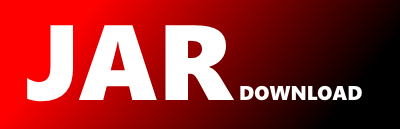
com.payline.ws.model.ManageWebWalletRequest Maven / Gradle / Ivy
package com.payline.ws.model;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlType;
/**
*
* This element is the request for the
* manageWebWallet
* method
*
*
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="version" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="contractNumber" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="selectedContractList" type="{http://obj.ws.payline.experian.com}selectedContractList"/>
* <element name="updatePersonalDetails" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="buyer" type="{http://obj.ws.payline.experian.com}buyer"/>
* <element name="owner" type="{http://obj.ws.payline.experian.com}owner"/>
* <element name="languageCode" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="customPaymentPageCode" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="securityMode" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="returnURL" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="cancelURL" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="notificationURL" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="privateDataList" type="{http://obj.ws.payline.experian.com}privateDataList"/>
* <element name="customPaymentTemplateURL" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="contractNumberWalletList" type="{http://obj.ws.payline.experian.com}contractNumberWalletList"/>
* <element name="merchantName" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="threeDSInfo" type="{http://obj.ws.payline.experian.com}threeDSInfo"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"version",
"contractNumber",
"selectedContractList",
"updatePersonalDetails",
"buyer",
"owner",
"languageCode",
"customPaymentPageCode",
"securityMode",
"returnURL",
"cancelURL",
"notificationURL",
"privateDataList",
"customPaymentTemplateURL",
"contractNumberWalletList",
"merchantName",
"threeDSInfo"
})
@XmlRootElement(name = "manageWebWalletRequest")
public class ManageWebWalletRequest {
@XmlElement(required = true)
protected String version;
@XmlElement(required = true)
protected String contractNumber;
@XmlElement(required = true, nillable = true)
protected SelectedContractList selectedContractList;
@XmlElement(required = true, nillable = true)
protected String updatePersonalDetails;
@XmlElement(required = true)
protected Buyer buyer;
@XmlElement(required = true, nillable = true)
protected Owner owner;
@XmlElement(required = true, nillable = true)
protected String languageCode;
@XmlElement(required = true, nillable = true)
protected String customPaymentPageCode;
@XmlElement(required = true, nillable = true)
protected String securityMode;
@XmlElement(required = true)
protected String returnURL;
@XmlElement(required = true)
protected String cancelURL;
@XmlElement(required = true, nillable = true)
protected String notificationURL;
@XmlElement(required = true, nillable = true)
protected PrivateDataList privateDataList;
@XmlElement(required = true, nillable = true)
protected String customPaymentTemplateURL;
@XmlElement(required = true, nillable = true)
protected ContractNumberWalletList contractNumberWalletList;
@XmlElement(required = true, nillable = true)
protected String merchantName;
@XmlElement(required = true, nillable = true)
protected ThreeDSInfo threeDSInfo;
/**
* Gets the value of the version property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getVersion() {
return version;
}
/**
* Sets the value of the version property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setVersion(String value) {
this.version = value;
}
/**
* Gets the value of the contractNumber property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getContractNumber() {
return contractNumber;
}
/**
* Sets the value of the contractNumber property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setContractNumber(String value) {
this.contractNumber = value;
}
/**
* Gets the value of the selectedContractList property.
*
* @return
* possible object is
* {@link SelectedContractList }
*
*/
public SelectedContractList getSelectedContractList() {
return selectedContractList;
}
/**
* Sets the value of the selectedContractList property.
*
* @param value
* allowed object is
* {@link SelectedContractList }
*
*/
public void setSelectedContractList(SelectedContractList value) {
this.selectedContractList = value;
}
/**
* Gets the value of the updatePersonalDetails property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getUpdatePersonalDetails() {
return updatePersonalDetails;
}
/**
* Sets the value of the updatePersonalDetails property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setUpdatePersonalDetails(String value) {
this.updatePersonalDetails = value;
}
/**
* Gets the value of the buyer property.
*
* @return
* possible object is
* {@link Buyer }
*
*/
public Buyer getBuyer() {
return buyer;
}
/**
* Sets the value of the buyer property.
*
* @param value
* allowed object is
* {@link Buyer }
*
*/
public void setBuyer(Buyer value) {
this.buyer = value;
}
/**
* Gets the value of the owner property.
*
* @return
* possible object is
* {@link Owner }
*
*/
public Owner getOwner() {
return owner;
}
/**
* Sets the value of the owner property.
*
* @param value
* allowed object is
* {@link Owner }
*
*/
public void setOwner(Owner value) {
this.owner = value;
}
/**
* Gets the value of the languageCode property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getLanguageCode() {
return languageCode;
}
/**
* Sets the value of the languageCode property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setLanguageCode(String value) {
this.languageCode = value;
}
/**
* Gets the value of the customPaymentPageCode property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCustomPaymentPageCode() {
return customPaymentPageCode;
}
/**
* Sets the value of the customPaymentPageCode property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setCustomPaymentPageCode(String value) {
this.customPaymentPageCode = value;
}
/**
* Gets the value of the securityMode property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getSecurityMode() {
return securityMode;
}
/**
* Sets the value of the securityMode property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setSecurityMode(String value) {
this.securityMode = value;
}
/**
* Gets the value of the returnURL property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getReturnURL() {
return returnURL;
}
/**
* Sets the value of the returnURL property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setReturnURL(String value) {
this.returnURL = value;
}
/**
* Gets the value of the cancelURL property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCancelURL() {
return cancelURL;
}
/**
* Sets the value of the cancelURL property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setCancelURL(String value) {
this.cancelURL = value;
}
/**
* Gets the value of the notificationURL property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getNotificationURL() {
return notificationURL;
}
/**
* Sets the value of the notificationURL property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setNotificationURL(String value) {
this.notificationURL = value;
}
/**
* Gets the value of the privateDataList property.
*
* @return
* possible object is
* {@link PrivateDataList }
*
*/
public PrivateDataList getPrivateDataList() {
return privateDataList;
}
/**
* Sets the value of the privateDataList property.
*
* @param value
* allowed object is
* {@link PrivateDataList }
*
*/
public void setPrivateDataList(PrivateDataList value) {
this.privateDataList = value;
}
/**
* Gets the value of the customPaymentTemplateURL property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCustomPaymentTemplateURL() {
return customPaymentTemplateURL;
}
/**
* Sets the value of the customPaymentTemplateURL property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setCustomPaymentTemplateURL(String value) {
this.customPaymentTemplateURL = value;
}
/**
* Gets the value of the contractNumberWalletList property.
*
* @return
* possible object is
* {@link ContractNumberWalletList }
*
*/
public ContractNumberWalletList getContractNumberWalletList() {
return contractNumberWalletList;
}
/**
* Sets the value of the contractNumberWalletList property.
*
* @param value
* allowed object is
* {@link ContractNumberWalletList }
*
*/
public void setContractNumberWalletList(ContractNumberWalletList value) {
this.contractNumberWalletList = value;
}
/**
* Gets the value of the merchantName property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getMerchantName() {
return merchantName;
}
/**
* Sets the value of the merchantName property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setMerchantName(String value) {
this.merchantName = value;
}
/**
* Gets the value of the threeDSInfo property.
*
* @return
* possible object is
* {@link ThreeDSInfo }
*
*/
public ThreeDSInfo getThreeDSInfo() {
return threeDSInfo;
}
/**
* Sets the value of the threeDSInfo property.
*
* @param value
* allowed object is
* {@link ThreeDSInfo }
*
*/
public void setThreeDSInfo(ThreeDSInfo value) {
this.threeDSInfo = value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy