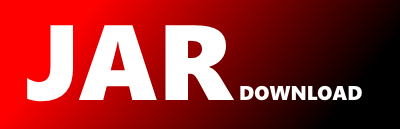
com.paypal.api.payments.PaymentCard Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rest-api-sdk Show documentation
Show all versions of rest-api-sdk Show documentation
PayPal SDK for integrating with the REST APIs
// Generated by delombok at Wed Oct 12 18:15:55 CDT 2016
package com.paypal.api.payments;
import com.paypal.base.rest.PayPalModel;
import java.util.List;
public class PaymentCard extends PayPalModel {
/**
* ID of the credit card being saved for later use.
*/
private String id;
/**
* Card number.
*/
private String number;
/**
* Type of the Card.
*/
private String type;
/**
* 2 digit card expiry month.
*/
private String expireMonth;
/**
* 4 digit card expiry year
*/
private String expireYear;
/**
* 2 digit card start month. Needed for UK Maestro Card.
*/
private String startMonth;
/**
* 4 digit card start year. Needed for UK Maestro Card.
*/
private String startYear;
/**
* Card validation code. Only supported when making a Payment but not when saving a payment card for future use.
*/
private String cvv2;
/**
* Card holder's first name.
*/
private String firstName;
/**
* Card holder's last name.
*/
private String lastName;
/**
* 2 letter country code
*/
private String billingCountry;
/**
* Billing Address associated with this card.
*/
private Address billingAddress;
/**
* A unique identifier of the customer to whom this card account belongs to. Generated and provided by the facilitator. This is required when creating or using a stored funding instrument in vault.
*/
private String externalCustomerId;
/**
* State of the funding instrument.
*/
private String status;
/**
* Date/Time until this resource can be used fund a payment.
*/
private String validUntil;
/**
* 1-2 digit card issue number. Needed for UK Maestro Card.
*/
private String issueNumber;
/**
* Fields required to support 3d secure information when processing credit card payments. Only supported when the `payment_method` is set to `credit_card`.
*/
private Card3dSecureInfo card3dSecureInfo;
/**
*/
private List links;
/**
* Default Constructor
*/
public PaymentCard() {
}
/**
* @deprecated Please use {@link #setCard3dSecureInfo(Card3dSecureInfo)} instead.
*
* Setter for 3dSecureInfo
*/
public PaymentCard set3dSecureInfo(Card3dSecureInfo card3dSecureInfo) {
this.card3dSecureInfo = card3dSecureInfo;
return this;
}
/**
* @deprecated Please use {@link #getCard3dSecureInfo()} instead.
*
* Getter for 3dSecureInfo
*/
public Card3dSecureInfo get3dSecureInfo() {
return this.card3dSecureInfo;
}
/**
* ID of the credit card being saved for later use.
*/
@java.lang.SuppressWarnings("all")
public String getId() {
return this.id;
}
/**
* Card number.
*/
@java.lang.SuppressWarnings("all")
public String getNumber() {
return this.number;
}
/**
* Type of the Card.
*/
@java.lang.SuppressWarnings("all")
public String getType() {
return this.type;
}
/**
* 2 digit card expiry month.
*/
@java.lang.SuppressWarnings("all")
public String getExpireMonth() {
return this.expireMonth;
}
/**
* 4 digit card expiry year
*/
@java.lang.SuppressWarnings("all")
public String getExpireYear() {
return this.expireYear;
}
/**
* 2 digit card start month. Needed for UK Maestro Card.
*/
@java.lang.SuppressWarnings("all")
public String getStartMonth() {
return this.startMonth;
}
/**
* 4 digit card start year. Needed for UK Maestro Card.
*/
@java.lang.SuppressWarnings("all")
public String getStartYear() {
return this.startYear;
}
/**
* Card validation code. Only supported when making a Payment but not when saving a payment card for future use.
*/
@java.lang.SuppressWarnings("all")
public String getCvv2() {
return this.cvv2;
}
/**
* Card holder's first name.
*/
@java.lang.SuppressWarnings("all")
public String getFirstName() {
return this.firstName;
}
/**
* Card holder's last name.
*/
@java.lang.SuppressWarnings("all")
public String getLastName() {
return this.lastName;
}
/**
* 2 letter country code
*/
@java.lang.SuppressWarnings("all")
public String getBillingCountry() {
return this.billingCountry;
}
/**
* Billing Address associated with this card.
*/
@java.lang.SuppressWarnings("all")
public Address getBillingAddress() {
return this.billingAddress;
}
/**
* A unique identifier of the customer to whom this card account belongs to. Generated and provided by the facilitator. This is required when creating or using a stored funding instrument in vault.
*/
@java.lang.SuppressWarnings("all")
public String getExternalCustomerId() {
return this.externalCustomerId;
}
/**
* State of the funding instrument.
*/
@java.lang.SuppressWarnings("all")
public String getStatus() {
return this.status;
}
/**
* Date/Time until this resource can be used fund a payment.
*/
@java.lang.SuppressWarnings("all")
public String getValidUntil() {
return this.validUntil;
}
/**
* 1-2 digit card issue number. Needed for UK Maestro Card.
*/
@java.lang.SuppressWarnings("all")
public String getIssueNumber() {
return this.issueNumber;
}
/**
* Fields required to support 3d secure information when processing credit card payments. Only supported when the `payment_method` is set to `credit_card`.
*/
@java.lang.SuppressWarnings("all")
public Card3dSecureInfo getCard3dSecureInfo() {
return this.card3dSecureInfo;
}
/**
*/
@java.lang.SuppressWarnings("all")
public List getLinks() {
return this.links;
}
/**
* ID of the credit card being saved for later use.
* @return this
*/
@java.lang.SuppressWarnings("all")
public PaymentCard setId(final String id) {
this.id = id;
return this;
}
/**
* Card number.
* @return this
*/
@java.lang.SuppressWarnings("all")
public PaymentCard setNumber(final String number) {
this.number = number;
return this;
}
/**
* Type of the Card.
* @return this
*/
@java.lang.SuppressWarnings("all")
public PaymentCard setType(final String type) {
this.type = type;
return this;
}
/**
* 2 digit card expiry month.
* @return this
*/
@java.lang.SuppressWarnings("all")
public PaymentCard setExpireMonth(final String expireMonth) {
this.expireMonth = expireMonth;
return this;
}
/**
* 4 digit card expiry year
* @return this
*/
@java.lang.SuppressWarnings("all")
public PaymentCard setExpireYear(final String expireYear) {
this.expireYear = expireYear;
return this;
}
/**
* 2 digit card start month. Needed for UK Maestro Card.
* @return this
*/
@java.lang.SuppressWarnings("all")
public PaymentCard setStartMonth(final String startMonth) {
this.startMonth = startMonth;
return this;
}
/**
* 4 digit card start year. Needed for UK Maestro Card.
* @return this
*/
@java.lang.SuppressWarnings("all")
public PaymentCard setStartYear(final String startYear) {
this.startYear = startYear;
return this;
}
/**
* Card validation code. Only supported when making a Payment but not when saving a payment card for future use.
* @return this
*/
@java.lang.SuppressWarnings("all")
public PaymentCard setCvv2(final String cvv2) {
this.cvv2 = cvv2;
return this;
}
/**
* Card holder's first name.
* @return this
*/
@java.lang.SuppressWarnings("all")
public PaymentCard setFirstName(final String firstName) {
this.firstName = firstName;
return this;
}
/**
* Card holder's last name.
* @return this
*/
@java.lang.SuppressWarnings("all")
public PaymentCard setLastName(final String lastName) {
this.lastName = lastName;
return this;
}
/**
* 2 letter country code
* @return this
*/
@java.lang.SuppressWarnings("all")
public PaymentCard setBillingCountry(final String billingCountry) {
this.billingCountry = billingCountry;
return this;
}
/**
* Billing Address associated with this card.
* @return this
*/
@java.lang.SuppressWarnings("all")
public PaymentCard setBillingAddress(final Address billingAddress) {
this.billingAddress = billingAddress;
return this;
}
/**
* A unique identifier of the customer to whom this card account belongs to. Generated and provided by the facilitator. This is required when creating or using a stored funding instrument in vault.
* @return this
*/
@java.lang.SuppressWarnings("all")
public PaymentCard setExternalCustomerId(final String externalCustomerId) {
this.externalCustomerId = externalCustomerId;
return this;
}
/**
* State of the funding instrument.
* @return this
*/
@java.lang.SuppressWarnings("all")
public PaymentCard setStatus(final String status) {
this.status = status;
return this;
}
/**
* Date/Time until this resource can be used fund a payment.
* @return this
*/
@java.lang.SuppressWarnings("all")
public PaymentCard setValidUntil(final String validUntil) {
this.validUntil = validUntil;
return this;
}
/**
* 1-2 digit card issue number. Needed for UK Maestro Card.
* @return this
*/
@java.lang.SuppressWarnings("all")
public PaymentCard setIssueNumber(final String issueNumber) {
this.issueNumber = issueNumber;
return this;
}
/**
* Fields required to support 3d secure information when processing credit card payments. Only supported when the `payment_method` is set to `credit_card`.
* @return this
*/
@java.lang.SuppressWarnings("all")
public PaymentCard setCard3dSecureInfo(final Card3dSecureInfo card3dSecureInfo) {
this.card3dSecureInfo = card3dSecureInfo;
return this;
}
/**
*
* @return this
*/
@java.lang.SuppressWarnings("all")
public PaymentCard setLinks(final List links) {
this.links = links;
return this;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof PaymentCard)) return false;
final PaymentCard other = (PaymentCard) o;
if (!other.canEqual((java.lang.Object) this)) return false;
if (!super.equals(o)) return false;
final java.lang.Object this$id = this.getId();
final java.lang.Object other$id = other.getId();
if (this$id == null ? other$id != null : !this$id.equals(other$id)) return false;
final java.lang.Object this$number = this.getNumber();
final java.lang.Object other$number = other.getNumber();
if (this$number == null ? other$number != null : !this$number.equals(other$number)) return false;
final java.lang.Object this$type = this.getType();
final java.lang.Object other$type = other.getType();
if (this$type == null ? other$type != null : !this$type.equals(other$type)) return false;
final java.lang.Object this$expireMonth = this.getExpireMonth();
final java.lang.Object other$expireMonth = other.getExpireMonth();
if (this$expireMonth == null ? other$expireMonth != null : !this$expireMonth.equals(other$expireMonth)) return false;
final java.lang.Object this$expireYear = this.getExpireYear();
final java.lang.Object other$expireYear = other.getExpireYear();
if (this$expireYear == null ? other$expireYear != null : !this$expireYear.equals(other$expireYear)) return false;
final java.lang.Object this$startMonth = this.getStartMonth();
final java.lang.Object other$startMonth = other.getStartMonth();
if (this$startMonth == null ? other$startMonth != null : !this$startMonth.equals(other$startMonth)) return false;
final java.lang.Object this$startYear = this.getStartYear();
final java.lang.Object other$startYear = other.getStartYear();
if (this$startYear == null ? other$startYear != null : !this$startYear.equals(other$startYear)) return false;
final java.lang.Object this$cvv2 = this.getCvv2();
final java.lang.Object other$cvv2 = other.getCvv2();
if (this$cvv2 == null ? other$cvv2 != null : !this$cvv2.equals(other$cvv2)) return false;
final java.lang.Object this$firstName = this.getFirstName();
final java.lang.Object other$firstName = other.getFirstName();
if (this$firstName == null ? other$firstName != null : !this$firstName.equals(other$firstName)) return false;
final java.lang.Object this$lastName = this.getLastName();
final java.lang.Object other$lastName = other.getLastName();
if (this$lastName == null ? other$lastName != null : !this$lastName.equals(other$lastName)) return false;
final java.lang.Object this$billingCountry = this.getBillingCountry();
final java.lang.Object other$billingCountry = other.getBillingCountry();
if (this$billingCountry == null ? other$billingCountry != null : !this$billingCountry.equals(other$billingCountry)) return false;
final java.lang.Object this$billingAddress = this.getBillingAddress();
final java.lang.Object other$billingAddress = other.getBillingAddress();
if (this$billingAddress == null ? other$billingAddress != null : !this$billingAddress.equals(other$billingAddress)) return false;
final java.lang.Object this$externalCustomerId = this.getExternalCustomerId();
final java.lang.Object other$externalCustomerId = other.getExternalCustomerId();
if (this$externalCustomerId == null ? other$externalCustomerId != null : !this$externalCustomerId.equals(other$externalCustomerId)) return false;
final java.lang.Object this$status = this.getStatus();
final java.lang.Object other$status = other.getStatus();
if (this$status == null ? other$status != null : !this$status.equals(other$status)) return false;
final java.lang.Object this$validUntil = this.getValidUntil();
final java.lang.Object other$validUntil = other.getValidUntil();
if (this$validUntil == null ? other$validUntil != null : !this$validUntil.equals(other$validUntil)) return false;
final java.lang.Object this$issueNumber = this.getIssueNumber();
final java.lang.Object other$issueNumber = other.getIssueNumber();
if (this$issueNumber == null ? other$issueNumber != null : !this$issueNumber.equals(other$issueNumber)) return false;
final java.lang.Object this$card3dSecureInfo = this.getCard3dSecureInfo();
final java.lang.Object other$card3dSecureInfo = other.getCard3dSecureInfo();
if (this$card3dSecureInfo == null ? other$card3dSecureInfo != null : !this$card3dSecureInfo.equals(other$card3dSecureInfo)) return false;
final java.lang.Object this$links = this.getLinks();
final java.lang.Object other$links = other.getLinks();
if (this$links == null ? other$links != null : !this$links.equals(other$links)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
protected boolean canEqual(final java.lang.Object other) {
return other instanceof PaymentCard;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public int hashCode() {
final int PRIME = 59;
int result = 1;
result = result * PRIME + super.hashCode();
final java.lang.Object $id = this.getId();
result = result * PRIME + ($id == null ? 43 : $id.hashCode());
final java.lang.Object $number = this.getNumber();
result = result * PRIME + ($number == null ? 43 : $number.hashCode());
final java.lang.Object $type = this.getType();
result = result * PRIME + ($type == null ? 43 : $type.hashCode());
final java.lang.Object $expireMonth = this.getExpireMonth();
result = result * PRIME + ($expireMonth == null ? 43 : $expireMonth.hashCode());
final java.lang.Object $expireYear = this.getExpireYear();
result = result * PRIME + ($expireYear == null ? 43 : $expireYear.hashCode());
final java.lang.Object $startMonth = this.getStartMonth();
result = result * PRIME + ($startMonth == null ? 43 : $startMonth.hashCode());
final java.lang.Object $startYear = this.getStartYear();
result = result * PRIME + ($startYear == null ? 43 : $startYear.hashCode());
final java.lang.Object $cvv2 = this.getCvv2();
result = result * PRIME + ($cvv2 == null ? 43 : $cvv2.hashCode());
final java.lang.Object $firstName = this.getFirstName();
result = result * PRIME + ($firstName == null ? 43 : $firstName.hashCode());
final java.lang.Object $lastName = this.getLastName();
result = result * PRIME + ($lastName == null ? 43 : $lastName.hashCode());
final java.lang.Object $billingCountry = this.getBillingCountry();
result = result * PRIME + ($billingCountry == null ? 43 : $billingCountry.hashCode());
final java.lang.Object $billingAddress = this.getBillingAddress();
result = result * PRIME + ($billingAddress == null ? 43 : $billingAddress.hashCode());
final java.lang.Object $externalCustomerId = this.getExternalCustomerId();
result = result * PRIME + ($externalCustomerId == null ? 43 : $externalCustomerId.hashCode());
final java.lang.Object $status = this.getStatus();
result = result * PRIME + ($status == null ? 43 : $status.hashCode());
final java.lang.Object $validUntil = this.getValidUntil();
result = result * PRIME + ($validUntil == null ? 43 : $validUntil.hashCode());
final java.lang.Object $issueNumber = this.getIssueNumber();
result = result * PRIME + ($issueNumber == null ? 43 : $issueNumber.hashCode());
final java.lang.Object $card3dSecureInfo = this.getCard3dSecureInfo();
result = result * PRIME + ($card3dSecureInfo == null ? 43 : $card3dSecureInfo.hashCode());
final java.lang.Object $links = this.getLinks();
result = result * PRIME + ($links == null ? 43 : $links.hashCode());
return result;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy