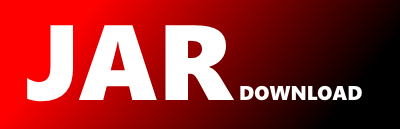
com.pathomation.Control Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pma-java Show documentation
Show all versions of pma-java Show documentation
Universal viewing of digital microscopy, whole slide imaging and digital pathology data.
pma-java is a Java wrapper library for PMA.start (http://www.free.pathomation.com), a universal viewer by Pathomation for whole slide imaging and microscopy.
YOU NEED TO HAVE PMA.START (OR PMA.CORE) RUNNING IN ORDER TO USE THIS LIBRARY. PMA-JAVA IS NOT A STAND-ALONE LIBRARY FOR WSI.
package com.pathomation;
import java.io.OutputStream;
import java.io.PrintWriter;
import java.io.StringWriter;
import java.net.HttpURLConnection;
import java.net.URL;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
import java.util.logging.Logger;
import javax.net.ssl.HttpsURLConnection;
import org.json.JSONArray;
import org.json.JSONObject;
import com.fasterxml.jackson.core.type.TypeReference;
import com.fasterxml.jackson.databind.ObjectMapper;
/**
* Wrapper class around PMA.control API
*
* @author Yassine Iddaoui
*
*/
public class Control {
public enum PmaTrainingSessionRole {
SUPERVISOR, TRAINEE, OBSERVER
}
public enum PmaInteractionMode {
LOCKED, TEST_ACTIVE, REVIEW, CONSENSUS_VIEW, BROWSE, BOARD, CONSENSUS_SCORE_EDIT, SELF_REVIEW, SELF_TEST,
HIDDEN, CLINICAL_INFORMATION_EDIT
}
// for logging purposes
public static Logger logger = null;
/**
* This method is used to get version info from PMA.control instance running at
* pmacontrolURL
*
* @param pmaControlURL PMA Control's URL
* @return Version information
*/
public static String getVersionInfo(String pmaControlURL) {
// Get version info from PMA.control instance running at pmacontrolURL
// why? because GetVersionInfo can be invoked WITHOUT a valid SessionID;
// apiUrl() takes session information into account
String url = PMA.join(pmaControlURL, "api/version");
try {
String jsonString = PMA.httpGet(url, "application/json");
// we remove ""
return jsonString.substring(1, jsonString.length() - 1);
} catch (Exception e) {
e.printStackTrace();
if (logger != null) {
StringWriter sw = new StringWriter();
e.printStackTrace(new PrintWriter(sw));
logger.severe(sw.toString());
}
return null;
}
}
/**
* This method is used to retrieve a list of currently defined training sessions
* in PMA.control
*
* @param pmaControlURL URL for PMA.Control
* @param pmaCoreSessionID PMA.core session ID
* @return List of registred sessions
*/
private static JSONArray getTrainingSessions(String pmaControlURL, String pmaCoreSessionID) {
String url = PMA.join(pmaControlURL, "api/Sessions?sessionID=" + PMA.pmaQ(pmaCoreSessionID));
try {
String jsonString = PMA.httpGet(url, "application/json");
JSONArray jsonResponse = PMA.getJSONArrayResponse(jsonString);
return jsonResponse;
} catch (Exception e) {
e.printStackTrace();
if (logger != null) {
StringWriter sw = new StringWriter();
e.printStackTrace(new PrintWriter(sw));
logger.severe(sw.toString());
}
return null;
}
}
/**
* Helper method to convert a JSON representation of a PMA.control training
* session to a proper Java-esque structure
*
* @param session Session details
* @return Map of formatted session information
*/
@SuppressWarnings({ "unchecked", "serial" })
public static Map formatTrainingSessionProperly(JSONObject session) {
Map sessionData = new HashMap<>();
sessionData.put("Id", session.optInt("Id"));
sessionData.put("Title", session.optString("Title"));
sessionData.put("LogoPath", session.optString("LogoPath"));
sessionData.put("StartsOn", session.optString("StartsOn"));
sessionData.put("EndsOn", session.optString("EndsOn"));
sessionData.put("ProjectId", session.optInt("ProjectId"));
sessionData.put("State", session.optString("State"));
sessionData.put("CaseCollections", new HashMap>());
sessionData.put("NumberOfParticipants", session.optJSONObject("Participants").length());
JSONArray collections = session.getJSONArray("CaseCollections");
for (int i = 0; i < collections.length(); i++) {
JSONObject collection = collections.optJSONObject(i);
((Map>) sessionData.get("CaseCollections"))
.put(collection.optInt("CaseCollectionId"), new HashMap() {
{
put("Title", collection.optString("Title"));
put("Url", collection.optString("Url"));
}
});
}
return sessionData;
}
// /**
// * This method is used to Retrieve a dictionary with currently defined training
// * sessions in PMA.control. The resulting dictionary use the session's
// * identifier as the dictionary key, and therefore this method is easier to
// * quickly retrieve and represent session-related data. However, this method
// * returns less verbose data than getSessions()
// *
// * @param pmaControlURL URL for PMA.Control
// * @param pmaCoreSessionID PMA.core session ID
// * @return Map of data related to registred session IDs
// */
// public static Map> getSessionIds(String pmaControlURL, String pmaCoreSessionID) {
// JSONArray fullSessions = getSessions(pmaControlURL, pmaCoreSessionID);
// Map> newSession = new HashMap<>();
// for (int i = 0; i < fullSessions.length(); i++) {
// Map sessData = new HashMap();
// sessData.put("LogoPath", fullSessions.getJSONObject(i).getString("LogoPath"));
// sessData.put("StartsOn", fullSessions.getJSONObject(i).getString("StartsOn"));
// sessData.put("EndsOn", fullSessions.getJSONObject(i).getString("EndsOn"));
// sessData.put("ModuleId", fullSessions.getJSONObject(i).getString("ModuleId"));
// sessData.put("State", fullSessions.getJSONObject(i).getString("State"));
// newSession.put(fullSessions.getJSONObject(i).getString("Id"), sessData);
// }
// return newSession;
// }
/**
* This method is used to retrieve a dictionary with currently defined training
* sessions in PMA.control. The resulting dictionary use the training session's
* identifier as the dictionary key, and therefore this method is easier to
* quickly retrieve and represent session-related data.
*
* @param pmaControlURL URL for PMA.Control
* @param pmaControlProjectID Project ID
* @param pmaCoreSessionID PMA.core session ID
* @return Map of data related to registred session IDs
*/
public static Map> getTrainingSessions(String pmaControlURL,
Integer pmaControlProjectID, String pmaCoreSessionID) {
JSONArray fullTrainingSessions = getTrainingSessions(pmaControlURL, pmaCoreSessionID);
Map> newTrainingSessionMap = new HashMap<>();
for (int i = 0; i < fullTrainingSessions.length(); i++) {
JSONObject session = fullTrainingSessions.optJSONObject(i);
if ((pmaControlProjectID != null) || (pmaControlProjectID == session.optInt("ProjectId"))) {
newTrainingSessionMap.put(session.optInt("Id"), formatTrainingSessionProperly(session));
}
}
return newTrainingSessionMap;
}
/**
* This method is used to get sessions for a certain participant
*
* @param pmaControlURL URL for PMA.Control
* @param participantUsername PMA.core username
* @param pmaCoreSessionID PMA.core session ID
* @return Map of sessions for a certain participant
*/
public static Map> getTrainingSessionsForParticipant(String pmaControlURL,
String participantUsername, String pmaCoreSessionID) {
JSONArray fullTrainingSessions = getTrainingSessions(pmaControlURL, pmaCoreSessionID);
Map> newTrainingSessionMap = new HashMap<>();
for (int i = 0; i < fullTrainingSessions.length(); i++) {
JSONObject session = fullTrainingSessions.optJSONObject(i);
JSONObject participants = session.optJSONObject("Participants");
for (String key : participants.keySet()) {
if (key.toLowerCase().equals(participantUsername.toLowerCase())) {
Map sMap = formatTrainingSessionProperly(session);
sMap.put("Role", participants.get(key));
newTrainingSessionMap.put(session.getInt("Id"), sMap);
}
}
}
return newTrainingSessionMap;
}
/**
* This method is used to extract the participants in a particular session
*
* @param pmaControlURL URL for PMA.Control
* @param pmaControlSessionID Training session ID
* @param pmaCoreSessionID PMA.core session ID
* @return Map of participants in a particular session
*/
public static Map getTrainingSessionParticipants(String pmaControlURL,
Integer pmaControlSessionID, String pmaCoreSessionID) {
String url = PMA.join(pmaControlURL,
"api/Sessions/" + pmaControlSessionID + "/Participants?sessionID=" + PMA.pmaQ(pmaCoreSessionID));
try {
String jsonString = PMA.httpGet(url, "application/json");
JSONArray sessionParticipants = PMA.getJSONArrayResponse(jsonString);
Map participants = new HashMap<>();
for (int i = 0; i < sessionParticipants.length(); i++) {
JSONObject sessionParticipant = sessionParticipants.optJSONObject(i);
participants.put(sessionParticipant.optString("User"), sessionParticipant);
}
return participants;
} catch (Exception e) {
e.printStackTrace();
if (logger != null) {
StringWriter sw = new StringWriter();
e.printStackTrace(new PrintWriter(sw));
logger.severe(sw.toString());
}
return null;
}
}
/**
* This method is used to check if a specific user participates in a specific
* session
*
* @param pmaControlURL URL for PMA.Control
* @param participantUsername PMA.core username
* @param pmaControlSessionID Training session ID
* @param pmaCoreSessionID PMA.core session ID
* @return True if a specific user participates in a specific session, false
* otherwise.
*/
public static Boolean isParticipantInTrainingSession(String pmaControlURL, String participantUsername,
Integer pmaControlSessionID, String pmaCoreSessionID) {
Map allParticipants = getTrainingSessionParticipants(pmaControlURL, pmaControlSessionID,
pmaCoreSessionID);
return allParticipants.containsKey(participantUsername);
}
/**
* This method is used to construct the session URL
*
* @param pmaControlCase Case name
* @throws Exception
*/
/**
*
* @param pmaControlURL URL for PMA.Control
* @param participantSessionID The participant's session ID to allow for
* eventual impersonation
* @param participantUsername participant username
* @param pmaControlSessionID Training session ID
* @param pmaControlCaseCollectionID Case collection ID
* @param pmaCoreSessionID PMA.core session ID, the administrative
* session ID to verify that the participant
* is registered for the training session
* @return Session's URL
* @throws Exception If participantUsername isn't registred for this session
*/
@SuppressWarnings("unchecked")
public static String getTrainingSessionURL(String pmaControlURL, String participantSessionID,
String participantUsername, Integer pmaControlSessionID, Integer pmaControlCaseCollectionID,
String pmaCoreSessionID) throws Exception {
if (isParticipantInTrainingSession(pmaControlURL, participantUsername, pmaControlSessionID, pmaCoreSessionID)) {
for (Entry> entry : ((Map>) getTrainingSession(
pmaControlURL, pmaControlSessionID, pmaCoreSessionID).get("CaseCollections")).entrySet()) {
if (entry.getKey() == pmaControlCaseCollectionID) {
return entry.getValue().get("Url").toString() + "?sessionID=" + participantSessionID;
}
}
} else {
throw new Exception("Participant " + participantUsername + " is not registered for this session");
}
return null;
}
/**
* This method is used to get all participants registered across all sessions
*
* @param pmaControlURL PMA.control URL
* @param pmaCoreSessionID PMA.core session ID
* @return Map of all participants registered across all sessions
*/
public static Map> getAllParticipants(String pmaControlURL, String pmaCoreSessionID) {
JSONArray fullTrainingSessions = getTrainingSessions(pmaControlURL, pmaCoreSessionID);
Map> userMap = new HashMap<>();
for (int i = 0; i < fullTrainingSessions.length(); i++) {
JSONObject session = fullTrainingSessions.optJSONObject(i);
Map sMap = formatTrainingSessionProperly(session);
JSONArray participants = session.optJSONArray("Participants");
for (int j = 0; j < participants.length(); j++) {
String participant = participants.optString(j);
if (!userMap.containsKey(participant)) {
userMap.put(participant, new HashMap());
}
((Map) userMap.get(participant)).put((int) sMap.get("Id"),
sMap.get("Title").toString());
}
}
return userMap;
}
/**
* This method is used to register a participant for a session, assign a
* specific role
*
* @param pmaControlURL PMA.control URL
* @param participantUsername PMA.core username
* @param pmaControlSessionID Training session ID
* @param pmaControlRole Role
* @param pmaCoreSessionID PMA.core session ID
* @return URL connection output in JSON format
* @throws Exception If user is ALREADY registered in the provided PMA.control
* training session
*/
public static String registerParticipantForTrainingSession(String pmaControlURL, String participantUsername,
Integer pmaControlSessionID, PmaTrainingSessionRole pmaControlRole, String pmaCoreSessionID)
throws Exception {
if (isParticipantInTrainingSession(pmaControlURL, participantUsername, pmaControlSessionID, pmaCoreSessionID)) {
throw new Exception("PMA.core user " + participantUsername
+ " is ALREADY registered in PMA.control training session " + pmaControlSessionID);
}
try {
String url = PMA.join(pmaControlURL, "api/Sessions/") + pmaControlSessionID.toString()
+ "/AddParticipant?SessionID=" + pmaCoreSessionID;
URL urlResource = new URL(url);
HttpURLConnection con;
if (url.startsWith("https")) {
con = (HttpsURLConnection) urlResource.openConnection();
} else {
con = (HttpURLConnection) urlResource.openConnection();
}
con.setRequestMethod("POST");
con.setRequestProperty("Content-Type", "application/json");
con.setUseCaches(false);
con.setDoOutput(true);
String data = "{ \"UserName\": \"" + participantUsername + "\", \"Role\": \"" + pmaControlRole + "\" }"; // default
// interaction
// mode
// =
// Locked
// + ", \"InteractionMode\": \"" +
// String.valueOf(pmacontrolInteractionMode.ordinal() + 1) + "\" }";
OutputStream os = con.getOutputStream();
os.write(data.getBytes("UTF-8"));
os.close();
String jsonString = PMA.getJSONAsStringBuffer(con).toString();
PMA.clearURLCache();
return jsonString;
} catch (Exception e) {
e.printStackTrace();
if (logger != null) {
StringWriter sw = new StringWriter();
e.printStackTrace(new PrintWriter(sw));
logger.severe(sw.toString());
}
return null;
}
}
/**
* This method is used to a ssign an interaction mode to a particpant for a
* given Case Collection within a training session
*
* @param pmaControlURL PMA.control URL
* @param participantUsername PMA.core username
* @param pmaControlSessionID Training session ID
* @param pmaControlCaseCollectionID Case collection ID
* @param pmaControlInteractionMode Interaction mode
* @param pmaCoreSessionID PMA.core session ID
* @return URL connection output in JSON format
* @throws Exception If user is NOT registered in the provided PMA.control
* training session
*/
public static String setParticipantInteractionMode(String pmaControlURL, String participantUsername,
Integer pmaControlSessionID, Integer pmaControlCaseCollectionID,
PmaInteractionMode pmaControlInteractionMode, String pmaCoreSessionID) throws Exception {
if (!isParticipantInTrainingSession(pmaControlURL, participantUsername, pmaControlSessionID,
pmaCoreSessionID)) {
throw new Exception("PMA.core user " + participantUsername
+ " is NOT registered in PMA.control training session " + pmaControlSessionID);
}
try {
String url = PMA.join(pmaControlURL, "api/Sessions/") + pmaControlSessionID + "/InteractionMode?SessionID="
+ pmaCoreSessionID;
URL urlResource = new URL(url);
HttpURLConnection con;
if (url.startsWith("https")) {
con = (HttpsURLConnection) urlResource.openConnection();
} else {
con = (HttpURLConnection) urlResource.openConnection();
}
con.setRequestMethod("POST");
con.setRequestProperty("Content-Type", "application/json");
con.setUseCaches(false);
con.setDoOutput(true);
String data = "{ \"UserName\": \"" + participantUsername + "\", " + " \"CaseCollectionId\": \""
+ pmaControlCaseCollectionID + "\", " + "\"InteractionMode\": \"" + pmaControlInteractionMode
+ "\" }"; // default interaction mode = Locked
// + ", \"InteractionMode\": \"" +
// String.valueOf(pmacontrolInteractionMode.ordinal() + 1) + "\" }";
OutputStream os = con.getOutputStream();
os.write(data.getBytes("UTF-8"));
os.close();
String jsonString = PMA.getJSONAsStringBuffer(con).toString();
PMA.clearURLCache();
return jsonString;
} catch (Exception e) {
e.printStackTrace();
if (logger != null) {
StringWriter sw = new StringWriter();
e.printStackTrace(new PrintWriter(sw));
logger.severe(sw.toString());
}
return null;
}
}
/**
* This method is an overload of previous one. We created to add the possibility
* to assign an interaction mode outside the defined enum values
*
* @param pmaControlURL PMA.control URL
* @param participantUsername PMA.core username
* @param pmaControlSessionID Training session ID
* @param pmaControlCaseCollectionID Case collection ID
* @param pmaControlInteractionMode Interaction mode
* @param pmaCoreSessionID PMA.core session ID
* @return URL connection output in JSON format
* @throws Exception If user is NOT registered in the provided PMA.control
* training session
*/
public static String setParticipantInteractionMode(String pmaControlURL, String participantUsername,
Integer pmaControlSessionID, Integer pmaControlCaseCollectionID, Integer pmaControlInteractionMode,
String pmaCoreSessionID) throws Exception {
if (!isParticipantInTrainingSession(pmaControlURL, participantUsername, pmaControlSessionID,
pmaCoreSessionID)) {
throw new Exception("PMA.core user " + participantUsername
+ " is NOT registered in PMA.control training session " + pmaControlSessionID);
}
try {
String url = PMA.join(pmaControlURL, "api/Sessions/") + pmaControlSessionID + "/InteractionMode?SessionID="
+ pmaCoreSessionID;
URL urlResource = new URL(url);
HttpURLConnection con;
if (url.startsWith("https")) {
con = (HttpsURLConnection) urlResource.openConnection();
} else {
con = (HttpURLConnection) urlResource.openConnection();
}
con.setRequestMethod("POST");
con.setRequestProperty("Content-Type", "application/json");
con.setUseCaches(false);
con.setDoOutput(true);
String data = "{ \"UserName\": \"" + participantUsername + "\", " + " \"CaseCollectionId\": \""
+ pmaControlCaseCollectionID + "\", " + "\"InteractionMode\": \"" + pmaControlInteractionMode
+ "\" }"; // default interaction mode = Locked
// + ", \"InteractionMode\": \"" +
// String.valueOf(pmacontrolInteractionMode.ordinal() + 1) + "\" }";
OutputStream os = con.getOutputStream();
os.write(data.getBytes("UTF-8"));
os.close();
String jsonString = PMA.getJSONAsStringBuffer(con).toString();
PMA.clearURLCache();
return jsonString;
} catch (Exception e) {
e.printStackTrace();
if (logger != null) {
StringWriter sw = new StringWriter();
e.printStackTrace(new PrintWriter(sw));
logger.severe(sw.toString());
}
return null;
}
}
/**
* This method is used to retrieve sessions (possibly filtered by project ID),
* titles only
*
* @param pmaControlURL URL for PMA.Control
* @param pmaControlProjectID Project's ID
* @param pmaCoreSessionID PMA.core session ID
* @return List of session titles
*/
public static List getTrainingSessionTitles(String pmaControlURL, Integer pmaControlProjectID,
String pmaCoreSessionID) {
try {
return new ArrayList(
getTrainingSessionTitlesDict(pmaControlURL, pmaControlProjectID, pmaCoreSessionID).values());
} catch (Exception e) {
e.printStackTrace();
if (logger != null) {
StringWriter sw = new StringWriter();
e.printStackTrace(new PrintWriter(sw));
logger.severe(sw.toString());
}
return null;
}
}
/**
* This method is used to retrieve (training) sessions (possibly filtered by
* project ID), returns a map of session IDs and titles
*
* @param pmaControlURL URL for PMA.Control
* @param pmaControlProjectID Project's ID
* @param pmaCoreSessionID PMA.core session ID
* @return Map of session IDs and titles
*/
public static Map getTrainingSessionTitlesDict(String pmaControlURL, Integer pmaControlProjectID,
String pmaCoreSessionID) {
Map map = new HashMap<>();
try {
JSONArray all = getTrainingSessions(pmaControlURL, pmaCoreSessionID);
for (int i = 0; i < all.length(); i++) {
JSONObject session = all.optJSONObject(i);
if (pmaControlProjectID == null) {
map.put(session.optInt("Id"), session.optString("Title"));
} else if (pmaControlProjectID == session.optInt("ProjectId")) {
map.put(session.optInt("Id"), session.optString("Title"));
}
}
return map;
} catch (Exception e) {
e.printStackTrace();
if (logger != null) {
StringWriter sw = new StringWriter();
e.printStackTrace(new PrintWriter(sw));
logger.severe(sw.toString());
}
return null;
}
}
/**
* This method is used to get the first (training) session with ID =
* pmaControlSessionID
*
* @param pmaControlURL URL for PMA.Control
* @param pmaControlSessionID Training session's ID
* @param pmaCoreSessionID PMA.core session ID
* @return First training session with ID = pmacontrolSessionID
*/
public static Map getTrainingSession(String pmaControlURL, Integer pmaControlSessionID,
String pmaCoreSessionID) {
JSONArray allTrainingSessions = getTrainingSessions(pmaControlURL, pmaCoreSessionID);
for (int i = 0; i < allTrainingSessions.length(); i++) {
JSONObject el = allTrainingSessions.optJSONObject(i);
if (el.optInt("Id") == pmaControlSessionID) {
// summarize training session-related information so that it makes sense
return formatTrainingSessionProperly(el);
}
}
return null;
}
/**
* This method is used to return the first (training) session that has keyword
* as part of its string; search is case insensitive
*
* @param pmaControlURL URL for PMA.Control
* @param keyword key word to search against
* @param pmaCoreSessionID PMA.core session ID
* @return Map of the first (training) session whose title matches the search
* criteria
*/
public static Map searchTrainingSession(String pmaControlURL, String keyword,
String pmaCoreSessionID) {
JSONArray all = getTrainingSessions(pmaControlURL, pmaCoreSessionID);
for (int i = 0; i < all.length(); i++) {
JSONObject el = all.optJSONObject(i);
if (el.optString("Title").toLowerCase().contains(keyword.toLowerCase())) {
return formatTrainingSessionProperly(el);
}
}
return null;
}
/**
* This method is used to retrieve all the data for all the defined case
* collections in PMA.control (RAW JSON data; not suited for human consumption)
*
* @param pmaControlURL URL for PMA.Control
* @param pmaCoreSessionID PMA.core session ID
* @param varargs Array of optional arguments
*
* project : First optional argument(String), default
* value(null), project case collections belong to
*
* @return Array of case collections
*/
private static JSONArray getCaseCollections(String pmaControlURL, String pmaCoreSessionID, String... varargs) {
// setting the default value when argument's value is omitted
String project = ((varargs.length > 0) && (varargs[0] != null)) ? varargs[0] : "";
String url = PMA.join(pmaControlURL, "api/CaseCollections?sessionID=" + PMA.pmaQ(pmaCoreSessionID)
+ ((project.length() > 0) ? ("&project=" + PMA.pmaQ(project)) : ""));
try {
String jsonString = PMA.httpGet(url, "application/json");
JSONArray jsonResponse = PMA.getJSONArrayResponse(jsonString);
return jsonResponse;
} catch (Exception e) {
e.printStackTrace();
if (logger != null) {
StringWriter sw = new StringWriter();
e.printStackTrace(new PrintWriter(sw));
logger.severe(sw.toString());
}
return null;
}
}
/**
* This method is used to retrieve case collections (possibly filtered by
* project ID), titles only
*
* @param pmaControlURL URL for PMA.Control
* @param pmaControlProjectID Project's ID
* @param pmaCoreSessionID PMA.core session ID
* @return List of case collections titles
*/
public static List getCaseCollectionTitles(String pmaControlURL, Integer pmaControlProjectID,
String pmaCoreSessionID) {
try {
return new ArrayList(
getCaseCollectionTitlesDict(pmaControlURL, pmaControlProjectID, pmaCoreSessionID).values());
} catch (Exception e) {
e.printStackTrace();
if (logger != null) {
StringWriter sw = new StringWriter();
e.printStackTrace(new PrintWriter(sw));
logger.severe(sw.toString());
}
return null;
}
}
/**
* This method is used to get a case collection by its title
*
* @param pmaControlURL URL for PMA.Control
* @param pmaCoreSessionID PMA.core session ID
* @param caseCollectionTitle The case collection's title
* @return The case collection in json object format
*/
public static JSONObject getCaseCollectionByTitle(String pmaControlURL, String pmaCoreSessionID,
String caseCollectionTitle) {
JSONArray caseCollections = getCaseCollections(pmaControlURL, pmaCoreSessionID);
if (caseCollections == null) {
return null;
} else {
for (int i = 0; i < caseCollections.length(); i++) {
if (caseCollections.optJSONObject(i).get("Title").toString().equals(caseCollectionTitle)) {
return caseCollections.optJSONObject(i);
}
}
// The case collection wasn't found!
return null;
}
}
/**
* This method is used to retrieve case collections (possibly filtered by
* project ID), returns a map of case collection IDs and titles
*
* @param pmaControlURL URL for PMA.Control
* @param pmaControlProjectID Project's ID
* @param pmaCoreSessionID PMA.core session ID
* @return Map of case collection IDs and titles
*/
public static Map getCaseCollectionTitlesDict(String pmaControlURL, Integer pmaControlProjectID,
String pmaCoreSessionID) {
Map map = new HashMap<>();
try {
JSONArray allColletions = getCaseCollections(pmaControlURL, pmaCoreSessionID);
for (int i = 0; i < allColletions.length(); i++) {
JSONObject collection = allColletions.optJSONObject(i);
if (pmaControlProjectID == null) {
map.put(collection.optInt("Id"), collection.optString("Title"));
} else if (pmaControlProjectID == collection.optInt("ProjectId")) {
map.put(collection.optInt("Id"), collection.optString("Title"));
}
}
return map;
} catch (Exception e) {
e.printStackTrace();
if (logger != null) {
StringWriter sw = new StringWriter();
e.printStackTrace(new PrintWriter(sw));
logger.severe(sw.toString());
}
return null;
}
}
/**
* This method is used to get a case collection details (by ID)
*
* @param pmaControlURL URL for PMA.Control
* @param pmaControlCaseCollectionID Case collection's ID
* @param pmaCoreSessionID PMA.core session ID
* @return The case collection in json object format
*/
public static JSONObject getCaseCollection(String pmaControlURL, Integer pmaControlCaseCollectionID,
String pmaCoreSessionID) {
JSONArray allCollections = getCaseCollections(pmaControlURL, pmaCoreSessionID);
for (int i = 0; i < allCollections.length(); i++) {
JSONObject collection = allCollections.optJSONObject(i);
if (collection.optInt("Id") == pmaControlCaseCollectionID) {
return collection;
}
}
// Case collection not found
return null;
}
/**
* This method is used to retrieve cases for a specific collection
*
* @param pmaControlURL PMA.control URL
* @param pmaControlCaseCollectionID Case collection ID
* @param pmaCoreSessionID PMA.core session ID
* @return List of cases for a specific collection
*/
public static JSONArray getCaseForCaseCollection(String pmaControlURL, Integer pmaControlCaseCollectionID,
String pmaCoreSessionID) {
return getCaseCollection(pmaControlURL, pmaControlCaseCollectionID, pmaCoreSessionID).optJSONArray("Cases");
}
/**
* This method is used to return the first collection that has keyword as part
* of its string; search is case insensitive
*
* @param pmaControlURL URL for PMA.Control
* @param keyword Keyword to seach collections against
* @param pmaCoreSessionID PMA.core session ID
* @return The first collection that matches the search criteria
*/
public static JSONObject searchCaseCollection(String pmaControlURL, String keyword, String pmaCoreSessionID) {
JSONArray allCollections = getCaseCollections(pmaControlURL, pmaCoreSessionID);
for (int i = 0; i < allCollections.length(); i++) {
JSONObject collection = allCollections.optJSONObject(i);
if (collection.getString("Title").toLowerCase().contains(keyword.toLowerCase())) {
// summary session-related information so that it makes sense
return collection;
}
}
return null;
}
/**
* Helper method to convert a list of sessions with default arguments into a
* summarized dictionary
*
* @param originalProjectSessions The original project sessions prior to
* formatting
* @return List of summarized maps of the project's sessions in ID, Title format
*/
@SuppressWarnings("serial")
private static List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy