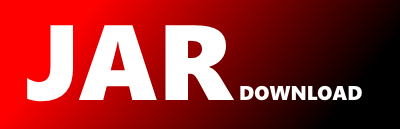
com.paypal.api.payments.InputFields Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rest-api-sdk Show documentation
Show all versions of rest-api-sdk Show documentation
PayPal SDK for integrating with the REST APIs
// Generated by delombok at Wed Oct 12 18:15:55 CDT 2016
package com.paypal.api.payments;
import com.paypal.base.rest.PayPalModel;
public class InputFields extends PayPalModel {
/**
* Enables the buyer to enter a note to the merchant on the PayPal page during checkout.
*/
private Boolean allowNote;
/**
* Determines whether or not PayPal displays shipping address fields on the experience pages. Allowed values: `0`, `1`, or `2`. When set to `0`, PayPal displays the shipping address on the PayPal pages. When set to `1`, PayPal does not display shipping address fields whatsoever. When set to `2`, if you do not pass the shipping address, PayPal obtains it from the buyer's account profile. For digital goods, this field is required, and you must set it to `1`.
*/
private int noShipping;
/**
* Determines if the PayPal pages should display the shipping address supplied in this call, rather than the shipping address on file with PayPal for this buyer. Displaying the address on file does not allow the buyer to edit the address. Allowed values: `0` or `1`. When set to `0`, the PayPal pages should display the address on file. When set to `1`, the PayPal pages should display the addresses supplied in this call instead of the address from the buyer's PayPal account.
*/
private int addressOverride;
/**
* Default Constructor
*/
public InputFields() {
}
/**
* Enables the buyer to enter a note to the merchant on the PayPal page during checkout.
*/
@java.lang.SuppressWarnings("all")
public Boolean getAllowNote() {
return this.allowNote;
}
/**
* Determines whether or not PayPal displays shipping address fields on the experience pages. Allowed values: `0`, `1`, or `2`. When set to `0`, PayPal displays the shipping address on the PayPal pages. When set to `1`, PayPal does not display shipping address fields whatsoever. When set to `2`, if you do not pass the shipping address, PayPal obtains it from the buyer's account profile. For digital goods, this field is required, and you must set it to `1`.
*/
@java.lang.SuppressWarnings("all")
public int getNoShipping() {
return this.noShipping;
}
/**
* Determines if the PayPal pages should display the shipping address supplied in this call, rather than the shipping address on file with PayPal for this buyer. Displaying the address on file does not allow the buyer to edit the address. Allowed values: `0` or `1`. When set to `0`, the PayPal pages should display the address on file. When set to `1`, the PayPal pages should display the addresses supplied in this call instead of the address from the buyer's PayPal account.
*/
@java.lang.SuppressWarnings("all")
public int getAddressOverride() {
return this.addressOverride;
}
/**
* Enables the buyer to enter a note to the merchant on the PayPal page during checkout.
* @return this
*/
@java.lang.SuppressWarnings("all")
public InputFields setAllowNote(final Boolean allowNote) {
this.allowNote = allowNote;
return this;
}
/**
* Determines whether or not PayPal displays shipping address fields on the experience pages. Allowed values: `0`, `1`, or `2`. When set to `0`, PayPal displays the shipping address on the PayPal pages. When set to `1`, PayPal does not display shipping address fields whatsoever. When set to `2`, if you do not pass the shipping address, PayPal obtains it from the buyer's account profile. For digital goods, this field is required, and you must set it to `1`.
* @return this
*/
@java.lang.SuppressWarnings("all")
public InputFields setNoShipping(final int noShipping) {
this.noShipping = noShipping;
return this;
}
/**
* Determines if the PayPal pages should display the shipping address supplied in this call, rather than the shipping address on file with PayPal for this buyer. Displaying the address on file does not allow the buyer to edit the address. Allowed values: `0` or `1`. When set to `0`, the PayPal pages should display the address on file. When set to `1`, the PayPal pages should display the addresses supplied in this call instead of the address from the buyer's PayPal account.
* @return this
*/
@java.lang.SuppressWarnings("all")
public InputFields setAddressOverride(final int addressOverride) {
this.addressOverride = addressOverride;
return this;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof InputFields)) return false;
final InputFields other = (InputFields) o;
if (!other.canEqual((java.lang.Object) this)) return false;
if (!super.equals(o)) return false;
final java.lang.Object this$allowNote = this.getAllowNote();
final java.lang.Object other$allowNote = other.getAllowNote();
if (this$allowNote == null ? other$allowNote != null : !this$allowNote.equals(other$allowNote)) return false;
if (this.getNoShipping() != other.getNoShipping()) return false;
if (this.getAddressOverride() != other.getAddressOverride()) return false;
return true;
}
@java.lang.SuppressWarnings("all")
protected boolean canEqual(final java.lang.Object other) {
return other instanceof InputFields;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public int hashCode() {
final int PRIME = 59;
int result = 1;
result = result * PRIME + super.hashCode();
final java.lang.Object $allowNote = this.getAllowNote();
result = result * PRIME + ($allowNote == null ? 43 : $allowNote.hashCode());
result = result * PRIME + this.getNoShipping();
result = result * PRIME + this.getAddressOverride();
return result;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy