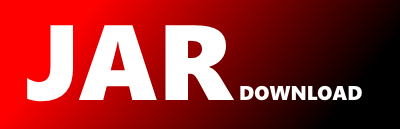
com.payline.ws.model.OrderDetail Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of payline-java-sdk Show documentation
Show all versions of payline-java-sdk Show documentation
The Payline API provides access to the various functions of the Payline payment solution. It is based on standard web service components, which include the SOAP protocol, the WSDL and XSD definition languages. These standards are supported by a large range of development tools on multiple platforms. This SDK covers all the functions of the Payline payment solution.
package com.payline.ws.model;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlType;
/**
*
* This element contains information about the
* order
* product
*
*
* Java class for orderDetail complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="orderDetail">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="ref" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="price" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="quantity" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="comment" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="category" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="brand" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="subcategory1" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="subcategory2" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="additionalData" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="taxRate" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="seller" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="sellerType" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "orderDetail", namespace = "http://obj.ws.payline.experian.com", propOrder = {
"ref",
"price",
"quantity",
"comment",
"category",
"brand",
"subcategory1",
"subcategory2",
"additionalData",
"taxRate",
"seller",
"sellerType"
})
public class OrderDetail {
@XmlElement(nillable = true)
protected String ref;
@XmlElement(nillable = true)
protected String price;
@XmlElement(nillable = true)
protected String quantity;
@XmlElement(nillable = true)
protected String comment;
@XmlElement(nillable = true)
protected String category;
@XmlElement(nillable = true)
protected String brand;
@XmlElement(nillable = true)
protected String subcategory1;
@XmlElement(nillable = true)
protected String subcategory2;
@XmlElement(nillable = true)
protected String additionalData;
@XmlElement(nillable = true)
protected String taxRate;
@XmlElement(nillable = true)
protected String seller;
@XmlElement(nillable = true)
protected String sellerType;
/**
* Gets the value of the ref property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getRef() {
return ref;
}
/**
* Sets the value of the ref property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setRef(String value) {
this.ref = value;
}
/**
* Gets the value of the price property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getPrice() {
return price;
}
/**
* Sets the value of the price property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setPrice(String value) {
this.price = value;
}
/**
* Gets the value of the quantity property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getQuantity() {
return quantity;
}
/**
* Sets the value of the quantity property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setQuantity(String value) {
this.quantity = value;
}
/**
* Gets the value of the comment property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getComment() {
return comment;
}
/**
* Sets the value of the comment property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setComment(String value) {
this.comment = value;
}
/**
* Gets the value of the category property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCategory() {
return category;
}
/**
* Sets the value of the category property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setCategory(String value) {
this.category = value;
}
/**
* Gets the value of the brand property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getBrand() {
return brand;
}
/**
* Sets the value of the brand property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setBrand(String value) {
this.brand = value;
}
/**
* Gets the value of the subcategory1 property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getSubcategory1() {
return subcategory1;
}
/**
* Sets the value of the subcategory1 property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setSubcategory1(String value) {
this.subcategory1 = value;
}
/**
* Gets the value of the subcategory2 property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getSubcategory2() {
return subcategory2;
}
/**
* Sets the value of the subcategory2 property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setSubcategory2(String value) {
this.subcategory2 = value;
}
/**
* Gets the value of the additionalData property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getAdditionalData() {
return additionalData;
}
/**
* Sets the value of the additionalData property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setAdditionalData(String value) {
this.additionalData = value;
}
/**
* Gets the value of the taxRate property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTaxRate() {
return taxRate;
}
/**
* Sets the value of the taxRate property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setTaxRate(String value) {
this.taxRate = value;
}
/**
* Gets the value of the seller property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getSeller() {
return seller;
}
/**
* Sets the value of the seller property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setSeller(String value) {
this.seller = value;
}
/**
* Gets the value of the sellerType property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getSellerType() {
return sellerType;
}
/**
* Sets the value of the sellerType property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setSellerType(String value) {
this.sellerType = value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy