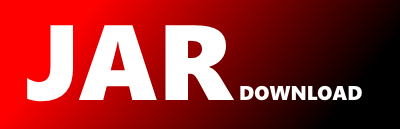
com.paypal.api.payments.Templates Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rest-api-sdk Show documentation
Show all versions of rest-api-sdk Show documentation
PayPal SDK for integrating with the REST APIs
// Generated by delombok at Wed Oct 12 18:15:55 CDT 2016
package com.paypal.api.payments;
import com.paypal.base.rest.*;
import java.util.List;
public class Templates extends PayPalResource {
/**
* List of addresses in merchant's profile.
*/
private List addresses;
/**
* List of emails in merchant's profile.
*/
private List emails;
/**
* List of phone numbers in merchant's profile.
*/
private List phones;
/**
* Array of templates.
*/
private List templates;
/**
* HATEOS links representing all the actions over the template list returned.
*/
private List links;
/**
* Default Constructor
*/
public Templates() {
}
/**
* Retrieve the details for a particular template by passing the template ID to the request URI.
* @deprecated Please use {@link Template#get(APIContext, String)} instead.
* @param apiContext
* {@link APIContext} used for the API call.
* @param templateId
* String
* @return Template
* @throws PayPalRESTException
*/
public static Template get(APIContext apiContext, String templateId) throws PayPalRESTException {
return Template.get(apiContext, templateId);
}
/**
* Retrieves the template information of the merchant.
* @param apiContext
* {@link APIContext} used for the API call.
* @return Templates
* @throws PayPalRESTException
*/
public static Templates getAll(APIContext apiContext) throws PayPalRESTException {
String resourcePath = "v1/invoicing/templates";
String payLoad = "";
return configureAndExecute(apiContext, HttpMethod.GET, resourcePath, payLoad, Templates.class);
}
/**
* List of addresses in merchant's profile.
*/
@java.lang.SuppressWarnings("all")
public List getAddresses() {
return this.addresses;
}
/**
* List of emails in merchant's profile.
*/
@java.lang.SuppressWarnings("all")
public List getEmails() {
return this.emails;
}
/**
* List of phone numbers in merchant's profile.
*/
@java.lang.SuppressWarnings("all")
public List getPhones() {
return this.phones;
}
/**
* Array of templates.
*/
@java.lang.SuppressWarnings("all")
public List getTemplates() {
return this.templates;
}
/**
* HATEOS links representing all the actions over the template list returned.
*/
@java.lang.SuppressWarnings("all")
public List getLinks() {
return this.links;
}
/**
* List of addresses in merchant's profile.
* @return this
*/
@java.lang.SuppressWarnings("all")
public Templates setAddresses(final List addresses) {
this.addresses = addresses;
return this;
}
/**
* List of emails in merchant's profile.
* @return this
*/
@java.lang.SuppressWarnings("all")
public Templates setEmails(final List emails) {
this.emails = emails;
return this;
}
/**
* List of phone numbers in merchant's profile.
* @return this
*/
@java.lang.SuppressWarnings("all")
public Templates setPhones(final List phones) {
this.phones = phones;
return this;
}
/**
* Array of templates.
* @return this
*/
@java.lang.SuppressWarnings("all")
public Templates setTemplates(final List templates) {
this.templates = templates;
return this;
}
/**
* HATEOS links representing all the actions over the template list returned.
* @return this
*/
@java.lang.SuppressWarnings("all")
public Templates setLinks(final List links) {
this.links = links;
return this;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Templates)) return false;
final Templates other = (Templates) o;
if (!other.canEqual((java.lang.Object) this)) return false;
if (!super.equals(o)) return false;
final java.lang.Object this$addresses = this.getAddresses();
final java.lang.Object other$addresses = other.getAddresses();
if (this$addresses == null ? other$addresses != null : !this$addresses.equals(other$addresses)) return false;
final java.lang.Object this$emails = this.getEmails();
final java.lang.Object other$emails = other.getEmails();
if (this$emails == null ? other$emails != null : !this$emails.equals(other$emails)) return false;
final java.lang.Object this$phones = this.getPhones();
final java.lang.Object other$phones = other.getPhones();
if (this$phones == null ? other$phones != null : !this$phones.equals(other$phones)) return false;
final java.lang.Object this$templates = this.getTemplates();
final java.lang.Object other$templates = other.getTemplates();
if (this$templates == null ? other$templates != null : !this$templates.equals(other$templates)) return false;
final java.lang.Object this$links = this.getLinks();
final java.lang.Object other$links = other.getLinks();
if (this$links == null ? other$links != null : !this$links.equals(other$links)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Templates;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public int hashCode() {
final int PRIME = 59;
int result = 1;
result = result * PRIME + super.hashCode();
final java.lang.Object $addresses = this.getAddresses();
result = result * PRIME + ($addresses == null ? 43 : $addresses.hashCode());
final java.lang.Object $emails = this.getEmails();
result = result * PRIME + ($emails == null ? 43 : $emails.hashCode());
final java.lang.Object $phones = this.getPhones();
result = result * PRIME + ($phones == null ? 43 : $phones.hashCode());
final java.lang.Object $templates = this.getTemplates();
result = result * PRIME + ($templates == null ? 43 : $templates.hashCode());
final java.lang.Object $links = this.getLinks();
result = result * PRIME + ($links == null ? 43 : $links.hashCode());
return result;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy