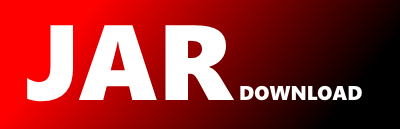
com.paypal.api.payments.CartBase Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rest-api-sdk Show documentation
Show all versions of rest-api-sdk Show documentation
PayPal SDK for integrating with the REST APIs
// Generated by delombok at Wed Oct 12 18:15:55 CDT 2016
package com.paypal.api.payments;
import com.paypal.base.rest.PayPalModel;
import java.util.List;
public class CartBase extends PayPalModel {
/**
* Merchant identifier to the purchase unit. Optional parameter
*/
private String referenceId;
/**
* Amount being collected.
*/
private Amount amount;
/**
* Recipient of the funds in this transaction.
*/
private Payee payee;
/**
* Description of transaction.
*/
private String description;
/**
* Note to the recipient of the funds in this transaction.
*/
private String noteToPayee;
/**
* Free-form field for the use of clients. Only supported when the `payment_method` is set to `paypal`.
*/
private String custom;
/**
* Invoice number used to track the payment. Only supported when the `payment_method` is set to `paypal`.
*/
private String invoiceNumber;
/**
* Soft descriptor used when charging this funding source. If length exceeds max length, the value will be truncated
*/
private String softDescriptor;
/**
* Soft descriptor city used when charging this funding source. If length exceeds max length, the value will be truncated. Only supported when the `payment_method` is set to `credit_card`
*/
private String softDescriptorCity;
/**
* Payment options requested for this purchase unit
*/
private PaymentOptions paymentOptions;
/**
* Items and related shipping address within a transaction.
*/
private ItemList itemList;
/**
* URL to send payment notifications
*/
private String notifyUrl;
/**
* Url on merchant site pertaining to this payment.
*/
private String orderUrl;
/**
* List of external funding being applied to the purchase unit. Each external_funding unit should have a unique reference_id
*/
private List externalFunding;
/**
* Default Constructor
*/
public CartBase() {
}
/**
* Parameterized Constructor
*/
public CartBase(Amount amount) {
this.amount = amount;
}
/**
* Merchant identifier to the purchase unit. Optional parameter
*/
@java.lang.SuppressWarnings("all")
public String getReferenceId() {
return this.referenceId;
}
/**
* Amount being collected.
*/
@java.lang.SuppressWarnings("all")
public Amount getAmount() {
return this.amount;
}
/**
* Recipient of the funds in this transaction.
*/
@java.lang.SuppressWarnings("all")
public Payee getPayee() {
return this.payee;
}
/**
* Description of transaction.
*/
@java.lang.SuppressWarnings("all")
public String getDescription() {
return this.description;
}
/**
* Note to the recipient of the funds in this transaction.
*/
@java.lang.SuppressWarnings("all")
public String getNoteToPayee() {
return this.noteToPayee;
}
/**
* Free-form field for the use of clients. Only supported when the `payment_method` is set to `paypal`.
*/
@java.lang.SuppressWarnings("all")
public String getCustom() {
return this.custom;
}
/**
* Invoice number used to track the payment. Only supported when the `payment_method` is set to `paypal`.
*/
@java.lang.SuppressWarnings("all")
public String getInvoiceNumber() {
return this.invoiceNumber;
}
/**
* Soft descriptor used when charging this funding source. If length exceeds max length, the value will be truncated
*/
@java.lang.SuppressWarnings("all")
public String getSoftDescriptor() {
return this.softDescriptor;
}
/**
* Soft descriptor city used when charging this funding source. If length exceeds max length, the value will be truncated. Only supported when the `payment_method` is set to `credit_card`
*/
@java.lang.SuppressWarnings("all")
public String getSoftDescriptorCity() {
return this.softDescriptorCity;
}
/**
* Payment options requested for this purchase unit
*/
@java.lang.SuppressWarnings("all")
public PaymentOptions getPaymentOptions() {
return this.paymentOptions;
}
/**
* Items and related shipping address within a transaction.
*/
@java.lang.SuppressWarnings("all")
public ItemList getItemList() {
return this.itemList;
}
/**
* URL to send payment notifications
*/
@java.lang.SuppressWarnings("all")
public String getNotifyUrl() {
return this.notifyUrl;
}
/**
* Url on merchant site pertaining to this payment.
*/
@java.lang.SuppressWarnings("all")
public String getOrderUrl() {
return this.orderUrl;
}
/**
* List of external funding being applied to the purchase unit. Each external_funding unit should have a unique reference_id
*/
@java.lang.SuppressWarnings("all")
public List getExternalFunding() {
return this.externalFunding;
}
/**
* Merchant identifier to the purchase unit. Optional parameter
* @return this
*/
@java.lang.SuppressWarnings("all")
public CartBase setReferenceId(final String referenceId) {
this.referenceId = referenceId;
return this;
}
/**
* Amount being collected.
* @return this
*/
@java.lang.SuppressWarnings("all")
public CartBase setAmount(final Amount amount) {
this.amount = amount;
return this;
}
/**
* Recipient of the funds in this transaction.
* @return this
*/
@java.lang.SuppressWarnings("all")
public CartBase setPayee(final Payee payee) {
this.payee = payee;
return this;
}
/**
* Description of transaction.
* @return this
*/
@java.lang.SuppressWarnings("all")
public CartBase setDescription(final String description) {
this.description = description;
return this;
}
/**
* Note to the recipient of the funds in this transaction.
* @return this
*/
@java.lang.SuppressWarnings("all")
public CartBase setNoteToPayee(final String noteToPayee) {
this.noteToPayee = noteToPayee;
return this;
}
/**
* Free-form field for the use of clients. Only supported when the `payment_method` is set to `paypal`.
* @return this
*/
@java.lang.SuppressWarnings("all")
public CartBase setCustom(final String custom) {
this.custom = custom;
return this;
}
/**
* Invoice number used to track the payment. Only supported when the `payment_method` is set to `paypal`.
* @return this
*/
@java.lang.SuppressWarnings("all")
public CartBase setInvoiceNumber(final String invoiceNumber) {
this.invoiceNumber = invoiceNumber;
return this;
}
/**
* Soft descriptor used when charging this funding source. If length exceeds max length, the value will be truncated
* @return this
*/
@java.lang.SuppressWarnings("all")
public CartBase setSoftDescriptor(final String softDescriptor) {
this.softDescriptor = softDescriptor;
return this;
}
/**
* Soft descriptor city used when charging this funding source. If length exceeds max length, the value will be truncated. Only supported when the `payment_method` is set to `credit_card`
* @return this
*/
@java.lang.SuppressWarnings("all")
public CartBase setSoftDescriptorCity(final String softDescriptorCity) {
this.softDescriptorCity = softDescriptorCity;
return this;
}
/**
* Payment options requested for this purchase unit
* @return this
*/
@java.lang.SuppressWarnings("all")
public CartBase setPaymentOptions(final PaymentOptions paymentOptions) {
this.paymentOptions = paymentOptions;
return this;
}
/**
* Items and related shipping address within a transaction.
* @return this
*/
@java.lang.SuppressWarnings("all")
public CartBase setItemList(final ItemList itemList) {
this.itemList = itemList;
return this;
}
/**
* URL to send payment notifications
* @return this
*/
@java.lang.SuppressWarnings("all")
public CartBase setNotifyUrl(final String notifyUrl) {
this.notifyUrl = notifyUrl;
return this;
}
/**
* Url on merchant site pertaining to this payment.
* @return this
*/
@java.lang.SuppressWarnings("all")
public CartBase setOrderUrl(final String orderUrl) {
this.orderUrl = orderUrl;
return this;
}
/**
* List of external funding being applied to the purchase unit. Each external_funding unit should have a unique reference_id
* @return this
*/
@java.lang.SuppressWarnings("all")
public CartBase setExternalFunding(final List externalFunding) {
this.externalFunding = externalFunding;
return this;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof CartBase)) return false;
final CartBase other = (CartBase) o;
if (!other.canEqual((java.lang.Object) this)) return false;
if (!super.equals(o)) return false;
final java.lang.Object this$referenceId = this.getReferenceId();
final java.lang.Object other$referenceId = other.getReferenceId();
if (this$referenceId == null ? other$referenceId != null : !this$referenceId.equals(other$referenceId)) return false;
final java.lang.Object this$amount = this.getAmount();
final java.lang.Object other$amount = other.getAmount();
if (this$amount == null ? other$amount != null : !this$amount.equals(other$amount)) return false;
final java.lang.Object this$payee = this.getPayee();
final java.lang.Object other$payee = other.getPayee();
if (this$payee == null ? other$payee != null : !this$payee.equals(other$payee)) return false;
final java.lang.Object this$description = this.getDescription();
final java.lang.Object other$description = other.getDescription();
if (this$description == null ? other$description != null : !this$description.equals(other$description)) return false;
final java.lang.Object this$noteToPayee = this.getNoteToPayee();
final java.lang.Object other$noteToPayee = other.getNoteToPayee();
if (this$noteToPayee == null ? other$noteToPayee != null : !this$noteToPayee.equals(other$noteToPayee)) return false;
final java.lang.Object this$custom = this.getCustom();
final java.lang.Object other$custom = other.getCustom();
if (this$custom == null ? other$custom != null : !this$custom.equals(other$custom)) return false;
final java.lang.Object this$invoiceNumber = this.getInvoiceNumber();
final java.lang.Object other$invoiceNumber = other.getInvoiceNumber();
if (this$invoiceNumber == null ? other$invoiceNumber != null : !this$invoiceNumber.equals(other$invoiceNumber)) return false;
final java.lang.Object this$softDescriptor = this.getSoftDescriptor();
final java.lang.Object other$softDescriptor = other.getSoftDescriptor();
if (this$softDescriptor == null ? other$softDescriptor != null : !this$softDescriptor.equals(other$softDescriptor)) return false;
final java.lang.Object this$softDescriptorCity = this.getSoftDescriptorCity();
final java.lang.Object other$softDescriptorCity = other.getSoftDescriptorCity();
if (this$softDescriptorCity == null ? other$softDescriptorCity != null : !this$softDescriptorCity.equals(other$softDescriptorCity)) return false;
final java.lang.Object this$paymentOptions = this.getPaymentOptions();
final java.lang.Object other$paymentOptions = other.getPaymentOptions();
if (this$paymentOptions == null ? other$paymentOptions != null : !this$paymentOptions.equals(other$paymentOptions)) return false;
final java.lang.Object this$itemList = this.getItemList();
final java.lang.Object other$itemList = other.getItemList();
if (this$itemList == null ? other$itemList != null : !this$itemList.equals(other$itemList)) return false;
final java.lang.Object this$notifyUrl = this.getNotifyUrl();
final java.lang.Object other$notifyUrl = other.getNotifyUrl();
if (this$notifyUrl == null ? other$notifyUrl != null : !this$notifyUrl.equals(other$notifyUrl)) return false;
final java.lang.Object this$orderUrl = this.getOrderUrl();
final java.lang.Object other$orderUrl = other.getOrderUrl();
if (this$orderUrl == null ? other$orderUrl != null : !this$orderUrl.equals(other$orderUrl)) return false;
final java.lang.Object this$externalFunding = this.getExternalFunding();
final java.lang.Object other$externalFunding = other.getExternalFunding();
if (this$externalFunding == null ? other$externalFunding != null : !this$externalFunding.equals(other$externalFunding)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
protected boolean canEqual(final java.lang.Object other) {
return other instanceof CartBase;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public int hashCode() {
final int PRIME = 59;
int result = 1;
result = result * PRIME + super.hashCode();
final java.lang.Object $referenceId = this.getReferenceId();
result = result * PRIME + ($referenceId == null ? 43 : $referenceId.hashCode());
final java.lang.Object $amount = this.getAmount();
result = result * PRIME + ($amount == null ? 43 : $amount.hashCode());
final java.lang.Object $payee = this.getPayee();
result = result * PRIME + ($payee == null ? 43 : $payee.hashCode());
final java.lang.Object $description = this.getDescription();
result = result * PRIME + ($description == null ? 43 : $description.hashCode());
final java.lang.Object $noteToPayee = this.getNoteToPayee();
result = result * PRIME + ($noteToPayee == null ? 43 : $noteToPayee.hashCode());
final java.lang.Object $custom = this.getCustom();
result = result * PRIME + ($custom == null ? 43 : $custom.hashCode());
final java.lang.Object $invoiceNumber = this.getInvoiceNumber();
result = result * PRIME + ($invoiceNumber == null ? 43 : $invoiceNumber.hashCode());
final java.lang.Object $softDescriptor = this.getSoftDescriptor();
result = result * PRIME + ($softDescriptor == null ? 43 : $softDescriptor.hashCode());
final java.lang.Object $softDescriptorCity = this.getSoftDescriptorCity();
result = result * PRIME + ($softDescriptorCity == null ? 43 : $softDescriptorCity.hashCode());
final java.lang.Object $paymentOptions = this.getPaymentOptions();
result = result * PRIME + ($paymentOptions == null ? 43 : $paymentOptions.hashCode());
final java.lang.Object $itemList = this.getItemList();
result = result * PRIME + ($itemList == null ? 43 : $itemList.hashCode());
final java.lang.Object $notifyUrl = this.getNotifyUrl();
result = result * PRIME + ($notifyUrl == null ? 43 : $notifyUrl.hashCode());
final java.lang.Object $orderUrl = this.getOrderUrl();
result = result * PRIME + ($orderUrl == null ? 43 : $orderUrl.hashCode());
final java.lang.Object $externalFunding = this.getExternalFunding();
result = result * PRIME + ($externalFunding == null ? 43 : $externalFunding.hashCode());
return result;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy