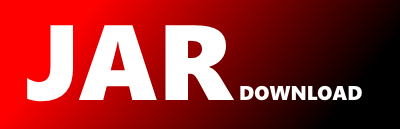
com.payline.ws.model.DoImmediateWalletPaymentRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of payline-java-sdk Show documentation
Show all versions of payline-java-sdk Show documentation
The Payline API provides access to the various functions of the Payline payment solution. It is based on standard web service components, which include the SOAP protocol, the WSDL and XSD definition languages. These standards are supported by a large range of development tools on multiple platforms. This SDK covers all the functions of the Payline payment solution.
package com.payline.ws.model;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlType;
/**
*
* This element is the request for the
* doImmediateWalletPayment method
*
*
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="version" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="payment" type="{http://obj.ws.payline.experian.com}payment"/>
* <element name="order" type="{http://obj.ws.payline.experian.com}order"/>
* <element name="buyer" type="{http://obj.ws.payline.experian.com}buyer"/>
* <element name="walletId" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="cardInd" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="cvx" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="recurring" type="{http://obj.ws.payline.experian.com}recurring" minOccurs="0"/>
* <element name="privateDataList" type="{http://obj.ws.payline.experian.com}privateDataList"/>
* <element name="media" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="authentication3DSecure" type="{http://obj.ws.payline.experian.com}authentication3DSecure"/>
* <element name="subMerchant" type="{http://obj.ws.payline.experian.com}subMerchant"/>
* <element name="linkedTransactionId" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="threeDSInfo" type="{http://obj.ws.payline.experian.com}threeDSInfo"/>
* <element name="travelFileNumber" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"version",
"payment",
"order",
"buyer",
"walletId",
"cardInd",
"cvx",
"recurring",
"privateDataList",
"media",
"authentication3DSecure",
"subMerchant",
"linkedTransactionId",
"threeDSInfo",
"travelFileNumber"
})
@XmlRootElement(name = "doImmediateWalletPaymentRequest")
public class DoImmediateWalletPaymentRequest {
@XmlElement(required = true)
protected String version;
@XmlElement(required = true)
protected Payment payment;
@XmlElement(required = true)
protected Order order;
@XmlElement(required = true, nillable = true)
protected Buyer buyer;
@XmlElement(required = true)
protected String walletId;
@XmlElement(required = true, nillable = true)
protected String cardInd;
@XmlElement(required = true, nillable = true)
protected String cvx;
@XmlElement(nillable = true)
protected Recurring recurring;
@XmlElement(required = true, nillable = true)
protected PrivateDataList privateDataList;
@XmlElement(required = true, nillable = true)
protected String media;
@XmlElement(required = true, nillable = true)
protected Authentication3DSecure authentication3DSecure;
@XmlElement(required = true, nillable = true)
protected SubMerchant subMerchant;
@XmlElement(required = true, nillable = true)
protected String linkedTransactionId;
@XmlElement(required = true, nillable = true)
protected ThreeDSInfo threeDSInfo;
@XmlElement(nillable = true)
protected String travelFileNumber;
/**
* Gets the value of the version property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getVersion() {
return version;
}
/**
* Sets the value of the version property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setVersion(String value) {
this.version = value;
}
/**
* Gets the value of the payment property.
*
* @return
* possible object is
* {@link Payment }
*
*/
public Payment getPayment() {
return payment;
}
/**
* Sets the value of the payment property.
*
* @param value
* allowed object is
* {@link Payment }
*
*/
public void setPayment(Payment value) {
this.payment = value;
}
/**
* Gets the value of the order property.
*
* @return
* possible object is
* {@link Order }
*
*/
public Order getOrder() {
return order;
}
/**
* Sets the value of the order property.
*
* @param value
* allowed object is
* {@link Order }
*
*/
public void setOrder(Order value) {
this.order = value;
}
/**
* Gets the value of the buyer property.
*
* @return
* possible object is
* {@link Buyer }
*
*/
public Buyer getBuyer() {
return buyer;
}
/**
* Sets the value of the buyer property.
*
* @param value
* allowed object is
* {@link Buyer }
*
*/
public void setBuyer(Buyer value) {
this.buyer = value;
}
/**
* Gets the value of the walletId property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getWalletId() {
return walletId;
}
/**
* Sets the value of the walletId property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setWalletId(String value) {
this.walletId = value;
}
/**
* Gets the value of the cardInd property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCardInd() {
return cardInd;
}
/**
* Sets the value of the cardInd property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setCardInd(String value) {
this.cardInd = value;
}
/**
* Gets the value of the cvx property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCvx() {
return cvx;
}
/**
* Sets the value of the cvx property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setCvx(String value) {
this.cvx = value;
}
/**
* Gets the value of the recurring property.
*
* @return
* possible object is
* {@link Recurring }
*
*/
public Recurring getRecurring() {
return recurring;
}
/**
* Sets the value of the recurring property.
*
* @param value
* allowed object is
* {@link Recurring }
*
*/
public void setRecurring(Recurring value) {
this.recurring = value;
}
/**
* Gets the value of the privateDataList property.
*
* @return
* possible object is
* {@link PrivateDataList }
*
*/
public PrivateDataList getPrivateDataList() {
return privateDataList;
}
/**
* Sets the value of the privateDataList property.
*
* @param value
* allowed object is
* {@link PrivateDataList }
*
*/
public void setPrivateDataList(PrivateDataList value) {
this.privateDataList = value;
}
/**
* Gets the value of the media property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getMedia() {
return media;
}
/**
* Sets the value of the media property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setMedia(String value) {
this.media = value;
}
/**
* Gets the value of the authentication3DSecure property.
*
* @return
* possible object is
* {@link Authentication3DSecure }
*
*/
public Authentication3DSecure getAuthentication3DSecure() {
return authentication3DSecure;
}
/**
* Sets the value of the authentication3DSecure property.
*
* @param value
* allowed object is
* {@link Authentication3DSecure }
*
*/
public void setAuthentication3DSecure(Authentication3DSecure value) {
this.authentication3DSecure = value;
}
/**
* Gets the value of the subMerchant property.
*
* @return
* possible object is
* {@link SubMerchant }
*
*/
public SubMerchant getSubMerchant() {
return subMerchant;
}
/**
* Sets the value of the subMerchant property.
*
* @param value
* allowed object is
* {@link SubMerchant }
*
*/
public void setSubMerchant(SubMerchant value) {
this.subMerchant = value;
}
/**
* Gets the value of the linkedTransactionId property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getLinkedTransactionId() {
return linkedTransactionId;
}
/**
* Sets the value of the linkedTransactionId property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setLinkedTransactionId(String value) {
this.linkedTransactionId = value;
}
/**
* Gets the value of the threeDSInfo property.
*
* @return
* possible object is
* {@link ThreeDSInfo }
*
*/
public ThreeDSInfo getThreeDSInfo() {
return threeDSInfo;
}
/**
* Sets the value of the threeDSInfo property.
*
* @param value
* allowed object is
* {@link ThreeDSInfo }
*
*/
public void setThreeDSInfo(ThreeDSInfo value) {
this.threeDSInfo = value;
}
/**
* Gets the value of the travelFileNumber property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTravelFileNumber() {
return travelFileNumber;
}
/**
* Sets the value of the travelFileNumber property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setTravelFileNumber(String value) {
this.travelFileNumber = value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy