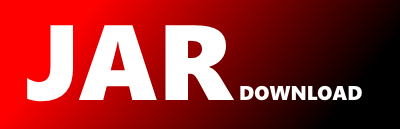
com.payline.ws.model.PaymentAdditional Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of payline-java-sdk Show documentation
Show all versions of payline-java-sdk Show documentation
The Payline API provides access to the various functions of the Payline payment solution. It is based on standard web service components, which include the SOAP protocol, the WSDL and XSD definition languages. These standards are supported by a large range of development tools on multiple platforms. This SDK covers all the functions of the Payline payment solution.
package com.payline.ws.model;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlType;
/**
*
* This element contains information about the
* paymentAdditional
*
*
* Java class for paymentAdditional complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="paymentAdditional">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="transaction" type="{http://obj.ws.payline.experian.com}transaction"/>
* <element name="payment" type="{http://obj.ws.payline.experian.com}payment"/>
* <element name="authorization" type="{http://obj.ws.payline.experian.com}authorization"/>
* <element name="authentication3DSecure" type="{http://obj.ws.payline.experian.com}authentication3DSecure" minOccurs="0"/>
* <element name="card" type="{http://obj.ws.payline.experian.com}cardOut" minOccurs="0"/>
* <element name="extendedCard" type="{http://obj.ws.payline.experian.com}extendedCardType" minOccurs="0"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "paymentAdditional", namespace = "http://obj.ws.payline.experian.com", propOrder = {
"transaction",
"payment",
"authorization",
"authentication3DSecure",
"card",
"extendedCard"
})
public class PaymentAdditional {
@XmlElement(required = true)
protected Transaction transaction;
@XmlElement(required = true)
protected Payment payment;
@XmlElement(required = true)
protected Authorization authorization;
@XmlElement(nillable = true)
protected Authentication3DSecure authentication3DSecure;
@XmlElement(nillable = true)
protected CardOut card;
@XmlElement(nillable = true)
protected ExtendedCardType extendedCard;
/**
* Gets the value of the transaction property.
*
* @return
* possible object is
* {@link Transaction }
*
*/
public Transaction getTransaction() {
return transaction;
}
/**
* Sets the value of the transaction property.
*
* @param value
* allowed object is
* {@link Transaction }
*
*/
public void setTransaction(Transaction value) {
this.transaction = value;
}
/**
* Gets the value of the payment property.
*
* @return
* possible object is
* {@link Payment }
*
*/
public Payment getPayment() {
return payment;
}
/**
* Sets the value of the payment property.
*
* @param value
* allowed object is
* {@link Payment }
*
*/
public void setPayment(Payment value) {
this.payment = value;
}
/**
* Gets the value of the authorization property.
*
* @return
* possible object is
* {@link Authorization }
*
*/
public Authorization getAuthorization() {
return authorization;
}
/**
* Sets the value of the authorization property.
*
* @param value
* allowed object is
* {@link Authorization }
*
*/
public void setAuthorization(Authorization value) {
this.authorization = value;
}
/**
* Gets the value of the authentication3DSecure property.
*
* @return
* possible object is
* {@link Authentication3DSecure }
*
*/
public Authentication3DSecure getAuthentication3DSecure() {
return authentication3DSecure;
}
/**
* Sets the value of the authentication3DSecure property.
*
* @param value
* allowed object is
* {@link Authentication3DSecure }
*
*/
public void setAuthentication3DSecure(Authentication3DSecure value) {
this.authentication3DSecure = value;
}
/**
* Gets the value of the card property.
*
* @return
* possible object is
* {@link CardOut }
*
*/
public CardOut getCard() {
return card;
}
/**
* Sets the value of the card property.
*
* @param value
* allowed object is
* {@link CardOut }
*
*/
public void setCard(CardOut value) {
this.card = value;
}
/**
* Gets the value of the extendedCard property.
*
* @return
* possible object is
* {@link ExtendedCardType }
*
*/
public ExtendedCardType getExtendedCard() {
return extendedCard;
}
/**
* Sets the value of the extendedCard property.
*
* @param value
* allowed object is
* {@link ExtendedCardType }
*
*/
public void setExtendedCard(ExtendedCardType value) {
this.extendedCard = value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy