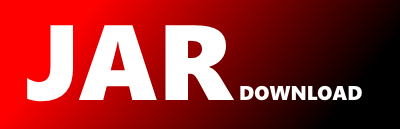
com.paypal.api.payments.InvoicingNotification Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rest-api-sdk Show documentation
Show all versions of rest-api-sdk Show documentation
PayPal SDK for integrating with the REST APIs
// Generated by delombok at Wed Oct 12 18:15:55 CDT 2016
package com.paypal.api.payments;
import com.paypal.base.rest.PayPalModel;
public class InvoicingNotification extends PayPalModel {
/**
* Subject of the notification.
*/
private String subject;
/**
* Note to the payer.
*/
private String note;
/**
* A flag indicating whether a copy of the email has to be sent to the merchant.
*/
private Boolean sendToMerchant;
/**
* Default Constructor
*/
public InvoicingNotification() {
}
/**
* Subject of the notification.
*/
@java.lang.SuppressWarnings("all")
public String getSubject() {
return this.subject;
}
/**
* Note to the payer.
*/
@java.lang.SuppressWarnings("all")
public String getNote() {
return this.note;
}
/**
* A flag indicating whether a copy of the email has to be sent to the merchant.
*/
@java.lang.SuppressWarnings("all")
public Boolean getSendToMerchant() {
return this.sendToMerchant;
}
/**
* Subject of the notification.
* @return this
*/
@java.lang.SuppressWarnings("all")
public InvoicingNotification setSubject(final String subject) {
this.subject = subject;
return this;
}
/**
* Note to the payer.
* @return this
*/
@java.lang.SuppressWarnings("all")
public InvoicingNotification setNote(final String note) {
this.note = note;
return this;
}
/**
* A flag indicating whether a copy of the email has to be sent to the merchant.
* @return this
*/
@java.lang.SuppressWarnings("all")
public InvoicingNotification setSendToMerchant(final Boolean sendToMerchant) {
this.sendToMerchant = sendToMerchant;
return this;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof InvoicingNotification)) return false;
final InvoicingNotification other = (InvoicingNotification) o;
if (!other.canEqual((java.lang.Object) this)) return false;
if (!super.equals(o)) return false;
final java.lang.Object this$subject = this.getSubject();
final java.lang.Object other$subject = other.getSubject();
if (this$subject == null ? other$subject != null : !this$subject.equals(other$subject)) return false;
final java.lang.Object this$note = this.getNote();
final java.lang.Object other$note = other.getNote();
if (this$note == null ? other$note != null : !this$note.equals(other$note)) return false;
final java.lang.Object this$sendToMerchant = this.getSendToMerchant();
final java.lang.Object other$sendToMerchant = other.getSendToMerchant();
if (this$sendToMerchant == null ? other$sendToMerchant != null : !this$sendToMerchant.equals(other$sendToMerchant)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
protected boolean canEqual(final java.lang.Object other) {
return other instanceof InvoicingNotification;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public int hashCode() {
final int PRIME = 59;
int result = 1;
result = result * PRIME + super.hashCode();
final java.lang.Object $subject = this.getSubject();
result = result * PRIME + ($subject == null ? 43 : $subject.hashCode());
final java.lang.Object $note = this.getNote();
result = result * PRIME + ($note == null ? 43 : $note.hashCode());
final java.lang.Object $sendToMerchant = this.getSendToMerchant();
result = result * PRIME + ($sendToMerchant == null ? 43 : $sendToMerchant.hashCode());
return result;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy