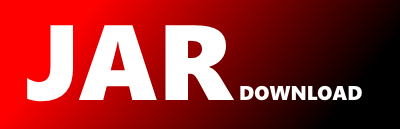
com.payline.ws.model.Cards Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of payline-java-sdk Show documentation
Show all versions of payline-java-sdk Show documentation
The Payline API provides access to the various functions of the Payline payment solution. It is based on standard web service components, which include the SOAP protocol, the WSDL and XSD definition languages. These standards are supported by a large range of development tools on multiple platforms. This SDK covers all the functions of the Payline payment solution.
package com.payline.ws.model;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlType;
/**
*
* This element contains element for a wallet
*
*
* Java class for cards complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="cards">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="walletId" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="lastName" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="firstName" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="email" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="shippingAddress" type="{http://obj.ws.payline.experian.com}address"/>
* <element name="card" type="{http://obj.ws.payline.experian.com}card"/>
* <element name="cardInd" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="comment" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="isDisabled" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="disableDate" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="extendedCard" type="{http://obj.ws.payline.experian.com}extendedCardType"/>
* <element name="default" type="{http://www.w3.org/2001/XMLSchema}string"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "cards", namespace = "http://obj.ws.payline.experian.com", propOrder = {
"walletId",
"lastName",
"firstName",
"email",
"shippingAddress",
"card",
"cardInd",
"comment",
"isDisabled",
"disableDate",
"extendedCard",
"_default"
})
public class Cards {
@XmlElement(required = true)
protected String walletId;
@XmlElement(required = true, nillable = true)
protected String lastName;
@XmlElement(required = true, nillable = true)
protected String firstName;
@XmlElement(required = true, nillable = true)
protected String email;
@XmlElement(required = true, nillable = true)
protected Address shippingAddress;
@XmlElement(required = true)
protected Card card;
@XmlElement(required = true, nillable = true)
protected String cardInd;
@XmlElement(required = true, nillable = true)
protected String comment;
@XmlElement(required = true, nillable = true)
protected String isDisabled;
@XmlElement(required = true, nillable = true)
protected String disableDate;
@XmlElement(required = true, nillable = true)
protected ExtendedCardType extendedCard;
@XmlElement(name = "default", required = true, nillable = true)
protected String _default;
/**
* Gets the value of the walletId property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getWalletId() {
return walletId;
}
/**
* Sets the value of the walletId property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setWalletId(String value) {
this.walletId = value;
}
/**
* Gets the value of the lastName property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getLastName() {
return lastName;
}
/**
* Sets the value of the lastName property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setLastName(String value) {
this.lastName = value;
}
/**
* Gets the value of the firstName property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getFirstName() {
return firstName;
}
/**
* Sets the value of the firstName property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setFirstName(String value) {
this.firstName = value;
}
/**
* Gets the value of the email property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getEmail() {
return email;
}
/**
* Sets the value of the email property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setEmail(String value) {
this.email = value;
}
/**
* Gets the value of the shippingAddress property.
*
* @return
* possible object is
* {@link Address }
*
*/
public Address getShippingAddress() {
return shippingAddress;
}
/**
* Sets the value of the shippingAddress property.
*
* @param value
* allowed object is
* {@link Address }
*
*/
public void setShippingAddress(Address value) {
this.shippingAddress = value;
}
/**
* Gets the value of the card property.
*
* @return
* possible object is
* {@link Card }
*
*/
public Card getCard() {
return card;
}
/**
* Sets the value of the card property.
*
* @param value
* allowed object is
* {@link Card }
*
*/
public void setCard(Card value) {
this.card = value;
}
/**
* Gets the value of the cardInd property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCardInd() {
return cardInd;
}
/**
* Sets the value of the cardInd property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setCardInd(String value) {
this.cardInd = value;
}
/**
* Gets the value of the comment property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getComment() {
return comment;
}
/**
* Sets the value of the comment property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setComment(String value) {
this.comment = value;
}
/**
* Gets the value of the isDisabled property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getIsDisabled() {
return isDisabled;
}
/**
* Sets the value of the isDisabled property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setIsDisabled(String value) {
this.isDisabled = value;
}
/**
* Gets the value of the disableDate property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getDisableDate() {
return disableDate;
}
/**
* Sets the value of the disableDate property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setDisableDate(String value) {
this.disableDate = value;
}
/**
* Gets the value of the extendedCard property.
*
* @return
* possible object is
* {@link ExtendedCardType }
*
*/
public ExtendedCardType getExtendedCard() {
return extendedCard;
}
/**
* Sets the value of the extendedCard property.
*
* @param value
* allowed object is
* {@link ExtendedCardType }
*
*/
public void setExtendedCard(ExtendedCardType value) {
this.extendedCard = value;
}
/**
* Gets the value of the default property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getDefault() {
return _default;
}
/**
* Sets the value of the default property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setDefault(String value) {
this._default = value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy