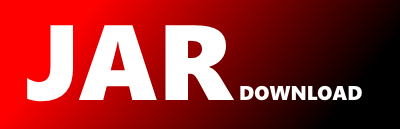
com.pathomation.UI.UI Maven / Gradle / Ivy
Show all versions of pma-java Show documentation
package com.pathomation.UI;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import java.util.logging.Logger;
import com.pathomation.Core;
/**
* Wrapper class around PMA.UI JavaScript framework
*
* @author Yassine Iddaoui
*
*/
public class UI {
public static String pmaStartUIJavascriptPath = "http://localhost:54001/Scripts/pmaui/";
public static String pmaUIJavascriptPath = "pma.ui/";
public static boolean pmaUIFrameworkEmbedded = false;
public static int pmaUIViewportCount = 0;
public static List pmaUIViewports = new ArrayList<>();
public static int pmaUIGalleryCount = 0;
public static List pmaUIGalleries = new ArrayList<>();
public static int pmaUILoaderCount = 0;
public static List pmaUILoaders = new ArrayList<>();
// for logging purposes
public static Logger logger = null;
/**
* Internal helper function to prevent PMA.UI framework from being loaded more
* than once
*
* @param sessionID session ID of the actual session
* @return The links related to JS and CSS files to be included
*/
public static String pmaEmbedPmaUIFramework(String sessionID) {
if (!pmaUIFrameworkEmbedded) {
if (!pmaStartUIJavascriptPath.endsWith("/")) {
pmaUIJavascriptPath += "/";
}
}
String htmlCode = "\n" + "\n" + "\n"
+ "\n" + "\n" + "\n"
+ "\n";
// System.out.print("\n");
// System.out.print(
// "\n");
// System.out.print(
// "\n");
// System.out.print("\n");
// System.out.print("\n");
// System.out.print("\n");
// System.out.print(
// "");
pmaUIFrameworkEmbedded = true;
return htmlCode;
}
/**
* Output HTML code to display a single slide through a PMA.UI viewport control
* authentication against PMA.core happens through a pre-established
*
* @param server PMA.Start or PMA.core instance
* @param slideRef path to the slide
* @param sessionID session id of the actual session
* @param varargs Array of optional arguments
*
* options : First optional argument(Map{@literal <}String,
* String{@literal >}), default value(null), Map of the options
* defining the display of the view port
*
* @return List of embed slides
*/
@SuppressWarnings("unchecked")
public static List embedSlideBySessionID(String server, String slideRef, String sessionID,
Map... varargs) {
// setting the default value when argument's value is omitted
Map options = varargs.length > 0 ? varargs[0] : null;
// pmaEmbedPmaUIFramework(sessionID);
pmaUIViewportCount++;
String viewPortID = "pma_viewport" + pmaUIViewportCount;
pmaUIViewports.add(viewPortID);
String htmlCode = pmaEmbedPmaUIFramework(sessionID) + "\n"
+ "";
List results = new ArrayList<>();
results.add(htmlCode);
results.add(viewPortID);
return results;
// return htmlCode;
// return viewPortID;
}
/**
* Output HTML code to display a single slide through a PMA.UI viewport control
* authentication against PMA.core happens in real-time through the provided
* $username and $password credentials Note that the username and password and
* NOT rendered in the HTML output (authentication happens on the server-side).
*
* @param server PMA.Start or PMA.core instance
* @param slideRef path to the slide
* @param username credentials' username
* @param varargs Array of optional arguments
*
* password : First optional argument(String), default
* value(""), credentials' password
*
*
* options : Second optional argument(Map{@literal <}String,
* String{@literal >}), default value(null), Map of the options
* defining the display of the view port
*
* @return List of embed slides by username
*/
@SuppressWarnings("unchecked")
public static List embedSlideByUsername(String server, String slideRef, String username,
Object... varargs) {
// setting the default values when arguments' values are omitted
String password = "";
Map options = null;
if (varargs.length > 0) {
if (!(varargs[0] instanceof String) && varargs[0] != null) {
if (logger != null) {
logger.severe("embedSlideByUsername() : Illegal argument");
}
throw new IllegalArgumentException("...");
}
password = (String) varargs[0];
}
if (varargs.length > 1) {
if (!(varargs[1] instanceof Map, ?>) && varargs[1] != null) {
if (logger != null) {
logger.severe("embedSlideByUsername() : Illegal argument");
}
throw new IllegalArgumentException("...");
}
options = (Map) varargs[1];
}
String session = Core.connect(server, username, password);
return embedSlideBySessionID(server, slideRef, session, options);
}
/**
* Output HTML code to display a gallery that shows all thumbnails that exist in
* a specific folder hosted by the specified PMA.core instance authentication
* against PMA.core happens through a pre-established SessionID
*
* @param server PMA.Start or PMA.core instance
* @param path folder containing the slides
* @param sessionID session id of the actual session
* @param varargs Array of optional arguments
*
* options : First optional argument(Map{@literal <}String,
* String{@literal >}), default value(null), Map of the options
* defining the display of the view port
*
* @return List of embed galleries by session ID
*/
@SuppressWarnings("unchecked")
public static List embedGalleryBySessionID(String server, String path, String sessionID,
Map... varargs) {
// setting the default value when argument's value is omitted
Map options = varargs.length > 0 ? varargs[0] : null;
pmaUIGalleryCount++;
String galleryID = "pma_gallery" + pmaUIGalleryCount;
pmaUIGalleries.add(galleryID);
String htmlCode = pmaEmbedPmaUIFramework(sessionID) + "\n"
+ "";
List results = new ArrayList<>();
results.add(htmlCode);
results.add(galleryID);
return results;
}
/**
* Output HTML code to display a gallery that shows all thumbnails that exist in
* a specific folder hosted by the specified PMA.core instance authentication
* against PMA.core happens in real-time through the provided $username and
* $password credentials Note that the username and password are NOT rendered in
* the HTML output (authentication happens on the server-side). * @param server
* PMA.Start of PMA.core
*
* @param server PMA.Start or PMA.core instance
* @param path folder containing the slides
* @param username credentials' username
* @param varargs Array of optional arguments
*
* password : First optional argument(String), default
* value(""), credentials' password
*
*
* options : Second optional argument(Map{@literal <}String,
* String{@literal >}), default value(null), Map of the options
* defining the display of the view port
*
* @return List of embed galleries by username
*/
@SuppressWarnings("unchecked")
public static List embedGalleryByUsername(String server, String path, String username, Object... varargs) {
// setting the default values when arguments' values are omitted
String password = "";
Map options = null;
if (varargs.length > 0) {
if (!(varargs[0] instanceof String) && varargs[0] != null) {
if (logger != null) {
logger.severe("embedGalleryByUsername() : Illegal argument");
}
throw new IllegalArgumentException("...");
}
password = (String) varargs[0];
}
if (varargs.length > 1) {
if (!(varargs[1] instanceof Map, ?>) && varargs[1] != null) {
if (logger != null) {
logger.severe("embedGalleryByUsername() : Illegal argument");
}
throw new IllegalArgumentException("...");
}
options = (Map) varargs[1];
}
String session = Core.connect(server, username, password);
return embedGalleryBySessionID(server, path, session, options);
}
/**
* Output HTML code to couple an earlier instantiated PMA.UI gallery to a PMA.UI
* viewport. The PMA.UI viewport can be instantiated earlier, or not at all
*
* @param galleryDiv HTML Div holding the gallery
* @param viewportDiv HTML Div holding the viewport
* @return Html code generated couple an earlier instantiated PMA.UI gallery to
* a PMA.UI viewport
* @throws Exception if galleryDiv is not a PMA.UI gallery or galleryDiv is not
* a valid PMA.UI gallery container or viewportDiv is not a
* PMA.UI viewport
*/
public static String linkGalleryToViewport(String galleryDiv, String viewportDiv) throws Exception {
// verify the validity of the galleryDiv argument
if (pmaUIViewports.contains(galleryDiv)) {
if (logger != null) {
logger.severe(
"galleryDiv is not a PMA.UI gallery (it's actually a viewport; did you switch the arguments up?)");
}
throw new Exception(
"galleryDiv is not a PMA.UI gallery (it's actually a viewport; did you switch the arguments up?)");
}
if (!pmaUIGalleries.contains(galleryDiv)) {
if (logger != null) {
logger.severe("galleryDiv is not a valid PMA.UI gallery container");
}
throw new Exception("galleryDiv is not a valid PMA.UI gallery container");
}
// verify the validity of the $viewportDiv argument
if (pmaUIGalleries.contains(viewportDiv)) {
if (logger != null) {
logger.severe(
"viewportDiv is not a PMA.UI viewport (it's actually a gallery; did you switch the arguments up?)");
}
throw new Exception(
"viewportDiv is not a PMA.UI viewport (it's actually a gallery; did you switch the arguments up?)");
}
pmaUILoaderCount++;
String loaderID = "pma_slideLoader" + pmaUILoaderCount;
pmaUILoaders.add(loaderID);
String htmlCode = "";
if (!pmaUIViewports.contains(viewportDiv)) {
// viewport container doesn't yet exist, but this doesn't have to be a
// showstopper; just create it on the fly
pmaUIViewports.add(viewportDiv);
pmaUIViewportCount++;
htmlCode = "\n";
}
htmlCode += "";
return htmlCode;
}
}