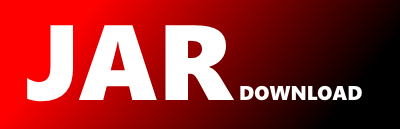
com.payline.ws.model.VerifyEnrollmentRequest Maven / Gradle / Ivy
package com.payline.ws.model;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlType;
/**
*
* This element is the request for the
* verifyEnrollment method
*
*
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="transient" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="version" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="card" type="{http://obj.ws.payline.experian.com}card"/>
* <element name="payment" type="{http://obj.ws.payline.experian.com}payment"/>
* <element name="orderRef" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="mdFieldValue" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="userAgent" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="walletId" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="walletCardInd" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="generateVirtualCvx" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="merchantName" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="merchantURL" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="merchantCountryCode" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="returnURL" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="order" type="{http://obj.ws.payline.experian.com}order"/>
* <element name="buyer" type="{http://obj.ws.payline.experian.com}buyer" minOccurs="0"/>
* <element name="subMerchant" type="{http://obj.ws.payline.experian.com}subMerchant" minOccurs="0"/>
* <element name="recurring" type="{http://obj.ws.payline.experian.com}recurring"/>
* <element name="threeDSInfo" type="{http://obj.ws.payline.experian.com}threeDSInfo"/>
* <element name="merchantScore" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="privateDataList" type="{http://obj.ws.payline.experian.com}privateDataList"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"_transient",
"version",
"card",
"payment",
"orderRef",
"mdFieldValue",
"userAgent",
"walletId",
"walletCardInd",
"generateVirtualCvx",
"merchantName",
"merchantURL",
"merchantCountryCode",
"returnURL",
"order",
"buyer",
"subMerchant",
"recurring",
"threeDSInfo",
"merchantScore",
"privateDataList"
})
@XmlRootElement(name = "verifyEnrollmentRequest")
public class VerifyEnrollmentRequest {
@XmlElement(name = "transient", required = true, nillable = true)
protected String _transient;
@XmlElement(required = true)
protected String version;
@XmlElement(required = true)
protected Card card;
@XmlElement(required = true)
protected Payment payment;
@XmlElement(required = true)
protected String orderRef;
@XmlElement(required = true, nillable = true)
protected String mdFieldValue;
@XmlElement(required = true, nillable = true)
protected String userAgent;
@XmlElement(required = true, nillable = true)
protected String walletId;
@XmlElement(required = true, nillable = true)
protected String walletCardInd;
@XmlElement(nillable = true)
protected String generateVirtualCvx;
@XmlElement(required = true, nillable = true)
protected String merchantName;
@XmlElement(nillable = true)
protected String merchantURL;
@XmlElement(nillable = true)
protected String merchantCountryCode;
@XmlElement(required = true, nillable = true)
protected String returnURL;
@XmlElement(required = true, nillable = true)
protected Order order;
@XmlElement(nillable = true)
protected Buyer buyer;
@XmlElement(nillable = true)
protected SubMerchant subMerchant;
@XmlElement(required = true, nillable = true)
protected Recurring recurring;
@XmlElement(required = true, nillable = true)
protected ThreeDSInfo threeDSInfo;
@XmlElement(required = true, nillable = true)
protected String merchantScore;
@XmlElement(required = true, nillable = true)
protected PrivateDataList privateDataList;
/**
* Gets the value of the transient property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTransient() {
return _transient;
}
/**
* Sets the value of the transient property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setTransient(String value) {
this._transient = value;
}
/**
* Gets the value of the version property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getVersion() {
return version;
}
/**
* Sets the value of the version property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setVersion(String value) {
this.version = value;
}
/**
* Gets the value of the card property.
*
* @return
* possible object is
* {@link Card }
*
*/
public Card getCard() {
return card;
}
/**
* Sets the value of the card property.
*
* @param value
* allowed object is
* {@link Card }
*
*/
public void setCard(Card value) {
this.card = value;
}
/**
* Gets the value of the payment property.
*
* @return
* possible object is
* {@link Payment }
*
*/
public Payment getPayment() {
return payment;
}
/**
* Sets the value of the payment property.
*
* @param value
* allowed object is
* {@link Payment }
*
*/
public void setPayment(Payment value) {
this.payment = value;
}
/**
* Gets the value of the orderRef property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getOrderRef() {
return orderRef;
}
/**
* Sets the value of the orderRef property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setOrderRef(String value) {
this.orderRef = value;
}
/**
* Gets the value of the mdFieldValue property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getMdFieldValue() {
return mdFieldValue;
}
/**
* Sets the value of the mdFieldValue property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setMdFieldValue(String value) {
this.mdFieldValue = value;
}
/**
* Gets the value of the userAgent property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getUserAgent() {
return userAgent;
}
/**
* Sets the value of the userAgent property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setUserAgent(String value) {
this.userAgent = value;
}
/**
* Gets the value of the walletId property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getWalletId() {
return walletId;
}
/**
* Sets the value of the walletId property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setWalletId(String value) {
this.walletId = value;
}
/**
* Gets the value of the walletCardInd property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getWalletCardInd() {
return walletCardInd;
}
/**
* Sets the value of the walletCardInd property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setWalletCardInd(String value) {
this.walletCardInd = value;
}
/**
* Gets the value of the generateVirtualCvx property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getGenerateVirtualCvx() {
return generateVirtualCvx;
}
/**
* Sets the value of the generateVirtualCvx property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setGenerateVirtualCvx(String value) {
this.generateVirtualCvx = value;
}
/**
* Gets the value of the merchantName property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getMerchantName() {
return merchantName;
}
/**
* Sets the value of the merchantName property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setMerchantName(String value) {
this.merchantName = value;
}
/**
* Gets the value of the merchantURL property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getMerchantURL() {
return merchantURL;
}
/**
* Sets the value of the merchantURL property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setMerchantURL(String value) {
this.merchantURL = value;
}
/**
* Gets the value of the merchantCountryCode property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getMerchantCountryCode() {
return merchantCountryCode;
}
/**
* Sets the value of the merchantCountryCode property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setMerchantCountryCode(String value) {
this.merchantCountryCode = value;
}
/**
* Gets the value of the returnURL property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getReturnURL() {
return returnURL;
}
/**
* Sets the value of the returnURL property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setReturnURL(String value) {
this.returnURL = value;
}
/**
* Gets the value of the order property.
*
* @return
* possible object is
* {@link Order }
*
*/
public Order getOrder() {
return order;
}
/**
* Sets the value of the order property.
*
* @param value
* allowed object is
* {@link Order }
*
*/
public void setOrder(Order value) {
this.order = value;
}
/**
* Gets the value of the buyer property.
*
* @return
* possible object is
* {@link Buyer }
*
*/
public Buyer getBuyer() {
return buyer;
}
/**
* Sets the value of the buyer property.
*
* @param value
* allowed object is
* {@link Buyer }
*
*/
public void setBuyer(Buyer value) {
this.buyer = value;
}
/**
* Gets the value of the subMerchant property.
*
* @return
* possible object is
* {@link SubMerchant }
*
*/
public SubMerchant getSubMerchant() {
return subMerchant;
}
/**
* Sets the value of the subMerchant property.
*
* @param value
* allowed object is
* {@link SubMerchant }
*
*/
public void setSubMerchant(SubMerchant value) {
this.subMerchant = value;
}
/**
* Gets the value of the recurring property.
*
* @return
* possible object is
* {@link Recurring }
*
*/
public Recurring getRecurring() {
return recurring;
}
/**
* Sets the value of the recurring property.
*
* @param value
* allowed object is
* {@link Recurring }
*
*/
public void setRecurring(Recurring value) {
this.recurring = value;
}
/**
* Gets the value of the threeDSInfo property.
*
* @return
* possible object is
* {@link ThreeDSInfo }
*
*/
public ThreeDSInfo getThreeDSInfo() {
return threeDSInfo;
}
/**
* Sets the value of the threeDSInfo property.
*
* @param value
* allowed object is
* {@link ThreeDSInfo }
*
*/
public void setThreeDSInfo(ThreeDSInfo value) {
this.threeDSInfo = value;
}
/**
* Gets the value of the merchantScore property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getMerchantScore() {
return merchantScore;
}
/**
* Sets the value of the merchantScore property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setMerchantScore(String value) {
this.merchantScore = value;
}
/**
* Gets the value of the privateDataList property.
*
* @return
* possible object is
* {@link PrivateDataList }
*
*/
public PrivateDataList getPrivateDataList() {
return privateDataList;
}
/**
* Sets the value of the privateDataList property.
*
* @param value
* allowed object is
* {@link PrivateDataList }
*
*/
public void setPrivateDataList(PrivateDataList value) {
this.privateDataList = value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy