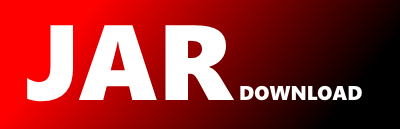
com.payline.ws.model.Address Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of payline-java-sdk Show documentation
Show all versions of payline-java-sdk Show documentation
The Payline API provides access to the various functions of the Payline payment solution. It is based on standard web service components, which include the SOAP protocol, the WSDL and XSD definition languages. These standards are supported by a large range of development tools on multiple platforms. This SDK covers all the functions of the Payline payment solution.
package com.payline.ws.model;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlType;
/**
*
* This element contains information about the
* address
*
*
* Java class for address complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="address">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="title" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="name" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="firstName" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="lastName" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="street1" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="street2" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="streetNumber" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="cityName" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="zipCode" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="country" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="phone" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="state" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="county" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="phoneType" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="addressCreateDate" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="email" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "address", namespace = "http://obj.ws.payline.experian.com", propOrder = {
"title",
"name",
"firstName",
"lastName",
"street1",
"street2",
"streetNumber",
"cityName",
"zipCode",
"country",
"phone",
"state",
"county",
"phoneType",
"addressCreateDate",
"email"
})
public class Address {
@XmlElement(nillable = true)
protected String title;
@XmlElement(nillable = true)
protected String name;
@XmlElement(nillable = true)
protected String firstName;
@XmlElement(nillable = true)
protected String lastName;
@XmlElement(nillable = true)
protected String street1;
@XmlElement(nillable = true)
protected String street2;
@XmlElement(nillable = true)
protected String streetNumber;
@XmlElement(nillable = true)
protected String cityName;
@XmlElement(nillable = true)
protected String zipCode;
@XmlElement(nillable = true)
protected String country;
@XmlElement(nillable = true)
protected String phone;
@XmlElement(nillable = true)
protected String state;
@XmlElement(nillable = true)
protected String county;
@XmlElement(nillable = true)
protected String phoneType;
@XmlElement(nillable = true)
protected String addressCreateDate;
@XmlElement(nillable = true)
protected String email;
/**
* Gets the value of the title property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTitle() {
return title;
}
/**
* Sets the value of the title property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setTitle(String value) {
this.title = value;
}
/**
* Gets the value of the name property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getName() {
return name;
}
/**
* Sets the value of the name property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setName(String value) {
this.name = value;
}
/**
* Gets the value of the firstName property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getFirstName() {
return firstName;
}
/**
* Sets the value of the firstName property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setFirstName(String value) {
this.firstName = value;
}
/**
* Gets the value of the lastName property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getLastName() {
return lastName;
}
/**
* Sets the value of the lastName property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setLastName(String value) {
this.lastName = value;
}
/**
* Gets the value of the street1 property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getStreet1() {
return street1;
}
/**
* Sets the value of the street1 property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setStreet1(String value) {
this.street1 = value;
}
/**
* Gets the value of the street2 property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getStreet2() {
return street2;
}
/**
* Sets the value of the street2 property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setStreet2(String value) {
this.street2 = value;
}
/**
* Gets the value of the streetNumber property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getStreetNumber() {
return streetNumber;
}
/**
* Sets the value of the streetNumber property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setStreetNumber(String value) {
this.streetNumber = value;
}
/**
* Gets the value of the cityName property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCityName() {
return cityName;
}
/**
* Sets the value of the cityName property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setCityName(String value) {
this.cityName = value;
}
/**
* Gets the value of the zipCode property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getZipCode() {
return zipCode;
}
/**
* Sets the value of the zipCode property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setZipCode(String value) {
this.zipCode = value;
}
/**
* Gets the value of the country property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCountry() {
return country;
}
/**
* Sets the value of the country property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setCountry(String value) {
this.country = value;
}
/**
* Gets the value of the phone property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getPhone() {
return phone;
}
/**
* Sets the value of the phone property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setPhone(String value) {
this.phone = value;
}
/**
* Gets the value of the state property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getState() {
return state;
}
/**
* Sets the value of the state property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setState(String value) {
this.state = value;
}
/**
* Gets the value of the county property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCounty() {
return county;
}
/**
* Sets the value of the county property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setCounty(String value) {
this.county = value;
}
/**
* Gets the value of the phoneType property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getPhoneType() {
return phoneType;
}
/**
* Sets the value of the phoneType property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setPhoneType(String value) {
this.phoneType = value;
}
/**
* Gets the value of the addressCreateDate property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getAddressCreateDate() {
return addressCreateDate;
}
/**
* Sets the value of the addressCreateDate property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setAddressCreateDate(String value) {
this.addressCreateDate = value;
}
/**
* Gets the value of the email property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getEmail() {
return email;
}
/**
* Sets the value of the email property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setEmail(String value) {
this.email = value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy