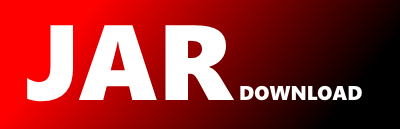
com.paypal.api.payments.PaymentHistory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rest-api-sdk Show documentation
Show all versions of rest-api-sdk Show documentation
PayPal SDK for integrating with the REST APIs
// Generated by delombok at Wed Oct 12 18:15:55 CDT 2016
package com.paypal.api.payments;
import com.paypal.base.rest.PayPalModel;
import java.util.ArrayList;
import java.util.List;
public class PaymentHistory extends PayPalModel {
/**
* A list of Payment resources
*/
private List payments;
/**
* Number of items returned in each range of results. Note that the last results range could have fewer items than the requested number of items.
*/
private int count;
/**
* Identifier of the next element to get the next range of results.
*/
private String nextId;
/**
* Default Constructor
*/
public PaymentHistory() {
payments = new ArrayList();
}
/**
* A list of Payment resources
*/
@java.lang.SuppressWarnings("all")
public List getPayments() {
return this.payments;
}
/**
* Number of items returned in each range of results. Note that the last results range could have fewer items than the requested number of items.
*/
@java.lang.SuppressWarnings("all")
public int getCount() {
return this.count;
}
/**
* Identifier of the next element to get the next range of results.
*/
@java.lang.SuppressWarnings("all")
public String getNextId() {
return this.nextId;
}
/**
* A list of Payment resources
* @return this
*/
@java.lang.SuppressWarnings("all")
public PaymentHistory setPayments(final List payments) {
this.payments = payments;
return this;
}
/**
* Number of items returned in each range of results. Note that the last results range could have fewer items than the requested number of items.
* @return this
*/
@java.lang.SuppressWarnings("all")
public PaymentHistory setCount(final int count) {
this.count = count;
return this;
}
/**
* Identifier of the next element to get the next range of results.
* @return this
*/
@java.lang.SuppressWarnings("all")
public PaymentHistory setNextId(final String nextId) {
this.nextId = nextId;
return this;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof PaymentHistory)) return false;
final PaymentHistory other = (PaymentHistory) o;
if (!other.canEqual((java.lang.Object) this)) return false;
if (!super.equals(o)) return false;
final java.lang.Object this$payments = this.getPayments();
final java.lang.Object other$payments = other.getPayments();
if (this$payments == null ? other$payments != null : !this$payments.equals(other$payments)) return false;
if (this.getCount() != other.getCount()) return false;
final java.lang.Object this$nextId = this.getNextId();
final java.lang.Object other$nextId = other.getNextId();
if (this$nextId == null ? other$nextId != null : !this$nextId.equals(other$nextId)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
protected boolean canEqual(final java.lang.Object other) {
return other instanceof PaymentHistory;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public int hashCode() {
final int PRIME = 59;
int result = 1;
result = result * PRIME + super.hashCode();
final java.lang.Object $payments = this.getPayments();
result = result * PRIME + ($payments == null ? 43 : $payments.hashCode());
result = result * PRIME + this.getCount();
final java.lang.Object $nextId = this.getNextId();
result = result * PRIME + ($nextId == null ? 43 : $nextId.hashCode());
return result;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy