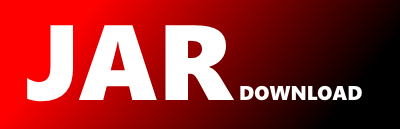
com.payline.ws.model.StatusHistory Maven / Gradle / Ivy
package com.payline.ws.model;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlType;
/**
*
* This element contains information about the
* status
* History
*
*
* Java class for statusHistory complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="statusHistory">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="transactionId" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="date" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="amount" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="fees" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="status" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="originTransactionId" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="amountValue" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="amountCurrency" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="feesValue" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="feesCurrency" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "statusHistory", namespace = "http://obj.ws.payline.experian.com", propOrder = {
"transactionId",
"date",
"amount",
"fees",
"status",
"originTransactionId",
"amountValue",
"amountCurrency",
"feesValue",
"feesCurrency"
})
public class StatusHistory {
@XmlElement(required = true)
protected String transactionId;
@XmlElement(required = true)
protected String date;
@XmlElement(required = true)
protected String amount;
@XmlElement(required = true)
protected String fees;
@XmlElement(required = true)
protected String status;
@XmlElement(required = true)
protected String originTransactionId;
@XmlElement(nillable = true)
protected String amountValue;
@XmlElement(nillable = true)
protected String amountCurrency;
@XmlElement(nillable = true)
protected String feesValue;
@XmlElement(nillable = true)
protected String feesCurrency;
/**
* Gets the value of the transactionId property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTransactionId() {
return transactionId;
}
/**
* Sets the value of the transactionId property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setTransactionId(String value) {
this.transactionId = value;
}
/**
* Gets the value of the date property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getDate() {
return date;
}
/**
* Sets the value of the date property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setDate(String value) {
this.date = value;
}
/**
* Gets the value of the amount property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getAmount() {
return amount;
}
/**
* Sets the value of the amount property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setAmount(String value) {
this.amount = value;
}
/**
* Gets the value of the fees property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getFees() {
return fees;
}
/**
* Sets the value of the fees property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setFees(String value) {
this.fees = value;
}
/**
* Gets the value of the status property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getStatus() {
return status;
}
/**
* Sets the value of the status property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setStatus(String value) {
this.status = value;
}
/**
* Gets the value of the originTransactionId property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getOriginTransactionId() {
return originTransactionId;
}
/**
* Sets the value of the originTransactionId property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setOriginTransactionId(String value) {
this.originTransactionId = value;
}
/**
* Gets the value of the amountValue property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getAmountValue() {
return amountValue;
}
/**
* Sets the value of the amountValue property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setAmountValue(String value) {
this.amountValue = value;
}
/**
* Gets the value of the amountCurrency property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getAmountCurrency() {
return amountCurrency;
}
/**
* Sets the value of the amountCurrency property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setAmountCurrency(String value) {
this.amountCurrency = value;
}
/**
* Gets the value of the feesValue property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getFeesValue() {
return feesValue;
}
/**
* Sets the value of the feesValue property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setFeesValue(String value) {
this.feesValue = value;
}
/**
* Gets the value of the feesCurrency property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getFeesCurrency() {
return feesCurrency;
}
/**
* Sets the value of the feesCurrency property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setFeesCurrency(String value) {
this.feesCurrency = value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy