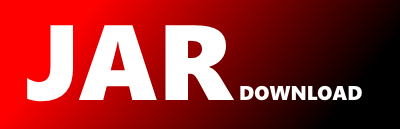
com.payline.ws.model.GetTokenResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of payline-java-sdk Show documentation
Show all versions of payline-java-sdk Show documentation
The Payline API provides access to the various functions of the Payline payment solution. It is based on standard web service components, which include the SOAP protocol, the WSDL and XSD definition languages. These standards are supported by a large range of development tools on multiple platforms. This SDK covers all the functions of the Payline payment solution.
package com.payline.ws.model;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlType;
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="result" type="{http://obj.ws.payline.experian.com}result"/>
* <element name="token" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="maskedCardNumber" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="expirationDate" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="virtualCard" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="cardType" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="cardProduct" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="acceptanceNetwork" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="bank" type="{http://www.w3.org/2001/XMLSchema}string"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"result",
"token",
"maskedCardNumber",
"expirationDate",
"virtualCard",
"cardType",
"cardProduct",
"acceptanceNetwork",
"bank"
})
@XmlRootElement(name = "getTokenResponse")
public class GetTokenResponse {
@XmlElement(required = true)
protected Result result;
@XmlElement(required = true)
protected String token;
@XmlElement(required = true)
protected String maskedCardNumber;
@XmlElement(required = true)
protected String expirationDate;
@XmlElement(required = true)
protected String virtualCard;
@XmlElement(required = true)
protected String cardType;
@XmlElement(required = true)
protected String cardProduct;
@XmlElement(required = true)
protected String acceptanceNetwork;
@XmlElement(required = true)
protected String bank;
/**
* Gets the value of the result property.
*
* @return
* possible object is
* {@link Result }
*
*/
public Result getResult() {
return result;
}
/**
* Sets the value of the result property.
*
* @param value
* allowed object is
* {@link Result }
*
*/
public void setResult(Result value) {
this.result = value;
}
/**
* Gets the value of the token property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getToken() {
return token;
}
/**
* Sets the value of the token property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setToken(String value) {
this.token = value;
}
/**
* Gets the value of the maskedCardNumber property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getMaskedCardNumber() {
return maskedCardNumber;
}
/**
* Sets the value of the maskedCardNumber property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setMaskedCardNumber(String value) {
this.maskedCardNumber = value;
}
/**
* Gets the value of the expirationDate property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getExpirationDate() {
return expirationDate;
}
/**
* Sets the value of the expirationDate property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setExpirationDate(String value) {
this.expirationDate = value;
}
/**
* Gets the value of the virtualCard property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getVirtualCard() {
return virtualCard;
}
/**
* Sets the value of the virtualCard property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setVirtualCard(String value) {
this.virtualCard = value;
}
/**
* Gets the value of the cardType property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCardType() {
return cardType;
}
/**
* Sets the value of the cardType property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setCardType(String value) {
this.cardType = value;
}
/**
* Gets the value of the cardProduct property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCardProduct() {
return cardProduct;
}
/**
* Sets the value of the cardProduct property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setCardProduct(String value) {
this.cardProduct = value;
}
/**
* Gets the value of the acceptanceNetwork property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getAcceptanceNetwork() {
return acceptanceNetwork;
}
/**
* Sets the value of the acceptanceNetwork property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setAcceptanceNetwork(String value) {
this.acceptanceNetwork = value;
}
/**
* Gets the value of the bank property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getBank() {
return bank;
}
/**
* Sets the value of the bank property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setBank(String value) {
this.bank = value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy