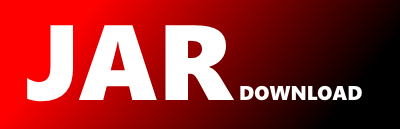
com.paypal.api.payments.FundingInstrument Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rest-api-sdk Show documentation
Show all versions of rest-api-sdk Show documentation
PayPal SDK for integrating with the REST APIs
// Generated by delombok at Wed Oct 12 18:15:55 CDT 2016
package com.paypal.api.payments;
import com.paypal.base.rest.PayPalModel;
public class FundingInstrument extends PayPalModel {
/**
* Credit Card instrument.
*/
private CreditCard creditCard;
/**
* PayPal vaulted credit Card instrument.
*/
private CreditCardToken creditCardToken;
/**
* Payment Card information.
*/
private PaymentCard paymentCard;
/**
* Bank Account information.
*/
private ExtendedBankAccount bankAccount;
/**
* Vaulted bank account instrument.
*/
private BankToken bankAccountToken;
/**
* PayPal credit funding instrument.
*/
private Credit credit;
/**
* Incentive funding instrument.
*/
private Incentive incentive;
/**
* External funding instrument.
*/
private ExternalFunding externalFunding;
/**
* Carrier account token instrument.
*/
private CarrierAccountToken carrierAccountToken;
/**
* Carrier account instrument
*/
private CarrierAccount carrierAccount;
/**
* Private Label Card funding instrument. These are store cards provided by merchants to drive business with value to customer with convenience and rewards.
*/
private PrivateLabelCard privateLabelCard;
/**
* Billing instrument that references pre-approval information for the payment
*/
private Billing billing;
/**
* Alternate Payment information - Mostly regional payment providers. For e.g iDEAL in Netherlands
*/
private AlternatePayment alternatePayment;
/**
* Default Constructor
*/
public FundingInstrument() {
}
/**
* Credit Card instrument.
*/
@java.lang.SuppressWarnings("all")
public CreditCard getCreditCard() {
return this.creditCard;
}
/**
* PayPal vaulted credit Card instrument.
*/
@java.lang.SuppressWarnings("all")
public CreditCardToken getCreditCardToken() {
return this.creditCardToken;
}
/**
* Payment Card information.
*/
@java.lang.SuppressWarnings("all")
public PaymentCard getPaymentCard() {
return this.paymentCard;
}
/**
* Bank Account information.
*/
@java.lang.SuppressWarnings("all")
public ExtendedBankAccount getBankAccount() {
return this.bankAccount;
}
/**
* Vaulted bank account instrument.
*/
@java.lang.SuppressWarnings("all")
public BankToken getBankAccountToken() {
return this.bankAccountToken;
}
/**
* PayPal credit funding instrument.
*/
@java.lang.SuppressWarnings("all")
public Credit getCredit() {
return this.credit;
}
/**
* Incentive funding instrument.
*/
@java.lang.SuppressWarnings("all")
public Incentive getIncentive() {
return this.incentive;
}
/**
* External funding instrument.
*/
@java.lang.SuppressWarnings("all")
public ExternalFunding getExternalFunding() {
return this.externalFunding;
}
/**
* Carrier account token instrument.
*/
@java.lang.SuppressWarnings("all")
public CarrierAccountToken getCarrierAccountToken() {
return this.carrierAccountToken;
}
/**
* Carrier account instrument
*/
@java.lang.SuppressWarnings("all")
public CarrierAccount getCarrierAccount() {
return this.carrierAccount;
}
/**
* Private Label Card funding instrument. These are store cards provided by merchants to drive business with value to customer with convenience and rewards.
*/
@java.lang.SuppressWarnings("all")
public PrivateLabelCard getPrivateLabelCard() {
return this.privateLabelCard;
}
/**
* Billing instrument that references pre-approval information for the payment
*/
@java.lang.SuppressWarnings("all")
public Billing getBilling() {
return this.billing;
}
/**
* Alternate Payment information - Mostly regional payment providers. For e.g iDEAL in Netherlands
*/
@java.lang.SuppressWarnings("all")
public AlternatePayment getAlternatePayment() {
return this.alternatePayment;
}
/**
* Credit Card instrument.
* @return this
*/
@java.lang.SuppressWarnings("all")
public FundingInstrument setCreditCard(final CreditCard creditCard) {
this.creditCard = creditCard;
return this;
}
/**
* PayPal vaulted credit Card instrument.
* @return this
*/
@java.lang.SuppressWarnings("all")
public FundingInstrument setCreditCardToken(final CreditCardToken creditCardToken) {
this.creditCardToken = creditCardToken;
return this;
}
/**
* Payment Card information.
* @return this
*/
@java.lang.SuppressWarnings("all")
public FundingInstrument setPaymentCard(final PaymentCard paymentCard) {
this.paymentCard = paymentCard;
return this;
}
/**
* Bank Account information.
* @return this
*/
@java.lang.SuppressWarnings("all")
public FundingInstrument setBankAccount(final ExtendedBankAccount bankAccount) {
this.bankAccount = bankAccount;
return this;
}
/**
* Vaulted bank account instrument.
* @return this
*/
@java.lang.SuppressWarnings("all")
public FundingInstrument setBankAccountToken(final BankToken bankAccountToken) {
this.bankAccountToken = bankAccountToken;
return this;
}
/**
* PayPal credit funding instrument.
* @return this
*/
@java.lang.SuppressWarnings("all")
public FundingInstrument setCredit(final Credit credit) {
this.credit = credit;
return this;
}
/**
* Incentive funding instrument.
* @return this
*/
@java.lang.SuppressWarnings("all")
public FundingInstrument setIncentive(final Incentive incentive) {
this.incentive = incentive;
return this;
}
/**
* External funding instrument.
* @return this
*/
@java.lang.SuppressWarnings("all")
public FundingInstrument setExternalFunding(final ExternalFunding externalFunding) {
this.externalFunding = externalFunding;
return this;
}
/**
* Carrier account token instrument.
* @return this
*/
@java.lang.SuppressWarnings("all")
public FundingInstrument setCarrierAccountToken(final CarrierAccountToken carrierAccountToken) {
this.carrierAccountToken = carrierAccountToken;
return this;
}
/**
* Carrier account instrument
* @return this
*/
@java.lang.SuppressWarnings("all")
public FundingInstrument setCarrierAccount(final CarrierAccount carrierAccount) {
this.carrierAccount = carrierAccount;
return this;
}
/**
* Private Label Card funding instrument. These are store cards provided by merchants to drive business with value to customer with convenience and rewards.
* @return this
*/
@java.lang.SuppressWarnings("all")
public FundingInstrument setPrivateLabelCard(final PrivateLabelCard privateLabelCard) {
this.privateLabelCard = privateLabelCard;
return this;
}
/**
* Billing instrument that references pre-approval information for the payment
* @return this
*/
@java.lang.SuppressWarnings("all")
public FundingInstrument setBilling(final Billing billing) {
this.billing = billing;
return this;
}
/**
* Alternate Payment information - Mostly regional payment providers. For e.g iDEAL in Netherlands
* @return this
*/
@java.lang.SuppressWarnings("all")
public FundingInstrument setAlternatePayment(final AlternatePayment alternatePayment) {
this.alternatePayment = alternatePayment;
return this;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof FundingInstrument)) return false;
final FundingInstrument other = (FundingInstrument) o;
if (!other.canEqual((java.lang.Object) this)) return false;
if (!super.equals(o)) return false;
final java.lang.Object this$creditCard = this.getCreditCard();
final java.lang.Object other$creditCard = other.getCreditCard();
if (this$creditCard == null ? other$creditCard != null : !this$creditCard.equals(other$creditCard)) return false;
final java.lang.Object this$creditCardToken = this.getCreditCardToken();
final java.lang.Object other$creditCardToken = other.getCreditCardToken();
if (this$creditCardToken == null ? other$creditCardToken != null : !this$creditCardToken.equals(other$creditCardToken)) return false;
final java.lang.Object this$paymentCard = this.getPaymentCard();
final java.lang.Object other$paymentCard = other.getPaymentCard();
if (this$paymentCard == null ? other$paymentCard != null : !this$paymentCard.equals(other$paymentCard)) return false;
final java.lang.Object this$bankAccount = this.getBankAccount();
final java.lang.Object other$bankAccount = other.getBankAccount();
if (this$bankAccount == null ? other$bankAccount != null : !this$bankAccount.equals(other$bankAccount)) return false;
final java.lang.Object this$bankAccountToken = this.getBankAccountToken();
final java.lang.Object other$bankAccountToken = other.getBankAccountToken();
if (this$bankAccountToken == null ? other$bankAccountToken != null : !this$bankAccountToken.equals(other$bankAccountToken)) return false;
final java.lang.Object this$credit = this.getCredit();
final java.lang.Object other$credit = other.getCredit();
if (this$credit == null ? other$credit != null : !this$credit.equals(other$credit)) return false;
final java.lang.Object this$incentive = this.getIncentive();
final java.lang.Object other$incentive = other.getIncentive();
if (this$incentive == null ? other$incentive != null : !this$incentive.equals(other$incentive)) return false;
final java.lang.Object this$externalFunding = this.getExternalFunding();
final java.lang.Object other$externalFunding = other.getExternalFunding();
if (this$externalFunding == null ? other$externalFunding != null : !this$externalFunding.equals(other$externalFunding)) return false;
final java.lang.Object this$carrierAccountToken = this.getCarrierAccountToken();
final java.lang.Object other$carrierAccountToken = other.getCarrierAccountToken();
if (this$carrierAccountToken == null ? other$carrierAccountToken != null : !this$carrierAccountToken.equals(other$carrierAccountToken)) return false;
final java.lang.Object this$carrierAccount = this.getCarrierAccount();
final java.lang.Object other$carrierAccount = other.getCarrierAccount();
if (this$carrierAccount == null ? other$carrierAccount != null : !this$carrierAccount.equals(other$carrierAccount)) return false;
final java.lang.Object this$privateLabelCard = this.getPrivateLabelCard();
final java.lang.Object other$privateLabelCard = other.getPrivateLabelCard();
if (this$privateLabelCard == null ? other$privateLabelCard != null : !this$privateLabelCard.equals(other$privateLabelCard)) return false;
final java.lang.Object this$billing = this.getBilling();
final java.lang.Object other$billing = other.getBilling();
if (this$billing == null ? other$billing != null : !this$billing.equals(other$billing)) return false;
final java.lang.Object this$alternatePayment = this.getAlternatePayment();
final java.lang.Object other$alternatePayment = other.getAlternatePayment();
if (this$alternatePayment == null ? other$alternatePayment != null : !this$alternatePayment.equals(other$alternatePayment)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
protected boolean canEqual(final java.lang.Object other) {
return other instanceof FundingInstrument;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public int hashCode() {
final int PRIME = 59;
int result = 1;
result = result * PRIME + super.hashCode();
final java.lang.Object $creditCard = this.getCreditCard();
result = result * PRIME + ($creditCard == null ? 43 : $creditCard.hashCode());
final java.lang.Object $creditCardToken = this.getCreditCardToken();
result = result * PRIME + ($creditCardToken == null ? 43 : $creditCardToken.hashCode());
final java.lang.Object $paymentCard = this.getPaymentCard();
result = result * PRIME + ($paymentCard == null ? 43 : $paymentCard.hashCode());
final java.lang.Object $bankAccount = this.getBankAccount();
result = result * PRIME + ($bankAccount == null ? 43 : $bankAccount.hashCode());
final java.lang.Object $bankAccountToken = this.getBankAccountToken();
result = result * PRIME + ($bankAccountToken == null ? 43 : $bankAccountToken.hashCode());
final java.lang.Object $credit = this.getCredit();
result = result * PRIME + ($credit == null ? 43 : $credit.hashCode());
final java.lang.Object $incentive = this.getIncentive();
result = result * PRIME + ($incentive == null ? 43 : $incentive.hashCode());
final java.lang.Object $externalFunding = this.getExternalFunding();
result = result * PRIME + ($externalFunding == null ? 43 : $externalFunding.hashCode());
final java.lang.Object $carrierAccountToken = this.getCarrierAccountToken();
result = result * PRIME + ($carrierAccountToken == null ? 43 : $carrierAccountToken.hashCode());
final java.lang.Object $carrierAccount = this.getCarrierAccount();
result = result * PRIME + ($carrierAccount == null ? 43 : $carrierAccount.hashCode());
final java.lang.Object $privateLabelCard = this.getPrivateLabelCard();
result = result * PRIME + ($privateLabelCard == null ? 43 : $privateLabelCard.hashCode());
final java.lang.Object $billing = this.getBilling();
result = result * PRIME + ($billing == null ? 43 : $billing.hashCode());
final java.lang.Object $alternatePayment = this.getAlternatePayment();
result = result * PRIME + ($alternatePayment == null ? 43 : $alternatePayment.hashCode());
return result;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy