*
*
* .ct.Schedule schedule = 6;
*/
public com.passkit.grpc.ct.Scheduler.ScheduleOrBuilder getScheduleOrBuilder() {
if (scheduleBuilder_ != null) {
return scheduleBuilder_.getMessageOrBuilder();
} else {
return schedule_ == null ?
com.passkit.grpc.ct.Scheduler.Schedule.getDefaultInstance() : schedule_;
}
}
/**
*
* Job schedule. You can set recursive or one-off job.
*
*
* .ct.Schedule schedule = 6;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.passkit.grpc.ct.Scheduler.Schedule, com.passkit.grpc.ct.Scheduler.Schedule.Builder, com.passkit.grpc.ct.Scheduler.ScheduleOrBuilder>
getScheduleFieldBuilder() {
if (scheduleBuilder_ == null) {
scheduleBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.passkit.grpc.ct.Scheduler.Schedule, com.passkit.grpc.ct.Scheduler.Schedule.Builder, com.passkit.grpc.ct.Scheduler.ScheduleOrBuilder>(
getSchedule(),
getParentForChildren(),
isClean());
schedule_ = null;
}
return scheduleBuilder_;
}
private int status_ = 0;
/**
*
* Job status. Writable values are JOB_STATUS_READY and JOB_STATUS_PAUSED only. Default is JOB_STATUS_READY.
* Setting status=JOB_STATUS_PAUSED sets nextRunDatetime=null only when Schedule.StartsAt is not set.
* Status will be always JOB_STATUS_READY, if you set Schedule.StartsAt.
*
*
* .ct.JobStatus status = 7;
* @return The enum numeric value on the wire for status.
*/
@java.lang.Override public int getStatusValue() {
return status_;
}
/**
*
* Job status. Writable values are JOB_STATUS_READY and JOB_STATUS_PAUSED only. Default is JOB_STATUS_READY.
* Setting status=JOB_STATUS_PAUSED sets nextRunDatetime=null only when Schedule.StartsAt is not set.
* Status will be always JOB_STATUS_READY, if you set Schedule.StartsAt.
*
*
* .ct.JobStatus status = 7;
* @param value The enum numeric value on the wire for status to set.
* @return This builder for chaining.
*/
public Builder setStatusValue(int value) {
status_ = value;
onChanged();
return this;
}
/**
*
* Job status. Writable values are JOB_STATUS_READY and JOB_STATUS_PAUSED only. Default is JOB_STATUS_READY.
* Setting status=JOB_STATUS_PAUSED sets nextRunDatetime=null only when Schedule.StartsAt is not set.
* Status will be always JOB_STATUS_READY, if you set Schedule.StartsAt.
*
*
* .ct.JobStatus status = 7;
* @return The status.
*/
@java.lang.Override
public com.passkit.grpc.ct.Scheduler.JobStatus getStatus() {
@SuppressWarnings("deprecation")
com.passkit.grpc.ct.Scheduler.JobStatus result = com.passkit.grpc.ct.Scheduler.JobStatus.valueOf(status_);
return result == null ? com.passkit.grpc.ct.Scheduler.JobStatus.UNRECOGNIZED : result;
}
/**
*
* Job status. Writable values are JOB_STATUS_READY and JOB_STATUS_PAUSED only. Default is JOB_STATUS_READY.
* Setting status=JOB_STATUS_PAUSED sets nextRunDatetime=null only when Schedule.StartsAt is not set.
* Status will be always JOB_STATUS_READY, if you set Schedule.StartsAt.
*
*
* .ct.JobStatus status = 7;
* @param value The status to set.
* @return This builder for chaining.
*/
public Builder setStatus(com.passkit.grpc.ct.Scheduler.JobStatus value) {
if (value == null) {
throw new NullPointerException();
}
status_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Job status. Writable values are JOB_STATUS_READY and JOB_STATUS_PAUSED only. Default is JOB_STATUS_READY.
* Setting status=JOB_STATUS_PAUSED sets nextRunDatetime=null only when Schedule.StartsAt is not set.
* Status will be always JOB_STATUS_READY, if you set Schedule.StartsAt.
*
*
* .ct.JobStatus status = 7;
* @return This builder for chaining.
*/
public Builder clearStatus() {
status_ = 0;
onChanged();
return this;
}
private com.google.protobuf.Timestamp nextRunDatetime_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder> nextRunDatetimeBuilder_;
/**
*
* Next scheduled run datetime. Read only.
*
*
* .google.protobuf.Timestamp nextRunDatetime = 8;
* @return Whether the nextRunDatetime field is set.
*/
public boolean hasNextRunDatetime() {
return nextRunDatetimeBuilder_ != null || nextRunDatetime_ != null;
}
/**
*
* Next scheduled run datetime. Read only.
*
*
* .google.protobuf.Timestamp nextRunDatetime = 8;
* @return The nextRunDatetime.
*/
public com.google.protobuf.Timestamp getNextRunDatetime() {
if (nextRunDatetimeBuilder_ == null) {
return nextRunDatetime_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : nextRunDatetime_;
} else {
return nextRunDatetimeBuilder_.getMessage();
}
}
/**
*
* Next scheduled run datetime. Read only.
*
*
* .google.protobuf.Timestamp nextRunDatetime = 8;
*/
public Builder setNextRunDatetime(com.google.protobuf.Timestamp value) {
if (nextRunDatetimeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
nextRunDatetime_ = value;
onChanged();
} else {
nextRunDatetimeBuilder_.setMessage(value);
}
return this;
}
/**
*
* Next scheduled run datetime. Read only.
*
*
* .google.protobuf.Timestamp nextRunDatetime = 8;
*/
public Builder setNextRunDatetime(
com.google.protobuf.Timestamp.Builder builderForValue) {
if (nextRunDatetimeBuilder_ == null) {
nextRunDatetime_ = builderForValue.build();
onChanged();
} else {
nextRunDatetimeBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Next scheduled run datetime. Read only.
*
*
* .google.protobuf.Timestamp nextRunDatetime = 8;
*/
public Builder mergeNextRunDatetime(com.google.protobuf.Timestamp value) {
if (nextRunDatetimeBuilder_ == null) {
if (nextRunDatetime_ != null) {
nextRunDatetime_ =
com.google.protobuf.Timestamp.newBuilder(nextRunDatetime_).mergeFrom(value).buildPartial();
} else {
nextRunDatetime_ = value;
}
onChanged();
} else {
nextRunDatetimeBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Next scheduled run datetime. Read only.
*
*
* .google.protobuf.Timestamp nextRunDatetime = 8;
*/
public Builder clearNextRunDatetime() {
if (nextRunDatetimeBuilder_ == null) {
nextRunDatetime_ = null;
onChanged();
} else {
nextRunDatetime_ = null;
nextRunDatetimeBuilder_ = null;
}
return this;
}
/**
*
* Next scheduled run datetime. Read only.
*
*
* .google.protobuf.Timestamp nextRunDatetime = 8;
*/
public com.google.protobuf.Timestamp.Builder getNextRunDatetimeBuilder() {
onChanged();
return getNextRunDatetimeFieldBuilder().getBuilder();
}
/**
*
* Next scheduled run datetime. Read only.
*
*
* .google.protobuf.Timestamp nextRunDatetime = 8;
*/
public com.google.protobuf.TimestampOrBuilder getNextRunDatetimeOrBuilder() {
if (nextRunDatetimeBuilder_ != null) {
return nextRunDatetimeBuilder_.getMessageOrBuilder();
} else {
return nextRunDatetime_ == null ?
com.google.protobuf.Timestamp.getDefaultInstance() : nextRunDatetime_;
}
}
/**
*
* Next scheduled run datetime. Read only.
*
*
* .google.protobuf.Timestamp nextRunDatetime = 8;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder>
getNextRunDatetimeFieldBuilder() {
if (nextRunDatetimeBuilder_ == null) {
nextRunDatetimeBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder>(
getNextRunDatetime(),
getParentForChildren(),
isClean());
nextRunDatetime_ = null;
}
return nextRunDatetimeBuilder_;
}
private com.google.protobuf.Timestamp lastRunDatetime_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder> lastRunDatetimeBuilder_;
/**
*
* The datetime when the job was executed last time. Read only.
*
*
* .google.protobuf.Timestamp lastRunDatetime = 9;
* @return Whether the lastRunDatetime field is set.
*/
public boolean hasLastRunDatetime() {
return lastRunDatetimeBuilder_ != null || lastRunDatetime_ != null;
}
/**
*
* The datetime when the job was executed last time. Read only.
*
*
* .google.protobuf.Timestamp lastRunDatetime = 9;
* @return The lastRunDatetime.
*/
public com.google.protobuf.Timestamp getLastRunDatetime() {
if (lastRunDatetimeBuilder_ == null) {
return lastRunDatetime_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : lastRunDatetime_;
} else {
return lastRunDatetimeBuilder_.getMessage();
}
}
/**
*
* The datetime when the job was executed last time. Read only.
*
*
* .google.protobuf.Timestamp lastRunDatetime = 9;
*/
public Builder setLastRunDatetime(com.google.protobuf.Timestamp value) {
if (lastRunDatetimeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
lastRunDatetime_ = value;
onChanged();
} else {
lastRunDatetimeBuilder_.setMessage(value);
}
return this;
}
/**
*
* The datetime when the job was executed last time. Read only.
*
*
* .google.protobuf.Timestamp lastRunDatetime = 9;
*/
public Builder setLastRunDatetime(
com.google.protobuf.Timestamp.Builder builderForValue) {
if (lastRunDatetimeBuilder_ == null) {
lastRunDatetime_ = builderForValue.build();
onChanged();
} else {
lastRunDatetimeBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* The datetime when the job was executed last time. Read only.
*
*
* .google.protobuf.Timestamp lastRunDatetime = 9;
*/
public Builder mergeLastRunDatetime(com.google.protobuf.Timestamp value) {
if (lastRunDatetimeBuilder_ == null) {
if (lastRunDatetime_ != null) {
lastRunDatetime_ =
com.google.protobuf.Timestamp.newBuilder(lastRunDatetime_).mergeFrom(value).buildPartial();
} else {
lastRunDatetime_ = value;
}
onChanged();
} else {
lastRunDatetimeBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* The datetime when the job was executed last time. Read only.
*
*
* .google.protobuf.Timestamp lastRunDatetime = 9;
*/
public Builder clearLastRunDatetime() {
if (lastRunDatetimeBuilder_ == null) {
lastRunDatetime_ = null;
onChanged();
} else {
lastRunDatetime_ = null;
lastRunDatetimeBuilder_ = null;
}
return this;
}
/**
*
* The datetime when the job was executed last time. Read only.
*
*
* .google.protobuf.Timestamp lastRunDatetime = 9;
*/
public com.google.protobuf.Timestamp.Builder getLastRunDatetimeBuilder() {
onChanged();
return getLastRunDatetimeFieldBuilder().getBuilder();
}
/**
*
* The datetime when the job was executed last time. Read only.
*
*
* .google.protobuf.Timestamp lastRunDatetime = 9;
*/
public com.google.protobuf.TimestampOrBuilder getLastRunDatetimeOrBuilder() {
if (lastRunDatetimeBuilder_ != null) {
return lastRunDatetimeBuilder_.getMessageOrBuilder();
} else {
return lastRunDatetime_ == null ?
com.google.protobuf.Timestamp.getDefaultInstance() : lastRunDatetime_;
}
}
/**
*
* The datetime when the job was executed last time. Read only.
*
*
* .google.protobuf.Timestamp lastRunDatetime = 9;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder>
getLastRunDatetimeFieldBuilder() {
if (lastRunDatetimeBuilder_ == null) {
lastRunDatetimeBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder>(
getLastRunDatetime(),
getParentForChildren(),
isClean());
lastRunDatetime_ = null;
}
return lastRunDatetimeBuilder_;
}
private java.util.List logs_ =
java.util.Collections.emptyList();
private void ensureLogsIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
logs_ = new java.util.ArrayList(logs_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.passkit.grpc.ct.Scheduler.JobHistory, com.passkit.grpc.ct.Scheduler.JobHistory.Builder, com.passkit.grpc.ct.Scheduler.JobHistoryOrBuilder> logsBuilder_;
/**
*
* 5 latest logs of executed jobs. Read only.
*
*
* repeated .ct.JobHistory logs = 10;
*/
public java.util.List getLogsList() {
if (logsBuilder_ == null) {
return java.util.Collections.unmodifiableList(logs_);
} else {
return logsBuilder_.getMessageList();
}
}
/**
*
* 5 latest logs of executed jobs. Read only.
*
*
* repeated .ct.JobHistory logs = 10;
*/
public int getLogsCount() {
if (logsBuilder_ == null) {
return logs_.size();
} else {
return logsBuilder_.getCount();
}
}
/**
*
* 5 latest logs of executed jobs. Read only.
*
*
* repeated .ct.JobHistory logs = 10;
*/
public com.passkit.grpc.ct.Scheduler.JobHistory getLogs(int index) {
if (logsBuilder_ == null) {
return logs_.get(index);
} else {
return logsBuilder_.getMessage(index);
}
}
/**
*
* 5 latest logs of executed jobs. Read only.
*
*
* repeated .ct.JobHistory logs = 10;
*/
public Builder setLogs(
int index, com.passkit.grpc.ct.Scheduler.JobHistory value) {
if (logsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureLogsIsMutable();
logs_.set(index, value);
onChanged();
} else {
logsBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* 5 latest logs of executed jobs. Read only.
*
*
* repeated .ct.JobHistory logs = 10;
*/
public Builder setLogs(
int index, com.passkit.grpc.ct.Scheduler.JobHistory.Builder builderForValue) {
if (logsBuilder_ == null) {
ensureLogsIsMutable();
logs_.set(index, builderForValue.build());
onChanged();
} else {
logsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* 5 latest logs of executed jobs. Read only.
*
*
* repeated .ct.JobHistory logs = 10;
*/
public Builder addLogs(com.passkit.grpc.ct.Scheduler.JobHistory value) {
if (logsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureLogsIsMutable();
logs_.add(value);
onChanged();
} else {
logsBuilder_.addMessage(value);
}
return this;
}
/**
*
* 5 latest logs of executed jobs. Read only.
*
*
* repeated .ct.JobHistory logs = 10;
*/
public Builder addLogs(
int index, com.passkit.grpc.ct.Scheduler.JobHistory value) {
if (logsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureLogsIsMutable();
logs_.add(index, value);
onChanged();
} else {
logsBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* 5 latest logs of executed jobs. Read only.
*
*
* repeated .ct.JobHistory logs = 10;
*/
public Builder addLogs(
com.passkit.grpc.ct.Scheduler.JobHistory.Builder builderForValue) {
if (logsBuilder_ == null) {
ensureLogsIsMutable();
logs_.add(builderForValue.build());
onChanged();
} else {
logsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* 5 latest logs of executed jobs. Read only.
*
*
* repeated .ct.JobHistory logs = 10;
*/
public Builder addLogs(
int index, com.passkit.grpc.ct.Scheduler.JobHistory.Builder builderForValue) {
if (logsBuilder_ == null) {
ensureLogsIsMutable();
logs_.add(index, builderForValue.build());
onChanged();
} else {
logsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* 5 latest logs of executed jobs. Read only.
*
*
* repeated .ct.JobHistory logs = 10;
*/
public Builder addAllLogs(
java.lang.Iterable extends com.passkit.grpc.ct.Scheduler.JobHistory> values) {
if (logsBuilder_ == null) {
ensureLogsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, logs_);
onChanged();
} else {
logsBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* 5 latest logs of executed jobs. Read only.
*
*
* repeated .ct.JobHistory logs = 10;
*/
public Builder clearLogs() {
if (logsBuilder_ == null) {
logs_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
logsBuilder_.clear();
}
return this;
}
/**
*
* 5 latest logs of executed jobs. Read only.
*
*
* repeated .ct.JobHistory logs = 10;
*/
public Builder removeLogs(int index) {
if (logsBuilder_ == null) {
ensureLogsIsMutable();
logs_.remove(index);
onChanged();
} else {
logsBuilder_.remove(index);
}
return this;
}
/**
*
* 5 latest logs of executed jobs. Read only.
*
*
* repeated .ct.JobHistory logs = 10;
*/
public com.passkit.grpc.ct.Scheduler.JobHistory.Builder getLogsBuilder(
int index) {
return getLogsFieldBuilder().getBuilder(index);
}
/**
*
* 5 latest logs of executed jobs. Read only.
*
*
* repeated .ct.JobHistory logs = 10;
*/
public com.passkit.grpc.ct.Scheduler.JobHistoryOrBuilder getLogsOrBuilder(
int index) {
if (logsBuilder_ == null) {
return logs_.get(index); } else {
return logsBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* 5 latest logs of executed jobs. Read only.
*
*
* repeated .ct.JobHistory logs = 10;
*/
public java.util.List extends com.passkit.grpc.ct.Scheduler.JobHistoryOrBuilder>
getLogsOrBuilderList() {
if (logsBuilder_ != null) {
return logsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(logs_);
}
}
/**
*
* 5 latest logs of executed jobs. Read only.
*
*
* repeated .ct.JobHistory logs = 10;
*/
public com.passkit.grpc.ct.Scheduler.JobHistory.Builder addLogsBuilder() {
return getLogsFieldBuilder().addBuilder(
com.passkit.grpc.ct.Scheduler.JobHistory.getDefaultInstance());
}
/**
*
* 5 latest logs of executed jobs. Read only.
*
*
* repeated .ct.JobHistory logs = 10;
*/
public com.passkit.grpc.ct.Scheduler.JobHistory.Builder addLogsBuilder(
int index) {
return getLogsFieldBuilder().addBuilder(
index, com.passkit.grpc.ct.Scheduler.JobHistory.getDefaultInstance());
}
/**
*
* 5 latest logs of executed jobs. Read only.
*
*
* repeated .ct.JobHistory logs = 10;
*/
public java.util.List
getLogsBuilderList() {
return getLogsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.passkit.grpc.ct.Scheduler.JobHistory, com.passkit.grpc.ct.Scheduler.JobHistory.Builder, com.passkit.grpc.ct.Scheduler.JobHistoryOrBuilder>
getLogsFieldBuilder() {
if (logsBuilder_ == null) {
logsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.passkit.grpc.ct.Scheduler.JobHistory, com.passkit.grpc.ct.Scheduler.JobHistory.Builder, com.passkit.grpc.ct.Scheduler.JobHistoryOrBuilder>(
logs_,
((bitField0_ & 0x00000001) != 0),
getParentForChildren(),
isClean());
logs_ = null;
}
return logsBuilder_;
}
private com.google.protobuf.Timestamp created_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder> createdBuilder_;
/**
*
* Datetime the job was created. Read only.
*
*
* .google.protobuf.Timestamp created = 11;
* @return Whether the created field is set.
*/
public boolean hasCreated() {
return createdBuilder_ != null || created_ != null;
}
/**
*
* Datetime the job was created. Read only.
*
*
* .google.protobuf.Timestamp created = 11;
* @return The created.
*/
public com.google.protobuf.Timestamp getCreated() {
if (createdBuilder_ == null) {
return created_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : created_;
} else {
return createdBuilder_.getMessage();
}
}
/**
*
* Datetime the job was created. Read only.
*
*
* .google.protobuf.Timestamp created = 11;
*/
public Builder setCreated(com.google.protobuf.Timestamp value) {
if (createdBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
created_ = value;
onChanged();
} else {
createdBuilder_.setMessage(value);
}
return this;
}
/**
*
* Datetime the job was created. Read only.
*
*
* .google.protobuf.Timestamp created = 11;
*/
public Builder setCreated(
com.google.protobuf.Timestamp.Builder builderForValue) {
if (createdBuilder_ == null) {
created_ = builderForValue.build();
onChanged();
} else {
createdBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Datetime the job was created. Read only.
*
*
* .google.protobuf.Timestamp created = 11;
*/
public Builder mergeCreated(com.google.protobuf.Timestamp value) {
if (createdBuilder_ == null) {
if (created_ != null) {
created_ =
com.google.protobuf.Timestamp.newBuilder(created_).mergeFrom(value).buildPartial();
} else {
created_ = value;
}
onChanged();
} else {
createdBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Datetime the job was created. Read only.
*
*
* .google.protobuf.Timestamp created = 11;
*/
public Builder clearCreated() {
if (createdBuilder_ == null) {
created_ = null;
onChanged();
} else {
created_ = null;
createdBuilder_ = null;
}
return this;
}
/**
*
* Datetime the job was created. Read only.
*
*
* .google.protobuf.Timestamp created = 11;
*/
public com.google.protobuf.Timestamp.Builder getCreatedBuilder() {
onChanged();
return getCreatedFieldBuilder().getBuilder();
}
/**
*
* Datetime the job was created. Read only.
*
*
* .google.protobuf.Timestamp created = 11;
*/
public com.google.protobuf.TimestampOrBuilder getCreatedOrBuilder() {
if (createdBuilder_ != null) {
return createdBuilder_.getMessageOrBuilder();
} else {
return created_ == null ?
com.google.protobuf.Timestamp.getDefaultInstance() : created_;
}
}
/**
*
* Datetime the job was created. Read only.
*
*
* .google.protobuf.Timestamp created = 11;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder>
getCreatedFieldBuilder() {
if (createdBuilder_ == null) {
createdBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder>(
getCreated(),
getParentForChildren(),
isClean());
created_ = null;
}
return createdBuilder_;
}
private com.google.protobuf.Timestamp updated_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder> updatedBuilder_;
/**
*
* Datetime the job was last updated. Read only.
*
*
* .google.protobuf.Timestamp updated = 12;
* @return Whether the updated field is set.
*/
public boolean hasUpdated() {
return updatedBuilder_ != null || updated_ != null;
}
/**
*
* Datetime the job was last updated. Read only.
*
*
* .google.protobuf.Timestamp updated = 12;
* @return The updated.
*/
public com.google.protobuf.Timestamp getUpdated() {
if (updatedBuilder_ == null) {
return updated_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : updated_;
} else {
return updatedBuilder_.getMessage();
}
}
/**
*
* Datetime the job was last updated. Read only.
*
*
* .google.protobuf.Timestamp updated = 12;
*/
public Builder setUpdated(com.google.protobuf.Timestamp value) {
if (updatedBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
updated_ = value;
onChanged();
} else {
updatedBuilder_.setMessage(value);
}
return this;
}
/**
*
* Datetime the job was last updated. Read only.
*
*
* .google.protobuf.Timestamp updated = 12;
*/
public Builder setUpdated(
com.google.protobuf.Timestamp.Builder builderForValue) {
if (updatedBuilder_ == null) {
updated_ = builderForValue.build();
onChanged();
} else {
updatedBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Datetime the job was last updated. Read only.
*
*
* .google.protobuf.Timestamp updated = 12;
*/
public Builder mergeUpdated(com.google.protobuf.Timestamp value) {
if (updatedBuilder_ == null) {
if (updated_ != null) {
updated_ =
com.google.protobuf.Timestamp.newBuilder(updated_).mergeFrom(value).buildPartial();
} else {
updated_ = value;
}
onChanged();
} else {
updatedBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Datetime the job was last updated. Read only.
*
*
* .google.protobuf.Timestamp updated = 12;
*/
public Builder clearUpdated() {
if (updatedBuilder_ == null) {
updated_ = null;
onChanged();
} else {
updated_ = null;
updatedBuilder_ = null;
}
return this;
}
/**
*
* Datetime the job was last updated. Read only.
*
*
* .google.protobuf.Timestamp updated = 12;
*/
public com.google.protobuf.Timestamp.Builder getUpdatedBuilder() {
onChanged();
return getUpdatedFieldBuilder().getBuilder();
}
/**
*
* Datetime the job was last updated. Read only.
*
*
* .google.protobuf.Timestamp updated = 12;
*/
public com.google.protobuf.TimestampOrBuilder getUpdatedOrBuilder() {
if (updatedBuilder_ != null) {
return updatedBuilder_.getMessageOrBuilder();
} else {
return updated_ == null ?
com.google.protobuf.Timestamp.getDefaultInstance() : updated_;
}
}
/**
*
* Datetime the job was last updated. Read only.
*
*
* .google.protobuf.Timestamp updated = 12;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder>
getUpdatedFieldBuilder() {
if (updatedBuilder_ == null) {
updatedBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder>(
getUpdated(),
getParentForChildren(),
isClean());
updated_ = null;
}
return updatedBuilder_;
}
private com.google.protobuf.Timestamp expiry_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder> expiryBuilder_;
/**
*
* Datetime when the job will for the last time and expires. Read only. Expiry setting is available in the Schedule field.
*
*
* .google.protobuf.Timestamp expiry = 13;
* @return Whether the expiry field is set.
*/
public boolean hasExpiry() {
return expiryBuilder_ != null || expiry_ != null;
}
/**
*
* Datetime when the job will for the last time and expires. Read only. Expiry setting is available in the Schedule field.
*
*
* .google.protobuf.Timestamp expiry = 13;
* @return The expiry.
*/
public com.google.protobuf.Timestamp getExpiry() {
if (expiryBuilder_ == null) {
return expiry_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : expiry_;
} else {
return expiryBuilder_.getMessage();
}
}
/**
*
* Datetime when the job will for the last time and expires. Read only. Expiry setting is available in the Schedule field.
*
*
* .google.protobuf.Timestamp expiry = 13;
*/
public Builder setExpiry(com.google.protobuf.Timestamp value) {
if (expiryBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
expiry_ = value;
onChanged();
} else {
expiryBuilder_.setMessage(value);
}
return this;
}
/**
*
* Datetime when the job will for the last time and expires. Read only. Expiry setting is available in the Schedule field.
*
*
* .google.protobuf.Timestamp expiry = 13;
*/
public Builder setExpiry(
com.google.protobuf.Timestamp.Builder builderForValue) {
if (expiryBuilder_ == null) {
expiry_ = builderForValue.build();
onChanged();
} else {
expiryBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Datetime when the job will for the last time and expires. Read only. Expiry setting is available in the Schedule field.
*
*
* .google.protobuf.Timestamp expiry = 13;
*/
public Builder mergeExpiry(com.google.protobuf.Timestamp value) {
if (expiryBuilder_ == null) {
if (expiry_ != null) {
expiry_ =
com.google.protobuf.Timestamp.newBuilder(expiry_).mergeFrom(value).buildPartial();
} else {
expiry_ = value;
}
onChanged();
} else {
expiryBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Datetime when the job will for the last time and expires. Read only. Expiry setting is available in the Schedule field.
*
*
* .google.protobuf.Timestamp expiry = 13;
*/
public Builder clearExpiry() {
if (expiryBuilder_ == null) {
expiry_ = null;
onChanged();
} else {
expiry_ = null;
expiryBuilder_ = null;
}
return this;
}
/**
*
* Datetime when the job will for the last time and expires. Read only. Expiry setting is available in the Schedule field.
*
*
* .google.protobuf.Timestamp expiry = 13;
*/
public com.google.protobuf.Timestamp.Builder getExpiryBuilder() {
onChanged();
return getExpiryFieldBuilder().getBuilder();
}
/**
*
* Datetime when the job will for the last time and expires. Read only. Expiry setting is available in the Schedule field.
*
*
* .google.protobuf.Timestamp expiry = 13;
*/
public com.google.protobuf.TimestampOrBuilder getExpiryOrBuilder() {
if (expiryBuilder_ != null) {
return expiryBuilder_.getMessageOrBuilder();
} else {
return expiry_ == null ?
com.google.protobuf.Timestamp.getDefaultInstance() : expiry_;
}
}
/**
*
* Datetime when the job will for the last time and expires. Read only. Expiry setting is available in the Schedule field.
*
*
* .google.protobuf.Timestamp expiry = 13;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder>
getExpiryFieldBuilder() {
if (expiryBuilder_ == null) {
expiryBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder>(
getExpiry(),
getParentForChildren(),
isClean());
expiry_ = null;
}
return expiryBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:ct.SchedulingJob)
}
// @@protoc_insertion_point(class_scope:ct.SchedulingJob)
private static final com.passkit.grpc.ct.Scheduler.SchedulingJob DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.passkit.grpc.ct.Scheduler.SchedulingJob();
}
public static com.passkit.grpc.ct.Scheduler.SchedulingJob getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public SchedulingJob parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new SchedulingJob(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.passkit.grpc.ct.Scheduler.SchedulingJob getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ScheduleOrBuilder extends
// @@protoc_insertion_point(interface_extends:ct.Schedule)
com.google.protobuf.MessageOrBuilder {
/**
*
* Crontab schedule expression. e.g. '0 * * * *', '@hourly'
* For month end job, '@monthend' is available.
* To run a one-off job now, '@now' is available.
* Crontab generator tool https://crontab.guru
* Reference https://en.wikipedia.org/wiki/Cron
*
*
* string schedule = 1;
* @return The schedule.
*/
java.lang.String getSchedule();
/**
*
* Crontab schedule expression. e.g. '0 * * * *', '@hourly'
* For month end job, '@monthend' is available.
* To run a one-off job now, '@now' is available.
* Crontab generator tool https://crontab.guru
* Reference https://en.wikipedia.org/wiki/Cron
*
*
* string schedule = 1;
* @return The bytes for schedule.
*/
com.google.protobuf.ByteString
getScheduleBytes();
/**
*
* Iana timezone. Default is UTC.
*
*
* string timezone = 2;
* @return The timezone.
*/
java.lang.String getTimezone();
/**
*
* Iana timezone. Default is UTC.
*
*
* string timezone = 2;
* @return The bytes for timezone.
*/
com.google.protobuf.ByteString
getTimezoneBytes();
/**
*
* Datetime to start the first job or resume the paused job.
*
*
* .google.protobuf.Timestamp startsAt = 3;
* @return Whether the startsAt field is set.
*/
boolean hasStartsAt();
/**
*
* Datetime to start the first job or resume the paused job.
*
*
* .google.protobuf.Timestamp startsAt = 3;
* @return The startsAt.
*/
com.google.protobuf.Timestamp getStartsAt();
/**
*
* Datetime to start the first job or resume the paused job.
*
*
* .google.protobuf.Timestamp startsAt = 3;
*/
com.google.protobuf.TimestampOrBuilder getStartsAtOrBuilder();
/**
*
* Set repeat count to repeat the job for certain times (eg. 3 will repeat the job for 3 times).
* If job is one off, set -1.
*
*
* sint32 repeatCount = 15;
* @return Whether the repeatCount field is set.
*/
boolean hasRepeatCount();
/**
*
* Set repeat count to repeat the job for certain times (eg. 3 will repeat the job for 3 times).
* If job is one off, set -1.
*
*
* sint32 repeatCount = 15;
* @return The repeatCount.
*/
int getRepeatCount();
/**
*
* The date which job will be expired.
*
*
* .google.protobuf.Timestamp expiryDate = 16;
* @return Whether the expiryDate field is set.
*/
boolean hasExpiryDate();
/**
*
* The date which job will be expired.
*
*
* .google.protobuf.Timestamp expiryDate = 16;
* @return The expiryDate.
*/
com.google.protobuf.Timestamp getExpiryDate();
/**
*
* The date which job will be expired.
*
*
* .google.protobuf.Timestamp expiryDate = 16;
*/
com.google.protobuf.TimestampOrBuilder getExpiryDateOrBuilder();
public com.passkit.grpc.ct.Scheduler.Schedule.ExpiryCase getExpiryCase();
}
/**
* Protobuf type {@code ct.Schedule}
*/
public static final class Schedule extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:ct.Schedule)
ScheduleOrBuilder {
private static final long serialVersionUID = 0L;
// Use Schedule.newBuilder() to construct.
private Schedule(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Schedule() {
schedule_ = "";
timezone_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new Schedule();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Schedule(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
schedule_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
timezone_ = s;
break;
}
case 26: {
com.google.protobuf.Timestamp.Builder subBuilder = null;
if (startsAt_ != null) {
subBuilder = startsAt_.toBuilder();
}
startsAt_ = input.readMessage(com.google.protobuf.Timestamp.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(startsAt_);
startsAt_ = subBuilder.buildPartial();
}
break;
}
case 120: {
expiry_ = input.readSInt32();
expiryCase_ = 15;
break;
}
case 130: {
com.google.protobuf.Timestamp.Builder subBuilder = null;
if (expiryCase_ == 16) {
subBuilder = ((com.google.protobuf.Timestamp) expiry_).toBuilder();
}
expiry_ =
input.readMessage(com.google.protobuf.Timestamp.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((com.google.protobuf.Timestamp) expiry_);
expiry_ = subBuilder.buildPartial();
}
expiryCase_ = 16;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.passkit.grpc.ct.Scheduler.internal_static_ct_Schedule_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.passkit.grpc.ct.Scheduler.internal_static_ct_Schedule_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.passkit.grpc.ct.Scheduler.Schedule.class, com.passkit.grpc.ct.Scheduler.Schedule.Builder.class);
}
private int expiryCase_ = 0;
private java.lang.Object expiry_;
public enum ExpiryCase
implements com.google.protobuf.Internal.EnumLite,
com.google.protobuf.AbstractMessage.InternalOneOfEnum {
REPEATCOUNT(15),
EXPIRYDATE(16),
EXPIRY_NOT_SET(0);
private final int value;
private ExpiryCase(int value) {
this.value = value;
}
/**
* @param value The number of the enum to look for.
* @return The enum associated with the given number.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static ExpiryCase valueOf(int value) {
return forNumber(value);
}
public static ExpiryCase forNumber(int value) {
switch (value) {
case 15: return REPEATCOUNT;
case 16: return EXPIRYDATE;
case 0: return EXPIRY_NOT_SET;
default: return null;
}
}
public int getNumber() {
return this.value;
}
};
public ExpiryCase
getExpiryCase() {
return ExpiryCase.forNumber(
expiryCase_);
}
public static final int SCHEDULE_FIELD_NUMBER = 1;
private volatile java.lang.Object schedule_;
/**
*
* Crontab schedule expression. e.g. '0 * * * *', '@hourly'
* For month end job, '@monthend' is available.
* To run a one-off job now, '@now' is available.
* Crontab generator tool https://crontab.guru
* Reference https://en.wikipedia.org/wiki/Cron
*
*
* string schedule = 1;
* @return The schedule.
*/
@java.lang.Override
public java.lang.String getSchedule() {
java.lang.Object ref = schedule_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
schedule_ = s;
return s;
}
}
/**
*
* Crontab schedule expression. e.g. '0 * * * *', '@hourly'
* For month end job, '@monthend' is available.
* To run a one-off job now, '@now' is available.
* Crontab generator tool https://crontab.guru
* Reference https://en.wikipedia.org/wiki/Cron
*
*
* string schedule = 1;
* @return The bytes for schedule.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getScheduleBytes() {
java.lang.Object ref = schedule_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
schedule_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int TIMEZONE_FIELD_NUMBER = 2;
private volatile java.lang.Object timezone_;
/**
*
* Iana timezone. Default is UTC.
*
*
* string timezone = 2;
* @return The timezone.
*/
@java.lang.Override
public java.lang.String getTimezone() {
java.lang.Object ref = timezone_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
timezone_ = s;
return s;
}
}
/**
*
* Iana timezone. Default is UTC.
*
*
* string timezone = 2;
* @return The bytes for timezone.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getTimezoneBytes() {
java.lang.Object ref = timezone_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
timezone_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int STARTSAT_FIELD_NUMBER = 3;
private com.google.protobuf.Timestamp startsAt_;
/**
*
* Datetime to start the first job or resume the paused job.
*
*
* .google.protobuf.Timestamp startsAt = 3;
* @return Whether the startsAt field is set.
*/
@java.lang.Override
public boolean hasStartsAt() {
return startsAt_ != null;
}
/**
*
* Datetime to start the first job or resume the paused job.
*
*
* .google.protobuf.Timestamp startsAt = 3;
* @return The startsAt.
*/
@java.lang.Override
public com.google.protobuf.Timestamp getStartsAt() {
return startsAt_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : startsAt_;
}
/**
*
* Datetime to start the first job or resume the paused job.
*
*
* .google.protobuf.Timestamp startsAt = 3;
*/
@java.lang.Override
public com.google.protobuf.TimestampOrBuilder getStartsAtOrBuilder() {
return getStartsAt();
}
public static final int REPEATCOUNT_FIELD_NUMBER = 15;
/**
*
* Set repeat count to repeat the job for certain times (eg. 3 will repeat the job for 3 times).
* If job is one off, set -1.
*
*
* sint32 repeatCount = 15;
* @return Whether the repeatCount field is set.
*/
@java.lang.Override
public boolean hasRepeatCount() {
return expiryCase_ == 15;
}
/**
*
* Set repeat count to repeat the job for certain times (eg. 3 will repeat the job for 3 times).
* If job is one off, set -1.
*
*
* sint32 repeatCount = 15;
* @return The repeatCount.
*/
@java.lang.Override
public int getRepeatCount() {
if (expiryCase_ == 15) {
return (java.lang.Integer) expiry_;
}
return 0;
}
public static final int EXPIRYDATE_FIELD_NUMBER = 16;
/**
*
* The date which job will be expired.
*
*
* .google.protobuf.Timestamp expiryDate = 16;
* @return Whether the expiryDate field is set.
*/
@java.lang.Override
public boolean hasExpiryDate() {
return expiryCase_ == 16;
}
/**
*
* The date which job will be expired.
*
*
* .google.protobuf.Timestamp expiryDate = 16;
* @return The expiryDate.
*/
@java.lang.Override
public com.google.protobuf.Timestamp getExpiryDate() {
if (expiryCase_ == 16) {
return (com.google.protobuf.Timestamp) expiry_;
}
return com.google.protobuf.Timestamp.getDefaultInstance();
}
/**
*
* The date which job will be expired.
*
*
* .google.protobuf.Timestamp expiryDate = 16;
*/
@java.lang.Override
public com.google.protobuf.TimestampOrBuilder getExpiryDateOrBuilder() {
if (expiryCase_ == 16) {
return (com.google.protobuf.Timestamp) expiry_;
}
return com.google.protobuf.Timestamp.getDefaultInstance();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(schedule_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, schedule_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(timezone_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, timezone_);
}
if (startsAt_ != null) {
output.writeMessage(3, getStartsAt());
}
if (expiryCase_ == 15) {
output.writeSInt32(
15, (int)((java.lang.Integer) expiry_));
}
if (expiryCase_ == 16) {
output.writeMessage(16, (com.google.protobuf.Timestamp) expiry_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(schedule_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, schedule_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(timezone_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, timezone_);
}
if (startsAt_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getStartsAt());
}
if (expiryCase_ == 15) {
size += com.google.protobuf.CodedOutputStream
.computeSInt32Size(
15, (int)((java.lang.Integer) expiry_));
}
if (expiryCase_ == 16) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(16, (com.google.protobuf.Timestamp) expiry_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.passkit.grpc.ct.Scheduler.Schedule)) {
return super.equals(obj);
}
com.passkit.grpc.ct.Scheduler.Schedule other = (com.passkit.grpc.ct.Scheduler.Schedule) obj;
if (!getSchedule()
.equals(other.getSchedule())) return false;
if (!getTimezone()
.equals(other.getTimezone())) return false;
if (hasStartsAt() != other.hasStartsAt()) return false;
if (hasStartsAt()) {
if (!getStartsAt()
.equals(other.getStartsAt())) return false;
}
if (!getExpiryCase().equals(other.getExpiryCase())) return false;
switch (expiryCase_) {
case 15:
if (getRepeatCount()
!= other.getRepeatCount()) return false;
break;
case 16:
if (!getExpiryDate()
.equals(other.getExpiryDate())) return false;
break;
case 0:
default:
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + SCHEDULE_FIELD_NUMBER;
hash = (53 * hash) + getSchedule().hashCode();
hash = (37 * hash) + TIMEZONE_FIELD_NUMBER;
hash = (53 * hash) + getTimezone().hashCode();
if (hasStartsAt()) {
hash = (37 * hash) + STARTSAT_FIELD_NUMBER;
hash = (53 * hash) + getStartsAt().hashCode();
}
switch (expiryCase_) {
case 15:
hash = (37 * hash) + REPEATCOUNT_FIELD_NUMBER;
hash = (53 * hash) + getRepeatCount();
break;
case 16:
hash = (37 * hash) + EXPIRYDATE_FIELD_NUMBER;
hash = (53 * hash) + getExpiryDate().hashCode();
break;
case 0:
default:
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.passkit.grpc.ct.Scheduler.Schedule parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.passkit.grpc.ct.Scheduler.Schedule parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.passkit.grpc.ct.Scheduler.Schedule parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.passkit.grpc.ct.Scheduler.Schedule parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.passkit.grpc.ct.Scheduler.Schedule parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.passkit.grpc.ct.Scheduler.Schedule parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.passkit.grpc.ct.Scheduler.Schedule parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.passkit.grpc.ct.Scheduler.Schedule parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.passkit.grpc.ct.Scheduler.Schedule parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.passkit.grpc.ct.Scheduler.Schedule parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.passkit.grpc.ct.Scheduler.Schedule parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.passkit.grpc.ct.Scheduler.Schedule parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.passkit.grpc.ct.Scheduler.Schedule prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code ct.Schedule}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:ct.Schedule)
com.passkit.grpc.ct.Scheduler.ScheduleOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.passkit.grpc.ct.Scheduler.internal_static_ct_Schedule_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.passkit.grpc.ct.Scheduler.internal_static_ct_Schedule_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.passkit.grpc.ct.Scheduler.Schedule.class, com.passkit.grpc.ct.Scheduler.Schedule.Builder.class);
}
// Construct using com.passkit.grpc.ct.Scheduler.Schedule.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
schedule_ = "";
timezone_ = "";
if (startsAtBuilder_ == null) {
startsAt_ = null;
} else {
startsAt_ = null;
startsAtBuilder_ = null;
}
expiryCase_ = 0;
expiry_ = null;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.passkit.grpc.ct.Scheduler.internal_static_ct_Schedule_descriptor;
}
@java.lang.Override
public com.passkit.grpc.ct.Scheduler.Schedule getDefaultInstanceForType() {
return com.passkit.grpc.ct.Scheduler.Schedule.getDefaultInstance();
}
@java.lang.Override
public com.passkit.grpc.ct.Scheduler.Schedule build() {
com.passkit.grpc.ct.Scheduler.Schedule result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.passkit.grpc.ct.Scheduler.Schedule buildPartial() {
com.passkit.grpc.ct.Scheduler.Schedule result = new com.passkit.grpc.ct.Scheduler.Schedule(this);
result.schedule_ = schedule_;
result.timezone_ = timezone_;
if (startsAtBuilder_ == null) {
result.startsAt_ = startsAt_;
} else {
result.startsAt_ = startsAtBuilder_.build();
}
if (expiryCase_ == 15) {
result.expiry_ = expiry_;
}
if (expiryCase_ == 16) {
if (expiryDateBuilder_ == null) {
result.expiry_ = expiry_;
} else {
result.expiry_ = expiryDateBuilder_.build();
}
}
result.expiryCase_ = expiryCase_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.passkit.grpc.ct.Scheduler.Schedule) {
return mergeFrom((com.passkit.grpc.ct.Scheduler.Schedule)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.passkit.grpc.ct.Scheduler.Schedule other) {
if (other == com.passkit.grpc.ct.Scheduler.Schedule.getDefaultInstance()) return this;
if (!other.getSchedule().isEmpty()) {
schedule_ = other.schedule_;
onChanged();
}
if (!other.getTimezone().isEmpty()) {
timezone_ = other.timezone_;
onChanged();
}
if (other.hasStartsAt()) {
mergeStartsAt(other.getStartsAt());
}
switch (other.getExpiryCase()) {
case REPEATCOUNT: {
setRepeatCount(other.getRepeatCount());
break;
}
case EXPIRYDATE: {
mergeExpiryDate(other.getExpiryDate());
break;
}
case EXPIRY_NOT_SET: {
break;
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.passkit.grpc.ct.Scheduler.Schedule parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.passkit.grpc.ct.Scheduler.Schedule) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int expiryCase_ = 0;
private java.lang.Object expiry_;
public ExpiryCase
getExpiryCase() {
return ExpiryCase.forNumber(
expiryCase_);
}
public Builder clearExpiry() {
expiryCase_ = 0;
expiry_ = null;
onChanged();
return this;
}
private java.lang.Object schedule_ = "";
/**
*
* Crontab schedule expression. e.g. '0 * * * *', '@hourly'
* For month end job, '@monthend' is available.
* To run a one-off job now, '@now' is available.
* Crontab generator tool https://crontab.guru
* Reference https://en.wikipedia.org/wiki/Cron
*
*
* string schedule = 1;
* @return The schedule.
*/
public java.lang.String getSchedule() {
java.lang.Object ref = schedule_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
schedule_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Crontab schedule expression. e.g. '0 * * * *', '@hourly'
* For month end job, '@monthend' is available.
* To run a one-off job now, '@now' is available.
* Crontab generator tool https://crontab.guru
* Reference https://en.wikipedia.org/wiki/Cron
*
*
* string schedule = 1;
* @return The bytes for schedule.
*/
public com.google.protobuf.ByteString
getScheduleBytes() {
java.lang.Object ref = schedule_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
schedule_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Crontab schedule expression. e.g. '0 * * * *', '@hourly'
* For month end job, '@monthend' is available.
* To run a one-off job now, '@now' is available.
* Crontab generator tool https://crontab.guru
* Reference https://en.wikipedia.org/wiki/Cron
*
*
* string schedule = 1;
* @param value The schedule to set.
* @return This builder for chaining.
*/
public Builder setSchedule(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
schedule_ = value;
onChanged();
return this;
}
/**
*
* Crontab schedule expression. e.g. '0 * * * *', '@hourly'
* For month end job, '@monthend' is available.
* To run a one-off job now, '@now' is available.
* Crontab generator tool https://crontab.guru
* Reference https://en.wikipedia.org/wiki/Cron
*
*
* string schedule = 1;
* @return This builder for chaining.
*/
public Builder clearSchedule() {
schedule_ = getDefaultInstance().getSchedule();
onChanged();
return this;
}
/**
*
* Crontab schedule expression. e.g. '0 * * * *', '@hourly'
* For month end job, '@monthend' is available.
* To run a one-off job now, '@now' is available.
* Crontab generator tool https://crontab.guru
* Reference https://en.wikipedia.org/wiki/Cron
*
*
* string schedule = 1;
* @param value The bytes for schedule to set.
* @return This builder for chaining.
*/
public Builder setScheduleBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
schedule_ = value;
onChanged();
return this;
}
private java.lang.Object timezone_ = "";
/**
*
* Iana timezone. Default is UTC.
*
*
* string timezone = 2;
* @return The timezone.
*/
public java.lang.String getTimezone() {
java.lang.Object ref = timezone_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
timezone_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Iana timezone. Default is UTC.
*
*
* string timezone = 2;
* @return The bytes for timezone.
*/
public com.google.protobuf.ByteString
getTimezoneBytes() {
java.lang.Object ref = timezone_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
timezone_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Iana timezone. Default is UTC.
*
*
* string timezone = 2;
* @param value The timezone to set.
* @return This builder for chaining.
*/
public Builder setTimezone(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
timezone_ = value;
onChanged();
return this;
}
/**
*
* Iana timezone. Default is UTC.
*
*
* string timezone = 2;
* @return This builder for chaining.
*/
public Builder clearTimezone() {
timezone_ = getDefaultInstance().getTimezone();
onChanged();
return this;
}
/**
*
* Iana timezone. Default is UTC.
*
*
* string timezone = 2;
* @param value The bytes for timezone to set.
* @return This builder for chaining.
*/
public Builder setTimezoneBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
timezone_ = value;
onChanged();
return this;
}
private com.google.protobuf.Timestamp startsAt_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder> startsAtBuilder_;
/**
*
* Datetime to start the first job or resume the paused job.
*
*
* .google.protobuf.Timestamp startsAt = 3;
* @return Whether the startsAt field is set.
*/
public boolean hasStartsAt() {
return startsAtBuilder_ != null || startsAt_ != null;
}
/**
*
* Datetime to start the first job or resume the paused job.
*
*
* .google.protobuf.Timestamp startsAt = 3;
* @return The startsAt.
*/
public com.google.protobuf.Timestamp getStartsAt() {
if (startsAtBuilder_ == null) {
return startsAt_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : startsAt_;
} else {
return startsAtBuilder_.getMessage();
}
}
/**
*
* Datetime to start the first job or resume the paused job.
*
*
* .google.protobuf.Timestamp startsAt = 3;
*/
public Builder setStartsAt(com.google.protobuf.Timestamp value) {
if (startsAtBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
startsAt_ = value;
onChanged();
} else {
startsAtBuilder_.setMessage(value);
}
return this;
}
/**
*
* Datetime to start the first job or resume the paused job.
*
*
* .google.protobuf.Timestamp startsAt = 3;
*/
public Builder setStartsAt(
com.google.protobuf.Timestamp.Builder builderForValue) {
if (startsAtBuilder_ == null) {
startsAt_ = builderForValue.build();
onChanged();
} else {
startsAtBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Datetime to start the first job or resume the paused job.
*
*
* .google.protobuf.Timestamp startsAt = 3;
*/
public Builder mergeStartsAt(com.google.protobuf.Timestamp value) {
if (startsAtBuilder_ == null) {
if (startsAt_ != null) {
startsAt_ =
com.google.protobuf.Timestamp.newBuilder(startsAt_).mergeFrom(value).buildPartial();
} else {
startsAt_ = value;
}
onChanged();
} else {
startsAtBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Datetime to start the first job or resume the paused job.
*
*
* .google.protobuf.Timestamp startsAt = 3;
*/
public Builder clearStartsAt() {
if (startsAtBuilder_ == null) {
startsAt_ = null;
onChanged();
} else {
startsAt_ = null;
startsAtBuilder_ = null;
}
return this;
}
/**
*
* Datetime to start the first job or resume the paused job.
*
*
* .google.protobuf.Timestamp startsAt = 3;
*/
public com.google.protobuf.Timestamp.Builder getStartsAtBuilder() {
onChanged();
return getStartsAtFieldBuilder().getBuilder();
}
/**
*
* Datetime to start the first job or resume the paused job.
*
*
* .google.protobuf.Timestamp startsAt = 3;
*/
public com.google.protobuf.TimestampOrBuilder getStartsAtOrBuilder() {
if (startsAtBuilder_ != null) {
return startsAtBuilder_.getMessageOrBuilder();
} else {
return startsAt_ == null ?
com.google.protobuf.Timestamp.getDefaultInstance() : startsAt_;
}
}
/**
*
* Datetime to start the first job or resume the paused job.
*
*
* .google.protobuf.Timestamp startsAt = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder>
getStartsAtFieldBuilder() {
if (startsAtBuilder_ == null) {
startsAtBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder>(
getStartsAt(),
getParentForChildren(),
isClean());
startsAt_ = null;
}
return startsAtBuilder_;
}
/**
*
* Set repeat count to repeat the job for certain times (eg. 3 will repeat the job for 3 times).
* If job is one off, set -1.
*
*
* sint32 repeatCount = 15;
* @return Whether the repeatCount field is set.
*/
public boolean hasRepeatCount() {
return expiryCase_ == 15;
}
/**
*
* Set repeat count to repeat the job for certain times (eg. 3 will repeat the job for 3 times).
* If job is one off, set -1.
*
*
* sint32 repeatCount = 15;
* @return The repeatCount.
*/
public int getRepeatCount() {
if (expiryCase_ == 15) {
return (java.lang.Integer) expiry_;
}
return 0;
}
/**
*
* Set repeat count to repeat the job for certain times (eg. 3 will repeat the job for 3 times).
* If job is one off, set -1.
*
*
* sint32 repeatCount = 15;
* @param value The repeatCount to set.
* @return This builder for chaining.
*/
public Builder setRepeatCount(int value) {
expiryCase_ = 15;
expiry_ = value;
onChanged();
return this;
}
/**
*
* Set repeat count to repeat the job for certain times (eg. 3 will repeat the job for 3 times).
* If job is one off, set -1.
*
*
* sint32 repeatCount = 15;
* @return This builder for chaining.
*/
public Builder clearRepeatCount() {
if (expiryCase_ == 15) {
expiryCase_ = 0;
expiry_ = null;
onChanged();
}
return this;
}
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder> expiryDateBuilder_;
/**
*
* The date which job will be expired.
*
*
* .google.protobuf.Timestamp expiryDate = 16;
* @return Whether the expiryDate field is set.
*/
@java.lang.Override
public boolean hasExpiryDate() {
return expiryCase_ == 16;
}
/**
*
* The date which job will be expired.
*
*
* .google.protobuf.Timestamp expiryDate = 16;
* @return The expiryDate.
*/
@java.lang.Override
public com.google.protobuf.Timestamp getExpiryDate() {
if (expiryDateBuilder_ == null) {
if (expiryCase_ == 16) {
return (com.google.protobuf.Timestamp) expiry_;
}
return com.google.protobuf.Timestamp.getDefaultInstance();
} else {
if (expiryCase_ == 16) {
return expiryDateBuilder_.getMessage();
}
return com.google.protobuf.Timestamp.getDefaultInstance();
}
}
/**
*
* The date which job will be expired.
*
*
* .google.protobuf.Timestamp expiryDate = 16;
*/
public Builder setExpiryDate(com.google.protobuf.Timestamp value) {
if (expiryDateBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
expiry_ = value;
onChanged();
} else {
expiryDateBuilder_.setMessage(value);
}
expiryCase_ = 16;
return this;
}
/**
*
* The date which job will be expired.
*
*
* .google.protobuf.Timestamp expiryDate = 16;
*/
public Builder setExpiryDate(
com.google.protobuf.Timestamp.Builder builderForValue) {
if (expiryDateBuilder_ == null) {
expiry_ = builderForValue.build();
onChanged();
} else {
expiryDateBuilder_.setMessage(builderForValue.build());
}
expiryCase_ = 16;
return this;
}
/**
*
* The date which job will be expired.
*
*
* .google.protobuf.Timestamp expiryDate = 16;
*/
public Builder mergeExpiryDate(com.google.protobuf.Timestamp value) {
if (expiryDateBuilder_ == null) {
if (expiryCase_ == 16 &&
expiry_ != com.google.protobuf.Timestamp.getDefaultInstance()) {
expiry_ = com.google.protobuf.Timestamp.newBuilder((com.google.protobuf.Timestamp) expiry_)
.mergeFrom(value).buildPartial();
} else {
expiry_ = value;
}
onChanged();
} else {
if (expiryCase_ == 16) {
expiryDateBuilder_.mergeFrom(value);
}
expiryDateBuilder_.setMessage(value);
}
expiryCase_ = 16;
return this;
}
/**
*
* The date which job will be expired.
*
*
* .google.protobuf.Timestamp expiryDate = 16;
*/
public Builder clearExpiryDate() {
if (expiryDateBuilder_ == null) {
if (expiryCase_ == 16) {
expiryCase_ = 0;
expiry_ = null;
onChanged();
}
} else {
if (expiryCase_ == 16) {
expiryCase_ = 0;
expiry_ = null;
}
expiryDateBuilder_.clear();
}
return this;
}
/**
*
* The date which job will be expired.
*
*
* .google.protobuf.Timestamp expiryDate = 16;
*/
public com.google.protobuf.Timestamp.Builder getExpiryDateBuilder() {
return getExpiryDateFieldBuilder().getBuilder();
}
/**
*
* The date which job will be expired.
*
*
* .google.protobuf.Timestamp expiryDate = 16;
*/
@java.lang.Override
public com.google.protobuf.TimestampOrBuilder getExpiryDateOrBuilder() {
if ((expiryCase_ == 16) && (expiryDateBuilder_ != null)) {
return expiryDateBuilder_.getMessageOrBuilder();
} else {
if (expiryCase_ == 16) {
return (com.google.protobuf.Timestamp) expiry_;
}
return com.google.protobuf.Timestamp.getDefaultInstance();
}
}
/**
*
* The date which job will be expired.
*
*
* .google.protobuf.Timestamp expiryDate = 16;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder>
getExpiryDateFieldBuilder() {
if (expiryDateBuilder_ == null) {
if (!(expiryCase_ == 16)) {
expiry_ = com.google.protobuf.Timestamp.getDefaultInstance();
}
expiryDateBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder>(
(com.google.protobuf.Timestamp) expiry_,
getParentForChildren(),
isClean());
expiry_ = null;
}
expiryCase_ = 16;
onChanged();;
return expiryDateBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:ct.Schedule)
}
// @@protoc_insertion_point(class_scope:ct.Schedule)
private static final com.passkit.grpc.ct.Scheduler.Schedule DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.passkit.grpc.ct.Scheduler.Schedule();
}
public static com.passkit.grpc.ct.Scheduler.Schedule getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Schedule parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Schedule(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.passkit.grpc.ct.Scheduler.Schedule getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface SchedulingJobResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:ct.SchedulingJobResponse)
com.google.protobuf.MessageOrBuilder {
/**
*
* Auto generated 22 char identifier. Read only.
*
*
* string id = 1;
* @return The id.
*/
java.lang.String getId();
/**
*
* Auto generated 22 char identifier. Read only.
*
*
* string id = 1;
* @return The bytes for id.
*/
com.google.protobuf.ByteString
getIdBytes();
/**
*
* Job status. Writable values are JOB_STATUS_READY and JOB_STATUS_PAUSED only.
*
*
* .ct.JobStatus status = 2;
* @return The enum numeric value on the wire for status.
*/
int getStatusValue();
/**
*
* Job status. Writable values are JOB_STATUS_READY and JOB_STATUS_PAUSED only.
*
*
* .ct.JobStatus status = 2;
* @return The status.
*/
com.passkit.grpc.ct.Scheduler.JobStatus getStatus();
/**
*
* Next scheduled run datetime. Read only.
*
*
* .google.protobuf.Timestamp nextRunDatetime = 3;
* @return Whether the nextRunDatetime field is set.
*/
boolean hasNextRunDatetime();
/**
*
* Next scheduled run datetime. Read only.
*
*
* .google.protobuf.Timestamp nextRunDatetime = 3;
* @return The nextRunDatetime.
*/
com.google.protobuf.Timestamp getNextRunDatetime();
/**
*
* Next scheduled run datetime. Read only.
*
*
* .google.protobuf.Timestamp nextRunDatetime = 3;
*/
com.google.protobuf.TimestampOrBuilder getNextRunDatetimeOrBuilder();
/**
*
* Datetime when the job will for the last time and expires. Read only. Expiry setting is available in the Schedule field.
*
*
* .google.protobuf.Timestamp expiry = 4;
* @return Whether the expiry field is set.
*/
boolean hasExpiry();
/**
*
* Datetime when the job will for the last time and expires. Read only. Expiry setting is available in the Schedule field.
*
*
* .google.protobuf.Timestamp expiry = 4;
* @return The expiry.
*/
com.google.protobuf.Timestamp getExpiry();
/**
*
* Datetime when the job will for the last time and expires. Read only. Expiry setting is available in the Schedule field.
*
*
* .google.protobuf.Timestamp expiry = 4;
*/
com.google.protobuf.TimestampOrBuilder getExpiryOrBuilder();
}
/**
* Protobuf type {@code ct.SchedulingJobResponse}
*/
public static final class SchedulingJobResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:ct.SchedulingJobResponse)
SchedulingJobResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use SchedulingJobResponse.newBuilder() to construct.
private SchedulingJobResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private SchedulingJobResponse() {
id_ = "";
status_ = 0;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new SchedulingJobResponse();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private SchedulingJobResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
id_ = s;
break;
}
case 16: {
int rawValue = input.readEnum();
status_ = rawValue;
break;
}
case 26: {
com.google.protobuf.Timestamp.Builder subBuilder = null;
if (nextRunDatetime_ != null) {
subBuilder = nextRunDatetime_.toBuilder();
}
nextRunDatetime_ = input.readMessage(com.google.protobuf.Timestamp.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(nextRunDatetime_);
nextRunDatetime_ = subBuilder.buildPartial();
}
break;
}
case 34: {
com.google.protobuf.Timestamp.Builder subBuilder = null;
if (expiry_ != null) {
subBuilder = expiry_.toBuilder();
}
expiry_ = input.readMessage(com.google.protobuf.Timestamp.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(expiry_);
expiry_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.passkit.grpc.ct.Scheduler.internal_static_ct_SchedulingJobResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.passkit.grpc.ct.Scheduler.internal_static_ct_SchedulingJobResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.passkit.grpc.ct.Scheduler.SchedulingJobResponse.class, com.passkit.grpc.ct.Scheduler.SchedulingJobResponse.Builder.class);
}
public static final int ID_FIELD_NUMBER = 1;
private volatile java.lang.Object id_;
/**
*
* Auto generated 22 char identifier. Read only.
*
*
* string id = 1;
* @return The id.
*/
@java.lang.Override
public java.lang.String getId() {
java.lang.Object ref = id_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
id_ = s;
return s;
}
}
/**
*
* Auto generated 22 char identifier. Read only.
*
*
* string id = 1;
* @return The bytes for id.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getIdBytes() {
java.lang.Object ref = id_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
id_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int STATUS_FIELD_NUMBER = 2;
private int status_;
/**
*
* Job status. Writable values are JOB_STATUS_READY and JOB_STATUS_PAUSED only.
*
*
* .ct.JobStatus status = 2;
* @return The enum numeric value on the wire for status.
*/
@java.lang.Override public int getStatusValue() {
return status_;
}
/**
*
* Job status. Writable values are JOB_STATUS_READY and JOB_STATUS_PAUSED only.
*
*
* .ct.JobStatus status = 2;
* @return The status.
*/
@java.lang.Override public com.passkit.grpc.ct.Scheduler.JobStatus getStatus() {
@SuppressWarnings("deprecation")
com.passkit.grpc.ct.Scheduler.JobStatus result = com.passkit.grpc.ct.Scheduler.JobStatus.valueOf(status_);
return result == null ? com.passkit.grpc.ct.Scheduler.JobStatus.UNRECOGNIZED : result;
}
public static final int NEXTRUNDATETIME_FIELD_NUMBER = 3;
private com.google.protobuf.Timestamp nextRunDatetime_;
/**
*
* Next scheduled run datetime. Read only.
*
*
* .google.protobuf.Timestamp nextRunDatetime = 3;
* @return Whether the nextRunDatetime field is set.
*/
@java.lang.Override
public boolean hasNextRunDatetime() {
return nextRunDatetime_ != null;
}
/**
*
* Next scheduled run datetime. Read only.
*
*
* .google.protobuf.Timestamp nextRunDatetime = 3;
* @return The nextRunDatetime.
*/
@java.lang.Override
public com.google.protobuf.Timestamp getNextRunDatetime() {
return nextRunDatetime_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : nextRunDatetime_;
}
/**
*
* Next scheduled run datetime. Read only.
*
*
* .google.protobuf.Timestamp nextRunDatetime = 3;
*/
@java.lang.Override
public com.google.protobuf.TimestampOrBuilder getNextRunDatetimeOrBuilder() {
return getNextRunDatetime();
}
public static final int EXPIRY_FIELD_NUMBER = 4;
private com.google.protobuf.Timestamp expiry_;
/**
*
* Datetime when the job will for the last time and expires. Read only. Expiry setting is available in the Schedule field.
*
*
* .google.protobuf.Timestamp expiry = 4;
* @return Whether the expiry field is set.
*/
@java.lang.Override
public boolean hasExpiry() {
return expiry_ != null;
}
/**
*
* Datetime when the job will for the last time and expires. Read only. Expiry setting is available in the Schedule field.
*
*
* .google.protobuf.Timestamp expiry = 4;
* @return The expiry.
*/
@java.lang.Override
public com.google.protobuf.Timestamp getExpiry() {
return expiry_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : expiry_;
}
/**
*
* Datetime when the job will for the last time and expires. Read only. Expiry setting is available in the Schedule field.
*
*
* .google.protobuf.Timestamp expiry = 4;
*/
@java.lang.Override
public com.google.protobuf.TimestampOrBuilder getExpiryOrBuilder() {
return getExpiry();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(id_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, id_);
}
if (status_ != com.passkit.grpc.ct.Scheduler.JobStatus.JOB_STATUS_NONE.getNumber()) {
output.writeEnum(2, status_);
}
if (nextRunDatetime_ != null) {
output.writeMessage(3, getNextRunDatetime());
}
if (expiry_ != null) {
output.writeMessage(4, getExpiry());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(id_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, id_);
}
if (status_ != com.passkit.grpc.ct.Scheduler.JobStatus.JOB_STATUS_NONE.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(2, status_);
}
if (nextRunDatetime_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getNextRunDatetime());
}
if (expiry_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, getExpiry());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.passkit.grpc.ct.Scheduler.SchedulingJobResponse)) {
return super.equals(obj);
}
com.passkit.grpc.ct.Scheduler.SchedulingJobResponse other = (com.passkit.grpc.ct.Scheduler.SchedulingJobResponse) obj;
if (!getId()
.equals(other.getId())) return false;
if (status_ != other.status_) return false;
if (hasNextRunDatetime() != other.hasNextRunDatetime()) return false;
if (hasNextRunDatetime()) {
if (!getNextRunDatetime()
.equals(other.getNextRunDatetime())) return false;
}
if (hasExpiry() != other.hasExpiry()) return false;
if (hasExpiry()) {
if (!getExpiry()
.equals(other.getExpiry())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + ID_FIELD_NUMBER;
hash = (53 * hash) + getId().hashCode();
hash = (37 * hash) + STATUS_FIELD_NUMBER;
hash = (53 * hash) + status_;
if (hasNextRunDatetime()) {
hash = (37 * hash) + NEXTRUNDATETIME_FIELD_NUMBER;
hash = (53 * hash) + getNextRunDatetime().hashCode();
}
if (hasExpiry()) {
hash = (37 * hash) + EXPIRY_FIELD_NUMBER;
hash = (53 * hash) + getExpiry().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.passkit.grpc.ct.Scheduler.SchedulingJobResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.passkit.grpc.ct.Scheduler.SchedulingJobResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.passkit.grpc.ct.Scheduler.SchedulingJobResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.passkit.grpc.ct.Scheduler.SchedulingJobResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.passkit.grpc.ct.Scheduler.SchedulingJobResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.passkit.grpc.ct.Scheduler.SchedulingJobResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.passkit.grpc.ct.Scheduler.SchedulingJobResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.passkit.grpc.ct.Scheduler.SchedulingJobResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.passkit.grpc.ct.Scheduler.SchedulingJobResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.passkit.grpc.ct.Scheduler.SchedulingJobResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.passkit.grpc.ct.Scheduler.SchedulingJobResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.passkit.grpc.ct.Scheduler.SchedulingJobResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.passkit.grpc.ct.Scheduler.SchedulingJobResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code ct.SchedulingJobResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:ct.SchedulingJobResponse)
com.passkit.grpc.ct.Scheduler.SchedulingJobResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.passkit.grpc.ct.Scheduler.internal_static_ct_SchedulingJobResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.passkit.grpc.ct.Scheduler.internal_static_ct_SchedulingJobResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.passkit.grpc.ct.Scheduler.SchedulingJobResponse.class, com.passkit.grpc.ct.Scheduler.SchedulingJobResponse.Builder.class);
}
// Construct using com.passkit.grpc.ct.Scheduler.SchedulingJobResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
id_ = "";
status_ = 0;
if (nextRunDatetimeBuilder_ == null) {
nextRunDatetime_ = null;
} else {
nextRunDatetime_ = null;
nextRunDatetimeBuilder_ = null;
}
if (expiryBuilder_ == null) {
expiry_ = null;
} else {
expiry_ = null;
expiryBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.passkit.grpc.ct.Scheduler.internal_static_ct_SchedulingJobResponse_descriptor;
}
@java.lang.Override
public com.passkit.grpc.ct.Scheduler.SchedulingJobResponse getDefaultInstanceForType() {
return com.passkit.grpc.ct.Scheduler.SchedulingJobResponse.getDefaultInstance();
}
@java.lang.Override
public com.passkit.grpc.ct.Scheduler.SchedulingJobResponse build() {
com.passkit.grpc.ct.Scheduler.SchedulingJobResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.passkit.grpc.ct.Scheduler.SchedulingJobResponse buildPartial() {
com.passkit.grpc.ct.Scheduler.SchedulingJobResponse result = new com.passkit.grpc.ct.Scheduler.SchedulingJobResponse(this);
result.id_ = id_;
result.status_ = status_;
if (nextRunDatetimeBuilder_ == null) {
result.nextRunDatetime_ = nextRunDatetime_;
} else {
result.nextRunDatetime_ = nextRunDatetimeBuilder_.build();
}
if (expiryBuilder_ == null) {
result.expiry_ = expiry_;
} else {
result.expiry_ = expiryBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.passkit.grpc.ct.Scheduler.SchedulingJobResponse) {
return mergeFrom((com.passkit.grpc.ct.Scheduler.SchedulingJobResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.passkit.grpc.ct.Scheduler.SchedulingJobResponse other) {
if (other == com.passkit.grpc.ct.Scheduler.SchedulingJobResponse.getDefaultInstance()) return this;
if (!other.getId().isEmpty()) {
id_ = other.id_;
onChanged();
}
if (other.status_ != 0) {
setStatusValue(other.getStatusValue());
}
if (other.hasNextRunDatetime()) {
mergeNextRunDatetime(other.getNextRunDatetime());
}
if (other.hasExpiry()) {
mergeExpiry(other.getExpiry());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.passkit.grpc.ct.Scheduler.SchedulingJobResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.passkit.grpc.ct.Scheduler.SchedulingJobResponse) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object id_ = "";
/**
*
* Auto generated 22 char identifier. Read only.
*
*
* string id = 1;
* @return The id.
*/
public java.lang.String getId() {
java.lang.Object ref = id_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
id_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Auto generated 22 char identifier. Read only.
*
*
* string id = 1;
* @return The bytes for id.
*/
public com.google.protobuf.ByteString
getIdBytes() {
java.lang.Object ref = id_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
id_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Auto generated 22 char identifier. Read only.
*
*
* string id = 1;
* @param value The id to set.
* @return This builder for chaining.
*/
public Builder setId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
id_ = value;
onChanged();
return this;
}
/**
*
* Auto generated 22 char identifier. Read only.
*
*
* string id = 1;
* @return This builder for chaining.
*/
public Builder clearId() {
id_ = getDefaultInstance().getId();
onChanged();
return this;
}
/**
*
* Auto generated 22 char identifier. Read only.
*
*
* string id = 1;
* @param value The bytes for id to set.
* @return This builder for chaining.
*/
public Builder setIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
id_ = value;
onChanged();
return this;
}
private int status_ = 0;
/**
*
* Job status. Writable values are JOB_STATUS_READY and JOB_STATUS_PAUSED only.
*
*
* .ct.JobStatus status = 2;
* @return The enum numeric value on the wire for status.
*/
@java.lang.Override public int getStatusValue() {
return status_;
}
/**
*
* Job status. Writable values are JOB_STATUS_READY and JOB_STATUS_PAUSED only.
*
*
* .ct.JobStatus status = 2;
* @param value The enum numeric value on the wire for status to set.
* @return This builder for chaining.
*/
public Builder setStatusValue(int value) {
status_ = value;
onChanged();
return this;
}
/**
*
* Job status. Writable values are JOB_STATUS_READY and JOB_STATUS_PAUSED only.
*
*
* .ct.JobStatus status = 2;
* @return The status.
*/
@java.lang.Override
public com.passkit.grpc.ct.Scheduler.JobStatus getStatus() {
@SuppressWarnings("deprecation")
com.passkit.grpc.ct.Scheduler.JobStatus result = com.passkit.grpc.ct.Scheduler.JobStatus.valueOf(status_);
return result == null ? com.passkit.grpc.ct.Scheduler.JobStatus.UNRECOGNIZED : result;
}
/**
*
* Job status. Writable values are JOB_STATUS_READY and JOB_STATUS_PAUSED only.
*
*
* .ct.JobStatus status = 2;
* @param value The status to set.
* @return This builder for chaining.
*/
public Builder setStatus(com.passkit.grpc.ct.Scheduler.JobStatus value) {
if (value == null) {
throw new NullPointerException();
}
status_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Job status. Writable values are JOB_STATUS_READY and JOB_STATUS_PAUSED only.
*
*
* .ct.JobStatus status = 2;
* @return This builder for chaining.
*/
public Builder clearStatus() {
status_ = 0;
onChanged();
return this;
}
private com.google.protobuf.Timestamp nextRunDatetime_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder> nextRunDatetimeBuilder_;
/**
*
* Next scheduled run datetime. Read only.
*
*
* .google.protobuf.Timestamp nextRunDatetime = 3;
* @return Whether the nextRunDatetime field is set.
*/
public boolean hasNextRunDatetime() {
return nextRunDatetimeBuilder_ != null || nextRunDatetime_ != null;
}
/**
*
* Next scheduled run datetime. Read only.
*
*
* .google.protobuf.Timestamp nextRunDatetime = 3;
* @return The nextRunDatetime.
*/
public com.google.protobuf.Timestamp getNextRunDatetime() {
if (nextRunDatetimeBuilder_ == null) {
return nextRunDatetime_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : nextRunDatetime_;
} else {
return nextRunDatetimeBuilder_.getMessage();
}
}
/**
*
* Next scheduled run datetime. Read only.
*
*
* .google.protobuf.Timestamp nextRunDatetime = 3;
*/
public Builder setNextRunDatetime(com.google.protobuf.Timestamp value) {
if (nextRunDatetimeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
nextRunDatetime_ = value;
onChanged();
} else {
nextRunDatetimeBuilder_.setMessage(value);
}
return this;
}
/**
*
* Next scheduled run datetime. Read only.
*
*
* .google.protobuf.Timestamp nextRunDatetime = 3;
*/
public Builder setNextRunDatetime(
com.google.protobuf.Timestamp.Builder builderForValue) {
if (nextRunDatetimeBuilder_ == null) {
nextRunDatetime_ = builderForValue.build();
onChanged();
} else {
nextRunDatetimeBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Next scheduled run datetime. Read only.
*
*
* .google.protobuf.Timestamp nextRunDatetime = 3;
*/
public Builder mergeNextRunDatetime(com.google.protobuf.Timestamp value) {
if (nextRunDatetimeBuilder_ == null) {
if (nextRunDatetime_ != null) {
nextRunDatetime_ =
com.google.protobuf.Timestamp.newBuilder(nextRunDatetime_).mergeFrom(value).buildPartial();
} else {
nextRunDatetime_ = value;
}
onChanged();
} else {
nextRunDatetimeBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Next scheduled run datetime. Read only.
*
*
* .google.protobuf.Timestamp nextRunDatetime = 3;
*/
public Builder clearNextRunDatetime() {
if (nextRunDatetimeBuilder_ == null) {
nextRunDatetime_ = null;
onChanged();
} else {
nextRunDatetime_ = null;
nextRunDatetimeBuilder_ = null;
}
return this;
}
/**
*
* Next scheduled run datetime. Read only.
*
*
* .google.protobuf.Timestamp nextRunDatetime = 3;
*/
public com.google.protobuf.Timestamp.Builder getNextRunDatetimeBuilder() {
onChanged();
return getNextRunDatetimeFieldBuilder().getBuilder();
}
/**
*
* Next scheduled run datetime. Read only.
*
*
* .google.protobuf.Timestamp nextRunDatetime = 3;
*/
public com.google.protobuf.TimestampOrBuilder getNextRunDatetimeOrBuilder() {
if (nextRunDatetimeBuilder_ != null) {
return nextRunDatetimeBuilder_.getMessageOrBuilder();
} else {
return nextRunDatetime_ == null ?
com.google.protobuf.Timestamp.getDefaultInstance() : nextRunDatetime_;
}
}
/**
*
* Next scheduled run datetime. Read only.
*
*
* .google.protobuf.Timestamp nextRunDatetime = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder>
getNextRunDatetimeFieldBuilder() {
if (nextRunDatetimeBuilder_ == null) {
nextRunDatetimeBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder>(
getNextRunDatetime(),
getParentForChildren(),
isClean());
nextRunDatetime_ = null;
}
return nextRunDatetimeBuilder_;
}
private com.google.protobuf.Timestamp expiry_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder> expiryBuilder_;
/**
*
* Datetime when the job will for the last time and expires. Read only. Expiry setting is available in the Schedule field.
*
*
* .google.protobuf.Timestamp expiry = 4;
* @return Whether the expiry field is set.
*/
public boolean hasExpiry() {
return expiryBuilder_ != null || expiry_ != null;
}
/**
*
* Datetime when the job will for the last time and expires. Read only. Expiry setting is available in the Schedule field.
*
*
* .google.protobuf.Timestamp expiry = 4;
* @return The expiry.
*/
public com.google.protobuf.Timestamp getExpiry() {
if (expiryBuilder_ == null) {
return expiry_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : expiry_;
} else {
return expiryBuilder_.getMessage();
}
}
/**
*
* Datetime when the job will for the last time and expires. Read only. Expiry setting is available in the Schedule field.
*
*
* .google.protobuf.Timestamp expiry = 4;
*/
public Builder setExpiry(com.google.protobuf.Timestamp value) {
if (expiryBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
expiry_ = value;
onChanged();
} else {
expiryBuilder_.setMessage(value);
}
return this;
}
/**
*
* Datetime when the job will for the last time and expires. Read only. Expiry setting is available in the Schedule field.
*
*
* .google.protobuf.Timestamp expiry = 4;
*/
public Builder setExpiry(
com.google.protobuf.Timestamp.Builder builderForValue) {
if (expiryBuilder_ == null) {
expiry_ = builderForValue.build();
onChanged();
} else {
expiryBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Datetime when the job will for the last time and expires. Read only. Expiry setting is available in the Schedule field.
*
*
* .google.protobuf.Timestamp expiry = 4;
*/
public Builder mergeExpiry(com.google.protobuf.Timestamp value) {
if (expiryBuilder_ == null) {
if (expiry_ != null) {
expiry_ =
com.google.protobuf.Timestamp.newBuilder(expiry_).mergeFrom(value).buildPartial();
} else {
expiry_ = value;
}
onChanged();
} else {
expiryBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Datetime when the job will for the last time and expires. Read only. Expiry setting is available in the Schedule field.
*
*
* .google.protobuf.Timestamp expiry = 4;
*/
public Builder clearExpiry() {
if (expiryBuilder_ == null) {
expiry_ = null;
onChanged();
} else {
expiry_ = null;
expiryBuilder_ = null;
}
return this;
}
/**
*
* Datetime when the job will for the last time and expires. Read only. Expiry setting is available in the Schedule field.
*
*
* .google.protobuf.Timestamp expiry = 4;
*/
public com.google.protobuf.Timestamp.Builder getExpiryBuilder() {
onChanged();
return getExpiryFieldBuilder().getBuilder();
}
/**
*
* Datetime when the job will for the last time and expires. Read only. Expiry setting is available in the Schedule field.
*
*
* .google.protobuf.Timestamp expiry = 4;
*/
public com.google.protobuf.TimestampOrBuilder getExpiryOrBuilder() {
if (expiryBuilder_ != null) {
return expiryBuilder_.getMessageOrBuilder();
} else {
return expiry_ == null ?
com.google.protobuf.Timestamp.getDefaultInstance() : expiry_;
}
}
/**
*
* Datetime when the job will for the last time and expires. Read only. Expiry setting is available in the Schedule field.
*
*
* .google.protobuf.Timestamp expiry = 4;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder>
getExpiryFieldBuilder() {
if (expiryBuilder_ == null) {
expiryBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder>(
getExpiry(),
getParentForChildren(),
isClean());
expiry_ = null;
}
return expiryBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:ct.SchedulingJobResponse)
}
// @@protoc_insertion_point(class_scope:ct.SchedulingJobResponse)
private static final com.passkit.grpc.ct.Scheduler.SchedulingJobResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.passkit.grpc.ct.Scheduler.SchedulingJobResponse();
}
public static com.passkit.grpc.ct.Scheduler.SchedulingJobResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public SchedulingJobResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new SchedulingJobResponse(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.passkit.grpc.ct.Scheduler.SchedulingJobResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface JobHistoryOrBuilder extends
// @@protoc_insertion_point(interface_extends:ct.JobHistory)
com.google.protobuf.MessageOrBuilder {
/**
*
* Job history id.
*
*
* string id = 1;
* @return The id.
*/
java.lang.String getId();
/**
*
* Job history id.
*
*
* string id = 1;
* @return The bytes for id.
*/
com.google.protobuf.ByteString
getIdBytes();
/**
*
* Job ID.
*
*
* string jobId = 2;
* @return The jobId.
*/
java.lang.String getJobId();
/**
*
* Job ID.
*
*
* string jobId = 2;
* @return The bytes for jobId.
*/
com.google.protobuf.ByteString
getJobIdBytes();
/**
*
* True if the job has succeeded. False if the job has failed.
*
*
* bool success = 3;
* @return The success.
*/
boolean getSuccess();
/**
*
* The job output log.
*
*
* string log = 4;
* @return The log.
*/
java.lang.String getLog();
/**
*
* The job output log.
*
*
* string log = 4;
* @return The bytes for log.
*/
com.google.protobuf.ByteString
getLogBytes();
/**
*
* The status code.
*
*
* uint32 statusCode = 5;
* @return The statusCode.
*/
int getStatusCode();
/**
*
* Scheduled run datetime for the job.
*
*
* .google.protobuf.Timestamp scheduledRunDateTime = 6;
* @return Whether the scheduledRunDateTime field is set.
*/
boolean hasScheduledRunDateTime();
/**
*
* Scheduled run datetime for the job.
*
*
* .google.protobuf.Timestamp scheduledRunDateTime = 6;
* @return The scheduledRunDateTime.
*/
com.google.protobuf.Timestamp getScheduledRunDateTime();
/**
*
* Scheduled run datetime for the job.
*
*
* .google.protobuf.Timestamp scheduledRunDateTime = 6;
*/
com.google.protobuf.TimestampOrBuilder getScheduledRunDateTimeOrBuilder();
/**
*
* Job completion datetime.
*
*
* .google.protobuf.Timestamp completedAt = 7;
* @return Whether the completedAt field is set.
*/
boolean hasCompletedAt();
/**
*
* Job completion datetime.
*
*
* .google.protobuf.Timestamp completedAt = 7;
* @return The completedAt.
*/
com.google.protobuf.Timestamp getCompletedAt();
/**
*
* Job completion datetime.
*
*
* .google.protobuf.Timestamp completedAt = 7;
*/
com.google.protobuf.TimestampOrBuilder getCompletedAtOrBuilder();
}
/**
* Protobuf type {@code ct.JobHistory}
*/
public static final class JobHistory extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:ct.JobHistory)
JobHistoryOrBuilder {
private static final long serialVersionUID = 0L;
// Use JobHistory.newBuilder() to construct.
private JobHistory(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private JobHistory() {
id_ = "";
jobId_ = "";
log_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new JobHistory();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private JobHistory(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
id_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
jobId_ = s;
break;
}
case 24: {
success_ = input.readBool();
break;
}
case 34: {
java.lang.String s = input.readStringRequireUtf8();
log_ = s;
break;
}
case 40: {
statusCode_ = input.readUInt32();
break;
}
case 50: {
com.google.protobuf.Timestamp.Builder subBuilder = null;
if (scheduledRunDateTime_ != null) {
subBuilder = scheduledRunDateTime_.toBuilder();
}
scheduledRunDateTime_ = input.readMessage(com.google.protobuf.Timestamp.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(scheduledRunDateTime_);
scheduledRunDateTime_ = subBuilder.buildPartial();
}
break;
}
case 58: {
com.google.protobuf.Timestamp.Builder subBuilder = null;
if (completedAt_ != null) {
subBuilder = completedAt_.toBuilder();
}
completedAt_ = input.readMessage(com.google.protobuf.Timestamp.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(completedAt_);
completedAt_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.passkit.grpc.ct.Scheduler.internal_static_ct_JobHistory_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.passkit.grpc.ct.Scheduler.internal_static_ct_JobHistory_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.passkit.grpc.ct.Scheduler.JobHistory.class, com.passkit.grpc.ct.Scheduler.JobHistory.Builder.class);
}
public static final int ID_FIELD_NUMBER = 1;
private volatile java.lang.Object id_;
/**
*
* Job history id.
*
*
* string id = 1;
* @return The id.
*/
@java.lang.Override
public java.lang.String getId() {
java.lang.Object ref = id_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
id_ = s;
return s;
}
}
/**
*
* Job history id.
*
*
* string id = 1;
* @return The bytes for id.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getIdBytes() {
java.lang.Object ref = id_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
id_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int JOBID_FIELD_NUMBER = 2;
private volatile java.lang.Object jobId_;
/**
*
* Job ID.
*
*
* string jobId = 2;
* @return The jobId.
*/
@java.lang.Override
public java.lang.String getJobId() {
java.lang.Object ref = jobId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
jobId_ = s;
return s;
}
}
/**
*
* Job ID.
*
*
* string jobId = 2;
* @return The bytes for jobId.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getJobIdBytes() {
java.lang.Object ref = jobId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
jobId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SUCCESS_FIELD_NUMBER = 3;
private boolean success_;
/**
*
* True if the job has succeeded. False if the job has failed.
*
*
* bool success = 3;
* @return The success.
*/
@java.lang.Override
public boolean getSuccess() {
return success_;
}
public static final int LOG_FIELD_NUMBER = 4;
private volatile java.lang.Object log_;
/**
*
* The job output log.
*
*
* string log = 4;
* @return The log.
*/
@java.lang.Override
public java.lang.String getLog() {
java.lang.Object ref = log_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
log_ = s;
return s;
}
}
/**
*
* The job output log.
*
*
* string log = 4;
* @return The bytes for log.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getLogBytes() {
java.lang.Object ref = log_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
log_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int STATUSCODE_FIELD_NUMBER = 5;
private int statusCode_;
/**
*
* The status code.
*
*
* uint32 statusCode = 5;
* @return The statusCode.
*/
@java.lang.Override
public int getStatusCode() {
return statusCode_;
}
public static final int SCHEDULEDRUNDATETIME_FIELD_NUMBER = 6;
private com.google.protobuf.Timestamp scheduledRunDateTime_;
/**
*
* Scheduled run datetime for the job.
*
*
* .google.protobuf.Timestamp scheduledRunDateTime = 6;
* @return Whether the scheduledRunDateTime field is set.
*/
@java.lang.Override
public boolean hasScheduledRunDateTime() {
return scheduledRunDateTime_ != null;
}
/**
*
* Scheduled run datetime for the job.
*
*
* .google.protobuf.Timestamp scheduledRunDateTime = 6;
* @return The scheduledRunDateTime.
*/
@java.lang.Override
public com.google.protobuf.Timestamp getScheduledRunDateTime() {
return scheduledRunDateTime_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : scheduledRunDateTime_;
}
/**
*
* Scheduled run datetime for the job.
*
*
* .google.protobuf.Timestamp scheduledRunDateTime = 6;
*/
@java.lang.Override
public com.google.protobuf.TimestampOrBuilder getScheduledRunDateTimeOrBuilder() {
return getScheduledRunDateTime();
}
public static final int COMPLETEDAT_FIELD_NUMBER = 7;
private com.google.protobuf.Timestamp completedAt_;
/**
*
* Job completion datetime.
*
*
* .google.protobuf.Timestamp completedAt = 7;
* @return Whether the completedAt field is set.
*/
@java.lang.Override
public boolean hasCompletedAt() {
return completedAt_ != null;
}
/**
*
* Job completion datetime.
*
*
* .google.protobuf.Timestamp completedAt = 7;
* @return The completedAt.
*/
@java.lang.Override
public com.google.protobuf.Timestamp getCompletedAt() {
return completedAt_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : completedAt_;
}
/**
*
* Job completion datetime.
*
*
* .google.protobuf.Timestamp completedAt = 7;
*/
@java.lang.Override
public com.google.protobuf.TimestampOrBuilder getCompletedAtOrBuilder() {
return getCompletedAt();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(id_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, id_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(jobId_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, jobId_);
}
if (success_ != false) {
output.writeBool(3, success_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(log_)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, log_);
}
if (statusCode_ != 0) {
output.writeUInt32(5, statusCode_);
}
if (scheduledRunDateTime_ != null) {
output.writeMessage(6, getScheduledRunDateTime());
}
if (completedAt_ != null) {
output.writeMessage(7, getCompletedAt());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(id_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, id_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(jobId_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, jobId_);
}
if (success_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(3, success_);
}
if (!com.google.protobuf.GeneratedMessageV3.isStringEmpty(log_)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(4, log_);
}
if (statusCode_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(5, statusCode_);
}
if (scheduledRunDateTime_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(6, getScheduledRunDateTime());
}
if (completedAt_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(7, getCompletedAt());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.passkit.grpc.ct.Scheduler.JobHistory)) {
return super.equals(obj);
}
com.passkit.grpc.ct.Scheduler.JobHistory other = (com.passkit.grpc.ct.Scheduler.JobHistory) obj;
if (!getId()
.equals(other.getId())) return false;
if (!getJobId()
.equals(other.getJobId())) return false;
if (getSuccess()
!= other.getSuccess()) return false;
if (!getLog()
.equals(other.getLog())) return false;
if (getStatusCode()
!= other.getStatusCode()) return false;
if (hasScheduledRunDateTime() != other.hasScheduledRunDateTime()) return false;
if (hasScheduledRunDateTime()) {
if (!getScheduledRunDateTime()
.equals(other.getScheduledRunDateTime())) return false;
}
if (hasCompletedAt() != other.hasCompletedAt()) return false;
if (hasCompletedAt()) {
if (!getCompletedAt()
.equals(other.getCompletedAt())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + ID_FIELD_NUMBER;
hash = (53 * hash) + getId().hashCode();
hash = (37 * hash) + JOBID_FIELD_NUMBER;
hash = (53 * hash) + getJobId().hashCode();
hash = (37 * hash) + SUCCESS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getSuccess());
hash = (37 * hash) + LOG_FIELD_NUMBER;
hash = (53 * hash) + getLog().hashCode();
hash = (37 * hash) + STATUSCODE_FIELD_NUMBER;
hash = (53 * hash) + getStatusCode();
if (hasScheduledRunDateTime()) {
hash = (37 * hash) + SCHEDULEDRUNDATETIME_FIELD_NUMBER;
hash = (53 * hash) + getScheduledRunDateTime().hashCode();
}
if (hasCompletedAt()) {
hash = (37 * hash) + COMPLETEDAT_FIELD_NUMBER;
hash = (53 * hash) + getCompletedAt().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.passkit.grpc.ct.Scheduler.JobHistory parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.passkit.grpc.ct.Scheduler.JobHistory parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.passkit.grpc.ct.Scheduler.JobHistory parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.passkit.grpc.ct.Scheduler.JobHistory parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.passkit.grpc.ct.Scheduler.JobHistory parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.passkit.grpc.ct.Scheduler.JobHistory parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.passkit.grpc.ct.Scheduler.JobHistory parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.passkit.grpc.ct.Scheduler.JobHistory parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.passkit.grpc.ct.Scheduler.JobHistory parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.passkit.grpc.ct.Scheduler.JobHistory parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.passkit.grpc.ct.Scheduler.JobHistory parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.passkit.grpc.ct.Scheduler.JobHistory parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.passkit.grpc.ct.Scheduler.JobHistory prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code ct.JobHistory}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:ct.JobHistory)
com.passkit.grpc.ct.Scheduler.JobHistoryOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.passkit.grpc.ct.Scheduler.internal_static_ct_JobHistory_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.passkit.grpc.ct.Scheduler.internal_static_ct_JobHistory_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.passkit.grpc.ct.Scheduler.JobHistory.class, com.passkit.grpc.ct.Scheduler.JobHistory.Builder.class);
}
// Construct using com.passkit.grpc.ct.Scheduler.JobHistory.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
id_ = "";
jobId_ = "";
success_ = false;
log_ = "";
statusCode_ = 0;
if (scheduledRunDateTimeBuilder_ == null) {
scheduledRunDateTime_ = null;
} else {
scheduledRunDateTime_ = null;
scheduledRunDateTimeBuilder_ = null;
}
if (completedAtBuilder_ == null) {
completedAt_ = null;
} else {
completedAt_ = null;
completedAtBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.passkit.grpc.ct.Scheduler.internal_static_ct_JobHistory_descriptor;
}
@java.lang.Override
public com.passkit.grpc.ct.Scheduler.JobHistory getDefaultInstanceForType() {
return com.passkit.grpc.ct.Scheduler.JobHistory.getDefaultInstance();
}
@java.lang.Override
public com.passkit.grpc.ct.Scheduler.JobHistory build() {
com.passkit.grpc.ct.Scheduler.JobHistory result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.passkit.grpc.ct.Scheduler.JobHistory buildPartial() {
com.passkit.grpc.ct.Scheduler.JobHistory result = new com.passkit.grpc.ct.Scheduler.JobHistory(this);
result.id_ = id_;
result.jobId_ = jobId_;
result.success_ = success_;
result.log_ = log_;
result.statusCode_ = statusCode_;
if (scheduledRunDateTimeBuilder_ == null) {
result.scheduledRunDateTime_ = scheduledRunDateTime_;
} else {
result.scheduledRunDateTime_ = scheduledRunDateTimeBuilder_.build();
}
if (completedAtBuilder_ == null) {
result.completedAt_ = completedAt_;
} else {
result.completedAt_ = completedAtBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.passkit.grpc.ct.Scheduler.JobHistory) {
return mergeFrom((com.passkit.grpc.ct.Scheduler.JobHistory)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.passkit.grpc.ct.Scheduler.JobHistory other) {
if (other == com.passkit.grpc.ct.Scheduler.JobHistory.getDefaultInstance()) return this;
if (!other.getId().isEmpty()) {
id_ = other.id_;
onChanged();
}
if (!other.getJobId().isEmpty()) {
jobId_ = other.jobId_;
onChanged();
}
if (other.getSuccess() != false) {
setSuccess(other.getSuccess());
}
if (!other.getLog().isEmpty()) {
log_ = other.log_;
onChanged();
}
if (other.getStatusCode() != 0) {
setStatusCode(other.getStatusCode());
}
if (other.hasScheduledRunDateTime()) {
mergeScheduledRunDateTime(other.getScheduledRunDateTime());
}
if (other.hasCompletedAt()) {
mergeCompletedAt(other.getCompletedAt());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.passkit.grpc.ct.Scheduler.JobHistory parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.passkit.grpc.ct.Scheduler.JobHistory) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object id_ = "";
/**
*
* Job history id.
*
*
* string id = 1;
* @return The id.
*/
public java.lang.String getId() {
java.lang.Object ref = id_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
id_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Job history id.
*
*
* string id = 1;
* @return The bytes for id.
*/
public com.google.protobuf.ByteString
getIdBytes() {
java.lang.Object ref = id_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
id_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Job history id.
*
*
* string id = 1;
* @param value The id to set.
* @return This builder for chaining.
*/
public Builder setId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
id_ = value;
onChanged();
return this;
}
/**
*
* Job history id.
*
*
* string id = 1;
* @return This builder for chaining.
*/
public Builder clearId() {
id_ = getDefaultInstance().getId();
onChanged();
return this;
}
/**
*
* Job history id.
*
*
* string id = 1;
* @param value The bytes for id to set.
* @return This builder for chaining.
*/
public Builder setIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
id_ = value;
onChanged();
return this;
}
private java.lang.Object jobId_ = "";
/**
*
* Job ID.
*
*
* string jobId = 2;
* @return The jobId.
*/
public java.lang.String getJobId() {
java.lang.Object ref = jobId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
jobId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Job ID.
*
*
* string jobId = 2;
* @return The bytes for jobId.
*/
public com.google.protobuf.ByteString
getJobIdBytes() {
java.lang.Object ref = jobId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
jobId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Job ID.
*
*
* string jobId = 2;
* @param value The jobId to set.
* @return This builder for chaining.
*/
public Builder setJobId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
jobId_ = value;
onChanged();
return this;
}
/**
*
* Job ID.
*
*
* string jobId = 2;
* @return This builder for chaining.
*/
public Builder clearJobId() {
jobId_ = getDefaultInstance().getJobId();
onChanged();
return this;
}
/**
*
* Job ID.
*
*
* string jobId = 2;
* @param value The bytes for jobId to set.
* @return This builder for chaining.
*/
public Builder setJobIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
jobId_ = value;
onChanged();
return this;
}
private boolean success_ ;
/**
*
* True if the job has succeeded. False if the job has failed.
*
*
* bool success = 3;
* @return The success.
*/
@java.lang.Override
public boolean getSuccess() {
return success_;
}
/**
*
* True if the job has succeeded. False if the job has failed.
*
*
* bool success = 3;
* @param value The success to set.
* @return This builder for chaining.
*/
public Builder setSuccess(boolean value) {
success_ = value;
onChanged();
return this;
}
/**
*
* True if the job has succeeded. False if the job has failed.
*
*
* bool success = 3;
* @return This builder for chaining.
*/
public Builder clearSuccess() {
success_ = false;
onChanged();
return this;
}
private java.lang.Object log_ = "";
/**
*
* The job output log.
*
*
* string log = 4;
* @return The log.
*/
public java.lang.String getLog() {
java.lang.Object ref = log_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
log_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* The job output log.
*
*
* string log = 4;
* @return The bytes for log.
*/
public com.google.protobuf.ByteString
getLogBytes() {
java.lang.Object ref = log_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
log_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* The job output log.
*
*
* string log = 4;
* @param value The log to set.
* @return This builder for chaining.
*/
public Builder setLog(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
log_ = value;
onChanged();
return this;
}
/**
*
* The job output log.
*
*
* string log = 4;
* @return This builder for chaining.
*/
public Builder clearLog() {
log_ = getDefaultInstance().getLog();
onChanged();
return this;
}
/**
*
* The job output log.
*
*
* string log = 4;
* @param value The bytes for log to set.
* @return This builder for chaining.
*/
public Builder setLogBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
log_ = value;
onChanged();
return this;
}
private int statusCode_ ;
/**
*
* The status code.
*
*
* uint32 statusCode = 5;
* @return The statusCode.
*/
@java.lang.Override
public int getStatusCode() {
return statusCode_;
}
/**
*
* The status code.
*
*
* uint32 statusCode = 5;
* @param value The statusCode to set.
* @return This builder for chaining.
*/
public Builder setStatusCode(int value) {
statusCode_ = value;
onChanged();
return this;
}
/**
*
* The status code.
*
*
* uint32 statusCode = 5;
* @return This builder for chaining.
*/
public Builder clearStatusCode() {
statusCode_ = 0;
onChanged();
return this;
}
private com.google.protobuf.Timestamp scheduledRunDateTime_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder> scheduledRunDateTimeBuilder_;
/**
*
* Scheduled run datetime for the job.
*
*
* .google.protobuf.Timestamp scheduledRunDateTime = 6;
* @return Whether the scheduledRunDateTime field is set.
*/
public boolean hasScheduledRunDateTime() {
return scheduledRunDateTimeBuilder_ != null || scheduledRunDateTime_ != null;
}
/**
*
* Scheduled run datetime for the job.
*
*
* .google.protobuf.Timestamp scheduledRunDateTime = 6;
* @return The scheduledRunDateTime.
*/
public com.google.protobuf.Timestamp getScheduledRunDateTime() {
if (scheduledRunDateTimeBuilder_ == null) {
return scheduledRunDateTime_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : scheduledRunDateTime_;
} else {
return scheduledRunDateTimeBuilder_.getMessage();
}
}
/**
*
* Scheduled run datetime for the job.
*
*
* .google.protobuf.Timestamp scheduledRunDateTime = 6;
*/
public Builder setScheduledRunDateTime(com.google.protobuf.Timestamp value) {
if (scheduledRunDateTimeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
scheduledRunDateTime_ = value;
onChanged();
} else {
scheduledRunDateTimeBuilder_.setMessage(value);
}
return this;
}
/**
*
* Scheduled run datetime for the job.
*
*
* .google.protobuf.Timestamp scheduledRunDateTime = 6;
*/
public Builder setScheduledRunDateTime(
com.google.protobuf.Timestamp.Builder builderForValue) {
if (scheduledRunDateTimeBuilder_ == null) {
scheduledRunDateTime_ = builderForValue.build();
onChanged();
} else {
scheduledRunDateTimeBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Scheduled run datetime for the job.
*
*
* .google.protobuf.Timestamp scheduledRunDateTime = 6;
*/
public Builder mergeScheduledRunDateTime(com.google.protobuf.Timestamp value) {
if (scheduledRunDateTimeBuilder_ == null) {
if (scheduledRunDateTime_ != null) {
scheduledRunDateTime_ =
com.google.protobuf.Timestamp.newBuilder(scheduledRunDateTime_).mergeFrom(value).buildPartial();
} else {
scheduledRunDateTime_ = value;
}
onChanged();
} else {
scheduledRunDateTimeBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Scheduled run datetime for the job.
*
*
* .google.protobuf.Timestamp scheduledRunDateTime = 6;
*/
public Builder clearScheduledRunDateTime() {
if (scheduledRunDateTimeBuilder_ == null) {
scheduledRunDateTime_ = null;
onChanged();
} else {
scheduledRunDateTime_ = null;
scheduledRunDateTimeBuilder_ = null;
}
return this;
}
/**
*
* Scheduled run datetime for the job.
*
*
* .google.protobuf.Timestamp scheduledRunDateTime = 6;
*/
public com.google.protobuf.Timestamp.Builder getScheduledRunDateTimeBuilder() {
onChanged();
return getScheduledRunDateTimeFieldBuilder().getBuilder();
}
/**
*
* Scheduled run datetime for the job.
*
*
* .google.protobuf.Timestamp scheduledRunDateTime = 6;
*/
public com.google.protobuf.TimestampOrBuilder getScheduledRunDateTimeOrBuilder() {
if (scheduledRunDateTimeBuilder_ != null) {
return scheduledRunDateTimeBuilder_.getMessageOrBuilder();
} else {
return scheduledRunDateTime_ == null ?
com.google.protobuf.Timestamp.getDefaultInstance() : scheduledRunDateTime_;
}
}
/**
*
* Scheduled run datetime for the job.
*
*
* .google.protobuf.Timestamp scheduledRunDateTime = 6;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder>
getScheduledRunDateTimeFieldBuilder() {
if (scheduledRunDateTimeBuilder_ == null) {
scheduledRunDateTimeBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder>(
getScheduledRunDateTime(),
getParentForChildren(),
isClean());
scheduledRunDateTime_ = null;
}
return scheduledRunDateTimeBuilder_;
}
private com.google.protobuf.Timestamp completedAt_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder> completedAtBuilder_;
/**
*
* Job completion datetime.
*
*
* .google.protobuf.Timestamp completedAt = 7;
* @return Whether the completedAt field is set.
*/
public boolean hasCompletedAt() {
return completedAtBuilder_ != null || completedAt_ != null;
}
/**
*
* Job completion datetime.
*
*
* .google.protobuf.Timestamp completedAt = 7;
* @return The completedAt.
*/
public com.google.protobuf.Timestamp getCompletedAt() {
if (completedAtBuilder_ == null) {
return completedAt_ == null ? com.google.protobuf.Timestamp.getDefaultInstance() : completedAt_;
} else {
return completedAtBuilder_.getMessage();
}
}
/**
*
* Job completion datetime.
*
*
* .google.protobuf.Timestamp completedAt = 7;
*/
public Builder setCompletedAt(com.google.protobuf.Timestamp value) {
if (completedAtBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
completedAt_ = value;
onChanged();
} else {
completedAtBuilder_.setMessage(value);
}
return this;
}
/**
*
* Job completion datetime.
*
*
* .google.protobuf.Timestamp completedAt = 7;
*/
public Builder setCompletedAt(
com.google.protobuf.Timestamp.Builder builderForValue) {
if (completedAtBuilder_ == null) {
completedAt_ = builderForValue.build();
onChanged();
} else {
completedAtBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Job completion datetime.
*
*
* .google.protobuf.Timestamp completedAt = 7;
*/
public Builder mergeCompletedAt(com.google.protobuf.Timestamp value) {
if (completedAtBuilder_ == null) {
if (completedAt_ != null) {
completedAt_ =
com.google.protobuf.Timestamp.newBuilder(completedAt_).mergeFrom(value).buildPartial();
} else {
completedAt_ = value;
}
onChanged();
} else {
completedAtBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Job completion datetime.
*
*
* .google.protobuf.Timestamp completedAt = 7;
*/
public Builder clearCompletedAt() {
if (completedAtBuilder_ == null) {
completedAt_ = null;
onChanged();
} else {
completedAt_ = null;
completedAtBuilder_ = null;
}
return this;
}
/**
*
* Job completion datetime.
*
*
* .google.protobuf.Timestamp completedAt = 7;
*/
public com.google.protobuf.Timestamp.Builder getCompletedAtBuilder() {
onChanged();
return getCompletedAtFieldBuilder().getBuilder();
}
/**
*
* Job completion datetime.
*
*
* .google.protobuf.Timestamp completedAt = 7;
*/
public com.google.protobuf.TimestampOrBuilder getCompletedAtOrBuilder() {
if (completedAtBuilder_ != null) {
return completedAtBuilder_.getMessageOrBuilder();
} else {
return completedAt_ == null ?
com.google.protobuf.Timestamp.getDefaultInstance() : completedAt_;
}
}
/**
*
* Job completion datetime.
*
*
* .google.protobuf.Timestamp completedAt = 7;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder>
getCompletedAtFieldBuilder() {
if (completedAtBuilder_ == null) {
completedAtBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Timestamp, com.google.protobuf.Timestamp.Builder, com.google.protobuf.TimestampOrBuilder>(
getCompletedAt(),
getParentForChildren(),
isClean());
completedAt_ = null;
}
return completedAtBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:ct.JobHistory)
}
// @@protoc_insertion_point(class_scope:ct.JobHistory)
private static final com.passkit.grpc.ct.Scheduler.JobHistory DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.passkit.grpc.ct.Scheduler.JobHistory();
}
public static com.passkit.grpc.ct.Scheduler.JobHistory getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public JobHistory parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new JobHistory(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.passkit.grpc.ct.Scheduler.JobHistory getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_ct_SchedulingJob_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_ct_SchedulingJob_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_ct_Schedule_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_ct_Schedule_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_ct_SchedulingJobResponse_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_ct_SchedulingJobResponse_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_ct_JobHistory_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_ct_JobHistory_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n\034ct/scheduler/scheduler.proto\022\002ct\032.prot" +
"oc-gen-openapiv2/options/annotations.pro" +
"to\032\037google/protobuf/timestamp.proto\"\206\004\n\r" +
"SchedulingJob\022\n\n\002id\030\001 \001(\t\022\023\n\013jobFunction" +
"\030\002 \001(\t\022\022\n\njobPayload\030\003 \001(\t\022\014\n\004name\030\004 \001(\t" +
"\022\023\n\013description\030\005 \001(\t\022\036\n\010schedule\030\006 \001(\0132" +
"\014.ct.Schedule\022\035\n\006status\030\007 \001(\0162\r.ct.JobSt" +
"atus\0223\n\017nextRunDatetime\030\010 \001(\0132\032.google.p" +
"rotobuf.Timestamp\0223\n\017lastRunDatetime\030\t \001" +
"(\0132\032.google.protobuf.Timestamp\022\034\n\004logs\030\n" +
" \003(\0132\016.ct.JobHistory\022+\n\007created\030\013 \001(\0132\032." +
"google.protobuf.Timestamp\022+\n\007updated\030\014 \001" +
"(\0132\032.google.protobuf.Timestamp\022*\n\006expiry" +
"\030\r \001(\0132\032.google.protobuf.Timestamp:P\222AM\n" +
"K*\016Scheduling Job2\031Recursive or one-off " +
"job.\322\001\013jobFunction\322\001\004name\322\001\010schedule\"\221\002\n" +
"\010Schedule\022\020\n\010schedule\030\001 \001(\t\022\020\n\010timezone\030" +
"\002 \001(\t\022,\n\010startsAt\030\003 \001(\0132\032.google.protobu" +
"f.Timestamp\022\025\n\013repeatCount\030\017 \001(\021H\000\0220\n\nex" +
"piryDate\030\020 \001(\0132\032.google.protobuf.Timesta" +
"mpH\000:`\222A]\n[*\010Schedule2DSet a schedule fo" +
"r the job. Set repeatCount to -1 if job " +
"is one-off.\322\001\010scheduleB\010\n\006expiry\"\374\001\n\025Sch" +
"edulingJobResponse\022\n\n\002id\030\001 \001(\t\022\035\n\006status" +
"\030\002 \001(\0162\r.ct.JobStatus\0223\n\017nextRunDatetime" +
"\030\003 \001(\0132\032.google.protobuf.Timestamp\022*\n\006ex" +
"piry\030\004 \001(\0132\032.google.protobuf.Timestamp:W" +
"\222AT\nR*\027Scheduling Job Response27A respon" +
"se object for create and update scheduli" +
"ng job.\"\217\002\n\nJobHistory\022\n\n\002id\030\001 \001(\t\022\r\n\005jo" +
"bId\030\002 \001(\t\022\017\n\007success\030\003 \001(\010\022\013\n\003log\030\004 \001(\t\022" +
"\022\n\nstatusCode\030\005 \001(\r\0228\n\024scheduledRunDateT" +
"ime\030\006 \001(\0132\032.google.protobuf.Timestamp\022/\n" +
"\013completedAt\030\007 \001(\0132\032.google.protobuf.Tim" +
"estamp:I\222AF\nD*\013Job History2\031Results of e" +
"xecuted jobs.\322\001\005jobId\322\001\003log\322\001\013completedA" +
"t*\337\001\n\tJobStatus\022\023\n\017JOB_STATUS_NONE\020\000\022\026\n\022" +
"JOB_STATUS_CREATED\020\001\022\024\n\020JOB_STATUS_READY" +
"\020\002\022\027\n\023JOB_STATUS_INFLIGHT\020\003\022\030\n\024JOB_STATU" +
"S_SUCCEEDED\020\004\022\025\n\021JOB_STATUS_FAILED\020\005\022\025\n\021" +
"JOB_STATUS_PAUSED\020\006\022\026\n\022JOB_STATUS_EXPIRE" +
"D\020\007\022\026\n\022JOB_STATUS_DELETED\020\010BM\n\023com.passk" +
"it.grpc.ctZ$stash.passkit.com/io/model/s" +
"dk/go/ct\252\002\017PassKit.Grpc.Ctb\006proto3"
};
descriptor = com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
grpc.gateway.protoc_gen_openapiv2.options.Annotations.getDescriptor(),
com.google.protobuf.TimestampProto.getDescriptor(),
});
internal_static_ct_SchedulingJob_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_ct_SchedulingJob_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_ct_SchedulingJob_descriptor,
new java.lang.String[] { "Id", "JobFunction", "JobPayload", "Name", "Description", "Schedule", "Status", "NextRunDatetime", "LastRunDatetime", "Logs", "Created", "Updated", "Expiry", });
internal_static_ct_Schedule_descriptor =
getDescriptor().getMessageTypes().get(1);
internal_static_ct_Schedule_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_ct_Schedule_descriptor,
new java.lang.String[] { "Schedule", "Timezone", "StartsAt", "RepeatCount", "ExpiryDate", "Expiry", });
internal_static_ct_SchedulingJobResponse_descriptor =
getDescriptor().getMessageTypes().get(2);
internal_static_ct_SchedulingJobResponse_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_ct_SchedulingJobResponse_descriptor,
new java.lang.String[] { "Id", "Status", "NextRunDatetime", "Expiry", });
internal_static_ct_JobHistory_descriptor =
getDescriptor().getMessageTypes().get(3);
internal_static_ct_JobHistory_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_ct_JobHistory_descriptor,
new java.lang.String[] { "Id", "JobId", "Success", "Log", "StatusCode", "ScheduledRunDateTime", "CompletedAt", });
com.google.protobuf.ExtensionRegistry registry =
com.google.protobuf.ExtensionRegistry.newInstance();
registry.add(grpc.gateway.protoc_gen_openapiv2.options.Annotations.openapiv2Schema);
com.google.protobuf.Descriptors.FileDescriptor
.internalUpdateFileDescriptor(descriptor, registry);
grpc.gateway.protoc_gen_openapiv2.options.Annotations.getDescriptor();
com.google.protobuf.TimestampProto.getDescriptor();
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy