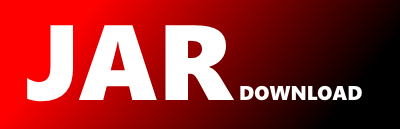
com.payline.ws.model.GetTransactionDetailsResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of payline-java-sdk Show documentation
Show all versions of payline-java-sdk Show documentation
The Payline API provides access to the various functions of the Payline payment solution. It is based on standard web service components, which include the SOAP protocol, the WSDL and XSD definition languages. These standards are supported by a large range of development tools on multiple platforms. This SDK covers all the functions of the Payline payment solution.
package com.payline.ws.model;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlType;
/**
*
* This element is the response for the
* getTransactionDetails method
*
*
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="result" type="{http://obj.ws.payline.experian.com}result"/>
* <element name="transaction" type="{http://obj.ws.payline.experian.com}transaction"/>
* <element name="payment" type="{http://obj.ws.payline.experian.com}payment"/>
* <element name="authorization" type="{http://obj.ws.payline.experian.com}authorization"/>
* <element name="order" type="{http://obj.ws.payline.experian.com}order"/>
* <element name="buyer" type="{http://obj.ws.payline.experian.com}buyer"/>
* <element name="privateDataList" type="{http://obj.ws.payline.experian.com}privateDataList"/>
* <element name="card" type="{http://obj.ws.payline.experian.com}cardOut"/>
* <element name="extendedCard" type="{http://obj.ws.payline.experian.com}extendedCardType"/>
* <element name="associatedTransactionsList" type="{http://obj.ws.payline.experian.com}associatedTransactionsList"/>
* <element name="statusHistoryList" type="{http://obj.ws.payline.experian.com}statusHistoryList"/>
* <element name="media" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="customerMedia" type="{http://obj.ws.payline.experian.com}media"/>
* <element name="contractNumber" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="paymentAdditionalList" type="{http://obj.ws.payline.experian.com}paymentAdditionalList" minOccurs="0"/>
* <element name="bankAccountData" type="{http://obj.ws.payline.experian.com}bankAccountData"/>
* <element name="subMerchant" type="{http://obj.ws.payline.experian.com}subMerchant"/>
* <element name="authentication3DSecure" type="{http://obj.ws.payline.experian.com}authentication3DSecure" minOccurs="0"/>
* <element name="pointOfSell" type="{http://obj.ws.payline.experian.com}pointOfSell"/>
* <element name="routingRule" type="{http://obj.ws.payline.experian.com}routingRule"/>
* <element name="linkedTransactionId" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="travelFileNumber" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"result",
"transaction",
"payment",
"authorization",
"order",
"buyer",
"privateDataList",
"card",
"extendedCard",
"associatedTransactionsList",
"statusHistoryList",
"media",
"customerMedia",
"contractNumber",
"paymentAdditionalList",
"bankAccountData",
"subMerchant",
"authentication3DSecure",
"pointOfSell",
"routingRule",
"linkedTransactionId",
"travelFileNumber"
})
@XmlRootElement(name = "getTransactionDetailsResponse")
public class GetTransactionDetailsResponse {
@XmlElement(required = true)
protected Result result;
@XmlElement(required = true, nillable = true)
protected Transaction transaction;
@XmlElement(required = true, nillable = true)
protected Payment payment;
@XmlElement(required = true, nillable = true)
protected Authorization authorization;
@XmlElement(required = true, nillable = true)
protected Order order;
@XmlElement(required = true, nillable = true)
protected Buyer buyer;
@XmlElement(required = true, nillable = true)
protected PrivateDataList privateDataList;
@XmlElement(required = true)
protected CardOut card;
@XmlElement(required = true)
protected ExtendedCardType extendedCard;
@XmlElement(required = true, nillable = true)
protected AssociatedTransactionsList associatedTransactionsList;
@XmlElement(required = true, nillable = true)
protected StatusHistoryList statusHistoryList;
@XmlElement(required = true, nillable = true)
protected String media;
@XmlElement(required = true)
protected Media customerMedia;
@XmlElement(required = true)
protected String contractNumber;
@XmlElement(nillable = true)
protected PaymentAdditionalList paymentAdditionalList;
@XmlElement(required = true, nillable = true)
protected BankAccountData bankAccountData;
@XmlElement(required = true, nillable = true)
protected SubMerchant subMerchant;
@XmlElement(nillable = true)
protected Authentication3DSecure authentication3DSecure;
@XmlElement(required = true)
protected PointOfSell pointOfSell;
@XmlElement(required = true)
protected RoutingRule routingRule;
@XmlElement(required = true, nillable = true)
protected String linkedTransactionId;
@XmlElement(nillable = true)
protected String travelFileNumber;
/**
* Gets the value of the result property.
*
* @return
* possible object is
* {@link Result }
*
*/
public Result getResult() {
return result;
}
/**
* Sets the value of the result property.
*
* @param value
* allowed object is
* {@link Result }
*
*/
public void setResult(Result value) {
this.result = value;
}
/**
* Gets the value of the transaction property.
*
* @return
* possible object is
* {@link Transaction }
*
*/
public Transaction getTransaction() {
return transaction;
}
/**
* Sets the value of the transaction property.
*
* @param value
* allowed object is
* {@link Transaction }
*
*/
public void setTransaction(Transaction value) {
this.transaction = value;
}
/**
* Gets the value of the payment property.
*
* @return
* possible object is
* {@link Payment }
*
*/
public Payment getPayment() {
return payment;
}
/**
* Sets the value of the payment property.
*
* @param value
* allowed object is
* {@link Payment }
*
*/
public void setPayment(Payment value) {
this.payment = value;
}
/**
* Gets the value of the authorization property.
*
* @return
* possible object is
* {@link Authorization }
*
*/
public Authorization getAuthorization() {
return authorization;
}
/**
* Sets the value of the authorization property.
*
* @param value
* allowed object is
* {@link Authorization }
*
*/
public void setAuthorization(Authorization value) {
this.authorization = value;
}
/**
* Gets the value of the order property.
*
* @return
* possible object is
* {@link Order }
*
*/
public Order getOrder() {
return order;
}
/**
* Sets the value of the order property.
*
* @param value
* allowed object is
* {@link Order }
*
*/
public void setOrder(Order value) {
this.order = value;
}
/**
* Gets the value of the buyer property.
*
* @return
* possible object is
* {@link Buyer }
*
*/
public Buyer getBuyer() {
return buyer;
}
/**
* Sets the value of the buyer property.
*
* @param value
* allowed object is
* {@link Buyer }
*
*/
public void setBuyer(Buyer value) {
this.buyer = value;
}
/**
* Gets the value of the privateDataList property.
*
* @return
* possible object is
* {@link PrivateDataList }
*
*/
public PrivateDataList getPrivateDataList() {
return privateDataList;
}
/**
* Sets the value of the privateDataList property.
*
* @param value
* allowed object is
* {@link PrivateDataList }
*
*/
public void setPrivateDataList(PrivateDataList value) {
this.privateDataList = value;
}
/**
* Gets the value of the card property.
*
* @return
* possible object is
* {@link CardOut }
*
*/
public CardOut getCard() {
return card;
}
/**
* Sets the value of the card property.
*
* @param value
* allowed object is
* {@link CardOut }
*
*/
public void setCard(CardOut value) {
this.card = value;
}
/**
* Gets the value of the extendedCard property.
*
* @return
* possible object is
* {@link ExtendedCardType }
*
*/
public ExtendedCardType getExtendedCard() {
return extendedCard;
}
/**
* Sets the value of the extendedCard property.
*
* @param value
* allowed object is
* {@link ExtendedCardType }
*
*/
public void setExtendedCard(ExtendedCardType value) {
this.extendedCard = value;
}
/**
* Gets the value of the associatedTransactionsList property.
*
* @return
* possible object is
* {@link AssociatedTransactionsList }
*
*/
public AssociatedTransactionsList getAssociatedTransactionsList() {
return associatedTransactionsList;
}
/**
* Sets the value of the associatedTransactionsList property.
*
* @param value
* allowed object is
* {@link AssociatedTransactionsList }
*
*/
public void setAssociatedTransactionsList(AssociatedTransactionsList value) {
this.associatedTransactionsList = value;
}
/**
* Gets the value of the statusHistoryList property.
*
* @return
* possible object is
* {@link StatusHistoryList }
*
*/
public StatusHistoryList getStatusHistoryList() {
return statusHistoryList;
}
/**
* Sets the value of the statusHistoryList property.
*
* @param value
* allowed object is
* {@link StatusHistoryList }
*
*/
public void setStatusHistoryList(StatusHistoryList value) {
this.statusHistoryList = value;
}
/**
* Gets the value of the media property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getMedia() {
return media;
}
/**
* Sets the value of the media property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setMedia(String value) {
this.media = value;
}
/**
* Gets the value of the customerMedia property.
*
* @return
* possible object is
* {@link Media }
*
*/
public Media getCustomerMedia() {
return customerMedia;
}
/**
* Sets the value of the customerMedia property.
*
* @param value
* allowed object is
* {@link Media }
*
*/
public void setCustomerMedia(Media value) {
this.customerMedia = value;
}
/**
* Gets the value of the contractNumber property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getContractNumber() {
return contractNumber;
}
/**
* Sets the value of the contractNumber property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setContractNumber(String value) {
this.contractNumber = value;
}
/**
* Gets the value of the paymentAdditionalList property.
*
* @return
* possible object is
* {@link PaymentAdditionalList }
*
*/
public PaymentAdditionalList getPaymentAdditionalList() {
return paymentAdditionalList;
}
/**
* Sets the value of the paymentAdditionalList property.
*
* @param value
* allowed object is
* {@link PaymentAdditionalList }
*
*/
public void setPaymentAdditionalList(PaymentAdditionalList value) {
this.paymentAdditionalList = value;
}
/**
* Gets the value of the bankAccountData property.
*
* @return
* possible object is
* {@link BankAccountData }
*
*/
public BankAccountData getBankAccountData() {
return bankAccountData;
}
/**
* Sets the value of the bankAccountData property.
*
* @param value
* allowed object is
* {@link BankAccountData }
*
*/
public void setBankAccountData(BankAccountData value) {
this.bankAccountData = value;
}
/**
* Gets the value of the subMerchant property.
*
* @return
* possible object is
* {@link SubMerchant }
*
*/
public SubMerchant getSubMerchant() {
return subMerchant;
}
/**
* Sets the value of the subMerchant property.
*
* @param value
* allowed object is
* {@link SubMerchant }
*
*/
public void setSubMerchant(SubMerchant value) {
this.subMerchant = value;
}
/**
* Gets the value of the authentication3DSecure property.
*
* @return
* possible object is
* {@link Authentication3DSecure }
*
*/
public Authentication3DSecure getAuthentication3DSecure() {
return authentication3DSecure;
}
/**
* Sets the value of the authentication3DSecure property.
*
* @param value
* allowed object is
* {@link Authentication3DSecure }
*
*/
public void setAuthentication3DSecure(Authentication3DSecure value) {
this.authentication3DSecure = value;
}
/**
* Gets the value of the pointOfSell property.
*
* @return
* possible object is
* {@link PointOfSell }
*
*/
public PointOfSell getPointOfSell() {
return pointOfSell;
}
/**
* Sets the value of the pointOfSell property.
*
* @param value
* allowed object is
* {@link PointOfSell }
*
*/
public void setPointOfSell(PointOfSell value) {
this.pointOfSell = value;
}
/**
* Gets the value of the routingRule property.
*
* @return
* possible object is
* {@link RoutingRule }
*
*/
public RoutingRule getRoutingRule() {
return routingRule;
}
/**
* Sets the value of the routingRule property.
*
* @param value
* allowed object is
* {@link RoutingRule }
*
*/
public void setRoutingRule(RoutingRule value) {
this.routingRule = value;
}
/**
* Gets the value of the linkedTransactionId property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getLinkedTransactionId() {
return linkedTransactionId;
}
/**
* Sets the value of the linkedTransactionId property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setLinkedTransactionId(String value) {
this.linkedTransactionId = value;
}
/**
* Gets the value of the travelFileNumber property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTravelFileNumber() {
return travelFileNumber;
}
/**
* Sets the value of the travelFileNumber property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setTravelFileNumber(String value) {
this.travelFileNumber = value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy