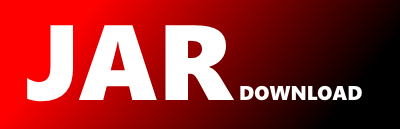
com.payline.ws.model.Order Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of payline-java-sdk Show documentation
Show all versions of payline-java-sdk Show documentation
The Payline API provides access to the various functions of the Payline payment solution. It is based on standard web service components, which include the SOAP protocol, the WSDL and XSD definition languages. These standards are supported by a large range of development tools on multiple platforms. This SDK covers all the functions of the Payline payment solution.
package com.payline.ws.model;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlType;
/**
*
* This element contains information about the
* order
*
*
* Java class for order complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="order">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="ref" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="origin" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="country" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="taxes" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="amount" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="currency" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="date" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="details" type="{http://obj.ws.payline.experian.com}details" minOccurs="0"/>
* <element name="deliveryTime" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="deliveryMode" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="deliveryExpectedDate" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="deliveryExpectedDelay" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="deliveryCharge" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="discountAmount" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="otaPackageType" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="otaDestinationCountry" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="bookingReference" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="orderDetail" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="orderExtended" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="orderOTA" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "order", namespace = "http://obj.ws.payline.experian.com", propOrder = {
"ref",
"origin",
"country",
"taxes",
"amount",
"currency",
"date",
"details",
"deliveryTime",
"deliveryMode",
"deliveryExpectedDate",
"deliveryExpectedDelay",
"deliveryCharge",
"discountAmount",
"otaPackageType",
"otaDestinationCountry",
"bookingReference",
"orderDetail",
"orderExtended",
"orderOTA"
})
public class Order {
@XmlElement(required = true)
protected String ref;
@XmlElement(nillable = true)
protected String origin;
@XmlElement(nillable = true)
protected String country;
@XmlElement(nillable = true)
protected String taxes;
@XmlElement(required = true)
protected String amount;
@XmlElement(required = true)
protected String currency;
@XmlElement(required = true)
protected String date;
@XmlElement(nillable = true)
protected Details details;
@XmlElement(nillable = true)
protected String deliveryTime;
@XmlElement(nillable = true)
protected String deliveryMode;
@XmlElement(nillable = true)
protected String deliveryExpectedDate;
@XmlElement(nillable = true)
protected String deliveryExpectedDelay;
@XmlElement(nillable = true)
protected String deliveryCharge;
@XmlElement(nillable = true)
protected String discountAmount;
@XmlElement(nillable = true)
protected String otaPackageType;
@XmlElement(nillable = true)
protected String otaDestinationCountry;
@XmlElement(nillable = true)
protected String bookingReference;
@XmlElement(nillable = true)
protected String orderDetail;
@XmlElement(nillable = true)
protected String orderExtended;
@XmlElement(nillable = true)
protected String orderOTA;
/**
* Gets the value of the ref property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getRef() {
return ref;
}
/**
* Sets the value of the ref property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setRef(String value) {
this.ref = value;
}
/**
* Gets the value of the origin property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getOrigin() {
return origin;
}
/**
* Sets the value of the origin property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setOrigin(String value) {
this.origin = value;
}
/**
* Gets the value of the country property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCountry() {
return country;
}
/**
* Sets the value of the country property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setCountry(String value) {
this.country = value;
}
/**
* Gets the value of the taxes property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTaxes() {
return taxes;
}
/**
* Sets the value of the taxes property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setTaxes(String value) {
this.taxes = value;
}
/**
* Gets the value of the amount property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getAmount() {
return amount;
}
/**
* Sets the value of the amount property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setAmount(String value) {
this.amount = value;
}
/**
* Gets the value of the currency property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCurrency() {
return currency;
}
/**
* Sets the value of the currency property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setCurrency(String value) {
this.currency = value;
}
/**
* Gets the value of the date property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getDate() {
return date;
}
/**
* Sets the value of the date property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setDate(String value) {
this.date = value;
}
/**
* Gets the value of the details property.
*
* @return
* possible object is
* {@link Details }
*
*/
public Details getDetails() {
return details;
}
/**
* Sets the value of the details property.
*
* @param value
* allowed object is
* {@link Details }
*
*/
public void setDetails(Details value) {
this.details = value;
}
/**
* Gets the value of the deliveryTime property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getDeliveryTime() {
return deliveryTime;
}
/**
* Sets the value of the deliveryTime property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setDeliveryTime(String value) {
this.deliveryTime = value;
}
/**
* Gets the value of the deliveryMode property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getDeliveryMode() {
return deliveryMode;
}
/**
* Sets the value of the deliveryMode property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setDeliveryMode(String value) {
this.deliveryMode = value;
}
/**
* Gets the value of the deliveryExpectedDate property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getDeliveryExpectedDate() {
return deliveryExpectedDate;
}
/**
* Sets the value of the deliveryExpectedDate property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setDeliveryExpectedDate(String value) {
this.deliveryExpectedDate = value;
}
/**
* Gets the value of the deliveryExpectedDelay property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getDeliveryExpectedDelay() {
return deliveryExpectedDelay;
}
/**
* Sets the value of the deliveryExpectedDelay property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setDeliveryExpectedDelay(String value) {
this.deliveryExpectedDelay = value;
}
/**
* Gets the value of the deliveryCharge property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getDeliveryCharge() {
return deliveryCharge;
}
/**
* Sets the value of the deliveryCharge property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setDeliveryCharge(String value) {
this.deliveryCharge = value;
}
/**
* Gets the value of the discountAmount property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getDiscountAmount() {
return discountAmount;
}
/**
* Sets the value of the discountAmount property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setDiscountAmount(String value) {
this.discountAmount = value;
}
/**
* Gets the value of the otaPackageType property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getOtaPackageType() {
return otaPackageType;
}
/**
* Sets the value of the otaPackageType property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setOtaPackageType(String value) {
this.otaPackageType = value;
}
/**
* Gets the value of the otaDestinationCountry property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getOtaDestinationCountry() {
return otaDestinationCountry;
}
/**
* Sets the value of the otaDestinationCountry property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setOtaDestinationCountry(String value) {
this.otaDestinationCountry = value;
}
/**
* Gets the value of the bookingReference property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getBookingReference() {
return bookingReference;
}
/**
* Sets the value of the bookingReference property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setBookingReference(String value) {
this.bookingReference = value;
}
/**
* Gets the value of the orderDetail property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getOrderDetail() {
return orderDetail;
}
/**
* Sets the value of the orderDetail property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setOrderDetail(String value) {
this.orderDetail = value;
}
/**
* Gets the value of the orderExtended property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getOrderExtended() {
return orderExtended;
}
/**
* Sets the value of the orderExtended property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setOrderExtended(String value) {
this.orderExtended = value;
}
/**
* Gets the value of the orderOTA property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getOrderOTA() {
return orderOTA;
}
/**
* Sets the value of the orderOTA property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setOrderOTA(String value) {
this.orderOTA = value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy