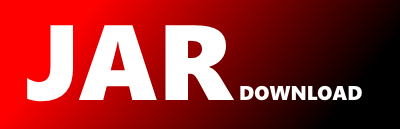
com.paypal.api.payments.ShippingAddress Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rest-api-sdk Show documentation
Show all versions of rest-api-sdk Show documentation
PayPal SDK for integrating with the REST APIs
// Generated by delombok at Wed Oct 12 18:15:55 CDT 2016
package com.paypal.api.payments;
public class ShippingAddress extends Address {
/**
* Address ID assigned in PayPal system.
*/
private String id;
/**
* Name of the recipient at this address.
*/
private String recipientName;
/**
* Default shipping address of the Payer.
*/
private Boolean defaultAddress;
/**
* Default Constructor
*/
public ShippingAddress() {
}
/**
* Parameterized Constructor
*/
public ShippingAddress(String recipientName) {
this.recipientName = recipientName;
}
/**
* Address ID assigned in PayPal system.
*/
@java.lang.SuppressWarnings("all")
public String getId() {
return this.id;
}
/**
* Name of the recipient at this address.
*/
@java.lang.SuppressWarnings("all")
public String getRecipientName() {
return this.recipientName;
}
/**
* Default shipping address of the Payer.
*/
@java.lang.SuppressWarnings("all")
public Boolean getDefaultAddress() {
return this.defaultAddress;
}
/**
* Address ID assigned in PayPal system.
* @return this
*/
@java.lang.SuppressWarnings("all")
public ShippingAddress setId(final String id) {
this.id = id;
return this;
}
/**
* Name of the recipient at this address.
* @return this
*/
@java.lang.SuppressWarnings("all")
public ShippingAddress setRecipientName(final String recipientName) {
this.recipientName = recipientName;
return this;
}
/**
* Default shipping address of the Payer.
* @return this
*/
@java.lang.SuppressWarnings("all")
public ShippingAddress setDefaultAddress(final Boolean defaultAddress) {
this.defaultAddress = defaultAddress;
return this;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof ShippingAddress)) return false;
final ShippingAddress other = (ShippingAddress) o;
if (!other.canEqual((java.lang.Object) this)) return false;
if (!super.equals(o)) return false;
final java.lang.Object this$id = this.getId();
final java.lang.Object other$id = other.getId();
if (this$id == null ? other$id != null : !this$id.equals(other$id)) return false;
final java.lang.Object this$recipientName = this.getRecipientName();
final java.lang.Object other$recipientName = other.getRecipientName();
if (this$recipientName == null ? other$recipientName != null : !this$recipientName.equals(other$recipientName)) return false;
final java.lang.Object this$defaultAddress = this.getDefaultAddress();
final java.lang.Object other$defaultAddress = other.getDefaultAddress();
if (this$defaultAddress == null ? other$defaultAddress != null : !this$defaultAddress.equals(other$defaultAddress)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
protected boolean canEqual(final java.lang.Object other) {
return other instanceof ShippingAddress;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public int hashCode() {
final int PRIME = 59;
int result = 1;
result = result * PRIME + super.hashCode();
final java.lang.Object $id = this.getId();
result = result * PRIME + ($id == null ? 43 : $id.hashCode());
final java.lang.Object $recipientName = this.getRecipientName();
result = result * PRIME + ($recipientName == null ? 43 : $recipientName.hashCode());
final java.lang.Object $defaultAddress = this.getDefaultAddress();
result = result * PRIME + ($defaultAddress == null ? 43 : $defaultAddress.hashCode());
return result;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy