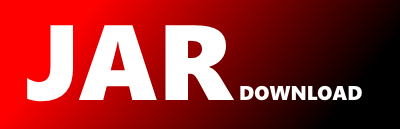
com.payneteasy.superfly.jira.provider.SuperflyPropertySet Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of superfly-jira-integration Show documentation
Show all versions of superfly-jira-integration Show documentation
Allows to use Superfly as authentication/authorization provider for JIRA
package com.payneteasy.superfly.jira.provider;
import java.util.Collection;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Map;
import java.util.Set;
import com.opensymphony.module.propertyset.AbstractPropertySet;
import com.opensymphony.module.propertyset.PropertyException;
import com.opensymphony.module.propertyset.PropertySet;
import com.opensymphony.user.User;
/**
* Provides Superfly-related property set.
*
* @author Roman Puchkovskiy
*/
public class SuperflyPropertySet extends AbstractPropertySet {
private String username;
private String email;
private Map attrsMapping;
@SuppressWarnings("unchecked")
@Override
public void init(Map config, Map args) {
super.init(config, args);
username = (String) args.get(SuperflyProfileProvider.USER_NAME);
email = (String) args.get(SuperflyProfileProvider.EMAIL);
attrsMapping = new HashMap();
}
@Override
public boolean exists(String key) throws PropertyException {
if (User.PROPERTY_FULLNAME.equals(key)) {
return true;
}
if (User.PROPERTY_EMAIL.equals(key)) {
return true;
}
return attrsMapping.containsKey(key);
}
@Override
protected Object get(int type, String key) throws PropertyException {
if (User.PROPERTY_FULLNAME.equals(key)) {
return username;
}
if (User.PROPERTY_EMAIL.equals(key)) {
return email;
}
return attrsMapping.get(key);
}
@Override
public Collection getKeys(String prefix, int type) throws PropertyException {
if (prefix == null) {
prefix = "";
}
Set result = new HashSet();
if (User.PROPERTY_FULLNAME.startsWith(prefix)) {
result.add(User.PROPERTY_FULLNAME);
}
if (User.PROPERTY_EMAIL.startsWith(prefix)) {
result.add(User.PROPERTY_EMAIL);
}
for (String key : attrsMapping.keySet()) {
if (key.startsWith(prefix)) {
result.add(key);
}
}
return result;
}
@Override
public boolean supportsType(int type) {
return type == PropertySet.STRING;
}
@Override
public int getType(String key) throws PropertyException {
if (User.PROPERTY_FULLNAME.equals(key)) {
return PropertySet.STRING;
}
if (User.PROPERTY_EMAIL.equals(key)) {
return PropertySet.STRING;
}
return exists(key) ? PropertySet.STRING : 0;
}
@Override
public void remove(String key) throws PropertyException {
// modifications are not supported
}
@Override
protected void setImpl(int type, String key, Object value)
throws PropertyException {
// modifications are not supported
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy