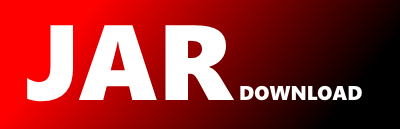
com.paypal.api.payments.Payout Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rest-api-sdk Show documentation
Show all versions of rest-api-sdk Show documentation
PayPal SDK for integrating with the REST APIs
// Generated by delombok at Wed Oct 12 18:15:55 CDT 2016
package com.paypal.api.payments;
import com.paypal.base.rest.*;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class Payout extends PayPalResource {
/**
* The original batch header as provided by the payment sender.
*/
private PayoutSenderBatchHeader senderBatchHeader;
/**
* An array of payout items (that is, a set of individual payouts).
*/
private List items;
/**
*/
private List links;
/**
* Default Constructor
*/
public Payout() {
}
/**
* Parameterized Constructor
*/
public Payout(PayoutSenderBatchHeader senderBatchHeader, List items) {
this.senderBatchHeader = senderBatchHeader;
this.items = items;
}
/**
* You can submit a payout with a synchronous API call, which immediately returns the results of a PayPal payment.
* @deprecated Please use {@link #createSynchronous(APIContext)} instead.
*
* @param accessToken
* Access Token used for the API call.
* @return PayoutBatch
* @throws PayPalRESTException
*/
public PayoutBatch createSynchronous(String accessToken) throws PayPalRESTException {
APIContext apiContext = new APIContext(accessToken);
Map parameters = new HashMap();
parameters.put("sync_mode", "true");
return create(apiContext, parameters);
}
/**
* You can submit a payout with a synchronous API call, which immediately returns the results of a PayPal payment.
*
* @param apiContext
* {@link APIContext} used for the API call.
* @return PayoutBatch
* @throws PayPalRESTException
*/
public PayoutBatch createSynchronous(APIContext apiContext) throws PayPalRESTException {
Map parameters = new HashMap();
parameters.put("sync_mode", "true");
return create(apiContext, parameters);
}
/**
* Create a payout batch resource by passing a sender_batch_header and an
* items array to the request URI. The sender_batch_header contains payout
* parameters that describe the handling of a batch resource while the items
* array conatins payout items.
* @deprecated Please use {@link #create(APIContext, Map)} instead.
*
* @param accessToken
* Access Token used for the API call.
* @param parameters
* Map
* @return PayoutBatch
* @throws PayPalRESTException
*/
public PayoutBatch create(String accessToken, Map parameters) throws PayPalRESTException {
APIContext apiContext = new APIContext(accessToken);
return create(apiContext, parameters);
}
/**
* Create a payout batch resource by passing a sender_batch_header and an
* items array to the request URI. The sender_batch_header contains payout
* parameters that describe the handling of a batch resource while the items
* array conatins payout items.
*
* @param apiContext
* {@link APIContext} used for the API call.
* @param parameters
* Map
* @return PayoutBatch
* @throws PayPalRESTException
*/
public PayoutBatch create(APIContext apiContext, Map parameters) throws PayPalRESTException {
if (parameters == null) {
parameters = new HashMap();
}
Object[] parametersObj = new Object[] {parameters};
String pattern = "v1/payments/payouts?sync_mode={0}";
String resourcePath = RESTUtil.formatURIPath(pattern, parametersObj);
String payLoad = this.toJSON();
return configureAndExecute(apiContext, HttpMethod.POST, resourcePath, payLoad, PayoutBatch.class);
}
/**
* Obtain the status of a specific batch resource by passing the payout
* batch ID to the request URI. You can issue this call multiple times to
* get the current status.
* @deprecated Please use {@link #get(APIContext, String)} instead.
*
* @param accessToken
* Access Token used for the API call.
* @param payoutBatchId
* String
* @return PayoutBatch
* @throws PayPalRESTException
*/
public static PayoutBatch get(String accessToken, String payoutBatchId) throws PayPalRESTException {
APIContext apiContext = new APIContext(accessToken);
return get(apiContext, payoutBatchId);
}
/**
* Obtain the status of a specific batch resource by passing the payout
* batch ID to the request URI. You can issue this call multiple times to
* get the current status.
*
* @param apiContext
* {@link APIContext} used for the API call.
* @param payoutBatchId
* String
* @return PayoutBatch
* @throws PayPalRESTException
*/
public static PayoutBatch get(APIContext apiContext, String payoutBatchId) throws PayPalRESTException {
if (payoutBatchId == null) {
throw new IllegalArgumentException("payoutBatchId cannot be null");
}
Object[] parameters = new Object[] {payoutBatchId};
String pattern = "v1/payments/payouts/{0}";
String resourcePath = RESTUtil.formatURIPath(pattern, parameters);
String payLoad = "";
return configureAndExecute(apiContext, HttpMethod.GET, resourcePath, payLoad, PayoutBatch.class);
}
/**
* The original batch header as provided by the payment sender.
*/
@java.lang.SuppressWarnings("all")
public PayoutSenderBatchHeader getSenderBatchHeader() {
return this.senderBatchHeader;
}
/**
* An array of payout items (that is, a set of individual payouts).
*/
@java.lang.SuppressWarnings("all")
public List getItems() {
return this.items;
}
/**
*/
@java.lang.SuppressWarnings("all")
public List getLinks() {
return this.links;
}
/**
* The original batch header as provided by the payment sender.
* @return this
*/
@java.lang.SuppressWarnings("all")
public Payout setSenderBatchHeader(final PayoutSenderBatchHeader senderBatchHeader) {
this.senderBatchHeader = senderBatchHeader;
return this;
}
/**
* An array of payout items (that is, a set of individual payouts).
* @return this
*/
@java.lang.SuppressWarnings("all")
public Payout setItems(final List items) {
this.items = items;
return this;
}
/**
*
* @return this
*/
@java.lang.SuppressWarnings("all")
public Payout setLinks(final List links) {
this.links = links;
return this;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Payout)) return false;
final Payout other = (Payout) o;
if (!other.canEqual((java.lang.Object) this)) return false;
if (!super.equals(o)) return false;
final java.lang.Object this$senderBatchHeader = this.getSenderBatchHeader();
final java.lang.Object other$senderBatchHeader = other.getSenderBatchHeader();
if (this$senderBatchHeader == null ? other$senderBatchHeader != null : !this$senderBatchHeader.equals(other$senderBatchHeader)) return false;
final java.lang.Object this$items = this.getItems();
final java.lang.Object other$items = other.getItems();
if (this$items == null ? other$items != null : !this$items.equals(other$items)) return false;
final java.lang.Object this$links = this.getLinks();
final java.lang.Object other$links = other.getLinks();
if (this$links == null ? other$links != null : !this$links.equals(other$links)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Payout;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public int hashCode() {
final int PRIME = 59;
int result = 1;
result = result * PRIME + super.hashCode();
final java.lang.Object $senderBatchHeader = this.getSenderBatchHeader();
result = result * PRIME + ($senderBatchHeader == null ? 43 : $senderBatchHeader.hashCode());
final java.lang.Object $items = this.getItems();
result = result * PRIME + ($items == null ? 43 : $items.hashCode());
final java.lang.Object $links = this.getLinks();
result = result * PRIME + ($links == null ? 43 : $links.hashCode());
return result;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy