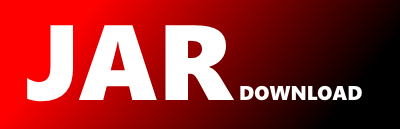
com.payline.ws.model.PointOfSell Maven / Gradle / Ivy
package com.payline.ws.model;
import java.util.ArrayList;
import java.util.List;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlType;
/**
*
* This element contains all information about point of
* sell
*
*
* Java class for pointOfSell complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="pointOfSell">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="id" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="siret" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="codeMcc">
* <simpleType>
* <restriction base="{http://www.w3.org/2001/XMLSchema}string">
* <length value="4"/>
* </restriction>
* </simpleType>
* </element>
* <element name="label" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="webmasterEmail" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="comments" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="webstoreURL" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="notificationURL" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="privateLifeURL" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="saleCondURL" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="buyerMustAcceptSaleCond" type="{http://www.w3.org/2001/XMLSchema}boolean" minOccurs="0"/>
* <element name="endOfPaymentRedirection" type="{http://www.w3.org/2001/XMLSchema}boolean" minOccurs="0"/>
* <element name="ticketSend" type="{http://obj.ws.payline.experian.com}ticketSend"/>
* <element name="contracts">
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="contract" type="{http://obj.ws.payline.experian.com}contract" maxOccurs="unbounded" minOccurs="0"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
* </element>
* <element name="virtualTerminal" type="{http://obj.ws.payline.experian.com}virtualTerminal"/>
* <element name="customPaymentPageCodeList">
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="customPaymentPageCode" type="{http://obj.ws.payline.experian.com}customPaymentPageCode" maxOccurs="unbounded" minOccurs="0"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
* </element>
* <element name="reference" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "pointOfSell", namespace = "http://obj.ws.payline.experian.com", propOrder = {
"id",
"siret",
"codeMcc",
"label",
"webmasterEmail",
"comments",
"webstoreURL",
"notificationURL",
"privateLifeURL",
"saleCondURL",
"buyerMustAcceptSaleCond",
"endOfPaymentRedirection",
"ticketSend",
"contracts",
"virtualTerminal",
"customPaymentPageCodeList",
"reference"
})
public class PointOfSell {
@XmlElement(nillable = true)
protected String id;
@XmlElement(required = true, nillable = true)
protected String siret;
@XmlElement(required = true, nillable = true)
protected String codeMcc;
@XmlElement(required = true, nillable = true)
protected String label;
@XmlElement(required = true, nillable = true)
protected String webmasterEmail;
@XmlElement(nillable = true)
protected String comments;
@XmlElement(required = true, nillable = true)
protected String webstoreURL;
@XmlElement(required = true, nillable = true)
protected String notificationURL;
@XmlElement(nillable = true)
protected String privateLifeURL;
@XmlElement(nillable = true)
protected String saleCondURL;
@XmlElement(nillable = true)
protected Boolean buyerMustAcceptSaleCond;
@XmlElement(nillable = true)
protected Boolean endOfPaymentRedirection;
@XmlElement(required = true, nillable = true)
protected TicketSend ticketSend;
@XmlElement(required = true)
protected PointOfSell.Contracts contracts;
@XmlElement(required = true, nillable = true)
protected VirtualTerminal virtualTerminal;
@XmlElement(required = true)
protected PointOfSell.CustomPaymentPageCodeList customPaymentPageCodeList;
@XmlElement(nillable = true)
protected String reference;
/**
* Gets the value of the id property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getId() {
return id;
}
/**
* Sets the value of the id property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setId(String value) {
this.id = value;
}
/**
* Gets the value of the siret property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getSiret() {
return siret;
}
/**
* Sets the value of the siret property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setSiret(String value) {
this.siret = value;
}
/**
* Gets the value of the codeMcc property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCodeMcc() {
return codeMcc;
}
/**
* Sets the value of the codeMcc property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setCodeMcc(String value) {
this.codeMcc = value;
}
/**
* Gets the value of the label property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getLabel() {
return label;
}
/**
* Sets the value of the label property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setLabel(String value) {
this.label = value;
}
/**
* Gets the value of the webmasterEmail property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getWebmasterEmail() {
return webmasterEmail;
}
/**
* Sets the value of the webmasterEmail property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setWebmasterEmail(String value) {
this.webmasterEmail = value;
}
/**
* Gets the value of the comments property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getComments() {
return comments;
}
/**
* Sets the value of the comments property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setComments(String value) {
this.comments = value;
}
/**
* Gets the value of the webstoreURL property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getWebstoreURL() {
return webstoreURL;
}
/**
* Sets the value of the webstoreURL property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setWebstoreURL(String value) {
this.webstoreURL = value;
}
/**
* Gets the value of the notificationURL property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getNotificationURL() {
return notificationURL;
}
/**
* Sets the value of the notificationURL property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setNotificationURL(String value) {
this.notificationURL = value;
}
/**
* Gets the value of the privateLifeURL property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getPrivateLifeURL() {
return privateLifeURL;
}
/**
* Sets the value of the privateLifeURL property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setPrivateLifeURL(String value) {
this.privateLifeURL = value;
}
/**
* Gets the value of the saleCondURL property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getSaleCondURL() {
return saleCondURL;
}
/**
* Sets the value of the saleCondURL property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setSaleCondURL(String value) {
this.saleCondURL = value;
}
/**
* Gets the value of the buyerMustAcceptSaleCond property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isBuyerMustAcceptSaleCond() {
return buyerMustAcceptSaleCond;
}
/**
* Sets the value of the buyerMustAcceptSaleCond property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setBuyerMustAcceptSaleCond(Boolean value) {
this.buyerMustAcceptSaleCond = value;
}
/**
* Gets the value of the endOfPaymentRedirection property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isEndOfPaymentRedirection() {
return endOfPaymentRedirection;
}
/**
* Sets the value of the endOfPaymentRedirection property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setEndOfPaymentRedirection(Boolean value) {
this.endOfPaymentRedirection = value;
}
/**
* Gets the value of the ticketSend property.
*
* @return
* possible object is
* {@link TicketSend }
*
*/
public TicketSend getTicketSend() {
return ticketSend;
}
/**
* Sets the value of the ticketSend property.
*
* @param value
* allowed object is
* {@link TicketSend }
*
*/
public void setTicketSend(TicketSend value) {
this.ticketSend = value;
}
/**
* Gets the value of the contracts property.
*
* @return
* possible object is
* {@link PointOfSell.Contracts }
*
*/
public PointOfSell.Contracts getContracts() {
return contracts;
}
/**
* Sets the value of the contracts property.
*
* @param value
* allowed object is
* {@link PointOfSell.Contracts }
*
*/
public void setContracts(PointOfSell.Contracts value) {
this.contracts = value;
}
/**
* Gets the value of the virtualTerminal property.
*
* @return
* possible object is
* {@link VirtualTerminal }
*
*/
public VirtualTerminal getVirtualTerminal() {
return virtualTerminal;
}
/**
* Sets the value of the virtualTerminal property.
*
* @param value
* allowed object is
* {@link VirtualTerminal }
*
*/
public void setVirtualTerminal(VirtualTerminal value) {
this.virtualTerminal = value;
}
/**
* Gets the value of the customPaymentPageCodeList property.
*
* @return
* possible object is
* {@link PointOfSell.CustomPaymentPageCodeList }
*
*/
public PointOfSell.CustomPaymentPageCodeList getCustomPaymentPageCodeList() {
return customPaymentPageCodeList;
}
/**
* Sets the value of the customPaymentPageCodeList property.
*
* @param value
* allowed object is
* {@link PointOfSell.CustomPaymentPageCodeList }
*
*/
public void setCustomPaymentPageCodeList(PointOfSell.CustomPaymentPageCodeList value) {
this.customPaymentPageCodeList = value;
}
/**
* Gets the value of the reference property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getReference() {
return reference;
}
/**
* Sets the value of the reference property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setReference(String value) {
this.reference = value;
}
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="contract" type="{http://obj.ws.payline.experian.com}contract" maxOccurs="unbounded" minOccurs="0"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"contract"
})
public static class Contracts {
@XmlElement(namespace = "http://obj.ws.payline.experian.com")
protected List contract;
/**
* Gets the value of the contract property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the contract property.
*
*
* For example, to add a new item, do as follows:
*
* getContract().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Contract }
*
*
*/
public List getContract() {
if (contract == null) {
contract = new ArrayList();
}
return this.contract;
}
}
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="customPaymentPageCode" type="{http://obj.ws.payline.experian.com}customPaymentPageCode" maxOccurs="unbounded" minOccurs="0"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"customPaymentPageCode"
})
public static class CustomPaymentPageCodeList {
@XmlElement(namespace = "http://obj.ws.payline.experian.com")
protected List customPaymentPageCode;
/**
* Gets the value of the customPaymentPageCode property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the customPaymentPageCode property.
*
*
* For example, to add a new item, do as follows:
*
* getCustomPaymentPageCode().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link CustomPaymentPageCode }
*
*
*/
public List getCustomPaymentPageCode() {
if (customPaymentPageCode == null) {
customPaymentPageCode = new ArrayList();
}
return this.customPaymentPageCode;
}
}
}