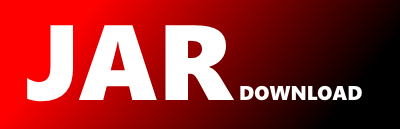
com.paypal.api.payments.ErrorDetails Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rest-api-sdk Show documentation
Show all versions of rest-api-sdk Show documentation
PayPal SDK for integrating with the REST APIs
// Generated by delombok at Wed Oct 12 18:15:55 CDT 2016
package com.paypal.api.payments;
import com.paypal.base.rest.PayPalModel;
public class ErrorDetails extends PayPalModel {
/**
* Name of the field that caused the error.
*/
private String field;
/**
* Reason for the error.
*/
private String issue;
/**
* Reference ID of the purchase_unit associated with this error
*/
private String purchaseUnitReferenceId;
/**
* PayPal internal error code.
*/
private String code;
/**
* Default Constructor
*/
public ErrorDetails() {
}
/**
* Parameterized Constructor
*/
public ErrorDetails(String field, String issue) {
this.field = field;
this.issue = issue;
}
/**
* Name of the field that caused the error.
*/
@java.lang.SuppressWarnings("all")
public String getField() {
return this.field;
}
/**
* Reason for the error.
*/
@java.lang.SuppressWarnings("all")
public String getIssue() {
return this.issue;
}
/**
* Reference ID of the purchase_unit associated with this error
*/
@java.lang.SuppressWarnings("all")
public String getPurchaseUnitReferenceId() {
return this.purchaseUnitReferenceId;
}
/**
* PayPal internal error code.
*/
@java.lang.SuppressWarnings("all")
public String getCode() {
return this.code;
}
/**
* Name of the field that caused the error.
* @return this
*/
@java.lang.SuppressWarnings("all")
public ErrorDetails setField(final String field) {
this.field = field;
return this;
}
/**
* Reason for the error.
* @return this
*/
@java.lang.SuppressWarnings("all")
public ErrorDetails setIssue(final String issue) {
this.issue = issue;
return this;
}
/**
* Reference ID of the purchase_unit associated with this error
* @return this
*/
@java.lang.SuppressWarnings("all")
public ErrorDetails setPurchaseUnitReferenceId(final String purchaseUnitReferenceId) {
this.purchaseUnitReferenceId = purchaseUnitReferenceId;
return this;
}
/**
* PayPal internal error code.
* @return this
*/
@java.lang.SuppressWarnings("all")
public ErrorDetails setCode(final String code) {
this.code = code;
return this;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof ErrorDetails)) return false;
final ErrorDetails other = (ErrorDetails) o;
if (!other.canEqual((java.lang.Object) this)) return false;
if (!super.equals(o)) return false;
final java.lang.Object this$field = this.getField();
final java.lang.Object other$field = other.getField();
if (this$field == null ? other$field != null : !this$field.equals(other$field)) return false;
final java.lang.Object this$issue = this.getIssue();
final java.lang.Object other$issue = other.getIssue();
if (this$issue == null ? other$issue != null : !this$issue.equals(other$issue)) return false;
final java.lang.Object this$purchaseUnitReferenceId = this.getPurchaseUnitReferenceId();
final java.lang.Object other$purchaseUnitReferenceId = other.getPurchaseUnitReferenceId();
if (this$purchaseUnitReferenceId == null ? other$purchaseUnitReferenceId != null : !this$purchaseUnitReferenceId.equals(other$purchaseUnitReferenceId)) return false;
final java.lang.Object this$code = this.getCode();
final java.lang.Object other$code = other.getCode();
if (this$code == null ? other$code != null : !this$code.equals(other$code)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
protected boolean canEqual(final java.lang.Object other) {
return other instanceof ErrorDetails;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public int hashCode() {
final int PRIME = 59;
int result = 1;
result = result * PRIME + super.hashCode();
final java.lang.Object $field = this.getField();
result = result * PRIME + ($field == null ? 43 : $field.hashCode());
final java.lang.Object $issue = this.getIssue();
result = result * PRIME + ($issue == null ? 43 : $issue.hashCode());
final java.lang.Object $purchaseUnitReferenceId = this.getPurchaseUnitReferenceId();
result = result * PRIME + ($purchaseUnitReferenceId == null ? 43 : $purchaseUnitReferenceId.hashCode());
final java.lang.Object $code = this.getCode();
result = result * PRIME + ($code == null ? 43 : $code.hashCode());
return result;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy