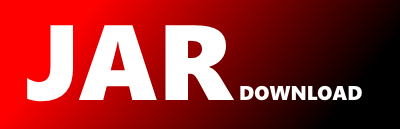
com.payintech.smoney.SMoneyService Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of s-money-client Show documentation
Show all versions of s-money-client Show documentation
S-Money API client for Java and Android
The newest version!
/*
* The MIT License (MIT)
*
* Copyright (c) 2013 - 2016 PayinTech
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in all
* copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
* SOFTWARE.
*/
package com.payintech.smoney;
import com.payintech.smoney.entity.*;
import okhttp3.RequestBody;
import retrofit2.Call;
import retrofit2.http.*;
import java.util.List;
import java.util.Map;
/**
* SMoneyService.
*
* @author Pierre Adam
* @author Jean-Pierre Boudic
* @author Thibault Meyer
* @version 16.09.13
* @since 15.11.01
*/
public interface SMoneyService {
/**
* Create a new user. The field User::id was generated by API.
* Define Type for Account type (normal, Pro)
* Field to fill on the user :
*
* - AppUserId (required)
* - Type (required)
* - Profile (required)
* --- Civility (optional)
* --- FirstName (required)
* --- LastName (required)
* --- Birthdate (required)
* --- PhoneNumber (optional)
* --- Email (optional)
* --- Alias (optional)
* --- Address (required)
* ----- Street (optional)
* ----- ZipCode (optional)
* ----- City (optional)
* ----- Country (required)
* - Company (required when type == 2)
* --- Name (required)
* --- Siret (required)
*
*
* @param user A new user (local instance)
* @return The newly created user as {@code UserEntity}
* @since 15.11.01
*/
@Headers({
"Accept: application/vnd.s-money.v1+json",
"Content-Type: application/vnd.s-money.v1+json"
})
@POST("users")
Call createUser(@Body UserEntity user);
/**
* List the first 50 registered users.
*
* @return A list of {@code UserEntity}
* @since 15.11.01
*/
@Headers("Accept: application/vnd.s-money.v1+json")
@GET("users")
Call> listUsers();
/**
* List registered users. By default, API return 50 results per page.
*
* @param pageNumber The page to retrieve
* @return A list of {@code UserEntity}
* @since 16.01.01
*/
@Headers("Accept: application/vnd.s-money.v1+json")
@GET("users")
Call> listUsers(@Query("page") Integer pageNumber);
/**
* List registered users.
*
* @param pageNumber The page to retrieve
* @param itemPerPage The number of items per page
* @return A list of {@code UserEntity}
* @since 16.01.01
*/
@Headers("Accept: application/vnd.s-money.v1+json")
@GET("users")
Call> listUsers(@Query("page") Integer pageNumber, @Query("perpage") Integer itemPerPage);
/**
* Get an user identified by its 3rd party client ID (appUserId).
*
* @param appUserId 3rd party user ID
* @return The requested user as {@code UserEntity}
* @since 15.11.01
*/
@Headers("Accept: application/vnd.s-money.v1+json")
@GET("users/{appuserid}")
Call getUser(@Path("appuserid") String appUserId);
/**
* Update an existing user. Only profile data can be updated.
*
* @param appUserId 3rd party user ID
* @param user User to update with new informatio
* @return The updated user updated as {@code UserEntity}
* @since 15.11.01
*/
@Headers({
"Accept: application/vnd.s-money.v1+json",
"Content-Type: application/vnd.s-money.v1+json"
})
@PUT("users/{appuserid}")
Call updateUser(@Path("appuserid") String appUserId, @Body UserEntity user);
/**
* Find users by criteria. Possible keys in the dictionary are:
*
* - firstname (optional)
* - lastname (optional)
* - email (optional)
*
*
* @param userOptions Dictionary of criteria
* @return A list of {@code UserEntity}
* @since 15.11.01
*/
@Headers("Accept: application/vnd.s-money.v1+json")
@GET("users/search")
Call> findUsers(@QueryMap Map userOptions);
/**
* Find users by criteria. Possible keys in the dictionary are:
*
* - firstname (optional)
* - lastname (optional)
* - email (optional)
*
*
* @param userOptions Dictionary of criteria
* @param pageNumber The page to retrieve
* @return A list of {@code UserEntity}
* @since 16.01.01
*/
@Headers("Accept: application/vnd.s-money.v1+json")
@GET("users/search")
Call> findUsers(@QueryMap Map userOptions, @Query("page") Integer pageNumber);
/**
* Find users by criteria. Possible keys in the dictionary are:
*
* - firstname (optional)
* - lastname (optional)
* - email (optional)
*
*
* @param userOptions Dictionary of criteria
* @param pageNumber The page to retrieve
* @param itemPerPage The number of items per page
* @return A list of {@code UserEntity}
* @since 16.01.01
*/
@Headers("Accept: application/vnd.s-money.v1+json")
@GET("users/search")
Call> findUsers(@QueryMap Map userOptions, @Query("page") Integer pageNumber, @Query("perpage") Integer itemPerPage);
/**
* List first 50 sub accounts of an user.
*
* @param appUserId 3rd party user ID
* @return A list of {@code SubAccountEntity}
* @since 15.11.01
*/
@Headers("Accept: application/vnd.s-money.v1+json")
@GET("users/{appuserid}/subaccounts")
Call> listSubAccounts(@Path("appuserid") String appUserId);
/**
* List sub accounts of an user.
*
* @param appUserId 3rd party user ID
* @param pageNumber The page to retrieve
* @return A list of {@code SubAccountEntity}
* @since 16.01.01
*/
@Headers("Accept: application/vnd.s-money.v1+json")
@GET("users/{appuserid}/subaccounts")
Call> listSubAccounts(@Path("appuserid") String appUserId, @Query("page") Integer pageNumber);
/**
* List sub accounts of an user.
*
* @param appUserId 3rd party user ID
* @param pageNumber The page to retrieve
* @param itemPerPage The number of items per page
* @return A list of {@code SubAccountEntity}
* @since 16.01.01
*/
@Headers("Accept: application/vnd.s-money.v1+json")
@GET("users/{appuserid}/subaccounts")
Call> listSubAccounts(@Path("appuserid") String appUserId, @Query("page") Integer pageNumber, @Query("perpage") Integer itemPerPage);
/**
* Get a sub accounts of an user.
*
* @param appUserId 3rd party user ID
* @param appAccountId The Account Id
* @return The requested sub account as {@code SubAccountEntity}
* @since 15.11.01
*/
@Headers("Accept: application/vnd.s-money.v1+json")
@GET("users/{appuserid}/subaccounts/{appaccountid}")
Call getSubAccount(@Path("appuserid") String appUserId, @Path("appaccountid") String appAccountId);
/**
* Create a sub account for an user.
* Field to fill on the subAccount :
*
* - AppAccountId (required)
* - DisplayName (optional)
*
*
* @param appUserId 3rd party user ID
* @param subAccount The sub account information
* @return The newly created sub account as {@code SubAccountEntity}
* @since 15.11.01
*/
@Headers({
"Accept: application/vnd.s-money.v1+json",
"Content-Type: application/vnd.s-money.v1+json"
})
@POST("users/{appuserid}/subaccounts")
Call createSubAccount(@Path("appuserid") String appUserId, @Body SubAccountEntity subAccount);
/**
* Update a sub account for an user.
* Field to fill on the subAccount :
*
* - DisplayName
*
*
* @param appUserId 3rd party user ID
* @param appAccountId 3rd party account ID
* @param subAccount The sub account information
* @return The updated sub account as {@code SubAccountEntity}
* @since 15.11.01
*/
@Headers({
"Accept: application/vnd.s-money.v1+json",
"Content-Type: application/vnd.s-money.v1+json"
})
@PUT("users/{appuserid}/subaccounts/{appaccountid}")
Call updateSubAccount(@Path("appuserid") String appUserId, @Path("appaccountid") String appAccountId, @Body SubAccountEntity subAccount);
/**
* Delete a sub account.
*
* @param appUserId 3rd party user ID
* @param appAccountId 3rd party account ID
* @return Nothing
* @since 15.11.01
*/
@Headers({
"Accept: application/vnd.s-money.v1+json",
"Content-Type: application/vnd.s-money.v1+json"
})
@DELETE("users/{appuserid}/subaccounts/{appaccountid}")
Call deleteSubAccount(@Path("appuserid") String appUserId, @Path("appaccountid") String appAccountId);
/**
* List first 50 registered cards of an user.
*
* @param appUserId 3rd party user ID
* @return A list of {@code CardEntity}
* @since 15.11.01
*/
@Headers("Accept: application/vnd.s-money.v1+json")
@GET("users/{appuserid}/cards")
Call> listCards(@Path("appuserid") String appUserId);
/**
* List registered cards of an user.
*
* @param appUserId 3rd party user ID
* @param pageNumber The page to retrieve
* @return A list of {@code CardEntity}
* @since 16.01.01
*/
@Headers("Accept: application/vnd.s-money.v1+json")
@GET("users/{appuserid}/cards")
Call> listCards(@Path("appuserid") String appUserId, @Query("page") Integer pageNumber);
/**
* List registered cards of an user.
*
* @param appUserId 3rd party user ID
* @param pageNumber The page to retrieve
* @param itemPerPage The number of items per page
* @return A list of {@code CardEntity}
* @since 16.01.01
*/
@Headers("Accept: application/vnd.s-money.v1+json")
@GET("users/{appuserid}/cards")
Call> listCards(@Path("appuserid") String appUserId, @Query("page") Integer pageNumber, @Query("perpage") Integer itemPerPage);
/**
* Create a card for an user.
* Field to fill on the card registration application :
*
* - Card (required)
* --- AppCardId (required)
* --- Name (optional)
* - UrlReturn (required)
* - AvailableCards (optional)
*
*
* @param appUserId 3rd party user ID
* @param cardApplication The card application form
* @return The newly created card as {@code CardRegistrationAnswerEntity}
* @since 15.11.01
*/
@Headers({
"Accept: application/vnd.s-money.v1+json",
"Content-Type: application/vnd.s-money.v1+json"
})
@POST("users/{appuserid}/cards/registrations")
Call createCard(@Path("appuserid") String appUserId, @Body CardRegistrationApplicationEntity cardApplication);
/**
* Get a card of an user.
*
* @param appUserId 3rd party user ID
* @param appCardId 3rd party card ID
* @return The user's card
* @since 15.11.01
*/
@Headers("Accept: application/vnd.s-money.v1+json")
@GET("users/{appuserid}/cards/{appcardid}")
Call getCard(@Path("appuserid") String appUserId, @Path("appcardid") String appCardId);
/**
* Delete a card.
*
* @param appUserId 3rd party user ID
* @param appCardId 3rd party card ID
* @return Nothing
* @since 15.11.01
*/
@DELETE("users/{appuserid}/cards/{appcardid}")
Call deleteCard(@Path("appuserid") String appUserId, @Path("appcardid") String appCardId);
/**
* Create a payment.
* Field to fill on the payment :
*
* - OrderId (required)
* - Beneficiary (required)
* --- AppAccountId (required)
* - Sender (optional)
* --- AppAccountId (required)
* - Amount (required)
* - message (optional)
* - fee (optional)
* --- AmountWithVAT (required)
* --- VAT (required)
*
*
* @param appUserId Sender 3rd party user ID
* @param payment An instantiated entity payment
* @return The newly created payment as {@code PaymentEntity}
* @since 15.11.01
*/
@Headers({
"Accept: application/vnd.s-money.v1+json",
"Content-Type: application/vnd.s-money.v1+json"
})
@POST("users/{appuserid}/payments")
Call createPayment(@Path("appuserid") String appUserId, @Body PaymentEntity payment);
/**
* Create multiple payments.
* (Each payments require a sender)
*
* - OrderId (required)
* - Beneficiary (required)
* --- AppAccountId (required)
* - Sender (required)
* --- AppAccountId (required)
* - Amount (required)
* - message (optional)
* - fee (optional)
* --- AmountWithVAT (required)
* --- VAT (required)
*
*
* @param appUserId Sender 3rd party user ID
* @param payments A list of instantiated entity payment
* @return A list of newly created {@code PaymentEntity}
* @since 15.11.01
*/
@Headers({
"Accept: application/vnd.s-money.v1+json",
"Content-Type: application/vnd.s-money.v1+json"
})
@POST("users/{appuserid}/payments")
Call> createPayments(@Path("appuserid") String appUserId, @Body List payments);
/**
* Get a payment by its 3rd party ID (OrderId).
*
* @param appUserId 3rd party user ID
* @param orderId 3rd party payment ID
* @return The requested payment as {@code PaymentEntity}
* @since 15.11.01
*/
@Headers("Accept: application/vnd.s-money.v1+json")
@GET("users/{appuserid}/payments/{orderid}")
Call getPayment(@Path("appuserid") String appUserId, @Path("orderid") String orderId);
/**
* List first 50 payments for a specific user.
*
* @param appUserId 3rd party user ID
* @return A list of {@code PaymentEntity}
* @since 15.11.01
*/
@Headers("Accept: application/vnd.s-money.v1+json")
@GET("users/{appuserid}/payments")
Call> listPayments(@Path("appuserid") String appUserId);
/**
* List payments for a specific user.
*
* @param appUserId 3rd party user ID
* @param pageNumber The page to retrieve
* @return A list of {@code PaymentEntity}
* @since 16.01.01
*/
@Headers("Accept: application/vnd.s-money.v1+json")
@GET("users/{appuserid}/payments")
Call> listPayments(@Path("appuserid") String appUserId, @Query("page") Integer pageNumber);
/**
* List payments for a specific user.
*
* @param appUserId 3rd party user ID
* @param pageNumber The page to retrieve
* @param itemPerPage The number of items per page
* @return A list of {@code PaymentEntity}
* @since 16.01.01
*/
@Headers("Accept: application/vnd.s-money.v1+json")
@GET("users/{appuserid}/payments")
Call> listPayments(@Path("appuserid") String appUserId, @Query("page") Integer pageNumber, @Query("perpage") Integer itemPerPage);
/**
* List the 50 first bank accounts for a given user.
*
* @param appUserId 3rd party user ID
* @return A list of {@code BankAccountEntity}
* @since 15.11.01
*/
@Headers("Accept: application/vnd.s-money.v1+json")
@GET("users/{appuserid}/bankaccounts")
Call> listBankAccounts(@Path("appuserid") String appUserId);
/**
* List bank accounts for a given user.
*
* @param appUserId 3rd party user ID
* @param pageNumber The page to retrieve
* @return A list of {@code BankAccountEntity}
* @since 16.01.01
*/
@Headers("Accept: application/vnd.s-money.v1+json")
@GET("users/{appuserid}/bankaccounts")
Call> listBankAccounts(@Path("appuserid") String appUserId, @Query("page") Integer pageNumber);
/**
* List bank accounts for a given user.
*
* @param appUserId 3rd party user ID
* @param pageNumber The page to retrieve
* @param itemPerPage The number of items per page
* @return A list of {@code BankAccountEntity}
* @since 16.01.01
*/
@Headers("Accept: application/vnd.s-money.v1+json")
@GET("users/{appuserid}/bankaccounts")
Call> listBankAccounts(@Path("appuserid") String appUserId, @Query("page") Integer pageNumber, @Query("perpage") Integer itemPerPage);
/**
* Get a bank account.
*
* @param appUserId 3rd party user ID
* @param bankAccountId 3rd party bank account ID
* @return The requested bank account as {@code BankAccountEntity}
* @since 15.11.01
*/
@Headers("Accept: application/vnd.s-money.v1+json")
@GET("users/{appuserid}/bankaccounts/{bankaccountid}")
Call getBankAccount(@Path("appuserid") String appUserId, @Path("bankaccountid") Long bankAccountId);
/**
* Create a Bank Account.
* Field to fill on the bank account :
*
* - DisplayName (required)
* - Bic (required)
* - Iban (required)
* - IsMine (required)
*
*
* @param appUserId 3rd party user ID
* @param bankAccount A bank account entity
* @return The newly created bank account as {@code BankAccountEntity}
* @since 15.11.01
*/
@Headers({
"Accept: application/vnd.s-money.v1+json",
"Content-Type: application/vnd.s-money.v1+json"
})
@POST("users/{appuserid}/bankaccounts")
Call createBankAccount(@Path("appuserid") String appUserId, @Body BankAccountEntity bankAccount);
/**
* Update a bank account.
*
* @param appUserId 3rd party user ID
* @param bankAccount The bank account entity to update
* @return The updated bank account as {@code BankAccountEntity}
* @since 15.11.01
*/
@Headers({
"Accept: application/vnd.s-money.v1+json",
"Content-Type: application/vnd.s-money.v1+json"
})
@PUT("users/{appuserid}/bankaccounts")
Call updateBankAccount(@Path("appuserid") String appUserId, @Body BankAccountEntity bankAccount);
/**
* Delete a Bank Account.
*
* @param appUserId 3rd party user ID
* @param bankAccountId 3rd party bank account ID to delete
* @return Nothing
* @since 15.11.01
*/
@DELETE("users/{appuserid}/bankaccounts/{bankaccountid}")
Call deleteBankAccount(@Path("appuserid") String appUserId, @Path("bankaccountid") Long bankAccountId);
/**
* List the 50 first moneyout operations for a given user.
*
* @param appUserId User Client Id
* @return A list of {@code MoneyOutEntity}
* @since 15.11.01
*/
@Headers("Accept: application/vnd.s-money.v1+json")
@GET("users/{appuserid}/moneyouts")
Call> listMoneyOuts(@Path("appuserid") String appUserId);
/**
* List money out operations for a given user.
*
* @param appUserId 3rd party user ID
* @param pageNumber The page to retrieve
* @return A list of {@code MoneyOutEntity}
* @since 16.01.01
*/
@Headers("Accept: application/vnd.s-money.v1+json")
@GET("users/{appuserid}/moneyouts")
Call> listMoneyOuts(@Path("appuserid") String appUserId, @Query("page") Integer pageNumber);
/**
* List money out operations for a given user.
*
* @param appUserId 3rd party user ID
* @param pageNumber The page to retrieve
* @param itemPerPage The number of items per page
* @return A list of {@code MoneyOutEntity}
* @since 16.01.01
*/
@Headers("Accept: application/vnd.s-money.v1+json")
@GET("users/{appuserid}/moneyouts")
Call> listMoneyOuts(@Path("appuserid") String appUserId, @Query("page") Integer pageNumber, @Query("perpage") Integer itemPerPage);
/**
* Create a money out operation.
* Field to fill on the money out operation:
*
* - Amount (required)
* - AccountId (optional)
* --- AppAccountId (required)
* - BankAccount (required)
* --- Id (required)
* - Fee (optional)
* --- AmountWithVAT (required)
* --- VAT (required)
* - Message (optional)
*
*
* @param appUserId 3rd party user ID
* @param moneyOut An instantiated {@code MoneyOutEntity}
* @return The newly created created {@code MoneyOutEntity}
* @since 15.11.01
*/
@Headers({
"Accept: application/vnd.s-money.v1+json",
"Content-Type: application/vnd.s-money.v1+json"
})
@POST("users/{appuserid}/moneyouts/oneshot")
Call createMoneyOutOneShot(@Path("appuserid") String appUserId, @Body MoneyOutEntity moneyOut);
/**
* Create a money out operation.
* Field to fill on the money out operation:
*
* - Amount (required)
* - AccountId (optional)
* --- AppAccountId (required)
* - BankAccount (required)
* --- displayname (optional)
* --- Bic (required)
* --- Iban (required)
* --- IsMine (required)
* - Fee (optional)
* --- AmountWithVAT (required)
* --- VAT (required)
* - Message (optional)
*
*
* @param appUserId 3rd party user ID
* @param moneyOut An instantiated {@code MoneyOutEntity}
* @return The newly created {@code MoneyOutEntity}
* @since 15.11.01
*/
@Headers({
"Accept: application/vnd.s-money.v1+json",
"Content-Type: application/vnd.s-money.v1+json"
})
@POST("users/{appuserid}/moneyouts/recurring")
Call createMoneyOutRecurring(@Path("appuserid") String appUserId, @Body MoneyOutEntity moneyOut);
/**
* Get money out entry by its Id.
*
* @param appUserId 3rd party user ID
* @param moneyOutId S-Money money out ID
* @return The requested money out information as {@code MoneyOutEntity}
* @since 15.11.01
*/
@Headers("Accept: application/vnd.s-money.v1+json")
@GET("users/{appuserid}/moneyouts/{moneyoutid}")
Call getMoneyOut(@Path("appuserid") String appUserId, @Path("moneyoutid") Long moneyOutId);
/**
* Get money out entry by its Id.
*
* @param appUserId 3rd party user ID
* @param moneyOutOrderId 3rd party money out orderId
* @return The requested money out information as {@code MoneyOutEntity}
* @since 16.01.01
*/
@Headers("Accept: application/vnd.s-money.v1+json")
@GET("users/{appuserid}/moneyouts/{moneyoutid}")
Call getMoneyOut(@Path("appuserid") String appUserId, @Path("moneyoutid") String moneyOutOrderId);
/**
* Create a Card Payment.
* Field to fill on the card payment entity :
*
* - Amount (required)
* - OrderId (required)
* - AvailableCards (optional)
* - Beneficiary (optional)
* --- AppAccountId (required)
* - Message (optional)
* - IsMine (required)
* - UrlReturn (required)
* - UrlCallback (optional)
* - Fee (optional)
* - Card (optional)
* --- AppCardId (required)
* --- Name (optional)
* - PaymentSchedule[] (optional)
* --- Amount (required)
* --- Date (required)
* --- Fee (optional)
*
*
* @param appUserId 3rd party user ID
* @param cardPayment A CardPayment entity
* @return The created card payment as {@code CardPaymentEntity}
* @since 15.11.01
*/
@Headers({
"Accept: application/vnd.s-money.v2+json",
"Content-Type: application/vnd.s-money.v2+json"
})
@POST("users/{appuserid}/payins/cardpayments")
Call createCardPayment(@Path("appuserid") String appUserId, @Body CardPaymentEntity cardPayment);
/**
* List the 50 first card payments for a given user.
*
* @param appUserId 3rd party user ID
* @return A list of {@code CardPaymentEntity}
* @since 15.11.01
*/
@Headers("Accept: application/vnd.s-money.v2+json")
@GET("users/{appuserid}/payins/cardpayments")
Call> listCardPayments(@Path("appuserid") String appUserId);
/**
* List card payments for a given user.
*
* @param appUserId 3rd party user ID
* @param pageNumber The page to retrieve
* @return A list of {@code CardPaymentEntity}
* @since 16.01.01
*/
@Headers("Accept: application/vnd.s-money.v2+json")
@GET("users/{appuserid}/payins/cardpayments")
Call> listCardPayments(@Path("appuserid") String appUserId, @Query("page") Integer pageNumber);
/**
* List card payments for a given user.
*
* @param appUserId 3rd party user ID
* @param pageNumber The page to retrieve
* @param itemPerPage The number of items per page
* @return A list of {@code CardPaymentEntity}
* @since 16.01.01
*/
@Headers("Accept: application/vnd.s-money.v2+json")
@GET("users/{appuserid}/payins/cardpayments")
Call> listCardPayments(@Path("appuserid") String appUserId, @Query("page") Integer pageNumber, @Query("perpage") Integer itemPerPage);
/**
* Get a card payments for a given user.
*
* @param appUserId 3rd party user ID
* @param orderId 3rd party payment ID
* @return The card payment as {@code CardPaymentEntity}
* @since 15.11.01
*/
@Headers("Accept: application/vnd.s-money.v2+json")
@GET("users/{appuserid}/payins/cardpayments/{orderid}")
Call getCardPayment(@Path("appuserid") String appUserId, @Path("orderid") String orderId);
/**
* List a scheduled payment for a given user.
*
* @param appUserId 3rd party user ID
* @param orderId 3rd party payment ID
* @param sequenceNumber The scheduled payment sequence number
* @return The scheduled payment
* @since 15.11.01
*/
@Headers("Accept: application/vnd.s-money.v2+json")
@GET("users/{appuserid}/payins/cardpayments/{orderid}/{sequencenumber}")
Call getCardScheduledPayment(@Path("appuserid") String appUserId, @Path("orderid") String orderId, @Path("sequencenumber") Integer sequenceNumber);
/**
* Refund an card payment order.
*
* @param appUserId 3rd party user ID
* @param orderId 3rd party payment ID
* @param refundForm The form to refund
* @return The refund information as {@code CardPaymentRefundAnswerEntity}
* @since 15.11.01
*/
@Headers({
"Accept: application/vnd.s-money.v2+json",
"Content-Type: application/vnd.s-money.v2+json"
})
@POST("users/{appuserid}/payins/cardpayments/{orderid}/refunds")
Call refundCardPayment(@Path("appuserid") String appUserId, @Path("orderid") String orderId, @Body CardPaymentRefundApplicationEntity refundForm);
/**
* Refund a scheduled payment of card payment order.
*
* @param appUserId Owner 3rd party user ID
* @param orderId 3rd party payment ID
* @param sequenceNumber The scheduled payment sequence number
* @param refundForm The form to refund
* @return The refund information as {@code CardPaymentRefundAnswerEntity}
* @since 15.11.01
*/
@Headers({
"Accept: application/vnd.s-money.v2+json",
"Content-Type: application/vnd.s-money.v2+json"
})
@POST("users/{appuserid}/payins/cardpayments/{orderid}/{sequencenumber}/refunds")
Call refundCardScheduledPayment(@Path("appuserid") String appUserId, @Path("orderid") String orderId, @Path("sequencenumber") Integer sequenceNumber, @Body CardPaymentRefundApplicationEntity refundForm);
/**
* Edit a scheduled payment of card payment order.
*
* @param appUserId Owner 3rd party user ID
* @param orderId 3rd party payment ID
* @param sequenceNumber The scheduled payment sequence number
* @param payment The scheduled payment information
* @return The refund information as {@code PaymentScheduleEntity}
* @since 15.11.01
*/
@Headers({
"Accept: application/vnd.s-money.v2+json",
"Content-Type: application/vnd.s-money.v2+json"
})
@PUT("users/{appuserid}/payins/cardpayments/{orderid}/{sequencenumber}")
Call refundCardScheduledPayment(@Path("appuserid") String appUserId, @Path("orderid") String orderId, @Path("sequencenumber") Integer sequenceNumber, @Body PaymentScheduleEntity payment);
/**
* Create KYC Request.
* Field to fill on the KYC request :
*
* - MultiPart File (required)
*
*
* @param appUserId Sender 3rd party user ID
* @param file The file to upload
* @return The newly created KYC request as {@code KycEntity}
* @since 15.11.01
*/
@Multipart
@Headers("Accept: application/vnd.s-money.v1+json")
@POST("users/{appuserid}/kyc")
Call createKYCRequest(@Path("appuserid") String appUserId, @Part("file1") RequestBody file);
/**
* Create KYC Request.
* Field to fill on the KYC request :
*
* - MultiPart File[] (required)
*
*
* @param appUserId Sender 3rd party user ID
* @param files The files to upload
* @return The KYC (entity) in request status
* @since 15.11.01
*/
@Multipart
@Headers("Accept: application/vnd.s-money.v1+json")
@POST("users/{appuserid}/kyc")
Call createKYCRequest(@Path("appuserid") String appUserId, @PartMap Map files);
/**
* List all KYC. This method is DEPRECATED and will be removed
* soon. Please use the method listKYCRequests
* instead.
*
* @param appUserId 3rd party user ID
* @return The list of {@code KycEntity}
* @see SMoneyService#listKYCRequests
* @since 15.11.01
*/
@Headers("Accept: application/vnd.s-money.v1+json")
@GET("users/{appuserid}/kyc")
@Deprecated
Call> getKYC(@Path("appuserid") String appUserId);
/**
* List Know Your Customer (KYC) requests.
*
* @param appUserId 3rd party user ID
* @return A list of {@code KycEntity}
* @since 16.11.01
*/
@Headers("Accept: application/vnd.s-money.v1+json")
@GET("users/{appuserid}/kyc")
Call> listKYCRequests(@Path("appuserid") String appUserId);
/**
* List Know Your Customer (KYC) requests.
*
* @param appUserId 3rd party user ID
* @param pageNumber The page to retrieve
* @return A list of {@code KycEntity}
* @since 16.11.01
*/
@Headers("Accept: application/vnd.s-money.v1+json")
@GET("users/{appuserid}/kyc")
Call> listKYCRequests(@Path("appuserid") String appUserId, @Query("page") Integer pageNumber);
/**
* List Know Your Customer (KYC) requests.
*
* @param appUserId 3rd party user ID
* @param pageNumber The page to retrieve
* @param itemPerPage The number of items per page
* @return A list of {@code KycEntity}
* @since 16.11.01
*/
@Headers("Accept: application/vnd.s-money.v1+json")
@GET("users/{appuserid}/kyc")
Call> listKYCRequests(@Path("appuserid") String appUserId, @Query("page") Integer pageNumber, @Query("perpage") Integer itemPerPage);
/**
* List the 50 first payments processed from a stored card. This method
* is DEPRECATED and will be removed soon. Please use the method
* listStoredCardPayment instead.
*
* @param appUserId 3rd party user ID
* @return A list of {@code StoredCardPaymentEntity}
* @see SMoneyService#listStoredCardPayments
* @since 15.11.01
*/
@Headers("Accept: application/vnd.s-money.v1+json")
@GET("users/{appuserid}/payins/storedcardpayments")
@Deprecated
Call> listStoredCardPayment(@Path("appuserid") String appUserId);
/**
* List the 50 first payments processed from a stored card.
*
* @param appUserId 3rd party user ID
* @return A list of {@code StoredCardPaymentEntity}
* @since 15.11.01
*/
@Headers("Accept: application/vnd.s-money.v1+json")
@GET("users/{appuserid}/payins/storedcardpayments")
Call> listStoredCardPayments(@Path("appuserid") String appUserId);
/**
* List payments processed from a stored card.
*
* @param appUserId 3rd party user ID
* @param pageNumber The page to retrieve
* @return A list of {@code StoredCardPaymentEntity}
* @since 16.01.01
*/
@Headers("Accept: application/vnd.s-money.v1+json")
@GET("users/{appuserid}/payins/storedcardpayments")
Call> listStoredCardPayments(@Path("appuserid") String appUserId, @Query("page") Integer pageNumber);
/**
* List payments processed from a stored card.
*
* @param appUserId 3rd party user ID
* @param pageNumber The page to retrieve
* @param itemPerPage The number of items per page
* @return A list of {@code StoredCardPaymentEntity}
* @since 16.01.01
*/
@Headers("Accept: application/vnd.s-money.v1+json")
@GET("users/{appuserid}/payins/storedcardpayments")
Call> listStoredCardPayments(@Path("appuserid") String appUserId, @Query("page") Integer pageNumber, @Query("perpage") Integer itemPerPage);
/**
* Create payment using a stored card.
* Field to fill on the payment by StoredCard :
*
* - OrderId (required)
* - AccountId (optional)
* --- AppAccountId (required)
* - Card (required)
* --- AppCardId (required)
* --- IsMine (required - If AccountId is not the card owner)
* - Amount (required)
* - Fee (optional)
* --- AmountWithVAT (required)
* --- VAT (required)
*
*
* @param appUserId Sender 3rd party user ID
* @param storedCardPay The StoredCardPayment Entity
* @return The newly created stored card payment as {@code StoredCardPaymentEntity}
* @since 15.11.01
*/
@Headers({
"Accept: application/vnd.s-money.v2+json",
"Content-Type: application/vnd.s-money.v2+json"
})
@POST("users/{appuserid}/payins/storedcardpayments")
Call createStoredCardPayment(@Path("appuserid") String appUserId, @Body StoredCardPaymentEntity storedCardPay);
/**
* Get a stored card payment.
*
* @param appUserId 3rd party user ID
* @param orderId 3rd party payment ID
* @return The requested stored card payment as {@code StoredCardPaymentEntity}
* @since 15.11.01
*/
@Headers("Accept: application/vnd.s-money.v1+json")
@GET("users/{appuserid}/payins/storedcardpayments/{orderid}")
Call getStoredCardPayment(@Path("appuserid") String appUserId, @Path("orderid") String orderId);
/**
* Refund a stored card payment.
* Field to fill on the refund :
*
* - amount (required)
* - orderId (optional)
* - refundFee (required)
*
*
* @param appUserId 3rd party user ID
* @param orderId 3rd party payment ID
* @param refundRequest The request for refund
* @return The newly created refund as {@code CardPaymentRefundAnswerEntity}
* @since 15.11.01
*/
@Headers({
"Accept: application/vnd.s-money.v2+json",
"Content-Type: application/vnd.s-money.v2+json"
})
@POST("users/{appuserid}/payins/storedcardpayments/{orderid}/refunds")
Call refundStoredCardPayment(@Path("appuserid") String appUserId, @Path("orderid") String orderId, @Body CardPaymentRefundApplicationEntity refundRequest);
/**
* Get history: all payments (All type: CB, moneyout...).
*
* @param appUserId 3rd party user ID
* @return A list of {@code HistoryItemEntity}
* @since 15.11.01
*/
@Headers("Accept: application/vnd.s-money.v1+json")
@GET("users/{appuserid}/historyitems")
Call> listHistoryItems(@Path("appuserid") String appUserId);
/**
* Get all references.
*
* @param appUserId 3rd party user ID
* @return A List of {@code ReferenceEntity}
* @since 15.11.01
*/
@Headers("Accept: application/vnd.s-money.v1+json")
@GET("users/{appuserid}/payins/banktransfers/references")
Call> listReferences(@Path("appuserid") String appUserId);
/**
* Get all references.
*
* @param appUserId 3rd party user ID
* @param pageNumber The page to retrieve
* @return A list of {@code ReferenceEntity}
* @since 16.01.01
*/
@Headers("Accept: application/vnd.s-money.v1+json")
@GET("users/{appuserid}/payins/banktransfers/references")
Call> listReferences(@Path("appuserid") String appUserId, @Query("page") Integer pageNumber);
/**
* Get all references.
*
* @param appUserId 3rd party user ID
* @param pageNumber The page to retrieve
* @param itemPerPage The number of items per page
* @return A list of {@code ReferenceEntity}
* @since 16.01.01
*/
@Headers("Accept: application/vnd.s-money.v1+json")
@GET("users/{appuserid}/payins/banktransfers/references")
Call> listReferences(@Path("appuserid") String appUserId, @Query("page") Integer pageNumber, @Query("perpage") Integer itemPerPage);
/**
* Create a reference for bank transfer.
* Field to fill on the reference :
*
* - Beneficiary (optional)
* --- AppAccountId (required)
* - IsMine (required)
*
*
* @param appUserId 3rd party user ID
* @param reference The reference for BankTransfer
* @return The newly created {@code ReferenceEntity}
* @since 15.11.01
*/
@Headers({
"Accept: application/vnd.s-money.v2+json",
"Content-Type: application/vnd.s-money.v2+json"
})
@POST("users/{appuserid}/payins/banktransfers/references")
Call createReference(@Path("appuserid") String appUserId, @Body ReferenceEntity reference);
/**
* Get a specific reference.
*
* @param appUserId 3rd party user ID
* @param referenceId S-Money reference ID
* @return The requested reference as {@code ReferenceEntity}
* @since 15.11.01
*/
@Headers("Accept: application/vnd.s-money.v1+json")
@GET("users/{appuserid}/payins/banktransfers/references/{referenceid}")
Call getReference(@Path("appuserid") String appUserId, @Path("referenceid") Long referenceId);
/**
* Get a specific reference.
*
* @param appUserId 3rd party user ID
* @param referenceCode S-Money reference code
* @return The requested reference as {@code ReferenceEntity}
* @since 15.11.01
*/
@Headers("Accept: application/vnd.s-money.v1+json")
@GET("users/{appuserid}/payins/banktransfers/references/{referencecode}")
Call getReference(@Path("appuserid") String appUserId, @Path("referencecode") String referenceCode);
/**
* Get 50 first bank transfers.
*
* @param appUserId 3rd party user ID
* @return A list of {@code BankTransferEntity}
* @since 15.11.01
*/
@Headers("Accept: application/vnd.s-money.v1+json")
@GET("users/{appuserid}/payins/banktransfers")
Call> listBankTransfers(@Path("appuserid") String appUserId);
/**
* Get bank transfers.
*
* @param appUserId 3rd party user ID
* @param pageNumber The page to retrieve
* @return A list of {@code BankTransferEntity}
* @since 16.01.01
*/
@Headers("Accept: application/vnd.s-money.v1+json")
@GET("users/{appuserid}/payins/banktransfers")
Call> listBankTransfers(@Path("appuserid") String appUserId, @Query("page") Integer pageNumber);
/**
* Get bank transfers.
*
* @param appUserId 3rd party user ID
* @param pageNumber The page to retrieve
* @param itemPerPage The number of items per page
* @return A list of {@code BankTransferEntity}
* @since 16.01.01
*/
@Headers("Accept: application/vnd.s-money.v1+json")
@GET("users/{appuserid}/payins/banktransfers")
Call> listBankTransfers(@Path("appuserid") String appUserId, @Query("page") Integer pageNumber, @Query("perpage") Integer itemPerPage);
/**
* Get a specific bank transfer.
*
* @param appUserId 3rd party user ID
* @param bankTransferId S-Money bank transfer ID
* @return A list of {@code BankTransferEntity}
* @since 15.11.01
*/
@Headers("Accept: application/vnd.s-money.v1+json")
@GET("users/{appuserid}/payins/banktransfers/{banktransferid}")
Call getBankTransfer(@Path("appuserid") String appUserId, @Path("banktransferid") Long bankTransferId);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy