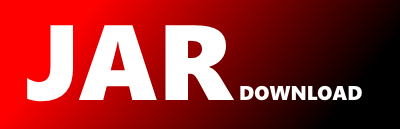
com.payline.ws.model.DoWebPaymentRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of payline-java-sdk Show documentation
Show all versions of payline-java-sdk Show documentation
The Payline API provides access to the various functions of the Payline payment solution. It is based on standard web service components, which include the SOAP protocol, the WSDL and XSD definition languages. These standards are supported by a large range of development tools on multiple platforms. This SDK covers all the functions of the Payline payment solution.
package com.payline.ws.model;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlType;
/**
*
* This element is the request for the
* doWebPayment
* method
*
*
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="version" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="payment" type="{http://obj.ws.payline.experian.com}payment"/>
* <element name="returnURL" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="cancelURL" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="order" type="{http://obj.ws.payline.experian.com}order"/>
* <element name="notificationURL" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="selectedContractList" type="{http://obj.ws.payline.experian.com}selectedContractList"/>
* <element name="secondSelectedContractList" type="{http://obj.ws.payline.experian.com}selectedContractList"/>
* <element name="privateDataList" type="{http://obj.ws.payline.experian.com}privateDataList"/>
* <element name="languageCode" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="customPaymentPageCode" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="buyer" type="{http://obj.ws.payline.experian.com}buyer" minOccurs="0"/>
* <element name="owner" type="{http://obj.ws.payline.experian.com}owner"/>
* <element name="securityMode" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="recurring" type="{http://obj.ws.payline.experian.com}recurring"/>
* <element name="customPaymentTemplateURL" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="contractNumberWalletList" type="{http://obj.ws.payline.experian.com}contractNumberWalletList"/>
* <element name="merchantName" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="subMerchant" type="{http://obj.ws.payline.experian.com}subMerchant"/>
* <element name="miscData" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="asynchronousRetryTimeout" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="threeDSInfo" type="{http://obj.ws.payline.experian.com}threeDSInfo"/>
* <element name="merchantScore" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="skipSmartDisplay" type="{http://www.w3.org/2001/XMLSchema}boolean"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"version",
"payment",
"returnURL",
"cancelURL",
"order",
"notificationURL",
"selectedContractList",
"secondSelectedContractList",
"privateDataList",
"languageCode",
"customPaymentPageCode",
"buyer",
"owner",
"securityMode",
"recurring",
"customPaymentTemplateURL",
"contractNumberWalletList",
"merchantName",
"subMerchant",
"miscData",
"asynchronousRetryTimeout",
"threeDSInfo",
"merchantScore",
"skipSmartDisplay"
})
@XmlRootElement(name = "doWebPaymentRequest")
public class DoWebPaymentRequest {
@XmlElement(required = true)
protected String version;
@XmlElement(required = true)
protected Payment payment;
@XmlElement(required = true)
protected String returnURL;
@XmlElement(required = true)
protected String cancelURL;
@XmlElement(required = true)
protected Order order;
@XmlElement(required = true, nillable = true)
protected String notificationURL;
@XmlElement(required = true, nillable = true)
protected SelectedContractList selectedContractList;
@XmlElement(required = true, nillable = true)
protected SelectedContractList secondSelectedContractList;
@XmlElement(required = true, nillable = true)
protected PrivateDataList privateDataList;
@XmlElement(required = true, nillable = true)
protected String languageCode;
@XmlElement(required = true, nillable = true)
protected String customPaymentPageCode;
protected Buyer buyer;
@XmlElement(required = true, nillable = true)
protected Owner owner;
@XmlElement(required = true, nillable = true)
protected String securityMode;
@XmlElement(required = true, nillable = true)
protected Recurring recurring;
@XmlElement(required = true, nillable = true)
protected String customPaymentTemplateURL;
@XmlElement(required = true, nillable = true)
protected ContractNumberWalletList contractNumberWalletList;
@XmlElement(required = true, nillable = true)
protected String merchantName;
@XmlElement(required = true, nillable = true)
protected SubMerchant subMerchant;
@XmlElement(required = true, nillable = true)
protected String miscData;
@XmlElement(required = true, nillable = true)
protected String asynchronousRetryTimeout;
@XmlElement(required = true, nillable = true)
protected ThreeDSInfo threeDSInfo;
@XmlElement(required = true, nillable = true)
protected String merchantScore;
@XmlElement(required = true, type = Boolean.class, nillable = true)
protected Boolean skipSmartDisplay;
/**
* Gets the value of the version property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getVersion() {
return version;
}
/**
* Sets the value of the version property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setVersion(String value) {
this.version = value;
}
/**
* Gets the value of the payment property.
*
* @return
* possible object is
* {@link Payment }
*
*/
public Payment getPayment() {
return payment;
}
/**
* Sets the value of the payment property.
*
* @param value
* allowed object is
* {@link Payment }
*
*/
public void setPayment(Payment value) {
this.payment = value;
}
/**
* Gets the value of the returnURL property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getReturnURL() {
return returnURL;
}
/**
* Sets the value of the returnURL property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setReturnURL(String value) {
this.returnURL = value;
}
/**
* Gets the value of the cancelURL property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCancelURL() {
return cancelURL;
}
/**
* Sets the value of the cancelURL property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setCancelURL(String value) {
this.cancelURL = value;
}
/**
* Gets the value of the order property.
*
* @return
* possible object is
* {@link Order }
*
*/
public Order getOrder() {
return order;
}
/**
* Sets the value of the order property.
*
* @param value
* allowed object is
* {@link Order }
*
*/
public void setOrder(Order value) {
this.order = value;
}
/**
* Gets the value of the notificationURL property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getNotificationURL() {
return notificationURL;
}
/**
* Sets the value of the notificationURL property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setNotificationURL(String value) {
this.notificationURL = value;
}
/**
* Gets the value of the selectedContractList property.
*
* @return
* possible object is
* {@link SelectedContractList }
*
*/
public SelectedContractList getSelectedContractList() {
return selectedContractList;
}
/**
* Sets the value of the selectedContractList property.
*
* @param value
* allowed object is
* {@link SelectedContractList }
*
*/
public void setSelectedContractList(SelectedContractList value) {
this.selectedContractList = value;
}
/**
* Gets the value of the secondSelectedContractList property.
*
* @return
* possible object is
* {@link SelectedContractList }
*
*/
public SelectedContractList getSecondSelectedContractList() {
return secondSelectedContractList;
}
/**
* Sets the value of the secondSelectedContractList property.
*
* @param value
* allowed object is
* {@link SelectedContractList }
*
*/
public void setSecondSelectedContractList(SelectedContractList value) {
this.secondSelectedContractList = value;
}
/**
* Gets the value of the privateDataList property.
*
* @return
* possible object is
* {@link PrivateDataList }
*
*/
public PrivateDataList getPrivateDataList() {
return privateDataList;
}
/**
* Sets the value of the privateDataList property.
*
* @param value
* allowed object is
* {@link PrivateDataList }
*
*/
public void setPrivateDataList(PrivateDataList value) {
this.privateDataList = value;
}
/**
* Gets the value of the languageCode property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getLanguageCode() {
return languageCode;
}
/**
* Sets the value of the languageCode property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setLanguageCode(String value) {
this.languageCode = value;
}
/**
* Gets the value of the customPaymentPageCode property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCustomPaymentPageCode() {
return customPaymentPageCode;
}
/**
* Sets the value of the customPaymentPageCode property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setCustomPaymentPageCode(String value) {
this.customPaymentPageCode = value;
}
/**
* Gets the value of the buyer property.
*
* @return
* possible object is
* {@link Buyer }
*
*/
public Buyer getBuyer() {
return buyer;
}
/**
* Sets the value of the buyer property.
*
* @param value
* allowed object is
* {@link Buyer }
*
*/
public void setBuyer(Buyer value) {
this.buyer = value;
}
/**
* Gets the value of the owner property.
*
* @return
* possible object is
* {@link Owner }
*
*/
public Owner getOwner() {
return owner;
}
/**
* Sets the value of the owner property.
*
* @param value
* allowed object is
* {@link Owner }
*
*/
public void setOwner(Owner value) {
this.owner = value;
}
/**
* Gets the value of the securityMode property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getSecurityMode() {
return securityMode;
}
/**
* Sets the value of the securityMode property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setSecurityMode(String value) {
this.securityMode = value;
}
/**
* Gets the value of the recurring property.
*
* @return
* possible object is
* {@link Recurring }
*
*/
public Recurring getRecurring() {
return recurring;
}
/**
* Sets the value of the recurring property.
*
* @param value
* allowed object is
* {@link Recurring }
*
*/
public void setRecurring(Recurring value) {
this.recurring = value;
}
/**
* Gets the value of the customPaymentTemplateURL property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCustomPaymentTemplateURL() {
return customPaymentTemplateURL;
}
/**
* Sets the value of the customPaymentTemplateURL property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setCustomPaymentTemplateURL(String value) {
this.customPaymentTemplateURL = value;
}
/**
* Gets the value of the contractNumberWalletList property.
*
* @return
* possible object is
* {@link ContractNumberWalletList }
*
*/
public ContractNumberWalletList getContractNumberWalletList() {
return contractNumberWalletList;
}
/**
* Sets the value of the contractNumberWalletList property.
*
* @param value
* allowed object is
* {@link ContractNumberWalletList }
*
*/
public void setContractNumberWalletList(ContractNumberWalletList value) {
this.contractNumberWalletList = value;
}
/**
* Gets the value of the merchantName property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getMerchantName() {
return merchantName;
}
/**
* Sets the value of the merchantName property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setMerchantName(String value) {
this.merchantName = value;
}
/**
* Gets the value of the subMerchant property.
*
* @return
* possible object is
* {@link SubMerchant }
*
*/
public SubMerchant getSubMerchant() {
return subMerchant;
}
/**
* Sets the value of the subMerchant property.
*
* @param value
* allowed object is
* {@link SubMerchant }
*
*/
public void setSubMerchant(SubMerchant value) {
this.subMerchant = value;
}
/**
* Gets the value of the miscData property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getMiscData() {
return miscData;
}
/**
* Sets the value of the miscData property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setMiscData(String value) {
this.miscData = value;
}
/**
* Gets the value of the asynchronousRetryTimeout property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getAsynchronousRetryTimeout() {
return asynchronousRetryTimeout;
}
/**
* Sets the value of the asynchronousRetryTimeout property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setAsynchronousRetryTimeout(String value) {
this.asynchronousRetryTimeout = value;
}
/**
* Gets the value of the threeDSInfo property.
*
* @return
* possible object is
* {@link ThreeDSInfo }
*
*/
public ThreeDSInfo getThreeDSInfo() {
return threeDSInfo;
}
/**
* Sets the value of the threeDSInfo property.
*
* @param value
* allowed object is
* {@link ThreeDSInfo }
*
*/
public void setThreeDSInfo(ThreeDSInfo value) {
this.threeDSInfo = value;
}
/**
* Gets the value of the merchantScore property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getMerchantScore() {
return merchantScore;
}
/**
* Sets the value of the merchantScore property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setMerchantScore(String value) {
this.merchantScore = value;
}
/**
* Gets the value of the skipSmartDisplay property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isSkipSmartDisplay() {
return skipSmartDisplay;
}
/**
* Sets the value of the skipSmartDisplay property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setSkipSmartDisplay(Boolean value) {
this.skipSmartDisplay = value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy