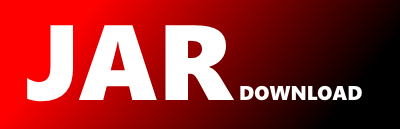
com.paypal.api.payments.InvoicingPaymentDetail Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rest-api-sdk Show documentation
Show all versions of rest-api-sdk Show documentation
PayPal SDK for integrating with the REST APIs
// Generated by delombok at Wed Oct 12 18:15:55 CDT 2016
package com.paypal.api.payments;
import com.paypal.base.rest.PayPalModel;
public class InvoicingPaymentDetail extends PayPalModel {
/**
* PayPal payment detail indicating whether payment was made in an invoicing flow via PayPal or externally. In the case of the mark-as-paid API, payment type is EXTERNAL and this is what is now supported. The PAYPAL value is provided for backward compatibility.
*/
private String type;
/**
* PayPal payment transaction id. Mandatory field in case the type value is PAYPAL.
*/
private String transactionId;
/**
* Type of the transaction.
*/
private String transactionType;
/**
* Date when the invoice was paid. Date format: yyyy-MM-dd z. For example, 2014-02-27 PST.
*/
private String date;
/**
* Payment mode or method. This field is mandatory if the value of the type field is OTHER.
*/
private String method;
/**
* Optional note associated with the payment.
*/
private String note;
/**
* Default Constructor
*/
public InvoicingPaymentDetail() {
}
/**
* Parameterized Constructor
*/
public InvoicingPaymentDetail(String method) {
this.method = method;
}
/**
* PayPal payment detail indicating whether payment was made in an invoicing flow via PayPal or externally. In the case of the mark-as-paid API, payment type is EXTERNAL and this is what is now supported. The PAYPAL value is provided for backward compatibility.
*/
@java.lang.SuppressWarnings("all")
public String getType() {
return this.type;
}
/**
* PayPal payment transaction id. Mandatory field in case the type value is PAYPAL.
*/
@java.lang.SuppressWarnings("all")
public String getTransactionId() {
return this.transactionId;
}
/**
* Type of the transaction.
*/
@java.lang.SuppressWarnings("all")
public String getTransactionType() {
return this.transactionType;
}
/**
* Date when the invoice was paid. Date format: yyyy-MM-dd z. For example, 2014-02-27 PST.
*/
@java.lang.SuppressWarnings("all")
public String getDate() {
return this.date;
}
/**
* Payment mode or method. This field is mandatory if the value of the type field is OTHER.
*/
@java.lang.SuppressWarnings("all")
public String getMethod() {
return this.method;
}
/**
* Optional note associated with the payment.
*/
@java.lang.SuppressWarnings("all")
public String getNote() {
return this.note;
}
/**
* PayPal payment detail indicating whether payment was made in an invoicing flow via PayPal or externally. In the case of the mark-as-paid API, payment type is EXTERNAL and this is what is now supported. The PAYPAL value is provided for backward compatibility.
* @return this
*/
@java.lang.SuppressWarnings("all")
public InvoicingPaymentDetail setType(final String type) {
this.type = type;
return this;
}
/**
* PayPal payment transaction id. Mandatory field in case the type value is PAYPAL.
* @return this
*/
@java.lang.SuppressWarnings("all")
public InvoicingPaymentDetail setTransactionId(final String transactionId) {
this.transactionId = transactionId;
return this;
}
/**
* Type of the transaction.
* @return this
*/
@java.lang.SuppressWarnings("all")
public InvoicingPaymentDetail setTransactionType(final String transactionType) {
this.transactionType = transactionType;
return this;
}
/**
* Date when the invoice was paid. Date format: yyyy-MM-dd z. For example, 2014-02-27 PST.
* @return this
*/
@java.lang.SuppressWarnings("all")
public InvoicingPaymentDetail setDate(final String date) {
this.date = date;
return this;
}
/**
* Payment mode or method. This field is mandatory if the value of the type field is OTHER.
* @return this
*/
@java.lang.SuppressWarnings("all")
public InvoicingPaymentDetail setMethod(final String method) {
this.method = method;
return this;
}
/**
* Optional note associated with the payment.
* @return this
*/
@java.lang.SuppressWarnings("all")
public InvoicingPaymentDetail setNote(final String note) {
this.note = note;
return this;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof InvoicingPaymentDetail)) return false;
final InvoicingPaymentDetail other = (InvoicingPaymentDetail) o;
if (!other.canEqual((java.lang.Object) this)) return false;
if (!super.equals(o)) return false;
final java.lang.Object this$type = this.getType();
final java.lang.Object other$type = other.getType();
if (this$type == null ? other$type != null : !this$type.equals(other$type)) return false;
final java.lang.Object this$transactionId = this.getTransactionId();
final java.lang.Object other$transactionId = other.getTransactionId();
if (this$transactionId == null ? other$transactionId != null : !this$transactionId.equals(other$transactionId)) return false;
final java.lang.Object this$transactionType = this.getTransactionType();
final java.lang.Object other$transactionType = other.getTransactionType();
if (this$transactionType == null ? other$transactionType != null : !this$transactionType.equals(other$transactionType)) return false;
final java.lang.Object this$date = this.getDate();
final java.lang.Object other$date = other.getDate();
if (this$date == null ? other$date != null : !this$date.equals(other$date)) return false;
final java.lang.Object this$method = this.getMethod();
final java.lang.Object other$method = other.getMethod();
if (this$method == null ? other$method != null : !this$method.equals(other$method)) return false;
final java.lang.Object this$note = this.getNote();
final java.lang.Object other$note = other.getNote();
if (this$note == null ? other$note != null : !this$note.equals(other$note)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
protected boolean canEqual(final java.lang.Object other) {
return other instanceof InvoicingPaymentDetail;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public int hashCode() {
final int PRIME = 59;
int result = 1;
result = result * PRIME + super.hashCode();
final java.lang.Object $type = this.getType();
result = result * PRIME + ($type == null ? 43 : $type.hashCode());
final java.lang.Object $transactionId = this.getTransactionId();
result = result * PRIME + ($transactionId == null ? 43 : $transactionId.hashCode());
final java.lang.Object $transactionType = this.getTransactionType();
result = result * PRIME + ($transactionType == null ? 43 : $transactionType.hashCode());
final java.lang.Object $date = this.getDate();
result = result * PRIME + ($date == null ? 43 : $date.hashCode());
final java.lang.Object $method = this.getMethod();
result = result * PRIME + ($method == null ? 43 : $method.hashCode());
final java.lang.Object $note = this.getNote();
result = result * PRIME + ($note == null ? 43 : $note.hashCode());
return result;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy