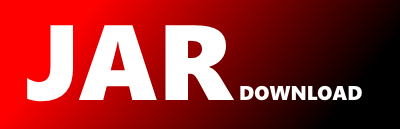
com.payline.ws.model.Authentication3DSecure Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of payline-java-sdk Show documentation
Show all versions of payline-java-sdk Show documentation
The Payline API provides access to the various functions of the Payline payment solution. It is based on standard web service components, which include the SOAP protocol, the WSDL and XSD definition languages. These standards are supported by a large range of development tools on multiple platforms. This SDK covers all the functions of the Payline payment solution.
package com.payline.ws.model;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlType;
/**
*
* This element contains element for a 3DSecure
* transaction
*
*
* Java class for authentication3DSecure complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="authentication3DSecure">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="md" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="pares" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="xid" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="eci" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="cavv" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="cavvAlgorithm" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="vadsResult" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="typeSecurisation" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="PaResStatus" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="VeResStatus" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="resultContainer" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="authenticationResult" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "authentication3DSecure", namespace = "http://obj.ws.payline.experian.com", propOrder = {
"md",
"pares",
"xid",
"eci",
"cavv",
"cavvAlgorithm",
"vadsResult",
"typeSecurisation",
"paResStatus",
"veResStatus",
"resultContainer",
"authenticationResult"
})
public class Authentication3DSecure {
@XmlElement(nillable = true)
protected String md;
@XmlElement(nillable = true)
protected String pares;
@XmlElement(nillable = true)
protected String xid;
@XmlElement(nillable = true)
protected String eci;
@XmlElement(nillable = true)
protected String cavv;
@XmlElement(nillable = true)
protected String cavvAlgorithm;
@XmlElement(nillable = true)
protected String vadsResult;
@XmlElement(nillable = true)
protected String typeSecurisation;
@XmlElement(name = "PaResStatus", nillable = true)
protected String paResStatus;
@XmlElement(name = "VeResStatus", nillable = true)
protected String veResStatus;
@XmlElement(nillable = true)
protected String resultContainer;
@XmlElement(nillable = true)
protected String authenticationResult;
/**
* Gets the value of the md property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getMd() {
return md;
}
/**
* Sets the value of the md property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setMd(String value) {
this.md = value;
}
/**
* Gets the value of the pares property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getPares() {
return pares;
}
/**
* Sets the value of the pares property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setPares(String value) {
this.pares = value;
}
/**
* Gets the value of the xid property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getXid() {
return xid;
}
/**
* Sets the value of the xid property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setXid(String value) {
this.xid = value;
}
/**
* Gets the value of the eci property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getEci() {
return eci;
}
/**
* Sets the value of the eci property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setEci(String value) {
this.eci = value;
}
/**
* Gets the value of the cavv property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCavv() {
return cavv;
}
/**
* Sets the value of the cavv property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setCavv(String value) {
this.cavv = value;
}
/**
* Gets the value of the cavvAlgorithm property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCavvAlgorithm() {
return cavvAlgorithm;
}
/**
* Sets the value of the cavvAlgorithm property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setCavvAlgorithm(String value) {
this.cavvAlgorithm = value;
}
/**
* Gets the value of the vadsResult property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getVadsResult() {
return vadsResult;
}
/**
* Sets the value of the vadsResult property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setVadsResult(String value) {
this.vadsResult = value;
}
/**
* Gets the value of the typeSecurisation property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTypeSecurisation() {
return typeSecurisation;
}
/**
* Sets the value of the typeSecurisation property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setTypeSecurisation(String value) {
this.typeSecurisation = value;
}
/**
* Gets the value of the paResStatus property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getPaResStatus() {
return paResStatus;
}
/**
* Sets the value of the paResStatus property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setPaResStatus(String value) {
this.paResStatus = value;
}
/**
* Gets the value of the veResStatus property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getVeResStatus() {
return veResStatus;
}
/**
* Sets the value of the veResStatus property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setVeResStatus(String value) {
this.veResStatus = value;
}
/**
* Gets the value of the resultContainer property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getResultContainer() {
return resultContainer;
}
/**
* Sets the value of the resultContainer property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setResultContainer(String value) {
this.resultContainer = value;
}
/**
* Gets the value of the authenticationResult property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getAuthenticationResult() {
return authenticationResult;
}
/**
* Sets the value of the authenticationResult property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setAuthenticationResult(String value) {
this.authenticationResult = value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy