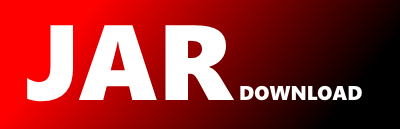
com.payline.ws.model.CreateWalletRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of payline-java-sdk Show documentation
Show all versions of payline-java-sdk Show documentation
The Payline API provides access to the various functions of the Payline payment solution. It is based on standard web service components, which include the SOAP protocol, the WSDL and XSD definition languages. These standards are supported by a large range of development tools on multiple platforms. This SDK covers all the functions of the Payline payment solution.
package com.payline.ws.model;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlType;
/**
*
* This element is the request for the
* createWallet
* method
*
*
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="version" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="contractNumber" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="wallet" type="{http://obj.ws.payline.experian.com}wallet"/>
* <element name="buyer" type="{http://obj.ws.payline.experian.com}buyer"/>
* <element name="owner" type="{http://obj.ws.payline.experian.com}owner"/>
* <element name="privateDataList" type="{http://obj.ws.payline.experian.com}privateDataList"/>
* <element name="authentication3DSecure" type="{http://obj.ws.payline.experian.com}authentication3DSecure"/>
* <element name="media" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="contractNumberWalletList" type="{http://obj.ws.payline.experian.com}contractNumberWalletList"/>
* <element name="transactionID" type="{http://www.w3.org/2001/XMLSchema}string"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"version",
"contractNumber",
"wallet",
"buyer",
"owner",
"privateDataList",
"authentication3DSecure",
"media",
"contractNumberWalletList",
"transactionID"
})
@XmlRootElement(name = "createWalletRequest")
public class CreateWalletRequest {
@XmlElement(required = true)
protected String version;
@XmlElement(required = true)
protected String contractNumber;
@XmlElement(required = true)
protected Wallet wallet;
@XmlElement(required = true, nillable = true)
protected Buyer buyer;
@XmlElement(required = true, nillable = true)
protected Owner owner;
@XmlElement(required = true, nillable = true)
protected PrivateDataList privateDataList;
@XmlElement(required = true, nillable = true)
protected Authentication3DSecure authentication3DSecure;
@XmlElement(required = true, nillable = true)
protected String media;
@XmlElement(required = true, nillable = true)
protected ContractNumberWalletList contractNumberWalletList;
@XmlElement(required = true, nillable = true)
protected String transactionID;
/**
* Gets the value of the version property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getVersion() {
return version;
}
/**
* Sets the value of the version property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setVersion(String value) {
this.version = value;
}
/**
* Gets the value of the contractNumber property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getContractNumber() {
return contractNumber;
}
/**
* Sets the value of the contractNumber property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setContractNumber(String value) {
this.contractNumber = value;
}
/**
* Gets the value of the wallet property.
*
* @return
* possible object is
* {@link Wallet }
*
*/
public Wallet getWallet() {
return wallet;
}
/**
* Sets the value of the wallet property.
*
* @param value
* allowed object is
* {@link Wallet }
*
*/
public void setWallet(Wallet value) {
this.wallet = value;
}
/**
* Gets the value of the buyer property.
*
* @return
* possible object is
* {@link Buyer }
*
*/
public Buyer getBuyer() {
return buyer;
}
/**
* Sets the value of the buyer property.
*
* @param value
* allowed object is
* {@link Buyer }
*
*/
public void setBuyer(Buyer value) {
this.buyer = value;
}
/**
* Gets the value of the owner property.
*
* @return
* possible object is
* {@link Owner }
*
*/
public Owner getOwner() {
return owner;
}
/**
* Sets the value of the owner property.
*
* @param value
* allowed object is
* {@link Owner }
*
*/
public void setOwner(Owner value) {
this.owner = value;
}
/**
* Gets the value of the privateDataList property.
*
* @return
* possible object is
* {@link PrivateDataList }
*
*/
public PrivateDataList getPrivateDataList() {
return privateDataList;
}
/**
* Sets the value of the privateDataList property.
*
* @param value
* allowed object is
* {@link PrivateDataList }
*
*/
public void setPrivateDataList(PrivateDataList value) {
this.privateDataList = value;
}
/**
* Gets the value of the authentication3DSecure property.
*
* @return
* possible object is
* {@link Authentication3DSecure }
*
*/
public Authentication3DSecure getAuthentication3DSecure() {
return authentication3DSecure;
}
/**
* Sets the value of the authentication3DSecure property.
*
* @param value
* allowed object is
* {@link Authentication3DSecure }
*
*/
public void setAuthentication3DSecure(Authentication3DSecure value) {
this.authentication3DSecure = value;
}
/**
* Gets the value of the media property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getMedia() {
return media;
}
/**
* Sets the value of the media property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setMedia(String value) {
this.media = value;
}
/**
* Gets the value of the contractNumberWalletList property.
*
* @return
* possible object is
* {@link ContractNumberWalletList }
*
*/
public ContractNumberWalletList getContractNumberWalletList() {
return contractNumberWalletList;
}
/**
* Sets the value of the contractNumberWalletList property.
*
* @param value
* allowed object is
* {@link ContractNumberWalletList }
*
*/
public void setContractNumberWalletList(ContractNumberWalletList value) {
this.contractNumberWalletList = value;
}
/**
* Gets the value of the transactionID property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTransactionID() {
return transactionID;
}
/**
* Sets the value of the transactionID property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setTransactionID(String value) {
this.transactionID = value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy