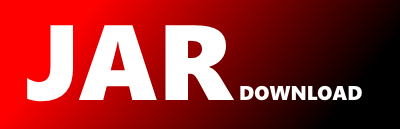
com.paypal.api.payments.Card3dSecureInfo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rest-api-sdk Show documentation
Show all versions of rest-api-sdk Show documentation
PayPal SDK for integrating with the REST APIs
// Generated by delombok at Wed Oct 12 18:15:55 CDT 2016
package com.paypal.api.payments;
import com.paypal.base.rest.PayPalModel;
public class Card3dSecureInfo extends PayPalModel {
/**
* Authorization status from 3ds provider. Should be echoed back in the response
*/
private String authStatus;
/**
* Numeric flag to indicate how the payment should be processed in relationship to 3d-secure. If 0 then ignore all 3d values and process as non-3ds
*/
private String eci;
/**
* Cardholder Authentication Verification Value (used by VISA).
*/
private String cavv;
/**
* Transaction identifier from authenticator.
*/
private String xid;
/**
* Name of the actual 3ds vendor who processed the 3ds request, e.g. Cardinal
*/
private String mpiVendor;
/**
* Default Constructor
*/
public Card3dSecureInfo() {
}
/**
* Authorization status from 3ds provider. Should be echoed back in the response
*/
@java.lang.SuppressWarnings("all")
public String getAuthStatus() {
return this.authStatus;
}
/**
* Numeric flag to indicate how the payment should be processed in relationship to 3d-secure. If 0 then ignore all 3d values and process as non-3ds
*/
@java.lang.SuppressWarnings("all")
public String getEci() {
return this.eci;
}
/**
* Cardholder Authentication Verification Value (used by VISA).
*/
@java.lang.SuppressWarnings("all")
public String getCavv() {
return this.cavv;
}
/**
* Transaction identifier from authenticator.
*/
@java.lang.SuppressWarnings("all")
public String getXid() {
return this.xid;
}
/**
* Name of the actual 3ds vendor who processed the 3ds request, e.g. Cardinal
*/
@java.lang.SuppressWarnings("all")
public String getMpiVendor() {
return this.mpiVendor;
}
/**
* Authorization status from 3ds provider. Should be echoed back in the response
* @return this
*/
@java.lang.SuppressWarnings("all")
public Card3dSecureInfo setAuthStatus(final String authStatus) {
this.authStatus = authStatus;
return this;
}
/**
* Numeric flag to indicate how the payment should be processed in relationship to 3d-secure. If 0 then ignore all 3d values and process as non-3ds
* @return this
*/
@java.lang.SuppressWarnings("all")
public Card3dSecureInfo setEci(final String eci) {
this.eci = eci;
return this;
}
/**
* Cardholder Authentication Verification Value (used by VISA).
* @return this
*/
@java.lang.SuppressWarnings("all")
public Card3dSecureInfo setCavv(final String cavv) {
this.cavv = cavv;
return this;
}
/**
* Transaction identifier from authenticator.
* @return this
*/
@java.lang.SuppressWarnings("all")
public Card3dSecureInfo setXid(final String xid) {
this.xid = xid;
return this;
}
/**
* Name of the actual 3ds vendor who processed the 3ds request, e.g. Cardinal
* @return this
*/
@java.lang.SuppressWarnings("all")
public Card3dSecureInfo setMpiVendor(final String mpiVendor) {
this.mpiVendor = mpiVendor;
return this;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Card3dSecureInfo)) return false;
final Card3dSecureInfo other = (Card3dSecureInfo) o;
if (!other.canEqual((java.lang.Object) this)) return false;
if (!super.equals(o)) return false;
final java.lang.Object this$authStatus = this.getAuthStatus();
final java.lang.Object other$authStatus = other.getAuthStatus();
if (this$authStatus == null ? other$authStatus != null : !this$authStatus.equals(other$authStatus)) return false;
final java.lang.Object this$eci = this.getEci();
final java.lang.Object other$eci = other.getEci();
if (this$eci == null ? other$eci != null : !this$eci.equals(other$eci)) return false;
final java.lang.Object this$cavv = this.getCavv();
final java.lang.Object other$cavv = other.getCavv();
if (this$cavv == null ? other$cavv != null : !this$cavv.equals(other$cavv)) return false;
final java.lang.Object this$xid = this.getXid();
final java.lang.Object other$xid = other.getXid();
if (this$xid == null ? other$xid != null : !this$xid.equals(other$xid)) return false;
final java.lang.Object this$mpiVendor = this.getMpiVendor();
final java.lang.Object other$mpiVendor = other.getMpiVendor();
if (this$mpiVendor == null ? other$mpiVendor != null : !this$mpiVendor.equals(other$mpiVendor)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Card3dSecureInfo;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public int hashCode() {
final int PRIME = 59;
int result = 1;
result = result * PRIME + super.hashCode();
final java.lang.Object $authStatus = this.getAuthStatus();
result = result * PRIME + ($authStatus == null ? 43 : $authStatus.hashCode());
final java.lang.Object $eci = this.getEci();
result = result * PRIME + ($eci == null ? 43 : $eci.hashCode());
final java.lang.Object $cavv = this.getCavv();
result = result * PRIME + ($cavv == null ? 43 : $cavv.hashCode());
final java.lang.Object $xid = this.getXid();
result = result * PRIME + ($xid == null ? 43 : $xid.hashCode());
final java.lang.Object $mpiVendor = this.getMpiVendor();
result = result * PRIME + ($mpiVendor == null ? 43 : $mpiVendor.hashCode());
return result;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy