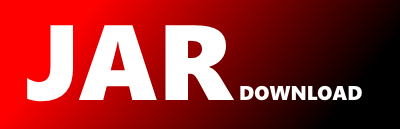
com.paypal.api.payments.ItemList Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rest-api-sdk Show documentation
Show all versions of rest-api-sdk Show documentation
PayPal SDK for integrating with the REST APIs
// Generated by delombok at Wed Oct 12 18:15:55 CDT 2016
package com.paypal.api.payments;
import com.paypal.base.rest.PayPalModel;
import java.util.ArrayList;
import java.util.List;
public class ItemList extends PayPalModel {
/**
* List of items.
*/
private List- items;
/**
* Shipping address.
*/
private ShippingAddress shippingAddress;
/**
* Shipping method used for this payment like USPSParcel etc.
*/
private String shippingMethod;
/**
* Allows merchant's to share payer’s contact number with PayPal for the current payment. Final contact number of payer associated with the transaction might be same as shipping_phone_number or different based on Payer’s action on PayPal. The phone number must be represented in its canonical international format, as defined by the E.164 numbering plan
*/
private String shippingPhoneNumber;
/**
* Default Constructor
*/
public ItemList() {
items = new ArrayList
- ();
}
/**
* List of items.
*/
@java.lang.SuppressWarnings("all")
public List
- getItems() {
return this.items;
}
/**
* Shipping address.
*/
@java.lang.SuppressWarnings("all")
public ShippingAddress getShippingAddress() {
return this.shippingAddress;
}
/**
* Shipping method used for this payment like USPSParcel etc.
*/
@java.lang.SuppressWarnings("all")
public String getShippingMethod() {
return this.shippingMethod;
}
/**
* Allows merchant's to share payer’s contact number with PayPal for the current payment. Final contact number of payer associated with the transaction might be same as shipping_phone_number or different based on Payer’s action on PayPal. The phone number must be represented in its canonical international format, as defined by the E.164 numbering plan
*/
@java.lang.SuppressWarnings("all")
public String getShippingPhoneNumber() {
return this.shippingPhoneNumber;
}
/**
* List of items.
* @return this
*/
@java.lang.SuppressWarnings("all")
public ItemList setItems(final List
- items) {
this.items = items;
return this;
}
/**
* Shipping address.
* @return this
*/
@java.lang.SuppressWarnings("all")
public ItemList setShippingAddress(final ShippingAddress shippingAddress) {
this.shippingAddress = shippingAddress;
return this;
}
/**
* Shipping method used for this payment like USPSParcel etc.
* @return this
*/
@java.lang.SuppressWarnings("all")
public ItemList setShippingMethod(final String shippingMethod) {
this.shippingMethod = shippingMethod;
return this;
}
/**
* Allows merchant's to share payer’s contact number with PayPal for the current payment. Final contact number of payer associated with the transaction might be same as shipping_phone_number or different based on Payer’s action on PayPal. The phone number must be represented in its canonical international format, as defined by the E.164 numbering plan
* @return this
*/
@java.lang.SuppressWarnings("all")
public ItemList setShippingPhoneNumber(final String shippingPhoneNumber) {
this.shippingPhoneNumber = shippingPhoneNumber;
return this;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof ItemList)) return false;
final ItemList other = (ItemList) o;
if (!other.canEqual((java.lang.Object) this)) return false;
if (!super.equals(o)) return false;
final java.lang.Object this$items = this.getItems();
final java.lang.Object other$items = other.getItems();
if (this$items == null ? other$items != null : !this$items.equals(other$items)) return false;
final java.lang.Object this$shippingAddress = this.getShippingAddress();
final java.lang.Object other$shippingAddress = other.getShippingAddress();
if (this$shippingAddress == null ? other$shippingAddress != null : !this$shippingAddress.equals(other$shippingAddress)) return false;
final java.lang.Object this$shippingMethod = this.getShippingMethod();
final java.lang.Object other$shippingMethod = other.getShippingMethod();
if (this$shippingMethod == null ? other$shippingMethod != null : !this$shippingMethod.equals(other$shippingMethod)) return false;
final java.lang.Object this$shippingPhoneNumber = this.getShippingPhoneNumber();
final java.lang.Object other$shippingPhoneNumber = other.getShippingPhoneNumber();
if (this$shippingPhoneNumber == null ? other$shippingPhoneNumber != null : !this$shippingPhoneNumber.equals(other$shippingPhoneNumber)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
protected boolean canEqual(final java.lang.Object other) {
return other instanceof ItemList;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public int hashCode() {
final int PRIME = 59;
int result = 1;
result = result * PRIME + super.hashCode();
final java.lang.Object $items = this.getItems();
result = result * PRIME + ($items == null ? 43 : $items.hashCode());
final java.lang.Object $shippingAddress = this.getShippingAddress();
result = result * PRIME + ($shippingAddress == null ? 43 : $shippingAddress.hashCode());
final java.lang.Object $shippingMethod = this.getShippingMethod();
result = result * PRIME + ($shippingMethod == null ? 43 : $shippingMethod.hashCode());
final java.lang.Object $shippingPhoneNumber = this.getShippingPhoneNumber();
result = result * PRIME + ($shippingPhoneNumber == null ? 43 : $shippingPhoneNumber.hashCode());
return result;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy