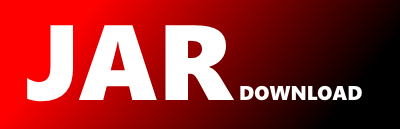
com.paypal.core.soap.CertificateSOAPHeaderAuthStrategy Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of paypal-core Show documentation
Show all versions of paypal-core Show documentation
PayPal Java SDK Core library base and common to PayPal SDKs. The paypal-core library is a dependency for all PayPal related Java SDKs
package com.paypal.core.soap;
import com.paypal.core.AuthenticationStrategy;
import com.paypal.core.credential.CertificateCredential;
import com.paypal.core.credential.SubjectAuthorization;
import com.paypal.core.credential.ThirdPartyAuthorization;
import com.paypal.core.credential.TokenAuthorization;
/**
* CertificateSOAPHeaderAuthStrategy
is an implementation of
* {@link AuthenticationStrategy} which acts on {@link CertificateCredential}
* and retrieves them as SOAP headers
*
*/
public class CertificateSOAPHeaderAuthStrategy implements
AuthenticationStrategy {
/**
* Instance of ThirdPartyAuthorization
*/
private ThirdPartyAuthorization thirdPartyAuthorization;
public CertificateSOAPHeaderAuthStrategy() {
}
/**
* @return the thirdPartyAuthorization
*/
public ThirdPartyAuthorization getThirdPartyAuthorization() {
return thirdPartyAuthorization;
}
/**
* @param thirdPartyAuthorization
* the thirdPartyAuthorization to set
*/
public void setThirdPartyAuthorization(
ThirdPartyAuthorization thirdPartyAuthorization) {
this.thirdPartyAuthorization = thirdPartyAuthorization;
}
public String generateHeaderStrategy(CertificateCredential credential) {
String payLoad = null;
if (thirdPartyAuthorization instanceof TokenAuthorization) {
payLoad = tokenAuthPayLoad();
} else if (thirdPartyAuthorization instanceof SubjectAuthorization) {
payLoad = authPayLoad(credential,
(SubjectAuthorization) thirdPartyAuthorization);
} else {
payLoad = authPayLoad(credential, null);
}
return payLoad;
}
/**
* Returns a empty soap header String, token authorization does not bear a
* credential part
*
* @return
*/
private String tokenAuthPayLoad() {
StringBuilder soapMsg = new StringBuilder();
soapMsg.append(" ");
return soapMsg.toString();
}
private String authPayLoad(CertificateCredential credential,
SubjectAuthorization subjectAuth) {
StringBuilder soapMsg = new StringBuilder();
soapMsg.append("");
soapMsg.append("");
soapMsg.append("" + credential.getUserName()
+ " ");
soapMsg.append("" + credential.getPassword()
+ " ");
// Append subject credential if available
if (subjectAuth != null) {
soapMsg.append("" + subjectAuth.getSubject()
+ " ");
}
soapMsg.append(" ");
soapMsg.append(" ");
return soapMsg.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy