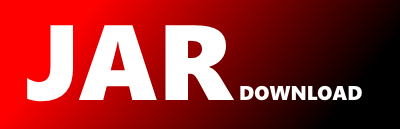
com.payline.ws.model.Transaction Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of payline-java-sdk Show documentation
Show all versions of payline-java-sdk Show documentation
The Payline API provides access to the various functions of the Payline payment solution. It is based on standard web service components, which include the SOAP protocol, the WSDL and XSD definition languages. These standards are supported by a large range of development tools on multiple platforms. This SDK covers all the functions of the Payline payment solution.
package com.payline.ws.model;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlType;
/**
*
* This element contains information about the
* transaction
*
*
* Java class for transaction complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="transaction">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="id" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="date" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="isDuplicated" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="isPossibleFraud" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="fraudResult" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="fraudResultDetails" type="{http://obj.ws.payline.experian.com}fraudResultDetails" minOccurs="0"/>
* <element name="explanation" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="threeDSecure" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="softDescriptor" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="score" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="scoring" type="{http://obj.ws.payline.experian.com}scoring" minOccurs="0"/>
* <element name="externalWalletType" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="externalWalletContractNumber" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="partnerAdditionalData" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="avs" type="{http://obj.ws.payline.experian.com}avs" minOccurs="0"/>
* <element name="customerId" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="type" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="orderReference" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="payment" type="{http://obj.ws.payline.experian.com}payment" minOccurs="0"/>
* <element name="pointOfSell" type="{http://obj.ws.payline.experian.com}pointOfSell" minOccurs="0"/>
* <element name="card" type="{http://obj.ws.payline.experian.com}cardOut" minOccurs="0"/>
* <element name="extendedCard" type="{http://obj.ws.payline.experian.com}extendedCardType" minOccurs="0"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "transaction", namespace = "http://obj.ws.payline.experian.com", propOrder = {
"id",
"date",
"isDuplicated",
"isPossibleFraud",
"fraudResult",
"fraudResultDetails",
"explanation",
"threeDSecure",
"softDescriptor",
"score",
"scoring",
"externalWalletType",
"externalWalletContractNumber",
"partnerAdditionalData",
"avs",
"customerId",
"type",
"orderReference",
"payment",
"pointOfSell",
"card",
"extendedCard"
})
public class Transaction {
@XmlElement(required = true)
protected String id;
@XmlElement(required = true)
protected String date;
@XmlElement(nillable = true)
protected String isDuplicated;
@XmlElement(required = true, nillable = true)
protected String isPossibleFraud;
@XmlElement(nillable = true)
protected String fraudResult;
@XmlElement(nillable = true)
protected FraudResultDetails fraudResultDetails;
@XmlElement(nillable = true)
protected String explanation;
@XmlElement(nillable = true)
protected String threeDSecure;
@XmlElement(nillable = true)
protected String softDescriptor;
@XmlElement(nillable = true)
protected String score;
@XmlElement(nillable = true)
protected Scoring scoring;
@XmlElement(nillable = true)
protected String externalWalletType;
@XmlElement(nillable = true)
protected String externalWalletContractNumber;
@XmlElement(nillable = true)
protected String partnerAdditionalData;
@XmlElement(nillable = true)
protected Avs avs;
@XmlElement(nillable = true)
protected String customerId;
protected String type;
@XmlElement(nillable = true)
protected String orderReference;
@XmlElement(nillable = true)
protected Payment payment;
@XmlElement(nillable = true)
protected PointOfSell pointOfSell;
@XmlElement(nillable = true)
protected CardOut card;
@XmlElement(nillable = true)
protected ExtendedCardType extendedCard;
/**
* Gets the value of the id property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getId() {
return id;
}
/**
* Sets the value of the id property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setId(String value) {
this.id = value;
}
/**
* Gets the value of the date property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getDate() {
return date;
}
/**
* Sets the value of the date property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setDate(String value) {
this.date = value;
}
/**
* Gets the value of the isDuplicated property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getIsDuplicated() {
return isDuplicated;
}
/**
* Sets the value of the isDuplicated property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setIsDuplicated(String value) {
this.isDuplicated = value;
}
/**
* Gets the value of the isPossibleFraud property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getIsPossibleFraud() {
return isPossibleFraud;
}
/**
* Sets the value of the isPossibleFraud property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setIsPossibleFraud(String value) {
this.isPossibleFraud = value;
}
/**
* Gets the value of the fraudResult property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getFraudResult() {
return fraudResult;
}
/**
* Sets the value of the fraudResult property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setFraudResult(String value) {
this.fraudResult = value;
}
/**
* Gets the value of the fraudResultDetails property.
*
* @return
* possible object is
* {@link FraudResultDetails }
*
*/
public FraudResultDetails getFraudResultDetails() {
return fraudResultDetails;
}
/**
* Sets the value of the fraudResultDetails property.
*
* @param value
* allowed object is
* {@link FraudResultDetails }
*
*/
public void setFraudResultDetails(FraudResultDetails value) {
this.fraudResultDetails = value;
}
/**
* Gets the value of the explanation property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getExplanation() {
return explanation;
}
/**
* Sets the value of the explanation property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setExplanation(String value) {
this.explanation = value;
}
/**
* Gets the value of the threeDSecure property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getThreeDSecure() {
return threeDSecure;
}
/**
* Sets the value of the threeDSecure property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setThreeDSecure(String value) {
this.threeDSecure = value;
}
/**
* Gets the value of the softDescriptor property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getSoftDescriptor() {
return softDescriptor;
}
/**
* Sets the value of the softDescriptor property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setSoftDescriptor(String value) {
this.softDescriptor = value;
}
/**
* Gets the value of the score property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getScore() {
return score;
}
/**
* Sets the value of the score property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setScore(String value) {
this.score = value;
}
/**
* Gets the value of the scoring property.
*
* @return
* possible object is
* {@link Scoring }
*
*/
public Scoring getScoring() {
return scoring;
}
/**
* Sets the value of the scoring property.
*
* @param value
* allowed object is
* {@link Scoring }
*
*/
public void setScoring(Scoring value) {
this.scoring = value;
}
/**
* Gets the value of the externalWalletType property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getExternalWalletType() {
return externalWalletType;
}
/**
* Sets the value of the externalWalletType property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setExternalWalletType(String value) {
this.externalWalletType = value;
}
/**
* Gets the value of the externalWalletContractNumber property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getExternalWalletContractNumber() {
return externalWalletContractNumber;
}
/**
* Sets the value of the externalWalletContractNumber property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setExternalWalletContractNumber(String value) {
this.externalWalletContractNumber = value;
}
/**
* Gets the value of the partnerAdditionalData property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getPartnerAdditionalData() {
return partnerAdditionalData;
}
/**
* Sets the value of the partnerAdditionalData property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setPartnerAdditionalData(String value) {
this.partnerAdditionalData = value;
}
/**
* Gets the value of the avs property.
*
* @return
* possible object is
* {@link Avs }
*
*/
public Avs getAvs() {
return avs;
}
/**
* Sets the value of the avs property.
*
* @param value
* allowed object is
* {@link Avs }
*
*/
public void setAvs(Avs value) {
this.avs = value;
}
/**
* Gets the value of the customerId property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCustomerId() {
return customerId;
}
/**
* Sets the value of the customerId property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setCustomerId(String value) {
this.customerId = value;
}
/**
* Gets the value of the type property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getType() {
return type;
}
/**
* Sets the value of the type property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setType(String value) {
this.type = value;
}
/**
* Gets the value of the orderReference property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getOrderReference() {
return orderReference;
}
/**
* Sets the value of the orderReference property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setOrderReference(String value) {
this.orderReference = value;
}
/**
* Gets the value of the payment property.
*
* @return
* possible object is
* {@link Payment }
*
*/
public Payment getPayment() {
return payment;
}
/**
* Sets the value of the payment property.
*
* @param value
* allowed object is
* {@link Payment }
*
*/
public void setPayment(Payment value) {
this.payment = value;
}
/**
* Gets the value of the pointOfSell property.
*
* @return
* possible object is
* {@link PointOfSell }
*
*/
public PointOfSell getPointOfSell() {
return pointOfSell;
}
/**
* Sets the value of the pointOfSell property.
*
* @param value
* allowed object is
* {@link PointOfSell }
*
*/
public void setPointOfSell(PointOfSell value) {
this.pointOfSell = value;
}
/**
* Gets the value of the card property.
*
* @return
* possible object is
* {@link CardOut }
*
*/
public CardOut getCard() {
return card;
}
/**
* Sets the value of the card property.
*
* @param value
* allowed object is
* {@link CardOut }
*
*/
public void setCard(CardOut value) {
this.card = value;
}
/**
* Gets the value of the extendedCard property.
*
* @return
* possible object is
* {@link ExtendedCardType }
*
*/
public ExtendedCardType getExtendedCard() {
return extendedCard;
}
/**
* Sets the value of the extendedCard property.
*
* @param value
* allowed object is
* {@link ExtendedCardType }
*
*/
public void setExtendedCard(ExtendedCardType value) {
this.extendedCard = value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy