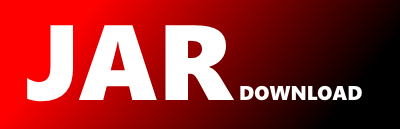
com.paypal.api.payments.PaymentDefinition Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rest-api-sdk Show documentation
Show all versions of rest-api-sdk Show documentation
PayPal SDK for integrating with the REST APIs
// Generated by delombok at Wed Oct 12 18:15:55 CDT 2016
package com.paypal.api.payments;
import com.paypal.base.rest.PayPalModel;
import java.util.List;
public class PaymentDefinition extends PayPalModel {
/**
* Identifier of the payment_definition. 128 characters max.
*/
private String id;
/**
* Name of the payment definition. 128 characters max.
*/
private String name;
/**
* Type of the payment definition. Allowed values: `TRIAL`, `REGULAR`.
*/
private String type;
/**
* How frequently the customer should be charged.
*/
private String frequencyInterval;
/**
* Frequency of the payment definition offered. Allowed values: `WEEK`, `DAY`, `YEAR`, `MONTH`.
*/
private String frequency;
/**
* Number of cycles in this payment definition.
*/
private String cycles;
/**
* Amount that will be charged at the end of each cycle for this payment definition.
*/
private Currency amount;
/**
* Array of charge_models for this payment definition.
*/
private List chargeModels;
/**
* Default Constructor
*/
public PaymentDefinition() {
}
/**
* Parameterized Constructor
*/
public PaymentDefinition(String name, String type, String frequencyInterval, String frequency, String cycles, Currency amount) {
this.name = name;
this.type = type;
this.frequencyInterval = frequencyInterval;
this.frequency = frequency;
this.cycles = cycles;
this.amount = amount;
}
/**
* Identifier of the payment_definition. 128 characters max.
*/
@java.lang.SuppressWarnings("all")
public String getId() {
return this.id;
}
/**
* Name of the payment definition. 128 characters max.
*/
@java.lang.SuppressWarnings("all")
public String getName() {
return this.name;
}
/**
* Type of the payment definition. Allowed values: `TRIAL`, `REGULAR`.
*/
@java.lang.SuppressWarnings("all")
public String getType() {
return this.type;
}
/**
* How frequently the customer should be charged.
*/
@java.lang.SuppressWarnings("all")
public String getFrequencyInterval() {
return this.frequencyInterval;
}
/**
* Frequency of the payment definition offered. Allowed values: `WEEK`, `DAY`, `YEAR`, `MONTH`.
*/
@java.lang.SuppressWarnings("all")
public String getFrequency() {
return this.frequency;
}
/**
* Number of cycles in this payment definition.
*/
@java.lang.SuppressWarnings("all")
public String getCycles() {
return this.cycles;
}
/**
* Amount that will be charged at the end of each cycle for this payment definition.
*/
@java.lang.SuppressWarnings("all")
public Currency getAmount() {
return this.amount;
}
/**
* Array of charge_models for this payment definition.
*/
@java.lang.SuppressWarnings("all")
public List getChargeModels() {
return this.chargeModels;
}
/**
* Identifier of the payment_definition. 128 characters max.
* @return this
*/
@java.lang.SuppressWarnings("all")
public PaymentDefinition setId(final String id) {
this.id = id;
return this;
}
/**
* Name of the payment definition. 128 characters max.
* @return this
*/
@java.lang.SuppressWarnings("all")
public PaymentDefinition setName(final String name) {
this.name = name;
return this;
}
/**
* Type of the payment definition. Allowed values: `TRIAL`, `REGULAR`.
* @return this
*/
@java.lang.SuppressWarnings("all")
public PaymentDefinition setType(final String type) {
this.type = type;
return this;
}
/**
* How frequently the customer should be charged.
* @return this
*/
@java.lang.SuppressWarnings("all")
public PaymentDefinition setFrequencyInterval(final String frequencyInterval) {
this.frequencyInterval = frequencyInterval;
return this;
}
/**
* Frequency of the payment definition offered. Allowed values: `WEEK`, `DAY`, `YEAR`, `MONTH`.
* @return this
*/
@java.lang.SuppressWarnings("all")
public PaymentDefinition setFrequency(final String frequency) {
this.frequency = frequency;
return this;
}
/**
* Number of cycles in this payment definition.
* @return this
*/
@java.lang.SuppressWarnings("all")
public PaymentDefinition setCycles(final String cycles) {
this.cycles = cycles;
return this;
}
/**
* Amount that will be charged at the end of each cycle for this payment definition.
* @return this
*/
@java.lang.SuppressWarnings("all")
public PaymentDefinition setAmount(final Currency amount) {
this.amount = amount;
return this;
}
/**
* Array of charge_models for this payment definition.
* @return this
*/
@java.lang.SuppressWarnings("all")
public PaymentDefinition setChargeModels(final List chargeModels) {
this.chargeModels = chargeModels;
return this;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof PaymentDefinition)) return false;
final PaymentDefinition other = (PaymentDefinition) o;
if (!other.canEqual((java.lang.Object) this)) return false;
if (!super.equals(o)) return false;
final java.lang.Object this$id = this.getId();
final java.lang.Object other$id = other.getId();
if (this$id == null ? other$id != null : !this$id.equals(other$id)) return false;
final java.lang.Object this$name = this.getName();
final java.lang.Object other$name = other.getName();
if (this$name == null ? other$name != null : !this$name.equals(other$name)) return false;
final java.lang.Object this$type = this.getType();
final java.lang.Object other$type = other.getType();
if (this$type == null ? other$type != null : !this$type.equals(other$type)) return false;
final java.lang.Object this$frequencyInterval = this.getFrequencyInterval();
final java.lang.Object other$frequencyInterval = other.getFrequencyInterval();
if (this$frequencyInterval == null ? other$frequencyInterval != null : !this$frequencyInterval.equals(other$frequencyInterval)) return false;
final java.lang.Object this$frequency = this.getFrequency();
final java.lang.Object other$frequency = other.getFrequency();
if (this$frequency == null ? other$frequency != null : !this$frequency.equals(other$frequency)) return false;
final java.lang.Object this$cycles = this.getCycles();
final java.lang.Object other$cycles = other.getCycles();
if (this$cycles == null ? other$cycles != null : !this$cycles.equals(other$cycles)) return false;
final java.lang.Object this$amount = this.getAmount();
final java.lang.Object other$amount = other.getAmount();
if (this$amount == null ? other$amount != null : !this$amount.equals(other$amount)) return false;
final java.lang.Object this$chargeModels = this.getChargeModels();
final java.lang.Object other$chargeModels = other.getChargeModels();
if (this$chargeModels == null ? other$chargeModels != null : !this$chargeModels.equals(other$chargeModels)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
protected boolean canEqual(final java.lang.Object other) {
return other instanceof PaymentDefinition;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public int hashCode() {
final int PRIME = 59;
int result = 1;
result = result * PRIME + super.hashCode();
final java.lang.Object $id = this.getId();
result = result * PRIME + ($id == null ? 43 : $id.hashCode());
final java.lang.Object $name = this.getName();
result = result * PRIME + ($name == null ? 43 : $name.hashCode());
final java.lang.Object $type = this.getType();
result = result * PRIME + ($type == null ? 43 : $type.hashCode());
final java.lang.Object $frequencyInterval = this.getFrequencyInterval();
result = result * PRIME + ($frequencyInterval == null ? 43 : $frequencyInterval.hashCode());
final java.lang.Object $frequency = this.getFrequency();
result = result * PRIME + ($frequency == null ? 43 : $frequency.hashCode());
final java.lang.Object $cycles = this.getCycles();
result = result * PRIME + ($cycles == null ? 43 : $cycles.hashCode());
final java.lang.Object $amount = this.getAmount();
result = result * PRIME + ($amount == null ? 43 : $amount.hashCode());
final java.lang.Object $chargeModels = this.getChargeModels();
result = result * PRIME + ($chargeModels == null ? 43 : $chargeModels.hashCode());
return result;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy