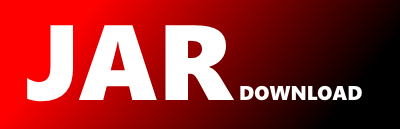
com.payline.ws.model.CustomerTrans Maven / Gradle / Ivy
package com.payline.ws.model;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlType;
/**
* Java class for CustomerTrans complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="CustomerTrans">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="IsLCLFAlerted" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="ExternalTransactionId" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="ReferenceOrder" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="CardCode" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="TransactionDate" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="Amount" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="Status" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="PosLabel" type="{http://www.w3.org/2001/XMLSchema}string"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "CustomerTrans", namespace = "http://obj.ws.payline.experian.com", propOrder = {
"isLCLFAlerted",
"externalTransactionId",
"referenceOrder",
"cardCode",
"transactionDate",
"amount",
"status",
"posLabel"
})
public class CustomerTrans {
@XmlElement(name = "IsLCLFAlerted", required = true)
protected String isLCLFAlerted;
@XmlElement(name = "ExternalTransactionId", required = true)
protected String externalTransactionId;
@XmlElement(name = "ReferenceOrder", required = true)
protected String referenceOrder;
@XmlElement(name = "CardCode", required = true)
protected String cardCode;
@XmlElement(name = "TransactionDate", required = true)
protected String transactionDate;
@XmlElement(name = "Amount", required = true)
protected String amount;
@XmlElement(name = "Status", required = true)
protected String status;
@XmlElement(name = "PosLabel", required = true)
protected String posLabel;
/**
* Gets the value of the isLCLFAlerted property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getIsLCLFAlerted() {
return isLCLFAlerted;
}
/**
* Sets the value of the isLCLFAlerted property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setIsLCLFAlerted(String value) {
this.isLCLFAlerted = value;
}
/**
* Gets the value of the externalTransactionId property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getExternalTransactionId() {
return externalTransactionId;
}
/**
* Sets the value of the externalTransactionId property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setExternalTransactionId(String value) {
this.externalTransactionId = value;
}
/**
* Gets the value of the referenceOrder property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getReferenceOrder() {
return referenceOrder;
}
/**
* Sets the value of the referenceOrder property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setReferenceOrder(String value) {
this.referenceOrder = value;
}
/**
* Gets the value of the cardCode property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCardCode() {
return cardCode;
}
/**
* Sets the value of the cardCode property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setCardCode(String value) {
this.cardCode = value;
}
/**
* Gets the value of the transactionDate property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTransactionDate() {
return transactionDate;
}
/**
* Sets the value of the transactionDate property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setTransactionDate(String value) {
this.transactionDate = value;
}
/**
* Gets the value of the amount property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getAmount() {
return amount;
}
/**
* Sets the value of the amount property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setAmount(String value) {
this.amount = value;
}
/**
* Gets the value of the status property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getStatus() {
return status;
}
/**
* Sets the value of the status property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setStatus(String value) {
this.status = value;
}
/**
* Gets the value of the posLabel property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getPosLabel() {
return posLabel;
}
/**
* Sets the value of the posLabel property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setPosLabel(String value) {
this.posLabel = value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy