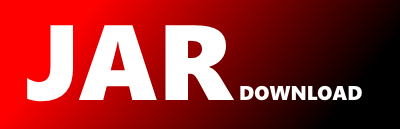
com.paypal.api.payments.PayoutItem Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rest-api-sdk Show documentation
Show all versions of rest-api-sdk Show documentation
PayPal SDK for integrating with the REST APIs
// Generated by delombok at Wed Oct 12 18:15:55 CDT 2016
package com.paypal.api.payments;
import com.paypal.base.rest.*;
public class PayoutItem extends PayPalResource {
/**
* The type of identification for the payment receiver. If this field is
* provided, the payout items without a `recipient_type` will use the
* provided value. If this field is not provided, each payout item must
* include a value for the `recipient_type`.
*/
private String recipientType;
/**
* The amount of money to pay a receiver.
*/
private Currency amount;
/**
* Note for notifications. The note is provided by the payment sender. This
* note can be any string. 4000 characters max.
*/
private String note;
/**
* The receiver of the payment. In a call response, the format of this value
* corresponds to the `recipient_type` specified in the request. 127
* characters max.
*/
private String receiver;
/**
* A sender-specific ID number, used in an accounting system for tracking
* purposes. 30 characters max.
*/
private String senderItemId;
/**
* Default Constructor
*/
public PayoutItem() {
}
/**
* Parameterized Constructor
*/
public PayoutItem(Currency amount, String receiver) {
this.amount = amount;
this.receiver = receiver;
}
/**
* Obtain the status of a payout item by passing the item ID to the request
* URI.
* @deprecated Please use {@link #get(APIContext, String)} instead.
*
* @param accessToken
* Access Token used for the API call.
* @param payoutItemId
* String
* @return PayoutItemDetails
* @throws PayPalRESTException
*/
public static PayoutItemDetails get(String accessToken, String payoutItemId) throws PayPalRESTException {
APIContext apiContext = new APIContext(accessToken);
return get(apiContext, payoutItemId);
}
/**
* Obtain the status of a payout item by passing the item ID to the request
* URI.
*
* @param apiContext
* {@link APIContext} used for the API call.
* @param payoutItemId
* String
* @return PayoutItemDetails
* @throws PayPalRESTException
*/
public static PayoutItemDetails get(APIContext apiContext, String payoutItemId) throws PayPalRESTException {
if (payoutItemId == null) {
throw new IllegalArgumentException("payoutItemId cannot be null");
}
Object[] parameters = new Object[] {payoutItemId};
String pattern = "v1/payments/payouts-item/{0}";
String resourcePath = RESTUtil.formatURIPath(pattern, parameters);
String payLoad = "";
return configureAndExecute(apiContext, HttpMethod.GET, resourcePath, payLoad, PayoutItemDetails.class);
}
/**
* Cancels the unclaimed payment using the items id passed in the request
* URI. If an unclaimed item is not claimed within 30 days, the funds will
* be automatically returned to the sender. This call can be used to cancel
* the unclaimed item prior to the automatic 30-day return.
* @deprecated Please use {@link #cancel(APIContext, String)} instead.
*
* @param accessToken
* Access Token used for the API call.
* @param payoutItemId
* String
* @return PayoutItemDetails
* @throws PayPalRESTException
*/
public static PayoutItemDetails cancel(String accessToken, String payoutItemId) throws PayPalRESTException {
APIContext apiContext = new APIContext(accessToken);
return cancel(apiContext, payoutItemId);
}
/**
* Cancels the unclaimed payment using the items id passed in the request
* URI. If an unclaimed item is not claimed within 30 days, the funds will
* be automatically returned to the sender. This call can be used to cancel
* the unclaimed item prior to the automatic 30-day return.
*
* @param apiContext
* {@link APIContext} used for the API call.
* @param payoutItemId
* String
* @return PayoutItemDetails
* @throws PayPalRESTException
*/
public static PayoutItemDetails cancel(APIContext apiContext, String payoutItemId) throws PayPalRESTException {
if (payoutItemId == null) {
throw new IllegalArgumentException("payoutItemId cannot be null");
}
Object[] parameters = new Object[] {payoutItemId};
String pattern = "v1/payments/payouts-item/{0}/cancel";
String resourcePath = RESTUtil.formatURIPath(pattern, parameters);
String payLoad = "";
return configureAndExecute(apiContext, HttpMethod.POST, resourcePath, payLoad, PayoutItemDetails.class);
}
/**
* The type of identification for the payment receiver. If this field is
* provided, the payout items without a `recipient_type` will use the
* provided value. If this field is not provided, each payout item must
* include a value for the `recipient_type`.
*/
@java.lang.SuppressWarnings("all")
public String getRecipientType() {
return this.recipientType;
}
/**
* The amount of money to pay a receiver.
*/
@java.lang.SuppressWarnings("all")
public Currency getAmount() {
return this.amount;
}
/**
* Note for notifications. The note is provided by the payment sender. This
* note can be any string. 4000 characters max.
*/
@java.lang.SuppressWarnings("all")
public String getNote() {
return this.note;
}
/**
* The receiver of the payment. In a call response, the format of this value
* corresponds to the `recipient_type` specified in the request. 127
* characters max.
*/
@java.lang.SuppressWarnings("all")
public String getReceiver() {
return this.receiver;
}
/**
* A sender-specific ID number, used in an accounting system for tracking
* purposes. 30 characters max.
*/
@java.lang.SuppressWarnings("all")
public String getSenderItemId() {
return this.senderItemId;
}
/**
* The type of identification for the payment receiver. If this field is
* provided, the payout items without a `recipient_type` will use the
* provided value. If this field is not provided, each payout item must
* include a value for the `recipient_type`.
* @return this
*/
@java.lang.SuppressWarnings("all")
public PayoutItem setRecipientType(final String recipientType) {
this.recipientType = recipientType;
return this;
}
/**
* The amount of money to pay a receiver.
* @return this
*/
@java.lang.SuppressWarnings("all")
public PayoutItem setAmount(final Currency amount) {
this.amount = amount;
return this;
}
/**
* Note for notifications. The note is provided by the payment sender. This
* note can be any string. 4000 characters max.
* @return this
*/
@java.lang.SuppressWarnings("all")
public PayoutItem setNote(final String note) {
this.note = note;
return this;
}
/**
* The receiver of the payment. In a call response, the format of this value
* corresponds to the `recipient_type` specified in the request. 127
* characters max.
* @return this
*/
@java.lang.SuppressWarnings("all")
public PayoutItem setReceiver(final String receiver) {
this.receiver = receiver;
return this;
}
/**
* A sender-specific ID number, used in an accounting system for tracking
* purposes. 30 characters max.
* @return this
*/
@java.lang.SuppressWarnings("all")
public PayoutItem setSenderItemId(final String senderItemId) {
this.senderItemId = senderItemId;
return this;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof PayoutItem)) return false;
final PayoutItem other = (PayoutItem) o;
if (!other.canEqual((java.lang.Object) this)) return false;
if (!super.equals(o)) return false;
final java.lang.Object this$recipientType = this.getRecipientType();
final java.lang.Object other$recipientType = other.getRecipientType();
if (this$recipientType == null ? other$recipientType != null : !this$recipientType.equals(other$recipientType)) return false;
final java.lang.Object this$amount = this.getAmount();
final java.lang.Object other$amount = other.getAmount();
if (this$amount == null ? other$amount != null : !this$amount.equals(other$amount)) return false;
final java.lang.Object this$note = this.getNote();
final java.lang.Object other$note = other.getNote();
if (this$note == null ? other$note != null : !this$note.equals(other$note)) return false;
final java.lang.Object this$receiver = this.getReceiver();
final java.lang.Object other$receiver = other.getReceiver();
if (this$receiver == null ? other$receiver != null : !this$receiver.equals(other$receiver)) return false;
final java.lang.Object this$senderItemId = this.getSenderItemId();
final java.lang.Object other$senderItemId = other.getSenderItemId();
if (this$senderItemId == null ? other$senderItemId != null : !this$senderItemId.equals(other$senderItemId)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
protected boolean canEqual(final java.lang.Object other) {
return other instanceof PayoutItem;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public int hashCode() {
final int PRIME = 59;
int result = 1;
result = result * PRIME + super.hashCode();
final java.lang.Object $recipientType = this.getRecipientType();
result = result * PRIME + ($recipientType == null ? 43 : $recipientType.hashCode());
final java.lang.Object $amount = this.getAmount();
result = result * PRIME + ($amount == null ? 43 : $amount.hashCode());
final java.lang.Object $note = this.getNote();
result = result * PRIME + ($note == null ? 43 : $note.hashCode());
final java.lang.Object $receiver = this.getReceiver();
result = result * PRIME + ($receiver == null ? 43 : $receiver.hashCode());
final java.lang.Object $senderItemId = this.getSenderItemId();
result = result * PRIME + ($senderItemId == null ? 43 : $senderItemId.hashCode());
return result;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy