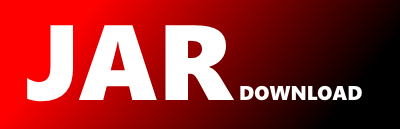
com.paypal.core.CredentialManager Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of paypal-core Show documentation
Show all versions of paypal-core Show documentation
PayPal Java SDK Core library base and common to PayPal SDKs. The paypal-core library is a dependency for all PayPal related Java SDKs
package com.paypal.core;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Map;
import java.util.Map.Entry;
import java.util.Set;
import com.paypal.core.credential.CertificateCredential;
import com.paypal.core.credential.ICredential;
import com.paypal.core.credential.SignatureCredential;
import com.paypal.core.credential.SubjectAuthorization;
import com.paypal.core.credential.ThirdPartyAuthorization;
import com.paypal.exception.InvalidCredentialException;
import com.paypal.exception.MissingCredentialException;
/**
* CredentialManager
acts as a factory for loading
* {@link ICredential} credential configured in application properties
*
*/
public final class CredentialManager {
/*
* Map used for to override ConfigManager configurations
*/
private Map configurationMap = null;
/**
* Credential Manager
*
* @param configurationMap
* {@link Map}
*/
public CredentialManager(Map configurationMap) {
if (configurationMap == null) {
throw new IllegalArgumentException(
"ConfigurationMap cannot be null");
}
this.configurationMap = configurationMap;
}
public ICredential getCredentialObject(String userId)
throws MissingCredentialException, InvalidCredentialException {
ICredential credential = null;
if (getAccounts(configurationMap).size() == 0) {
throw new MissingCredentialException(
"No API accounts have been configured in application properties");
}
String prefix = Constants.ACCOUNT_PREFIX;
Map credMap = getValuesByCategory(configurationMap,
prefix);
if (userId != null && userId.trim().length() != 0) {
for (Entry entry : credMap.entrySet()) {
if (entry.getKey().endsWith(
Constants.CREDENTIAL_USERNAME_SUFFIX)
&& entry.getValue().equalsIgnoreCase(userId)) {
String acctKey = entry.getKey().substring(0,
entry.getKey().indexOf('.'));
credential = returnCredential(credMap, acctKey);
}
}
if (credential == null) {
throw new MissingCredentialException(
"Account for the username does not exists in the properties file");
}
} else {
int index = 1;
String userName = (String) credMap.get(prefix + index
+ Constants.CREDENTIAL_USERNAME_SUFFIX);
if (userName != null && userName.trim().length() != 0) {
credential = returnCredential(credMap, prefix + index);
} else {
throw new MissingCredentialException(
"Associate valid account for index 1");
}
}
return credential;
}
private Set getAccounts(Map configurationMap) {
String key = Constants.EMPTY_STRING;
Set set = new HashSet();
for (Object obj : configurationMap.keySet()) {
key = (String) obj;
if (key.contains("acct")) {
int pos = key.indexOf('.');
String acct = key.substring(0, pos);
set.add(acct);
}
}
return set;
}
private Map getValuesByCategory(
Map configurationMap, String category) {
String key = Constants.EMPTY_STRING;
HashMap map = new HashMap();
for (Object obj : configurationMap.keySet()) {
key = (String) obj;
if (key.contains(category)) {
map.put(key, configurationMap.get(key));
}
}
return map;
}
private ICredential returnCredential(Map credMap,
String acctKey) throws InvalidCredentialException {
ICredential credential = null;
String userName = (String) credMap.get(acctKey
+ Constants.CREDENTIAL_USERNAME_SUFFIX);
String password = (String) credMap.get(acctKey
+ Constants.CREDENTIAL_PASSWORD_SUFFIX);
String appId = (String) credMap.get(acctKey
+ Constants.CREDENTIAL_APPLICATIONID_SUFFIX);
String subject = (String) credMap.get(acctKey
+ Constants.CREDENTIAL_SUBJECT_SUFFIX);
if (credMap.get(acctKey + Constants.CREDENTIAL_SIGNATURE_SUFFIX) != null) {
String signature = (String) credMap.get(acctKey
+ Constants.CREDENTIAL_SIGNATURE_SUFFIX);
credential = new SignatureCredential(userName, password, signature);
((SignatureCredential) credential).setApplicationId(appId);
if (subject != null && subject.trim().length() > 0) {
ThirdPartyAuthorization thirdPartyAuthorization = new SubjectAuthorization(
subject);
((SignatureCredential) credential)
.setThirdPartyAuthorization(thirdPartyAuthorization);
}
} else if (credMap.get(acctKey + Constants.CREDENTIAL_CERTPATH_SUFFIX) != null) {
String certPath = (String) credMap.get(acctKey
+ Constants.CREDENTIAL_CERTPATH_SUFFIX);
String certKey = (String) credMap.get(acctKey
+ Constants.CREDENTIAL_CERTKEY_SUFFIX);
credential = new CertificateCredential(userName, password,
certPath, certKey);
((CertificateCredential) credential).setApplicationId(appId);
if (subject != null && subject.trim().length() > 0) {
ThirdPartyAuthorization thirdPartyAuthorization = new SubjectAuthorization(
subject);
((CertificateCredential) credential)
.setThirdPartyAuthorization(thirdPartyAuthorization);
}
} else {
throw new InvalidCredentialException(
"The account does not have a valid credential type(signature/certificate)");
}
return credential;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy