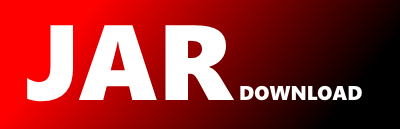
com.paypal.api.payments.Invoice Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rest-api-sdk Show documentation
Show all versions of rest-api-sdk Show documentation
PayPal SDK for integrating with the REST APIs
// Generated by delombok at Wed Oct 12 18:15:55 CDT 2016
package com.paypal.api.payments;
import com.paypal.api.openidconnect.Tokeninfo;
import com.paypal.base.rest.*;
import java.util.List;
import java.util.Map;
public class Invoice extends PayPalResource {
/**
* The unique invoice resource identifier.
*/
private String id;
/**
* Unique number that appears on the invoice. If left blank will be auto-incremented from the last number. 25 characters max.
*/
private String number;
/**
* The template ID used for the invoice. Useful for copy functionality.
*/
private String templateId;
/**
* URI of the invoice resource.
*/
private String uri;
/**
* Status of the invoice.
*/
private String status;
/**
* Information about the merchant who is sending the invoice.
*/
private MerchantInfo merchantInfo;
/**
* The required invoice recipient email address and any optional billing information. One recipient is supported.
*/
private List billingInfo;
/**
* For invoices sent by email, one or more email addresses to which to send a Cc: copy of the notification. Supports only email addresses under participant.
*/
private List ccInfo;
/**
* The shipping information for entities to whom items are being shipped.
*/
private ShippingInfo shippingInfo;
/**
* The list of items to include in the invoice. Maximum value is 100 items per invoice.
*/
private List items;
/**
* The date when the invoice was enabled. The date format is *yyyy*-*MM*-*dd* *z* as defined in [Internet Date/Time Format](http://tools.ietf.org/html/rfc3339#section-5.6).
*/
private String invoiceDate;
/**
* Optional. The payment deadline for the invoice. Value is either `term_type` or `due_date` but not both.
*/
private PaymentTerm paymentTerm;
/**
* Reference data, such as PO number, to add to the invoice. Maximum length is 60 characters.
*/
private String reference;
/**
* The invoice level discount, as a percent or an amount value.
*/
private Cost discount;
/**
* The shipping cost, as a percent or an amount value.
*/
private ShippingCost shippingCost;
/**
* The custom amount to apply on an invoice. If you include a label, the amount cannot be empty.
*/
private CustomAmount custom;
/**
* Indicates whether the invoice allows a partial payment. If set to `false`, invoice must be paid in full. If set to `true`, the invoice allows partial payments. Default is `false`.
*/
private Boolean allowPartialPayment;
/**
* If `allow_partial_payment` is set to `true`, the minimum amount allowed for a partial payment.
*/
private Currency minimumAmountDue;
/**
* Indicates whether tax is calculated before or after a discount. If set to `false`, the tax is calculated before a discount. If set to `true`, the tax is calculated after a discount. Default is `false`.
*/
private Boolean taxCalculatedAfterDiscount;
/**
* Indicates whether the unit price includes tax. Default is `false`.
*/
private Boolean taxInclusive;
/**
* General terms of the invoice. 4000 characters max.
*/
private String terms;
/**
* Note to the payer. 4000 characters max.
*/
private String note;
/**
* A private bookkeeping memo for the merchant. Maximum length is 150 characters.
*/
private String merchantMemo;
/**
* Full URL of an external image to use as the logo. Maximum length is 4000 characters.
*/
private String logoUrl;
/**
* The total amount of the invoice.
*/
private Currency totalAmount;
/**
* List of payment details for the invoice.
*/
private List payments;
/**
* List of refund details for the invoice.
*/
private List refunds;
/**
* Audit information for the invoice.
*/
private Metadata metadata;
/**
* Any miscellaneous invoice data. Maximum length is 4000 characters.
*/
private String additionalData;
/**
* Gratuity to include with the invoice.
*/
private Currency gratuity;
/**
* Payment summary of the invoice including amount paid through PayPal and other sources.
*/
private PaymentSummary paidAmount;
/**
* Payment summary of the invoice including amount refunded through PayPal and other sources.
*/
private PaymentSummary refundedAmount;
/**
* List of files attached to the invoice.
*/
private List attachments;
/**
* HATEOS links representing all the actions over the invoice resource based on the current invoice status.
*/
private List links;
/**
* Default Constructor
*/
public Invoice() {
}
/**
* Parameterized Constructor
*/
public Invoice(MerchantInfo merchantInfo) {
this.merchantInfo = merchantInfo;
}
/**
* @deprecated Please use {@link #getPayments()} instead.
* @return {@link List} of {@link PaymentDetail}
*/
public List getPaymentDetails() {
return this.payments;
}
/**
* @deprecated Please use {@link #setPayments(List)} instead.
* @param details
* @return {@link Invoice}
*/
public Invoice setPaymentDetails(List details) {
this.payments = details;
return this;
}
/**
* @deprecated Please use {@link #getRefunds()} instead.
* @return {@link List} of {@link RefundDetail}
*/
public List getRefundDetails() {
return this.refunds;
}
/**
* @deprecated Please use {@link #setRefunds(List)} instead.
* @param details
* @return {@link Invoice}
*/
public Invoice setRefundDetails(List details) {
this.refunds = details;
return this;
}
/**
* Creates an invoice. Include invoice details including merchant information in the request.
* @deprecated Please use {@link #create(APIContext)} instead.
* @param accessToken
* Access Token used for the API call.
* @return Invoice
* @throws PayPalRESTException
*/
public Invoice create(String accessToken) throws PayPalRESTException {
APIContext apiContext = new APIContext(accessToken);
return create(apiContext);
}
/**
* Creates an invoice. Include invoice details including merchant information in the request.
* @param apiContext
* {@link APIContext} used for the API call.
* @return Invoice
* @throws PayPalRESTException
*/
public Invoice create(APIContext apiContext) throws PayPalRESTException {
String resourcePath = "v1/invoicing/invoices";
String payLoad = this.toJSON();
return configureAndExecute(apiContext, HttpMethod.POST, resourcePath, payLoad, Invoice.class);
}
/**
* Searches for an invoice or invoices. Include a search object that specifies your search criteria in the request.
* @deprecated Please use {@link #search(APIContext, Search)} instead.
* @param accessToken
* Access Token used for the API call.
* @param search
* Search
* @return Invoices
* @throws PayPalRESTException
*/
public Invoices search(String accessToken, Search search) throws PayPalRESTException {
APIContext apiContext = new APIContext(accessToken);
return search(apiContext, search);
}
/**
* Searches for an invoice or invoices. Include a search object that specifies your search criteria in the request.
* @param apiContext
* {@link APIContext} used for the API call.
* @param search
* Search
* @return Invoices
* @throws PayPalRESTException
*/
public Invoices search(APIContext apiContext, Search search) throws PayPalRESTException {
if (search == null) {
throw new IllegalArgumentException("search cannot be null");
}
Object[] parameters = new Object[] {this.getId()};
String pattern = "v1/invoicing/search";
String resourcePath = RESTUtil.formatURIPath(pattern, parameters);
String payLoad = search.toJSON();
return configureAndExecute(apiContext, HttpMethod.POST, resourcePath, payLoad, Invoices.class);
}
/**
* Sends an invoice, by ID, to a recipient. Optionally, set the `notify_merchant` query parameter to send the merchant an invoice update notification. By default, `notify_merchant` is `true`.
* @deprecated Please use {@link #send(APIContext)} instead.
* @param accessToken
* Access Token used for the API call.
* @throws PayPalRESTException
*/
public void send(String accessToken) throws PayPalRESTException {
APIContext apiContext = new APIContext(accessToken);
send(apiContext);
}
/**
* Sends an invoice, by ID, to a recipient. Optionally, set the `notify_merchant` query parameter to send the merchant an invoice update notification. By default, `notify_merchant` is `true`.
* @param apiContext
* {@link APIContext} used for the API call.
* @throws PayPalRESTException
*/
public void send(APIContext apiContext) throws PayPalRESTException {
if (this.getId() == null) {
throw new IllegalArgumentException("Id cannot be null");
}
Object[] parameters = new Object[] {this.getId()};
String pattern = "v1/invoicing/invoices/{0}/send";
String resourcePath = RESTUtil.formatURIPath(pattern, parameters);
String payLoad = "";
configureAndExecute(apiContext, HttpMethod.POST, resourcePath, payLoad, null);
}
/**
* Sends a reminder about a specific invoice, by ID, to a recipient. Include a notification object that defines the reminder subject and other details in the JSON request body.
* @deprecated Please use {@link #remind(APIContext, Notification)} instead.
* @param accessToken
* Access Token used for the API call.
* @param notification
* Notification
* @throws PayPalRESTException
*/
public void remind(String accessToken, Notification notification) throws PayPalRESTException {
APIContext apiContext = new APIContext(accessToken);
remind(apiContext, notification);
}
/**
* Sends a reminder about a specific invoice, by ID, to a recipient. Include a notification object that defines the reminder subject and other details in the JSON request body.
* @param apiContext
* {@link APIContext} used for the API call.
* @param notification
* Notification
* @throws PayPalRESTException
*/
public void remind(APIContext apiContext, Notification notification) throws PayPalRESTException {
if (this.getId() == null) {
throw new IllegalArgumentException("Id cannot be null");
}
if (notification == null) {
throw new IllegalArgumentException("notification cannot be null");
}
Object[] parameters = new Object[] {this.getId()};
String pattern = "v1/invoicing/invoices/{0}/remind";
String resourcePath = RESTUtil.formatURIPath(pattern, parameters);
String payLoad = notification.toJSON();
configureAndExecute(apiContext, HttpMethod.POST, resourcePath, payLoad, null);
}
/**
* Cancels an invoice, by ID.
* @deprecated Please use {@link #cancel(APIContext, CancelNotification)} instead.
* @param accessToken
* Access Token used for the API call.
* @param cancelNotification
* CancelNotification
* @throws PayPalRESTException
*/
public void cancel(String accessToken, CancelNotification cancelNotification) throws PayPalRESTException {
APIContext apiContext = new APIContext(accessToken);
cancel(apiContext, cancelNotification);
}
/**
* Cancels an invoice, by ID.
* @param apiContext
* {@link APIContext} used for the API call.
* @param cancelNotification
* CancelNotification
* @throws PayPalRESTException
*/
public void cancel(APIContext apiContext, CancelNotification cancelNotification) throws PayPalRESTException {
if (this.getId() == null) {
throw new IllegalArgumentException("Id cannot be null");
}
if (cancelNotification == null) {
throw new IllegalArgumentException("cancelNotification cannot be null");
}
Object[] parameters = new Object[] {this.getId()};
String pattern = "v1/invoicing/invoices/{0}/cancel";
String resourcePath = RESTUtil.formatURIPath(pattern, parameters);
String payLoad = cancelNotification.toJSON();
configureAndExecute(apiContext, HttpMethod.POST, resourcePath, payLoad, null);
}
/**
* Marks the status of a specified invoice, by ID, as paid. Include a payment detail object that defines the payment method and other details in the JSON request body.
* @deprecated Please use {@link #recordPayment(APIContext, PaymentDetail)} instead.
* @param accessToken
* Access Token used for the API call.
* @param paymentDetail
* PaymentDetail
* @throws PayPalRESTException
*/
public void recordPayment(String accessToken, PaymentDetail paymentDetail) throws PayPalRESTException {
APIContext apiContext = new APIContext(accessToken);
recordPayment(apiContext, paymentDetail);
}
/**
* Marks the status of a specified invoice, by ID, as paid. Include a payment detail object that defines the payment method and other details in the JSON request body.
* @param apiContext
* {@link APIContext} used for the API call.
* @param paymentDetail
* PaymentDetail
* @throws PayPalRESTException
*/
public void recordPayment(APIContext apiContext, PaymentDetail paymentDetail) throws PayPalRESTException {
if (this.getId() == null) {
throw new IllegalArgumentException("Id cannot be null");
}
if (paymentDetail == null) {
throw new IllegalArgumentException("paymentDetail cannot be null");
}
Object[] parameters = new Object[] {this.getId()};
String pattern = "v1/invoicing/invoices/{0}/record-payment";
String resourcePath = RESTUtil.formatURIPath(pattern, parameters);
String payLoad = paymentDetail.toJSON();
configureAndExecute(apiContext, HttpMethod.POST, resourcePath, payLoad, null);
}
/**
* Marks the status of a specified invoice, by ID, as refunded. Include a refund detail object that defines the refund type and other details in the JSON request body.
* @deprecated Please use {@link #recordRefund(APIContext, RefundDetail)} instead.
* @param accessToken
* Access Token used for the API call.
* @param refundDetail
* RefundDetail
* @throws PayPalRESTException
*/
public void recordRefund(String accessToken, RefundDetail refundDetail) throws PayPalRESTException {
APIContext apiContext = new APIContext(accessToken);
recordRefund(apiContext, refundDetail);
}
/**
* Marks the status of a specified invoice, by ID, as refunded. Include a refund detail object that defines the refund type and other details in the JSON request body.
* @param apiContext
* {@link APIContext} used for the API call.
* @param refundDetail
* RefundDetail
* @throws PayPalRESTException
*/
public void recordRefund(APIContext apiContext, RefundDetail refundDetail) throws PayPalRESTException {
if (this.getId() == null) {
throw new IllegalArgumentException("Id cannot be null");
}
if (refundDetail == null) {
throw new IllegalArgumentException("refundDetail cannot be null");
}
Object[] parameters = new Object[] {this.getId()};
String pattern = "v1/invoicing/invoices/{0}/record-refund";
String resourcePath = RESTUtil.formatURIPath(pattern, parameters);
String payLoad = refundDetail.toJSON();
configureAndExecute(apiContext, HttpMethod.POST, resourcePath, payLoad, null);
}
/**
* Gets the details for a specified invoice, by ID.
* @deprecated Please use {@link #get(APIContext, String)} instead.
* @param accessToken
* Access Token used for the API call.
* @param invoiceId
* String
* @return Invoice
* @throws PayPalRESTException
*/
public static Invoice get(String accessToken, String invoiceId) throws PayPalRESTException {
APIContext apiContext = new APIContext(accessToken);
return get(apiContext, invoiceId);
}
/**
* Gets the details for a specified invoice, by ID.
* @param apiContext
* {@link APIContext} used for the API call.
* @param invoiceId
* String
* @return Invoice
* @throws PayPalRESTException
*/
public static Invoice get(APIContext apiContext, String invoiceId) throws PayPalRESTException {
if (invoiceId == null) {
throw new IllegalArgumentException("invoiceId cannot be null");
}
Object[] parameters = new Object[] {invoiceId};
String pattern = "v1/invoicing/invoices/{0}";
String resourcePath = RESTUtil.formatURIPath(pattern, parameters);
String payLoad = "";
return configureAndExecute(apiContext, HttpMethod.GET, resourcePath, payLoad, Invoice.class);
}
/**
* Lists some or all merchant invoices. Filters the response by any specified optional query string parameters.
* @deprecated Please use {@link #getAll(APIContext)} instead.
* @param accessToken
* Access Token used for the API call.
* @return Invoices
* @throws PayPalRESTException
*/
public static Invoices getAll(String accessToken) throws PayPalRESTException {
APIContext apiContext = new APIContext(accessToken);
return getAll(apiContext, null);
}
/**
* Lists some or all merchant invoices. Filters the response by any specified optional query string parameters.
* @param apiContext
* {@link APIContext} used for the API call.
* @return Invoices
* @throws PayPalRESTException
*/
public static Invoices getAll(APIContext apiContext) throws PayPalRESTException {
return getAll(apiContext, null);
}
/**
* Lists some or all merchant invoices. Filters the response by any specified optional query string parameters.
* @param apiContext
* {@link APIContext} used for the API call.
* @param options
* {@link Map} of query parameters. Allowed options: page, page_size, total_count_required.
* @return Invoices
* @throws PayPalRESTException
*/
public static Invoices getAll(APIContext apiContext, Map options) throws PayPalRESTException {
String pattern = "v1/invoicing/invoices";
String resourcePath = RESTUtil.formatURIPath(pattern, null, options);
String payLoad = "";
return configureAndExecute(apiContext, HttpMethod.GET, resourcePath, payLoad, Invoices.class);
}
/**
* Fully updates an invoice by passing the invoice ID to the request URI. In addition, pass a complete invoice object in the request JSON. Partial updates are not supported.
* @deprecated Please use {@link #update(APIContext)} instead.
* @param accessToken
* Access Token used for the API call.
* @return Invoice
* @throws PayPalRESTException
*/
public Invoice update(String accessToken) throws PayPalRESTException {
APIContext apiContext = new APIContext(accessToken);
return update(apiContext);
}
/**
* Fully updates an invoice by passing the invoice ID to the request URI. In addition, pass a complete invoice object in the request JSON. Partial updates are not supported.
* @param apiContext
* {@link APIContext} used for the API call.
* @return Invoice
* @throws PayPalRESTException
*/
public Invoice update(APIContext apiContext) throws PayPalRESTException {
if (this.getId() == null) {
throw new IllegalArgumentException("Id cannot be null");
}
Object[] parameters = new Object[] {this.getId()};
String pattern = "v1/invoicing/invoices/{0}";
String resourcePath = RESTUtil.formatURIPath(pattern, parameters);
String payLoad = this.toJSON();
return configureAndExecute(apiContext, HttpMethod.PUT, resourcePath, payLoad, Invoice.class);
}
/**
* Delete a particular invoice by passing the invoice ID to the request URI.
* @deprecated Please use {@link #delete(APIContext)} instead.
* @param accessToken
* Access Token used for the API call.
* @throws PayPalRESTException
*/
public void delete(String accessToken) throws PayPalRESTException {
APIContext apiContext = new APIContext(accessToken);
delete(apiContext);
}
/**
* Delete a particular invoice by passing the invoice ID to the request URI.
* @param apiContext
* {@link APIContext} used for the API call.
* @throws PayPalRESTException
*/
public void delete(APIContext apiContext) throws PayPalRESTException {
if (this.getId() == null) {
throw new IllegalArgumentException("Id cannot be null");
}
apiContext.setRequestId(null);
Object[] parameters = new Object[] {this.getId()};
String pattern = "v1/invoicing/invoices/{0}";
String resourcePath = RESTUtil.formatURIPath(pattern, parameters);
String payLoad = "";
configureAndExecute(apiContext, HttpMethod.DELETE, resourcePath, payLoad, null);
apiContext.setRequestId(null);
}
/**
* Delete external payment.
* @param apiContext
* {@link APIContext} used for the API call.
* @return
* @throws PayPalRESTException
*/
public void deleteExternalPayment(APIContext apiContext) throws PayPalRESTException {
if (this.getId() == null) {
throw new IllegalArgumentException("Id cannot be null");
}
apiContext.setMaskRequestId(true);
Object[] parameters = new Object[] {this.getId()};
String pattern = "v1/invoicing/invoices/{0}/payment-records/{1}";
String resourcePath = RESTUtil.formatURIPath(pattern, parameters);
String payLoad = "";
configureAndExecute(apiContext, HttpMethod.DELETE, resourcePath, payLoad, null);
}
/**
* Delete external refund.
* @param apiContext
* {@link APIContext} used for the API call.
* @return
* @throws PayPalRESTException
*/
public void deleteExternalRefund(APIContext apiContext) throws PayPalRESTException {
if (this.getId() == null) {
throw new IllegalArgumentException("Id cannot be null");
}
apiContext.setMaskRequestId(true);
Object[] parameters = new Object[] {this.getId()};
String pattern = "v1/invoicing/invoices/{0}/refund-records/{1}";
String resourcePath = RESTUtil.formatURIPath(pattern, parameters);
String payLoad = "";
configureAndExecute(apiContext, HttpMethod.DELETE, resourcePath, payLoad, null);
}
/**
* Generates a QR code for an invoice, by ID. The request generates a QR code that is 500 pixels in width and height. To change the dimensions of the returned code, specify optional query parameters.
* @deprecated Please use {@link #qrCode(APIContext, String, Map)} instead.
* @param apiContext
* {@link APIContext} used for the API call.
* @param invoiceId
* String
* @return object
* @throws PayPalRESTException
*/
public static Image qrCode(APIContext apiContext, String invoiceId) throws PayPalRESTException {
return qrCode(apiContext, invoiceId, null);
}
/**
* Generates a QR code for an invoice, by ID. The request generates a QR code that is 500 pixels in width and height. To change the dimensions of the returned code, specify optional query parameters.
* @param apiContext
* {@link APIContext} used for the API call.
* @param invoiceId
* String
* @param options
* {@link Map} of options. Valid values are: width, height, action.
* @return object
* @throws PayPalRESTException
*/
public static Image qrCode(APIContext apiContext, String invoiceId, Map options) throws PayPalRESTException {
if (invoiceId == null) {
throw new IllegalArgumentException("invoiceId cannot be null");
}
String pattern = "v1/invoicing/invoices/{0}/qr-code";
String resourcePath = RESTUtil.formatURIPath(pattern, options, invoiceId);
String payLoad = "";
return configureAndExecute(apiContext, HttpMethod.GET, resourcePath, payLoad, Image.class);
}
/**
* Generates the next invoice number.
* @param apiContext
* {@link APIContext} used for the API call.
* @return object
* @throws PayPalRESTException
*/
public InvoiceNumber generateNumber(APIContext apiContext) throws PayPalRESTException {
Object[] parameters = new Object[] {this.getId()};
String pattern = "v1/invoicing/invoices/next-invoice-number";
String resourcePath = RESTUtil.formatURIPath(pattern, parameters);
String payLoad = "";
return configureAndExecute(apiContext, HttpMethod.POST, resourcePath, payLoad, InvoiceNumber.class);
}
/**
* Fetches long lived refresh token from authorization code, for third party merchant invoicing use.
*
* @param context context
* @param authorizationCode authorization code
* @return {@link String} Refresh Token
* @throws PayPalRESTException
*/
public static String fetchRefreshToken(APIContext context, String authorizationCode) throws PayPalRESTException {
return Tokeninfo.createFromAuthorizationCode(context, authorizationCode).getRefreshToken();
}
/**
* The unique invoice resource identifier.
*/
@java.lang.SuppressWarnings("all")
public String getId() {
return this.id;
}
/**
* Unique number that appears on the invoice. If left blank will be auto-incremented from the last number. 25 characters max.
*/
@java.lang.SuppressWarnings("all")
public String getNumber() {
return this.number;
}
/**
* The template ID used for the invoice. Useful for copy functionality.
*/
@java.lang.SuppressWarnings("all")
public String getTemplateId() {
return this.templateId;
}
/**
* URI of the invoice resource.
*/
@java.lang.SuppressWarnings("all")
public String getUri() {
return this.uri;
}
/**
* Status of the invoice.
*/
@java.lang.SuppressWarnings("all")
public String getStatus() {
return this.status;
}
/**
* Information about the merchant who is sending the invoice.
*/
@java.lang.SuppressWarnings("all")
public MerchantInfo getMerchantInfo() {
return this.merchantInfo;
}
/**
* The required invoice recipient email address and any optional billing information. One recipient is supported.
*/
@java.lang.SuppressWarnings("all")
public List getBillingInfo() {
return this.billingInfo;
}
/**
* For invoices sent by email, one or more email addresses to which to send a Cc: copy of the notification. Supports only email addresses under participant.
*/
@java.lang.SuppressWarnings("all")
public List getCcInfo() {
return this.ccInfo;
}
/**
* The shipping information for entities to whom items are being shipped.
*/
@java.lang.SuppressWarnings("all")
public ShippingInfo getShippingInfo() {
return this.shippingInfo;
}
/**
* The list of items to include in the invoice. Maximum value is 100 items per invoice.
*/
@java.lang.SuppressWarnings("all")
public List getItems() {
return this.items;
}
/**
* The date when the invoice was enabled. The date format is *yyyy*-*MM*-*dd* *z* as defined in [Internet Date/Time Format](http://tools.ietf.org/html/rfc3339#section-5.6).
*/
@java.lang.SuppressWarnings("all")
public String getInvoiceDate() {
return this.invoiceDate;
}
/**
* Optional. The payment deadline for the invoice. Value is either `term_type` or `due_date` but not both.
*/
@java.lang.SuppressWarnings("all")
public PaymentTerm getPaymentTerm() {
return this.paymentTerm;
}
/**
* Reference data, such as PO number, to add to the invoice. Maximum length is 60 characters.
*/
@java.lang.SuppressWarnings("all")
public String getReference() {
return this.reference;
}
/**
* The invoice level discount, as a percent or an amount value.
*/
@java.lang.SuppressWarnings("all")
public Cost getDiscount() {
return this.discount;
}
/**
* The shipping cost, as a percent or an amount value.
*/
@java.lang.SuppressWarnings("all")
public ShippingCost getShippingCost() {
return this.shippingCost;
}
/**
* The custom amount to apply on an invoice. If you include a label, the amount cannot be empty.
*/
@java.lang.SuppressWarnings("all")
public CustomAmount getCustom() {
return this.custom;
}
/**
* Indicates whether the invoice allows a partial payment. If set to `false`, invoice must be paid in full. If set to `true`, the invoice allows partial payments. Default is `false`.
*/
@java.lang.SuppressWarnings("all")
public Boolean getAllowPartialPayment() {
return this.allowPartialPayment;
}
/**
* If `allow_partial_payment` is set to `true`, the minimum amount allowed for a partial payment.
*/
@java.lang.SuppressWarnings("all")
public Currency getMinimumAmountDue() {
return this.minimumAmountDue;
}
/**
* Indicates whether tax is calculated before or after a discount. If set to `false`, the tax is calculated before a discount. If set to `true`, the tax is calculated after a discount. Default is `false`.
*/
@java.lang.SuppressWarnings("all")
public Boolean getTaxCalculatedAfterDiscount() {
return this.taxCalculatedAfterDiscount;
}
/**
* Indicates whether the unit price includes tax. Default is `false`.
*/
@java.lang.SuppressWarnings("all")
public Boolean getTaxInclusive() {
return this.taxInclusive;
}
/**
* General terms of the invoice. 4000 characters max.
*/
@java.lang.SuppressWarnings("all")
public String getTerms() {
return this.terms;
}
/**
* Note to the payer. 4000 characters max.
*/
@java.lang.SuppressWarnings("all")
public String getNote() {
return this.note;
}
/**
* A private bookkeeping memo for the merchant. Maximum length is 150 characters.
*/
@java.lang.SuppressWarnings("all")
public String getMerchantMemo() {
return this.merchantMemo;
}
/**
* Full URL of an external image to use as the logo. Maximum length is 4000 characters.
*/
@java.lang.SuppressWarnings("all")
public String getLogoUrl() {
return this.logoUrl;
}
/**
* The total amount of the invoice.
*/
@java.lang.SuppressWarnings("all")
public Currency getTotalAmount() {
return this.totalAmount;
}
/**
* List of payment details for the invoice.
*/
@java.lang.SuppressWarnings("all")
public List getPayments() {
return this.payments;
}
/**
* List of refund details for the invoice.
*/
@java.lang.SuppressWarnings("all")
public List getRefunds() {
return this.refunds;
}
/**
* Audit information for the invoice.
*/
@java.lang.SuppressWarnings("all")
public Metadata getMetadata() {
return this.metadata;
}
/**
* Any miscellaneous invoice data. Maximum length is 4000 characters.
*/
@java.lang.SuppressWarnings("all")
public String getAdditionalData() {
return this.additionalData;
}
/**
* Gratuity to include with the invoice.
*/
@java.lang.SuppressWarnings("all")
public Currency getGratuity() {
return this.gratuity;
}
/**
* Payment summary of the invoice including amount paid through PayPal and other sources.
*/
@java.lang.SuppressWarnings("all")
public PaymentSummary getPaidAmount() {
return this.paidAmount;
}
/**
* Payment summary of the invoice including amount refunded through PayPal and other sources.
*/
@java.lang.SuppressWarnings("all")
public PaymentSummary getRefundedAmount() {
return this.refundedAmount;
}
/**
* List of files attached to the invoice.
*/
@java.lang.SuppressWarnings("all")
public List getAttachments() {
return this.attachments;
}
/**
* HATEOS links representing all the actions over the invoice resource based on the current invoice status.
*/
@java.lang.SuppressWarnings("all")
public List getLinks() {
return this.links;
}
/**
* The unique invoice resource identifier.
* @return this
*/
@java.lang.SuppressWarnings("all")
public Invoice setId(final String id) {
this.id = id;
return this;
}
/**
* Unique number that appears on the invoice. If left blank will be auto-incremented from the last number. 25 characters max.
* @return this
*/
@java.lang.SuppressWarnings("all")
public Invoice setNumber(final String number) {
this.number = number;
return this;
}
/**
* The template ID used for the invoice. Useful for copy functionality.
* @return this
*/
@java.lang.SuppressWarnings("all")
public Invoice setTemplateId(final String templateId) {
this.templateId = templateId;
return this;
}
/**
* URI of the invoice resource.
* @return this
*/
@java.lang.SuppressWarnings("all")
public Invoice setUri(final String uri) {
this.uri = uri;
return this;
}
/**
* Status of the invoice.
* @return this
*/
@java.lang.SuppressWarnings("all")
public Invoice setStatus(final String status) {
this.status = status;
return this;
}
/**
* Information about the merchant who is sending the invoice.
* @return this
*/
@java.lang.SuppressWarnings("all")
public Invoice setMerchantInfo(final MerchantInfo merchantInfo) {
this.merchantInfo = merchantInfo;
return this;
}
/**
* The required invoice recipient email address and any optional billing information. One recipient is supported.
* @return this
*/
@java.lang.SuppressWarnings("all")
public Invoice setBillingInfo(final List billingInfo) {
this.billingInfo = billingInfo;
return this;
}
/**
* For invoices sent by email, one or more email addresses to which to send a Cc: copy of the notification. Supports only email addresses under participant.
* @return this
*/
@java.lang.SuppressWarnings("all")
public Invoice setCcInfo(final List ccInfo) {
this.ccInfo = ccInfo;
return this;
}
/**
* The shipping information for entities to whom items are being shipped.
* @return this
*/
@java.lang.SuppressWarnings("all")
public Invoice setShippingInfo(final ShippingInfo shippingInfo) {
this.shippingInfo = shippingInfo;
return this;
}
/**
* The list of items to include in the invoice. Maximum value is 100 items per invoice.
* @return this
*/
@java.lang.SuppressWarnings("all")
public Invoice setItems(final List items) {
this.items = items;
return this;
}
/**
* The date when the invoice was enabled. The date format is *yyyy*-*MM*-*dd* *z* as defined in [Internet Date/Time Format](http://tools.ietf.org/html/rfc3339#section-5.6).
* @return this
*/
@java.lang.SuppressWarnings("all")
public Invoice setInvoiceDate(final String invoiceDate) {
this.invoiceDate = invoiceDate;
return this;
}
/**
* Optional. The payment deadline for the invoice. Value is either `term_type` or `due_date` but not both.
* @return this
*/
@java.lang.SuppressWarnings("all")
public Invoice setPaymentTerm(final PaymentTerm paymentTerm) {
this.paymentTerm = paymentTerm;
return this;
}
/**
* Reference data, such as PO number, to add to the invoice. Maximum length is 60 characters.
* @return this
*/
@java.lang.SuppressWarnings("all")
public Invoice setReference(final String reference) {
this.reference = reference;
return this;
}
/**
* The invoice level discount, as a percent or an amount value.
* @return this
*/
@java.lang.SuppressWarnings("all")
public Invoice setDiscount(final Cost discount) {
this.discount = discount;
return this;
}
/**
* The shipping cost, as a percent or an amount value.
* @return this
*/
@java.lang.SuppressWarnings("all")
public Invoice setShippingCost(final ShippingCost shippingCost) {
this.shippingCost = shippingCost;
return this;
}
/**
* The custom amount to apply on an invoice. If you include a label, the amount cannot be empty.
* @return this
*/
@java.lang.SuppressWarnings("all")
public Invoice setCustom(final CustomAmount custom) {
this.custom = custom;
return this;
}
/**
* Indicates whether the invoice allows a partial payment. If set to `false`, invoice must be paid in full. If set to `true`, the invoice allows partial payments. Default is `false`.
* @return this
*/
@java.lang.SuppressWarnings("all")
public Invoice setAllowPartialPayment(final Boolean allowPartialPayment) {
this.allowPartialPayment = allowPartialPayment;
return this;
}
/**
* If `allow_partial_payment` is set to `true`, the minimum amount allowed for a partial payment.
* @return this
*/
@java.lang.SuppressWarnings("all")
public Invoice setMinimumAmountDue(final Currency minimumAmountDue) {
this.minimumAmountDue = minimumAmountDue;
return this;
}
/**
* Indicates whether tax is calculated before or after a discount. If set to `false`, the tax is calculated before a discount. If set to `true`, the tax is calculated after a discount. Default is `false`.
* @return this
*/
@java.lang.SuppressWarnings("all")
public Invoice setTaxCalculatedAfterDiscount(final Boolean taxCalculatedAfterDiscount) {
this.taxCalculatedAfterDiscount = taxCalculatedAfterDiscount;
return this;
}
/**
* Indicates whether the unit price includes tax. Default is `false`.
* @return this
*/
@java.lang.SuppressWarnings("all")
public Invoice setTaxInclusive(final Boolean taxInclusive) {
this.taxInclusive = taxInclusive;
return this;
}
/**
* General terms of the invoice. 4000 characters max.
* @return this
*/
@java.lang.SuppressWarnings("all")
public Invoice setTerms(final String terms) {
this.terms = terms;
return this;
}
/**
* Note to the payer. 4000 characters max.
* @return this
*/
@java.lang.SuppressWarnings("all")
public Invoice setNote(final String note) {
this.note = note;
return this;
}
/**
* A private bookkeeping memo for the merchant. Maximum length is 150 characters.
* @return this
*/
@java.lang.SuppressWarnings("all")
public Invoice setMerchantMemo(final String merchantMemo) {
this.merchantMemo = merchantMemo;
return this;
}
/**
* Full URL of an external image to use as the logo. Maximum length is 4000 characters.
* @return this
*/
@java.lang.SuppressWarnings("all")
public Invoice setLogoUrl(final String logoUrl) {
this.logoUrl = logoUrl;
return this;
}
/**
* The total amount of the invoice.
* @return this
*/
@java.lang.SuppressWarnings("all")
public Invoice setTotalAmount(final Currency totalAmount) {
this.totalAmount = totalAmount;
return this;
}
/**
* List of payment details for the invoice.
* @return this
*/
@java.lang.SuppressWarnings("all")
public Invoice setPayments(final List payments) {
this.payments = payments;
return this;
}
/**
* List of refund details for the invoice.
* @return this
*/
@java.lang.SuppressWarnings("all")
public Invoice setRefunds(final List refunds) {
this.refunds = refunds;
return this;
}
/**
* Audit information for the invoice.
* @return this
*/
@java.lang.SuppressWarnings("all")
public Invoice setMetadata(final Metadata metadata) {
this.metadata = metadata;
return this;
}
/**
* Any miscellaneous invoice data. Maximum length is 4000 characters.
* @return this
*/
@java.lang.SuppressWarnings("all")
public Invoice setAdditionalData(final String additionalData) {
this.additionalData = additionalData;
return this;
}
/**
* Gratuity to include with the invoice.
* @return this
*/
@java.lang.SuppressWarnings("all")
public Invoice setGratuity(final Currency gratuity) {
this.gratuity = gratuity;
return this;
}
/**
* Payment summary of the invoice including amount paid through PayPal and other sources.
* @return this
*/
@java.lang.SuppressWarnings("all")
public Invoice setPaidAmount(final PaymentSummary paidAmount) {
this.paidAmount = paidAmount;
return this;
}
/**
* Payment summary of the invoice including amount refunded through PayPal and other sources.
* @return this
*/
@java.lang.SuppressWarnings("all")
public Invoice setRefundedAmount(final PaymentSummary refundedAmount) {
this.refundedAmount = refundedAmount;
return this;
}
/**
* List of files attached to the invoice.
* @return this
*/
@java.lang.SuppressWarnings("all")
public Invoice setAttachments(final List attachments) {
this.attachments = attachments;
return this;
}
/**
* HATEOS links representing all the actions over the invoice resource based on the current invoice status.
* @return this
*/
@java.lang.SuppressWarnings("all")
public Invoice setLinks(final List links) {
this.links = links;
return this;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Invoice)) return false;
final Invoice other = (Invoice) o;
if (!other.canEqual((java.lang.Object) this)) return false;
if (!super.equals(o)) return false;
final java.lang.Object this$id = this.getId();
final java.lang.Object other$id = other.getId();
if (this$id == null ? other$id != null : !this$id.equals(other$id)) return false;
final java.lang.Object this$number = this.getNumber();
final java.lang.Object other$number = other.getNumber();
if (this$number == null ? other$number != null : !this$number.equals(other$number)) return false;
final java.lang.Object this$templateId = this.getTemplateId();
final java.lang.Object other$templateId = other.getTemplateId();
if (this$templateId == null ? other$templateId != null : !this$templateId.equals(other$templateId)) return false;
final java.lang.Object this$uri = this.getUri();
final java.lang.Object other$uri = other.getUri();
if (this$uri == null ? other$uri != null : !this$uri.equals(other$uri)) return false;
final java.lang.Object this$status = this.getStatus();
final java.lang.Object other$status = other.getStatus();
if (this$status == null ? other$status != null : !this$status.equals(other$status)) return false;
final java.lang.Object this$merchantInfo = this.getMerchantInfo();
final java.lang.Object other$merchantInfo = other.getMerchantInfo();
if (this$merchantInfo == null ? other$merchantInfo != null : !this$merchantInfo.equals(other$merchantInfo)) return false;
final java.lang.Object this$billingInfo = this.getBillingInfo();
final java.lang.Object other$billingInfo = other.getBillingInfo();
if (this$billingInfo == null ? other$billingInfo != null : !this$billingInfo.equals(other$billingInfo)) return false;
final java.lang.Object this$ccInfo = this.getCcInfo();
final java.lang.Object other$ccInfo = other.getCcInfo();
if (this$ccInfo == null ? other$ccInfo != null : !this$ccInfo.equals(other$ccInfo)) return false;
final java.lang.Object this$shippingInfo = this.getShippingInfo();
final java.lang.Object other$shippingInfo = other.getShippingInfo();
if (this$shippingInfo == null ? other$shippingInfo != null : !this$shippingInfo.equals(other$shippingInfo)) return false;
final java.lang.Object this$items = this.getItems();
final java.lang.Object other$items = other.getItems();
if (this$items == null ? other$items != null : !this$items.equals(other$items)) return false;
final java.lang.Object this$invoiceDate = this.getInvoiceDate();
final java.lang.Object other$invoiceDate = other.getInvoiceDate();
if (this$invoiceDate == null ? other$invoiceDate != null : !this$invoiceDate.equals(other$invoiceDate)) return false;
final java.lang.Object this$paymentTerm = this.getPaymentTerm();
final java.lang.Object other$paymentTerm = other.getPaymentTerm();
if (this$paymentTerm == null ? other$paymentTerm != null : !this$paymentTerm.equals(other$paymentTerm)) return false;
final java.lang.Object this$reference = this.getReference();
final java.lang.Object other$reference = other.getReference();
if (this$reference == null ? other$reference != null : !this$reference.equals(other$reference)) return false;
final java.lang.Object this$discount = this.getDiscount();
final java.lang.Object other$discount = other.getDiscount();
if (this$discount == null ? other$discount != null : !this$discount.equals(other$discount)) return false;
final java.lang.Object this$shippingCost = this.getShippingCost();
final java.lang.Object other$shippingCost = other.getShippingCost();
if (this$shippingCost == null ? other$shippingCost != null : !this$shippingCost.equals(other$shippingCost)) return false;
final java.lang.Object this$custom = this.getCustom();
final java.lang.Object other$custom = other.getCustom();
if (this$custom == null ? other$custom != null : !this$custom.equals(other$custom)) return false;
final java.lang.Object this$allowPartialPayment = this.getAllowPartialPayment();
final java.lang.Object other$allowPartialPayment = other.getAllowPartialPayment();
if (this$allowPartialPayment == null ? other$allowPartialPayment != null : !this$allowPartialPayment.equals(other$allowPartialPayment)) return false;
final java.lang.Object this$minimumAmountDue = this.getMinimumAmountDue();
final java.lang.Object other$minimumAmountDue = other.getMinimumAmountDue();
if (this$minimumAmountDue == null ? other$minimumAmountDue != null : !this$minimumAmountDue.equals(other$minimumAmountDue)) return false;
final java.lang.Object this$taxCalculatedAfterDiscount = this.getTaxCalculatedAfterDiscount();
final java.lang.Object other$taxCalculatedAfterDiscount = other.getTaxCalculatedAfterDiscount();
if (this$taxCalculatedAfterDiscount == null ? other$taxCalculatedAfterDiscount != null : !this$taxCalculatedAfterDiscount.equals(other$taxCalculatedAfterDiscount)) return false;
final java.lang.Object this$taxInclusive = this.getTaxInclusive();
final java.lang.Object other$taxInclusive = other.getTaxInclusive();
if (this$taxInclusive == null ? other$taxInclusive != null : !this$taxInclusive.equals(other$taxInclusive)) return false;
final java.lang.Object this$terms = this.getTerms();
final java.lang.Object other$terms = other.getTerms();
if (this$terms == null ? other$terms != null : !this$terms.equals(other$terms)) return false;
final java.lang.Object this$note = this.getNote();
final java.lang.Object other$note = other.getNote();
if (this$note == null ? other$note != null : !this$note.equals(other$note)) return false;
final java.lang.Object this$merchantMemo = this.getMerchantMemo();
final java.lang.Object other$merchantMemo = other.getMerchantMemo();
if (this$merchantMemo == null ? other$merchantMemo != null : !this$merchantMemo.equals(other$merchantMemo)) return false;
final java.lang.Object this$logoUrl = this.getLogoUrl();
final java.lang.Object other$logoUrl = other.getLogoUrl();
if (this$logoUrl == null ? other$logoUrl != null : !this$logoUrl.equals(other$logoUrl)) return false;
final java.lang.Object this$totalAmount = this.getTotalAmount();
final java.lang.Object other$totalAmount = other.getTotalAmount();
if (this$totalAmount == null ? other$totalAmount != null : !this$totalAmount.equals(other$totalAmount)) return false;
final java.lang.Object this$payments = this.getPayments();
final java.lang.Object other$payments = other.getPayments();
if (this$payments == null ? other$payments != null : !this$payments.equals(other$payments)) return false;
final java.lang.Object this$refunds = this.getRefunds();
final java.lang.Object other$refunds = other.getRefunds();
if (this$refunds == null ? other$refunds != null : !this$refunds.equals(other$refunds)) return false;
final java.lang.Object this$metadata = this.getMetadata();
final java.lang.Object other$metadata = other.getMetadata();
if (this$metadata == null ? other$metadata != null : !this$metadata.equals(other$metadata)) return false;
final java.lang.Object this$additionalData = this.getAdditionalData();
final java.lang.Object other$additionalData = other.getAdditionalData();
if (this$additionalData == null ? other$additionalData != null : !this$additionalData.equals(other$additionalData)) return false;
final java.lang.Object this$gratuity = this.getGratuity();
final java.lang.Object other$gratuity = other.getGratuity();
if (this$gratuity == null ? other$gratuity != null : !this$gratuity.equals(other$gratuity)) return false;
final java.lang.Object this$paidAmount = this.getPaidAmount();
final java.lang.Object other$paidAmount = other.getPaidAmount();
if (this$paidAmount == null ? other$paidAmount != null : !this$paidAmount.equals(other$paidAmount)) return false;
final java.lang.Object this$refundedAmount = this.getRefundedAmount();
final java.lang.Object other$refundedAmount = other.getRefundedAmount();
if (this$refundedAmount == null ? other$refundedAmount != null : !this$refundedAmount.equals(other$refundedAmount)) return false;
final java.lang.Object this$attachments = this.getAttachments();
final java.lang.Object other$attachments = other.getAttachments();
if (this$attachments == null ? other$attachments != null : !this$attachments.equals(other$attachments)) return false;
final java.lang.Object this$links = this.getLinks();
final java.lang.Object other$links = other.getLinks();
if (this$links == null ? other$links != null : !this$links.equals(other$links)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Invoice;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public int hashCode() {
final int PRIME = 59;
int result = 1;
result = result * PRIME + super.hashCode();
final java.lang.Object $id = this.getId();
result = result * PRIME + ($id == null ? 43 : $id.hashCode());
final java.lang.Object $number = this.getNumber();
result = result * PRIME + ($number == null ? 43 : $number.hashCode());
final java.lang.Object $templateId = this.getTemplateId();
result = result * PRIME + ($templateId == null ? 43 : $templateId.hashCode());
final java.lang.Object $uri = this.getUri();
result = result * PRIME + ($uri == null ? 43 : $uri.hashCode());
final java.lang.Object $status = this.getStatus();
result = result * PRIME + ($status == null ? 43 : $status.hashCode());
final java.lang.Object $merchantInfo = this.getMerchantInfo();
result = result * PRIME + ($merchantInfo == null ? 43 : $merchantInfo.hashCode());
final java.lang.Object $billingInfo = this.getBillingInfo();
result = result * PRIME + ($billingInfo == null ? 43 : $billingInfo.hashCode());
final java.lang.Object $ccInfo = this.getCcInfo();
result = result * PRIME + ($ccInfo == null ? 43 : $ccInfo.hashCode());
final java.lang.Object $shippingInfo = this.getShippingInfo();
result = result * PRIME + ($shippingInfo == null ? 43 : $shippingInfo.hashCode());
final java.lang.Object $items = this.getItems();
result = result * PRIME + ($items == null ? 43 : $items.hashCode());
final java.lang.Object $invoiceDate = this.getInvoiceDate();
result = result * PRIME + ($invoiceDate == null ? 43 : $invoiceDate.hashCode());
final java.lang.Object $paymentTerm = this.getPaymentTerm();
result = result * PRIME + ($paymentTerm == null ? 43 : $paymentTerm.hashCode());
final java.lang.Object $reference = this.getReference();
result = result * PRIME + ($reference == null ? 43 : $reference.hashCode());
final java.lang.Object $discount = this.getDiscount();
result = result * PRIME + ($discount == null ? 43 : $discount.hashCode());
final java.lang.Object $shippingCost = this.getShippingCost();
result = result * PRIME + ($shippingCost == null ? 43 : $shippingCost.hashCode());
final java.lang.Object $custom = this.getCustom();
result = result * PRIME + ($custom == null ? 43 : $custom.hashCode());
final java.lang.Object $allowPartialPayment = this.getAllowPartialPayment();
result = result * PRIME + ($allowPartialPayment == null ? 43 : $allowPartialPayment.hashCode());
final java.lang.Object $minimumAmountDue = this.getMinimumAmountDue();
result = result * PRIME + ($minimumAmountDue == null ? 43 : $minimumAmountDue.hashCode());
final java.lang.Object $taxCalculatedAfterDiscount = this.getTaxCalculatedAfterDiscount();
result = result * PRIME + ($taxCalculatedAfterDiscount == null ? 43 : $taxCalculatedAfterDiscount.hashCode());
final java.lang.Object $taxInclusive = this.getTaxInclusive();
result = result * PRIME + ($taxInclusive == null ? 43 : $taxInclusive.hashCode());
final java.lang.Object $terms = this.getTerms();
result = result * PRIME + ($terms == null ? 43 : $terms.hashCode());
final java.lang.Object $note = this.getNote();
result = result * PRIME + ($note == null ? 43 : $note.hashCode());
final java.lang.Object $merchantMemo = this.getMerchantMemo();
result = result * PRIME + ($merchantMemo == null ? 43 : $merchantMemo.hashCode());
final java.lang.Object $logoUrl = this.getLogoUrl();
result = result * PRIME + ($logoUrl == null ? 43 : $logoUrl.hashCode());
final java.lang.Object $totalAmount = this.getTotalAmount();
result = result * PRIME + ($totalAmount == null ? 43 : $totalAmount.hashCode());
final java.lang.Object $payments = this.getPayments();
result = result * PRIME + ($payments == null ? 43 : $payments.hashCode());
final java.lang.Object $refunds = this.getRefunds();
result = result * PRIME + ($refunds == null ? 43 : $refunds.hashCode());
final java.lang.Object $metadata = this.getMetadata();
result = result * PRIME + ($metadata == null ? 43 : $metadata.hashCode());
final java.lang.Object $additionalData = this.getAdditionalData();
result = result * PRIME + ($additionalData == null ? 43 : $additionalData.hashCode());
final java.lang.Object $gratuity = this.getGratuity();
result = result * PRIME + ($gratuity == null ? 43 : $gratuity.hashCode());
final java.lang.Object $paidAmount = this.getPaidAmount();
result = result * PRIME + ($paidAmount == null ? 43 : $paidAmount.hashCode());
final java.lang.Object $refundedAmount = this.getRefundedAmount();
result = result * PRIME + ($refundedAmount == null ? 43 : $refundedAmount.hashCode());
final java.lang.Object $attachments = this.getAttachments();
result = result * PRIME + ($attachments == null ? 43 : $attachments.hashCode());
final java.lang.Object $links = this.getLinks();
result = result * PRIME + ($links == null ? 43 : $links.hashCode());
return result;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy