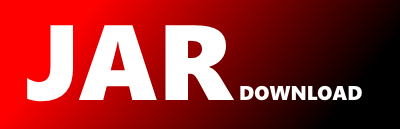
com.paypal.api.payments.RecipientBankingInstruction Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rest-api-sdk Show documentation
Show all versions of rest-api-sdk Show documentation
PayPal SDK for integrating with the REST APIs
// Generated by delombok at Wed Oct 12 18:15:55 CDT 2016
package com.paypal.api.payments;
import com.paypal.base.rest.PayPalModel;
public class RecipientBankingInstruction extends PayPalModel {
/**
* Name of the financial institution.
*/
private String bankName;
/**
* Name of the account holder
*/
private String accountHolderName;
/**
* bank account number
*/
private String accountNumber;
/**
* bank routing number
*/
private String routingNumber;
/**
* IBAN equivalent of the bank
*/
private String internationalBankAccountNumber;
/**
* BIC identifier of the financial institution
*/
private String bankIdentifierCode;
/**
* Default Constructor
*/
public RecipientBankingInstruction() {
}
/**
* Parameterized Constructor
*/
public RecipientBankingInstruction(String bankName, String accountHolderName, String internationalBankAccountNumber) {
this.bankName = bankName;
this.accountHolderName = accountHolderName;
this.internationalBankAccountNumber = internationalBankAccountNumber;
}
/**
* Name of the financial institution.
*/
@java.lang.SuppressWarnings("all")
public String getBankName() {
return this.bankName;
}
/**
* Name of the account holder
*/
@java.lang.SuppressWarnings("all")
public String getAccountHolderName() {
return this.accountHolderName;
}
/**
* bank account number
*/
@java.lang.SuppressWarnings("all")
public String getAccountNumber() {
return this.accountNumber;
}
/**
* bank routing number
*/
@java.lang.SuppressWarnings("all")
public String getRoutingNumber() {
return this.routingNumber;
}
/**
* IBAN equivalent of the bank
*/
@java.lang.SuppressWarnings("all")
public String getInternationalBankAccountNumber() {
return this.internationalBankAccountNumber;
}
/**
* BIC identifier of the financial institution
*/
@java.lang.SuppressWarnings("all")
public String getBankIdentifierCode() {
return this.bankIdentifierCode;
}
/**
* Name of the financial institution.
* @return this
*/
@java.lang.SuppressWarnings("all")
public RecipientBankingInstruction setBankName(final String bankName) {
this.bankName = bankName;
return this;
}
/**
* Name of the account holder
* @return this
*/
@java.lang.SuppressWarnings("all")
public RecipientBankingInstruction setAccountHolderName(final String accountHolderName) {
this.accountHolderName = accountHolderName;
return this;
}
/**
* bank account number
* @return this
*/
@java.lang.SuppressWarnings("all")
public RecipientBankingInstruction setAccountNumber(final String accountNumber) {
this.accountNumber = accountNumber;
return this;
}
/**
* bank routing number
* @return this
*/
@java.lang.SuppressWarnings("all")
public RecipientBankingInstruction setRoutingNumber(final String routingNumber) {
this.routingNumber = routingNumber;
return this;
}
/**
* IBAN equivalent of the bank
* @return this
*/
@java.lang.SuppressWarnings("all")
public RecipientBankingInstruction setInternationalBankAccountNumber(final String internationalBankAccountNumber) {
this.internationalBankAccountNumber = internationalBankAccountNumber;
return this;
}
/**
* BIC identifier of the financial institution
* @return this
*/
@java.lang.SuppressWarnings("all")
public RecipientBankingInstruction setBankIdentifierCode(final String bankIdentifierCode) {
this.bankIdentifierCode = bankIdentifierCode;
return this;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof RecipientBankingInstruction)) return false;
final RecipientBankingInstruction other = (RecipientBankingInstruction) o;
if (!other.canEqual((java.lang.Object) this)) return false;
if (!super.equals(o)) return false;
final java.lang.Object this$bankName = this.getBankName();
final java.lang.Object other$bankName = other.getBankName();
if (this$bankName == null ? other$bankName != null : !this$bankName.equals(other$bankName)) return false;
final java.lang.Object this$accountHolderName = this.getAccountHolderName();
final java.lang.Object other$accountHolderName = other.getAccountHolderName();
if (this$accountHolderName == null ? other$accountHolderName != null : !this$accountHolderName.equals(other$accountHolderName)) return false;
final java.lang.Object this$accountNumber = this.getAccountNumber();
final java.lang.Object other$accountNumber = other.getAccountNumber();
if (this$accountNumber == null ? other$accountNumber != null : !this$accountNumber.equals(other$accountNumber)) return false;
final java.lang.Object this$routingNumber = this.getRoutingNumber();
final java.lang.Object other$routingNumber = other.getRoutingNumber();
if (this$routingNumber == null ? other$routingNumber != null : !this$routingNumber.equals(other$routingNumber)) return false;
final java.lang.Object this$internationalBankAccountNumber = this.getInternationalBankAccountNumber();
final java.lang.Object other$internationalBankAccountNumber = other.getInternationalBankAccountNumber();
if (this$internationalBankAccountNumber == null ? other$internationalBankAccountNumber != null : !this$internationalBankAccountNumber.equals(other$internationalBankAccountNumber)) return false;
final java.lang.Object this$bankIdentifierCode = this.getBankIdentifierCode();
final java.lang.Object other$bankIdentifierCode = other.getBankIdentifierCode();
if (this$bankIdentifierCode == null ? other$bankIdentifierCode != null : !this$bankIdentifierCode.equals(other$bankIdentifierCode)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
protected boolean canEqual(final java.lang.Object other) {
return other instanceof RecipientBankingInstruction;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public int hashCode() {
final int PRIME = 59;
int result = 1;
result = result * PRIME + super.hashCode();
final java.lang.Object $bankName = this.getBankName();
result = result * PRIME + ($bankName == null ? 43 : $bankName.hashCode());
final java.lang.Object $accountHolderName = this.getAccountHolderName();
result = result * PRIME + ($accountHolderName == null ? 43 : $accountHolderName.hashCode());
final java.lang.Object $accountNumber = this.getAccountNumber();
result = result * PRIME + ($accountNumber == null ? 43 : $accountNumber.hashCode());
final java.lang.Object $routingNumber = this.getRoutingNumber();
result = result * PRIME + ($routingNumber == null ? 43 : $routingNumber.hashCode());
final java.lang.Object $internationalBankAccountNumber = this.getInternationalBankAccountNumber();
result = result * PRIME + ($internationalBankAccountNumber == null ? 43 : $internationalBankAccountNumber.hashCode());
final java.lang.Object $bankIdentifierCode = this.getBankIdentifierCode();
result = result * PRIME + ($bankIdentifierCode == null ? 43 : $bankIdentifierCode.hashCode());
return result;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy