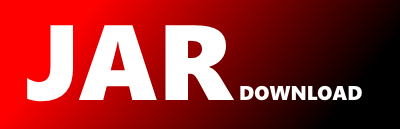
com.payline.ws.model.DoAuthorizationResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of payline-java-sdk Show documentation
Show all versions of payline-java-sdk Show documentation
The Payline API provides access to the various functions of the Payline payment solution. It is based on standard web service components, which include the SOAP protocol, the WSDL and XSD definition languages. These standards are supported by a large range of development tools on multiple platforms. This SDK covers all the functions of the Payline payment solution.
package com.payline.ws.model;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlType;
/**
*
* This element is the reponse from the
* doAuthorization method
*
*
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="transient" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="result" type="{http://obj.ws.payline.experian.com}result"/>
* <element name="transaction" type="{http://obj.ws.payline.experian.com}transaction"/>
* <element name="authorization" type="{http://obj.ws.payline.experian.com}authorization"/>
* <element name="card" type="{http://obj.ws.payline.experian.com}cardOut"/>
* <element name="extendedCard" type="{http://obj.ws.payline.experian.com}extendedCardType"/>
* <element name="privateDataList" type="{http://obj.ws.payline.experian.com}privateDataList"/>
* <element name="contractNumber" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="linkedTransactionId" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="travelFileNumber" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="authentication3DSecure" type="{http://obj.ws.payline.experian.com}authentication3DSecure" minOccurs="0"/>
* <element name="resultContainer" type="{http://www.w3.org/2001/XMLSchema}string"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"_transient",
"result",
"transaction",
"authorization",
"card",
"extendedCard",
"privateDataList",
"contractNumber",
"linkedTransactionId",
"travelFileNumber",
"authentication3DSecure",
"resultContainer"
})
@XmlRootElement(name = "doAuthorizationResponse")
public class DoAuthorizationResponse {
@XmlElement(name = "transient", required = true, nillable = true)
protected String _transient;
@XmlElement(required = true)
protected Result result;
@XmlElement(required = true)
protected Transaction transaction;
@XmlElement(required = true)
protected Authorization authorization;
@XmlElement(required = true)
protected CardOut card;
@XmlElement(required = true)
protected ExtendedCardType extendedCard;
@XmlElement(required = true, nillable = true)
protected PrivateDataList privateDataList;
@XmlElement(required = true)
protected String contractNumber;
@XmlElement(required = true, nillable = true)
protected String linkedTransactionId;
@XmlElement(nillable = true)
protected String travelFileNumber;
@XmlElement(nillable = true)
protected Authentication3DSecure authentication3DSecure;
@XmlElement(required = true, nillable = true)
protected String resultContainer;
/**
* Gets the value of the transient property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTransient() {
return _transient;
}
/**
* Sets the value of the transient property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setTransient(String value) {
this._transient = value;
}
/**
* Gets the value of the result property.
*
* @return
* possible object is
* {@link Result }
*
*/
public Result getResult() {
return result;
}
/**
* Sets the value of the result property.
*
* @param value
* allowed object is
* {@link Result }
*
*/
public void setResult(Result value) {
this.result = value;
}
/**
* Gets the value of the transaction property.
*
* @return
* possible object is
* {@link Transaction }
*
*/
public Transaction getTransaction() {
return transaction;
}
/**
* Sets the value of the transaction property.
*
* @param value
* allowed object is
* {@link Transaction }
*
*/
public void setTransaction(Transaction value) {
this.transaction = value;
}
/**
* Gets the value of the authorization property.
*
* @return
* possible object is
* {@link Authorization }
*
*/
public Authorization getAuthorization() {
return authorization;
}
/**
* Sets the value of the authorization property.
*
* @param value
* allowed object is
* {@link Authorization }
*
*/
public void setAuthorization(Authorization value) {
this.authorization = value;
}
/**
* Gets the value of the card property.
*
* @return
* possible object is
* {@link CardOut }
*
*/
public CardOut getCard() {
return card;
}
/**
* Sets the value of the card property.
*
* @param value
* allowed object is
* {@link CardOut }
*
*/
public void setCard(CardOut value) {
this.card = value;
}
/**
* Gets the value of the extendedCard property.
*
* @return
* possible object is
* {@link ExtendedCardType }
*
*/
public ExtendedCardType getExtendedCard() {
return extendedCard;
}
/**
* Sets the value of the extendedCard property.
*
* @param value
* allowed object is
* {@link ExtendedCardType }
*
*/
public void setExtendedCard(ExtendedCardType value) {
this.extendedCard = value;
}
/**
* Gets the value of the privateDataList property.
*
* @return
* possible object is
* {@link PrivateDataList }
*
*/
public PrivateDataList getPrivateDataList() {
return privateDataList;
}
/**
* Sets the value of the privateDataList property.
*
* @param value
* allowed object is
* {@link PrivateDataList }
*
*/
public void setPrivateDataList(PrivateDataList value) {
this.privateDataList = value;
}
/**
* Gets the value of the contractNumber property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getContractNumber() {
return contractNumber;
}
/**
* Sets the value of the contractNumber property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setContractNumber(String value) {
this.contractNumber = value;
}
/**
* Gets the value of the linkedTransactionId property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getLinkedTransactionId() {
return linkedTransactionId;
}
/**
* Sets the value of the linkedTransactionId property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setLinkedTransactionId(String value) {
this.linkedTransactionId = value;
}
/**
* Gets the value of the travelFileNumber property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTravelFileNumber() {
return travelFileNumber;
}
/**
* Sets the value of the travelFileNumber property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setTravelFileNumber(String value) {
this.travelFileNumber = value;
}
/**
* Gets the value of the authentication3DSecure property.
*
* @return
* possible object is
* {@link Authentication3DSecure }
*
*/
public Authentication3DSecure getAuthentication3DSecure() {
return authentication3DSecure;
}
/**
* Sets the value of the authentication3DSecure property.
*
* @param value
* allowed object is
* {@link Authentication3DSecure }
*
*/
public void setAuthentication3DSecure(Authentication3DSecure value) {
this.authentication3DSecure = value;
}
/**
* Gets the value of the resultContainer property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getResultContainer() {
return resultContainer;
}
/**
* Sets the value of the resultContainer property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setResultContainer(String value) {
this.resultContainer = value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy